Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / MS / Internal / Ink / InkSerializedFormat / HuffModule.cs / 1 / HuffModule.cs
using MS.Utility; using System; using System.Runtime.InteropServices; using System.Security; using System.Globalization; using System.Windows; using System.Windows.Input; using System.Windows.Ink; using MS.Internal.Ink.InkSerializedFormat; using System.Collections.Generic; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// HuffModule /// internal class HuffModule { ////// Ctor /// internal HuffModule() { } ////// GetDefCodec /// internal HuffCodec GetDefCodec(uint index) { HuffCodec huffCodec = null; if (AlgoModule.DefaultBAACount > index) { huffCodec = _defaultHuffCodecs[index]; if (huffCodec == null) { huffCodec = new HuffCodec(index); _defaultHuffCodecs[index] = huffCodec; } } else { throw new ArgumentOutOfRangeException("index"); } return huffCodec; } ////// FindCodec /// /// internal HuffCodec FindCodec(byte algoData) { byte codec = (byte)(algoData & 0x1f); //unused //if ((0x20 & algoData) != 0) //{ // int iLookup = (algoData & 0x1f); // if ((iLookup > 0) && (iLookup <= _lookupList.Count)) // { // codec = _lookupList[iLookup - 1].Byte; // } //} if (codec < AlgoModule.DefaultBAACount) { return GetDefCodec((uint)codec); } if ((int)codec >= _huffCodecs.Count + AlgoModule.DefaultBAACount) { throw new ArgumentException(StrokeCollectionSerializer.ISFDebugMessage("invalid codec computed")); } return _huffCodecs[(int)(codec - AlgoModule.DefaultBAACount)]; } ////// FindDtXf /// /// internal DataXform FindDtXf(byte algoData) { //unused //if ((0x20 & algoData) != 0) //{ // int lookupIndex = (int)(algoData & 0x1f); // if ((lookupIndex > 0) && (lookupIndex < _lookupList.Count)) // { // return _lookupList[lookupIndex].DeltaDelta; // } //} return this.DefaultDeltaDelta; } ////// Private lazy init'd /// private DeltaDelta DefaultDeltaDelta { get { if (_defaultDtxf == null) { _defaultDtxf = new DeltaDelta(); } return _defaultDtxf; } } ////// Privates /// private DeltaDelta _defaultDtxf; //unused //private List_lookupList = new List (); private List _huffCodecs = new List (); private HuffCodec[] _defaultHuffCodecs = new HuffCodec[AlgoModule.DefaultBAACount]; //unused ///// ///// Simple helper class ///// //private class CodeLookup //{ // internal CodeLookup(DeltaDelta dd, byte b) // { // if (dd == null) { throw new ArgumentNullException(); } // DeltaDelta = dd; // Byte = b; // } // internal DeltaDelta DeltaDelta; // internal Byte Byte; //} } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using MS.Utility; using System; using System.Runtime.InteropServices; using System.Security; using System.Globalization; using System.Windows; using System.Windows.Input; using System.Windows.Ink; using MS.Internal.Ink.InkSerializedFormat; using System.Collections.Generic; using SR = MS.Internal.PresentationCore.SR; using SRID = MS.Internal.PresentationCore.SRID; namespace MS.Internal.Ink.InkSerializedFormat { ////// HuffModule /// internal class HuffModule { ////// Ctor /// internal HuffModule() { } ////// GetDefCodec /// internal HuffCodec GetDefCodec(uint index) { HuffCodec huffCodec = null; if (AlgoModule.DefaultBAACount > index) { huffCodec = _defaultHuffCodecs[index]; if (huffCodec == null) { huffCodec = new HuffCodec(index); _defaultHuffCodecs[index] = huffCodec; } } else { throw new ArgumentOutOfRangeException("index"); } return huffCodec; } ////// FindCodec /// /// internal HuffCodec FindCodec(byte algoData) { byte codec = (byte)(algoData & 0x1f); //unused //if ((0x20 & algoData) != 0) //{ // int iLookup = (algoData & 0x1f); // if ((iLookup > 0) && (iLookup <= _lookupList.Count)) // { // codec = _lookupList[iLookup - 1].Byte; // } //} if (codec < AlgoModule.DefaultBAACount) { return GetDefCodec((uint)codec); } if ((int)codec >= _huffCodecs.Count + AlgoModule.DefaultBAACount) { throw new ArgumentException(StrokeCollectionSerializer.ISFDebugMessage("invalid codec computed")); } return _huffCodecs[(int)(codec - AlgoModule.DefaultBAACount)]; } ////// FindDtXf /// /// internal DataXform FindDtXf(byte algoData) { //unused //if ((0x20 & algoData) != 0) //{ // int lookupIndex = (int)(algoData & 0x1f); // if ((lookupIndex > 0) && (lookupIndex < _lookupList.Count)) // { // return _lookupList[lookupIndex].DeltaDelta; // } //} return this.DefaultDeltaDelta; } ////// Private lazy init'd /// private DeltaDelta DefaultDeltaDelta { get { if (_defaultDtxf == null) { _defaultDtxf = new DeltaDelta(); } return _defaultDtxf; } } ////// Privates /// private DeltaDelta _defaultDtxf; //unused //private List_lookupList = new List (); private List _huffCodecs = new List (); private HuffCodec[] _defaultHuffCodecs = new HuffCodec[AlgoModule.DefaultBAACount]; //unused ///// ///// Simple helper class ///// //private class CodeLookup //{ // internal CodeLookup(DeltaDelta dd, byte b) // { // if (dd == null) { throw new ArgumentNullException(); } // DeltaDelta = dd; // Byte = b; // } // internal DeltaDelta DeltaDelta; // internal Byte Byte; //} } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
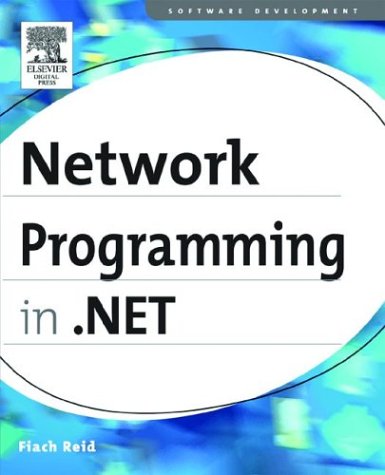
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RequestCachePolicyConverter.cs
- SqlReferenceCollection.cs
- XDeferredAxisSource.cs
- KerberosTicketHashIdentifierClause.cs
- LiteralControl.cs
- FixUpCollection.cs
- Mouse.cs
- RealizationContext.cs
- TypeToArgumentTypeConverter.cs
- SerTrace.cs
- ProfileEventArgs.cs
- FolderBrowserDialogDesigner.cs
- LinqDataSourceUpdateEventArgs.cs
- ClientApiGenerator.cs
- Directory.cs
- Point3DCollection.cs
- MatrixValueSerializer.cs
- WpfKnownType.cs
- SettingsContext.cs
- Base64Encoder.cs
- XPathPatternParser.cs
- DataGridViewCellFormattingEventArgs.cs
- DeviceFiltersSection.cs
- ServicesUtilities.cs
- GridViewSortEventArgs.cs
- ListItemViewAttribute.cs
- WorkflowInstanceTerminatedRecord.cs
- DataControlFieldCollection.cs
- ChannelManager.cs
- ButtonPopupAdapter.cs
- HttpCachePolicyElement.cs
- documentsequencetextview.cs
- SqlCachedBuffer.cs
- BindingSource.cs
- Point.cs
- OracleNumber.cs
- DeviceSpecificDialogCachedState.cs
- FrameworkReadOnlyPropertyMetadata.cs
- FontWeights.cs
- FileSystemWatcher.cs
- Exceptions.cs
- Parsers.cs
- SpnEndpointIdentity.cs
- StickyNoteAnnotations.cs
- _AuthenticationState.cs
- EntityDataSourceValidationException.cs
- ContextBase.cs
- TransformGroup.cs
- MsmqMessageSerializationFormat.cs
- ServiceContractGenerator.cs
- TextEffectCollection.cs
- OleDbStruct.cs
- String.cs
- PropertyGridView.cs
- PagePropertiesChangingEventArgs.cs
- XmlHelper.cs
- ItemDragEvent.cs
- SerializableAttribute.cs
- LogLogRecordHeader.cs
- EntityUtil.cs
- CodeNamespaceImportCollection.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- ButtonField.cs
- ReachDocumentReferenceCollectionSerializer.cs
- ErrorHandler.cs
- HeaderedContentControl.cs
- DataSet.cs
- Drawing.cs
- _ProxyChain.cs
- CultureMapper.cs
- SelectedDatesCollection.cs
- AbandonedMutexException.cs
- SecurityAccessDeniedException.cs
- Content.cs
- Ops.cs
- DefaultValueMapping.cs
- CodeMemberField.cs
- StoryFragments.cs
- LogRestartAreaEnumerator.cs
- MouseOverProperty.cs
- RubberbandSelector.cs
- ContentPresenter.cs
- BindingMAnagerBase.cs
- TransformationRules.cs
- WebBrowserEvent.cs
- UnsafeNativeMethods.cs
- ValidatorCompatibilityHelper.cs
- UnknownBitmapDecoder.cs
- OutOfProcStateClientManager.cs
- StylusEventArgs.cs
- XslTransformFileEditor.cs
- VersionedStreamOwner.cs
- OptimalTextSource.cs
- DeflateStream.cs
- CollectionChangedEventManager.cs
- XmlDataCollection.cs
- EmptyReadOnlyDictionaryInternal.cs
- CharacterMetrics.cs
- OnOperation.cs
- DeviceFilterDictionary.cs