Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / Utils / Boolean / DomainConstraint.cs / 1305376 / DomainConstraint.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Diagnostics; namespace System.Data.Common.Utils.Boolean { ////// Represents a variable with finite domain, e.g., c in {1, 2, 3} /// ///Type of domain variables (int in the above example). ///Type of the identifier (c above -- it need not be int). internal class DomainVariable{ private readonly T_Variable _identifier; private readonly Set _domain; private readonly int _hashCode; private readonly IEqualityComparer _identifierComparer; /// /// Constructs a new domain variable. /// /// Identifier /// Domain of variable. /// Comparer of identifier internal DomainVariable(T_Variable identifier, Setdomain, IEqualityComparer identifierComparer) { Debug.Assert(null != identifier && null != domain); _identifier = identifier; _domain = domain.AsReadOnly(); _identifierComparer = identifierComparer ?? EqualityComparer .Default; int domainHashCode = _domain.GetElementsHashCode(); int identifierHashCode = _identifierComparer.GetHashCode(_identifier); _hashCode = domainHashCode ^ identifierHashCode; } internal DomainVariable(T_Variable identifier, Set domain) : this(identifier, domain, null) { } /// /// Gets the variable. /// internal T_Variable Identifier { get { return _identifier; } } ////// Gets the domain of this variable. /// internal SetDomain { get { return _domain; } } public override int GetHashCode() { return _hashCode; } public override bool Equals(object obj) { if (Object.ReferenceEquals(this, obj)) { return true; } DomainVariable other = obj as DomainVariable ; if (null == other) { return false; } if (_hashCode != other._hashCode) { return false; } return (_identifierComparer.Equals(_identifier, other._identifier) && _domain.SetEquals(other._domain)); } public override string ToString() { return StringUtil.FormatInvariant("{0}{{{1}}}", _identifier.ToString(), _domain); } } /// /// Represents a constraint of the form: /// /// Var1 in Range /// ///Type of range elements. ///Type of the variable. internal class DomainConstraint{ private readonly DomainVariable _variable; private readonly Set _range; private readonly int _hashCode; /// /// Constructs a new constraint for the given variable and range. /// /// Variable in constraint. /// Range of constraint. internal DomainConstraint(DomainVariablevariable, Set range) { Debug.Assert(null != variable && null != range); _variable = variable; _range = range.AsReadOnly(); _hashCode = _variable.GetHashCode() ^ _range.GetElementsHashCode(); } /// /// Constructor supporting a singleton range domain constraint /// internal DomainConstraint(DomainVariablevariable, T_Element element) : this(variable, new Set (new T_Element[] { element }).MakeReadOnly()) { } /// /// Gets the variable for this constraint. /// internal DomainVariableVariable { get { return _variable; } } /// /// Get the range for this constraint. /// internal SetRange { get { return _range; } } /// /// Inverts this constraint (this iff. !result) /// !(Var in Range) iff. Var in (Var.Domain - Range) /// ///internal DomainConstraint InvertDomainConstraint() { return new DomainConstraint (_variable, _variable.Domain.Difference(_range).AsReadOnly()); } public override bool Equals(object obj) { if (Object.ReferenceEquals(this, obj)) { return true; } DomainConstraint other = obj as DomainConstraint ; if (null == other) { return false; } if (_hashCode != other._hashCode) { return false; } return (_range.SetEquals(other._range) && _variable.Equals(other._variable)); } public override int GetHashCode() { return _hashCode; } public override string ToString() { return StringUtil.FormatInvariant("{0} in [{1}]", _variable, _range); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Diagnostics; namespace System.Data.Common.Utils.Boolean { ////// Represents a variable with finite domain, e.g., c in {1, 2, 3} /// ///Type of domain variables (int in the above example). ///Type of the identifier (c above -- it need not be int). internal class DomainVariable{ private readonly T_Variable _identifier; private readonly Set _domain; private readonly int _hashCode; private readonly IEqualityComparer _identifierComparer; /// /// Constructs a new domain variable. /// /// Identifier /// Domain of variable. /// Comparer of identifier internal DomainVariable(T_Variable identifier, Setdomain, IEqualityComparer identifierComparer) { Debug.Assert(null != identifier && null != domain); _identifier = identifier; _domain = domain.AsReadOnly(); _identifierComparer = identifierComparer ?? EqualityComparer .Default; int domainHashCode = _domain.GetElementsHashCode(); int identifierHashCode = _identifierComparer.GetHashCode(_identifier); _hashCode = domainHashCode ^ identifierHashCode; } internal DomainVariable(T_Variable identifier, Set domain) : this(identifier, domain, null) { } /// /// Gets the variable. /// internal T_Variable Identifier { get { return _identifier; } } ////// Gets the domain of this variable. /// internal SetDomain { get { return _domain; } } public override int GetHashCode() { return _hashCode; } public override bool Equals(object obj) { if (Object.ReferenceEquals(this, obj)) { return true; } DomainVariable other = obj as DomainVariable ; if (null == other) { return false; } if (_hashCode != other._hashCode) { return false; } return (_identifierComparer.Equals(_identifier, other._identifier) && _domain.SetEquals(other._domain)); } public override string ToString() { return StringUtil.FormatInvariant("{0}{{{1}}}", _identifier.ToString(), _domain); } } /// /// Represents a constraint of the form: /// /// Var1 in Range /// ///Type of range elements. ///Type of the variable. internal class DomainConstraint{ private readonly DomainVariable _variable; private readonly Set _range; private readonly int _hashCode; /// /// Constructs a new constraint for the given variable and range. /// /// Variable in constraint. /// Range of constraint. internal DomainConstraint(DomainVariablevariable, Set range) { Debug.Assert(null != variable && null != range); _variable = variable; _range = range.AsReadOnly(); _hashCode = _variable.GetHashCode() ^ _range.GetElementsHashCode(); } /// /// Constructor supporting a singleton range domain constraint /// internal DomainConstraint(DomainVariablevariable, T_Element element) : this(variable, new Set (new T_Element[] { element }).MakeReadOnly()) { } /// /// Gets the variable for this constraint. /// internal DomainVariableVariable { get { return _variable; } } /// /// Get the range for this constraint. /// internal SetRange { get { return _range; } } /// /// Inverts this constraint (this iff. !result) /// !(Var in Range) iff. Var in (Var.Domain - Range) /// ///internal DomainConstraint InvertDomainConstraint() { return new DomainConstraint (_variable, _variable.Domain.Difference(_range).AsReadOnly()); } public override bool Equals(object obj) { if (Object.ReferenceEquals(this, obj)) { return true; } DomainConstraint other = obj as DomainConstraint ; if (null == other) { return false; } if (_hashCode != other._hashCode) { return false; } return (_range.SetEquals(other._range) && _variable.Equals(other._variable)); } public override int GetHashCode() { return _hashCode; } public override string ToString() { return StringUtil.FormatInvariant("{0} in [{1}]", _variable, _range); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
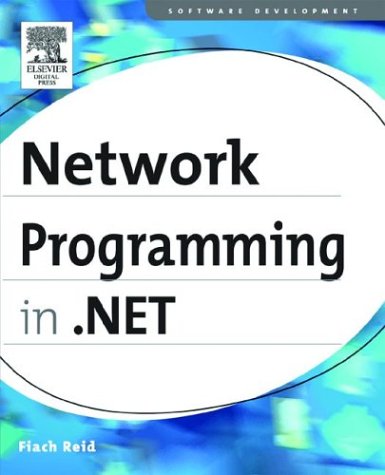
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SslStream.cs
- Color.cs
- SoapTypeAttribute.cs
- SQLDateTimeStorage.cs
- DataGridViewCellStyle.cs
- LineProperties.cs
- PreservationFileReader.cs
- DataGridSortingEventArgs.cs
- ImmutableObjectAttribute.cs
- BasicKeyConstraint.cs
- QueryOpeningEnumerator.cs
- CombinedTcpChannel.cs
- SmtpDateTime.cs
- OperationAbortedException.cs
- EntityDataSourceColumn.cs
- PropertyTabChangedEvent.cs
- BackoffTimeoutHelper.cs
- MenuItemStyle.cs
- ResXBuildProvider.cs
- UTF8Encoding.cs
- MetadataItem_Static.cs
- TreeNodeStyleCollection.cs
- DataGridViewSelectedColumnCollection.cs
- FieldBuilder.cs
- HtmlElement.cs
- StringFunctions.cs
- Oid.cs
- ByteStack.cs
- PagesChangedEventArgs.cs
- DrawingContextDrawingContextWalker.cs
- ISAPIWorkerRequest.cs
- TimeSpanSecondsConverter.cs
- AppDomainProtocolHandler.cs
- Math.cs
- RectAnimationBase.cs
- FastEncoderWindow.cs
- ItemList.cs
- MergeLocalizationDirectives.cs
- DrawingDrawingContext.cs
- BoundColumn.cs
- ControlLocalizer.cs
- CardSpacePolicyElement.cs
- ZipIOExtraFieldPaddingElement.cs
- DbParameterHelper.cs
- HtmlFormWrapper.cs
- WebPartEditorCancelVerb.cs
- EventProviderTraceListener.cs
- X509ChainPolicy.cs
- SecurityUtils.cs
- FontCacheUtil.cs
- NumberFormatter.cs
- TextPenaltyModule.cs
- DBDataPermissionAttribute.cs
- TemplateApplicationHelper.cs
- ConfigUtil.cs
- Variable.cs
- LeftCellWrapper.cs
- SqlConnectionHelper.cs
- XmlSerializerAssemblyAttribute.cs
- DataGridLinkButton.cs
- CommonDialog.cs
- DateTimeOffsetAdapter.cs
- SemaphoreFullException.cs
- Splitter.cs
- RegionData.cs
- AudioLevelUpdatedEventArgs.cs
- SymLanguageType.cs
- FrameworkEventSource.cs
- SystemResourceHost.cs
- PasswordTextNavigator.cs
- SchemaElement.cs
- PageRequestManager.cs
- DependentList.cs
- ErrorFormatterPage.cs
- StylusPointProperties.cs
- MetadataHelper.cs
- SoapIgnoreAttribute.cs
- ClassImporter.cs
- LineServicesCallbacks.cs
- TemplateBindingExpression.cs
- TextDecoration.cs
- SimplePropertyEntry.cs
- DefaultMemberAttribute.cs
- BindingCompleteEventArgs.cs
- SpellerInterop.cs
- TagPrefixInfo.cs
- WebPartEditorApplyVerb.cs
- DataSourceCacheDurationConverter.cs
- XamlStream.cs
- CodeAttachEventStatement.cs
- PackageRelationship.cs
- DatePicker.cs
- MobileUserControl.cs
- BoolExpr.cs
- Function.cs
- TraceUtils.cs
- ServiceOperationWrapper.cs
- StateInitializationDesigner.cs
- DeploymentSectionCache.cs
- MulticastNotSupportedException.cs