Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / XmlUtils / System / Xml / Xsl / Runtime / XmlNavigatorStack.cs / 1 / XmlNavigatorStack.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; namespace System.Xml.Xsl.Runtime { ////// A dynamic stack of IXmlNavigators. /// internal struct XmlNavigatorStack { private XPathNavigator[] stkNav; // Stack of XPathNavigators private int sp; // Stack pointer (size of stack) #if DEBUG private const int InitialStackSize = 2; #else private const int InitialStackSize = 8; #endif ////// Push a navigator onto the stack /// public void Push(XPathNavigator nav) { if (this.stkNav == null) { this.stkNav = new XPathNavigator[InitialStackSize]; } else { if (this.sp >= this.stkNav.Length) { // Resize the stack XPathNavigator[] stkOld = this.stkNav; this.stkNav = new XPathNavigator[2 * this.sp]; Array.Copy(stkOld, this.stkNav, this.sp); } } this.stkNav[this.sp++] = nav; } ////// Pop the topmost navigator and return it /// public XPathNavigator Pop() { Debug.Assert(!IsEmpty); return this.stkNav[--this.sp]; } ////// Returns the navigator at the top of the stack without adjusting the stack pointer /// public XPathNavigator Peek() { Debug.Assert(!IsEmpty); return this.stkNav[this.sp - 1]; } ////// Remove all navigators from the stack /// public void Reset() { this.sp = 0; } ////// Returns true if there are no navigators in the stack /// public bool IsEmpty { get { return this.sp == 0; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; using System.Xml; using System.Xml.XPath; using System.Diagnostics; namespace System.Xml.Xsl.Runtime { ////// A dynamic stack of IXmlNavigators. /// internal struct XmlNavigatorStack { private XPathNavigator[] stkNav; // Stack of XPathNavigators private int sp; // Stack pointer (size of stack) #if DEBUG private const int InitialStackSize = 2; #else private const int InitialStackSize = 8; #endif ////// Push a navigator onto the stack /// public void Push(XPathNavigator nav) { if (this.stkNav == null) { this.stkNav = new XPathNavigator[InitialStackSize]; } else { if (this.sp >= this.stkNav.Length) { // Resize the stack XPathNavigator[] stkOld = this.stkNav; this.stkNav = new XPathNavigator[2 * this.sp]; Array.Copy(stkOld, this.stkNav, this.sp); } } this.stkNav[this.sp++] = nav; } ////// Pop the topmost navigator and return it /// public XPathNavigator Pop() { Debug.Assert(!IsEmpty); return this.stkNav[--this.sp]; } ////// Returns the navigator at the top of the stack without adjusting the stack pointer /// public XPathNavigator Peek() { Debug.Assert(!IsEmpty); return this.stkNav[this.sp - 1]; } ////// Remove all navigators from the stack /// public void Reset() { this.sp = 0; } ////// Returns true if there are no navigators in the stack /// public bool IsEmpty { get { return this.sp == 0; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
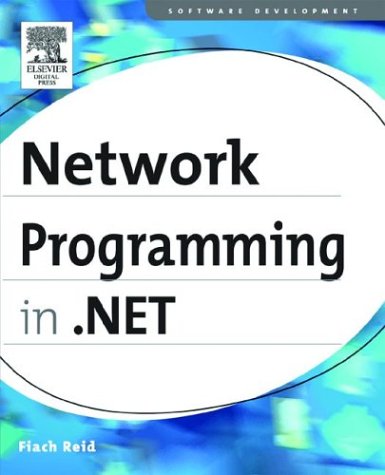
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProfileModule.cs
- SQLStringStorage.cs
- FileUpload.cs
- _PooledStream.cs
- OptimalBreakSession.cs
- Triplet.cs
- DataSpaceManager.cs
- HuffModule.cs
- ObjectPropertyMapping.cs
- SetStateEventArgs.cs
- SafeRegistryHandle.cs
- FrameAutomationPeer.cs
- DbConnectionClosed.cs
- Panel.cs
- HttpModule.cs
- XNameTypeConverter.cs
- SqlCommandSet.cs
- StackSpiller.Bindings.cs
- FileFormatException.cs
- DataKey.cs
- IERequestCache.cs
- KeyFrames.cs
- ControlParameter.cs
- SystemException.cs
- DictionaryManager.cs
- IFlowDocumentViewer.cs
- FrameworkTextComposition.cs
- MatrixCamera.cs
- HostedTransportConfigurationBase.cs
- ProcessModelInfo.cs
- UnsafeNativeMethods.cs
- OdbcDataAdapter.cs
- GeneralTransformGroup.cs
- DefaultEventAttribute.cs
- SimpleWebHandlerParser.cs
- Operators.cs
- EntityViewGenerator.cs
- ListBoxDesigner.cs
- HtmlControlPersistable.cs
- DesignerDataSchemaClass.cs
- AsyncWaitHandle.cs
- Mouse.cs
- ToolBar.cs
- ImageListStreamer.cs
- UrlParameterReader.cs
- DataGridViewColumnTypePicker.cs
- ActivityWithResult.cs
- BaseHashHelper.cs
- XmlWrappingReader.cs
- WebRequestModulesSection.cs
- SQLBinaryStorage.cs
- ApplicationDirectory.cs
- TokenCreationException.cs
- Visitor.cs
- FormsIdentity.cs
- VersionPair.cs
- connectionpool.cs
- BaseCodeDomTreeGenerator.cs
- AssertSection.cs
- DataGridViewColumnStateChangedEventArgs.cs
- MarkupCompiler.cs
- MSHTMLHost.cs
- WizardForm.cs
- MessageBox.cs
- _DisconnectOverlappedAsyncResult.cs
- CustomPopupPlacement.cs
- PageAsyncTask.cs
- Soap.cs
- MetadataItem_Static.cs
- PropertyGeneratedEventArgs.cs
- FocusWithinProperty.cs
- ImageMapEventArgs.cs
- SizeChangedEventArgs.cs
- DataControlButton.cs
- FrameAutomationPeer.cs
- Glyph.cs
- JumpItem.cs
- EntryPointNotFoundException.cs
- Operator.cs
- DetailsView.cs
- WebPartDeleteVerb.cs
- ObjectNotFoundException.cs
- NavigationWindow.cs
- InstanceLockLostException.cs
- ColorBlend.cs
- RequestTimeoutManager.cs
- IndexExpression.cs
- QuaternionAnimation.cs
- OracleLob.cs
- Menu.cs
- CodeAccessPermission.cs
- WpfWebRequestHelper.cs
- Composition.cs
- SendSecurityHeaderElementContainer.cs
- Sql8ConformanceChecker.cs
- Unit.cs
- NotifyInputEventArgs.cs
- Vector3DAnimationBase.cs
- ObjectContextServiceProvider.cs
- RelationshipEnd.cs