Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / DataOracleClient / System / Data / OracleClient / OracleNumber.cs / 1 / OracleNumber.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data.SqlTypes; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Text; //--------------------------------------------------------------------- // OracleNumber // // Contains all the information about a single column in a result set, // and implements the methods necessary to describe column to Oracle // and to extract the column data from the native buffer used to fetch // it. // [StructLayout(LayoutKind.Sequential, Pack=1)] public struct OracleNumber : IComparable, INullable { // Used to bracket the range of double precision values that a number can be constructed from static private double doubleMinValue = -9.99999999999999E+125; static private double doubleMaxValue = 9.99999999999999E+125; // DEVNOTE: the following constants are derived by uncommenting out the // code below and running the OCITest program. The code below // will compute the correct constant values and will print them // to the console output, where you can cut and past back into // this file. private static readonly byte[] OciNumberValue_DecimalMaxValue= { 0x10, 0xcf, 0x08, 0x5d, 0x1d, 0x11, 0x1a, 0x0f, 0x1b, 0x2c, 0x26, 0x3b, 0x5d, 0x31, 0x63, 0x1f, 0x28 }; private static readonly byte[] OciNumberValue_DecimalMinValue= { 0x11, 0x30, 0x5e, 0x09, 0x49, 0x55, 0x4c, 0x57, 0x4b, 0x3a, 0x40, 0x2b, 0x09, 0x35, 0x03, 0x47, 0x3e, 0x66 }; private static readonly byte[] OciNumberValue_E = { 0x15, 0xc1, 0x03, 0x48, 0x53, 0x52, 0x53, 0x55, 0x3c, 0x05, 0x35, 0x24, 0x25, 0x03, 0x58, 0x30, 0x0e, 0x35, 0x43, 0x19, 0x62, 0x4d }; private static readonly byte[] OciNumberValue_MaxValue = { 0x14, 0xff, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64 }; private static readonly byte[] OciNumberValue_MinValue = { 0x15, 0x00, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x66 }; private static readonly byte[] OciNumberValue_MinusOne = { 0x03, 0x3e, 0x64, 0x66 }; private static readonly byte[] OciNumberValue_One = { 0x02, 0xc1, 0x02 }; private static readonly byte[] OciNumberValue_Pi = { 0x15, 0xc1, 0x04, 0x0f, 0x10, 0x5d, 0x42, 0x24, 0x5a, 0x50, 0x21, 0x27, 0x2f, 0x1b, 0x2c, 0x27, 0x21, 0x50, 0x33, 0x1d, 0x55, 0x15 }; private static readonly byte[] OciNumberValue_TwoPow64 = { 0x0b, 0xca, 0x13, 0x2d, 0x44, 0x2d, 0x08, 0x26, 0x0a, 0x38, 0x11, 0x11 }; private static readonly byte[] OciNumberValue_Zero = { 0x01, 0x80 }; #if GENERATENUMBERCONSTANTS // This is the place that computes the static byte array constant values for the // various constants this class exposes; run this and capture the output to construct // the values that are used above. static OracleNumber() { OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); byte[] result = new byte[22]; //----------------------------------------------------------------- FromDecimal(errorHandle, Decimal.MaxValue, result); PrintByteConstant("OciNumberValue_DecimalMaxValue", result); //------------------------------------------------------------------ FromDecimal(errorHandle, Decimal.MinValue, result); PrintByteConstant("OciNumberValue_DecimalMinValue", result); //----------------------------------------------------------------- FromInt32(errorHandle, 1, result); UnsafeNativeMethods.OCINumberExp( errorHandle, // err result, // p result // result ); PrintByteConstant("OciNumberValue_E", result); //------------------------------------------------------------------ OracleNumber MaxValue = new OracleNumber("9.99999999999999999999999999999999999999E+125"); PrintByteConstant("OciNumberValue_MaxValue", MaxValue._value); //------------------------------------------------------------------ OracleNumber MinValue = new OracleNumber("-9.99999999999999999999999999999999999999E+125"); PrintByteConstant("OciNumberValue_MinValue", MinValue._value); //----------------------------------------------------------------- FromInt32(errorHandle, -1, result); PrintByteConstant("OciNumberValue_MinusOne", result); //------------------------------------------------------------------ FromInt32(errorHandle, 1, result); PrintByteConstant("OciNumberValue_One", result); //----------------------------------------------------------------- UnsafeNativeMethods.OCINumberSetPi( errorHandle, // err result // result ); PrintByteConstant("OciNumberValue_Pi", result); //----------------------------------------------------------------- FromInt32(errorHandle, 2, result); PrintByteConstant("OciNumberValue_Two", result); //----------------------------------------------------------------- FromInt32(errorHandle, 2, result); UnsafeNativeMethods.OCINumberIntPower( errorHandle, // err result, // base 64, // exp result // result ); PrintByteConstant("OciNumberValue_TwoPow64", result); //------------------------------------------------------------------ UnsafeNativeMethods.OCINumberSetZero( errorHandle, // err result // result ); PrintByteConstant("OciNumberValue_Zero", result); //----------------------------------------------------------------- } static void PrintByteConstant(string name, byte[] value) { Console.Write(String.Format("private static readonly byte[] {0,-25}= {{ 0x{1,-2:x2}", name, value[0])); for (int i = 1; i <= value[0]; i++) { Console.Write(String.Format(", 0x{0,-2:x2}", value[i])); } Console.WriteLine(" };"); } #endif //GENERATENUMBERCONSTANTS private byte[] _value; // null == value is null public static readonly OracleNumber E = new OracleNumber(OciNumberValue_E); public static readonly Int32 MaxPrecision = 38; public static readonly Int32 MaxScale = 127; public static readonly Int32 MinScale = -84; public static readonly OracleNumber MaxValue = new OracleNumber(OciNumberValue_MaxValue); public static readonly OracleNumber MinValue = new OracleNumber(OciNumberValue_MinValue); public static readonly OracleNumber MinusOne = new OracleNumber(OciNumberValue_MinusOne); public static readonly OracleNumber Null= new OracleNumber(true); public static readonly OracleNumber One = new OracleNumber(OciNumberValue_One); public static readonly OracleNumber PI = new OracleNumber(OciNumberValue_Pi); public static readonly OracleNumber Zero= new OracleNumber(OciNumberValue_Zero); // (internal) Construct from nothing private OracleNumber(bool isNull) { _value = (isNull) ? null : new byte[22]; } // (internal) Construct from a constant byte array private OracleNumber(byte[] bits) { _value = bits; } // Construct from System.Decimal public OracleNumber (Decimal decValue) : this (false) { OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); FromDecimal(errorHandle, decValue, _value); } // Construct from System.Double public OracleNumber (double dblValue) : this (false) { OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); FromDouble(errorHandle, dblValue, _value); } // Construct from System.Int32 public OracleNumber (Int32 intValue) : this (false) { OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); FromInt32(errorHandle, intValue, _value); } // Construct from System.Int64 public OracleNumber (Int64 longValue) : this (false) { OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); FromInt64(errorHandle, longValue, _value); } // Copy constructor public OracleNumber (OracleNumber from) { byte[] fromvalue = from._value; if (null != fromvalue) _value = (byte[])fromvalue.Clone(); else _value = null; } // (internal) Construct from System.String internal OracleNumber (string s) : this (false) { OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); FromString(errorHandle, s, _value); } // (internal) construct from a row/parameter binding internal OracleNumber (NativeBuffer buffer, int valueOffset) : this (false) { buffer.ReadBytes(valueOffset, _value, 0, 22); } public bool IsNull { get { return (null == _value); } } public Decimal Value { get { return (Decimal)this; } } public int CompareTo (object obj) { if (obj.GetType() == typeof(OracleNumber)) { OracleNumber value2 = (OracleNumber)obj; // If both values are Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) { return value2.IsNull ? 0 : -1; } if (value2.IsNull) { return 1; } // Neither value is null, do the comparison. OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); int result = InternalCmp(errorHandle, _value, value2._value); return result; } throw ADP.WrongType(obj.GetType(), typeof(OracleNumber)); } public override bool Equals(object value) { if (value is OracleNumber) return (this == (OracleNumber)value).Value; else return false; } public override int GetHashCode() { return IsNull ? 0 : _value.GetHashCode(); } static internal decimal MarshalToDecimal (NativeBuffer buffer, int valueOffset, OracleConnection connection) { byte[] value = buffer.ReadBytes(valueOffset, 22); OciErrorHandle errorHandle = connection.ErrorHandle; decimal result = ToDecimal(errorHandle, value); return result; } static internal int MarshalToInt32 (NativeBuffer buffer, int valueOffset, OracleConnection connection) { byte[] value = buffer.ReadBytes(valueOffset, 22); OciErrorHandle errorHandle = connection.ErrorHandle; int result = ToInt32(errorHandle, value); return result; } static internal long MarshalToInt64 (NativeBuffer buffer, int valueOffset, OracleConnection connection) { byte[] value = buffer.ReadBytes(valueOffset, 22); OciErrorHandle errorHandle = connection.ErrorHandle; long result = ToInt64(errorHandle, value); return result; } static internal int MarshalToNative (object value, NativeBuffer buffer, int offset, OracleConnection connection) { byte[] from; if ( value is OracleNumber ) { from = ((OracleNumber)value)._value; } else { OciErrorHandle errorHandle = connection.ErrorHandle; from = new byte[22]; if ( value is Decimal ) { FromDecimal(errorHandle, (decimal)value, from); } else if ( value is int ) { FromInt32(errorHandle, (int)value, from); } else if ( value is long ) { FromInt64(errorHandle, (long)value, from); } else { //if ( value is double ) FromDouble(errorHandle, (double)value, from); } } buffer.WriteBytes(offset, from, 0, 22); return 22; } public static OracleNumber Parse(string s) { if (null == s) { throw ADP.ArgumentNull("s"); } return new OracleNumber(s); } //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Internal Operators (used in the conversion routines) // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// private static void InternalAdd (OciErrorHandle errorHandle, byte[] x, byte[] y, byte[] result) { int rc = UnsafeNativeMethods.OCINumberAdd( errorHandle, // err x, // number1 y, // number2 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static int InternalCmp (OciErrorHandle errorHandle, byte[] value1, byte[] value2) { int result; int rc = UnsafeNativeMethods.OCINumberCmp( errorHandle, // err value1, // number1 value2, // number2 out result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } private static void InternalDiv (OciErrorHandle errorHandle, byte[] x, byte[] y, byte[] result) { int rc = UnsafeNativeMethods.OCINumberDiv( errorHandle, // err x, // number1 y, // number2 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static bool InternalIsInt (OciErrorHandle errorHandle, byte[] n) { int isInt; int rc = UnsafeNativeMethods.OCINumberIsInt( errorHandle, // err n, // number out isInt // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return (0 != isInt); } private static void InternalMod (OciErrorHandle errorHandle, byte[] x, byte[] y, byte[] result) { int rc = UnsafeNativeMethods.OCINumberMod( errorHandle, // err x, // number1 y, // number2 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void InternalMul (OciErrorHandle errorHandle, byte[] x, byte[] y, byte[] result) { int rc = UnsafeNativeMethods.OCINumberMul( errorHandle, // err x, // number1 y, // number2 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void InternalNeg (OciErrorHandle errorHandle, byte[] x, byte[] result) { int rc = UnsafeNativeMethods.OCINumberNeg( errorHandle, // err x, // number1 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static int InternalSign (OciErrorHandle errorHandle, byte[] n) { int sign; int rc = UnsafeNativeMethods.OCINumberSign( errorHandle, // err n, // number out sign // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return sign; } private static void InternalShift (OciErrorHandle errorHandle, byte[] n, int digits, byte[] result) { int rc = UnsafeNativeMethods.OCINumberShift( errorHandle, // err n, // nDig digits, // nDig result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void InternalSub (OciErrorHandle errorHandle, byte[] x, byte[] y, byte[] result) { int rc = UnsafeNativeMethods.OCINumberSub( errorHandle, // err x, // number1 y, // number2 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void InternalTrunc (OciErrorHandle errorHandle, byte[] n, int position, byte[] result) { int rc = UnsafeNativeMethods.OCINumberTrunc( errorHandle, // err n, // number position, // decplace result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void FromDecimal (OciErrorHandle errorHandle, decimal decimalValue, byte[] result) { int[] unpackedDecimal = Decimal.GetBits(decimalValue); ulong lowMidPart = ((ulong)((uint)unpackedDecimal[1]) << 32) | (ulong)((uint)unpackedDecimal[0]); uint highPart = (uint)unpackedDecimal[2]; int sign = (unpackedDecimal[3] >> 31); int scale = ((unpackedDecimal[3] >> 16) & 0x7f); FromUInt64(errorHandle, lowMidPart, result); if (0 != highPart) { // Bummer, the value is larger than an Int64... byte[] temp = new byte[22]; FromUInt32 (errorHandle, highPart, temp); InternalMul (errorHandle, temp, OciNumberValue_TwoPow64, temp); InternalAdd (errorHandle, result, temp, result); } // If the sign bit indicates negative, negate the result; if (0 != sign) { InternalNeg (errorHandle, result, result); } // Adjust for a scale value. if (0 != scale) { InternalShift(errorHandle, result, -scale, result); } } private static void FromDouble (OciErrorHandle errorHandle, double dblValue, byte[] result) { if (dblValue < doubleMinValue || dblValue > doubleMaxValue) { throw ADP.OperationResultedInOverflow(); } int rc = UnsafeNativeMethods.OCINumberFromReal( errorHandle, // err ref dblValue, // rnum 8, // rnum_length result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void FromInt32 (OciErrorHandle errorHandle, int intValue, byte[] result) { int rc = UnsafeNativeMethods.OCINumberFromInt( errorHandle, // err ref intValue, // inum 4, // inum_length OCI.SIGN.OCI_NUMBER_SIGNED, // inum_s_flag result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void FromUInt32 (OciErrorHandle errorHandle, UInt32 uintValue, byte[] result) { int rc = UnsafeNativeMethods.OCINumberFromInt( errorHandle, // err ref uintValue, // inum 4, // inum_length OCI.SIGN.OCI_NUMBER_UNSIGNED,//inum_s_flag result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void FromInt64 (OciErrorHandle errorHandle, long longValue, byte[] result) { int rc = UnsafeNativeMethods.OCINumberFromInt( errorHandle, // err ref longValue, // inum 8, // inum_length OCI.SIGN.OCI_NUMBER_SIGNED, // inum_s_flag result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void FromUInt64 (OciErrorHandle errorHandle, UInt64 ulongValue, byte[] result) { int rc = UnsafeNativeMethods.OCINumberFromInt( errorHandle, // err ref ulongValue, // inum 8, // inum_length OCI.SIGN.OCI_NUMBER_UNSIGNED,//inum_s_flag result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } //3 NOTE: While Oracle will dish out up to 64 characters if you use the //3 "TM9" format string, they don't accept the "TM9" format string when //3 they're unformatting and they'll only accept 63 characters in the format //3 string you pass to OCINumberFromText. // "....+....1....+....2....+....3....+....4....+....5....+....6...." private const string WholeDigitPattern = "999999999999999999999999999999999999999999999999999999999999999"; private const int WholeDigitPattern_Length = 63; private void FromStringOfDigits(OciErrorHandle errorHandle, string s, byte[] result) { if (s.Length <= WholeDigitPattern_Length) { int rc = UnsafeNativeMethods.OCINumberFromText( errorHandle, // err s, // str unchecked((uint)s.Length), // str_length WholeDigitPattern, // fmt WholeDigitPattern_Length, // fmt_length IntPtr.Zero, // nls_params 0, // nls_p_length result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } else { byte[] temp = new byte[22]; string leftPart = s.Substring(0,WholeDigitPattern_Length); string rightPart = s.Substring(WholeDigitPattern_Length); FromStringOfDigits(errorHandle, leftPart, temp); FromStringOfDigits(errorHandle, rightPart, result); InternalShift(errorHandle, temp, rightPart.Length, temp); InternalAdd(errorHandle, result, temp, result); } } private void FromString (OciErrorHandle errorHandle, string s, byte[] result) { // DEVNOTE: this only supports the format [{+/-}]digits.digits[E[{+/-}]exponent], // that is all that SQLNumeric supports, so that's all I'll support. It // is also all that the ToString method on this class will produce... byte[] temp = new byte[22]; int exponent = 0; s = s.Trim(); int exponentAt = s.IndexOfAny("eE".ToCharArray()); if (exponentAt > 0) { exponent = Int32.Parse(s.Substring(exponentAt+1), CultureInfo.InvariantCulture); s = s.Substring(0,exponentAt); } bool isNegative = false; if ('-' == s[0]) { isNegative = true; s = s.Substring(1); } else if ('+' == s[0]) { s = s.Substring(1); } int decimalPointAt = s.IndexOf('.'); if (0 <= decimalPointAt) { string fractionalDigits = s.Substring(decimalPointAt+1); FromStringOfDigits(errorHandle, fractionalDigits, result); InternalShift(errorHandle, result, -fractionalDigits.Length, result); if (0 != decimalPointAt) { FromStringOfDigits(errorHandle, s.Substring(0,decimalPointAt), temp); InternalAdd(errorHandle, result, temp, result); } } else { FromStringOfDigits(errorHandle, s, result); } if (0 != exponent) { InternalShift(errorHandle, result, exponent, result); } if (isNegative) { InternalNeg(errorHandle, result, result); } GC.KeepAlive(s); } private static Decimal ToDecimal (OciErrorHandle errorHandle, byte[] value) { byte[] temp1 = (byte[])value.Clone(); byte[] temp2 = new byte[22]; byte scale = 0; int sign = InternalSign(errorHandle, temp1); if (sign < 0) { InternalNeg(errorHandle, temp1, temp1); } if ( !InternalIsInt(errorHandle, temp1) ) { // DEVNOTE: I happen to know how to figure out how many decimal places the value has, // but it means cracking the value. Oracle doesn't provide API support to // tell us how many decimal digits there are in a nubmer int decimalShift = 2 * (temp1[0] - ((temp1[1] & 0x7f)-64) - 1); InternalShift(errorHandle, temp1, decimalShift, temp1); scale += (byte)decimalShift; while( !InternalIsInt(errorHandle, temp1) ) { InternalShift(errorHandle, temp1, 1, temp1); scale++; } } InternalMod(errorHandle, temp1, OciNumberValue_TwoPow64, temp2); ulong loMid = ToUInt64(errorHandle, temp2); InternalDiv(errorHandle, temp1, OciNumberValue_TwoPow64, temp2); InternalTrunc(errorHandle, temp2, 0, temp2); uint hi = ToUInt32(errorHandle, temp2); Decimal decimalValue = new Decimal( (int)(loMid & 0xffffffff),// lo (int)(loMid >> 32), // mid (int)hi, // hi (sign < 0), // isNegative scale // scale ); return decimalValue; } private static int ToInt32 (OciErrorHandle errorHandle, byte[] value) { int result; int rc = UnsafeNativeMethods.OCINumberToInt( errorHandle, // err value, // number 4, // rsl_length OCI.SIGN.OCI_NUMBER_SIGNED, // rsl_flag out result // rsl ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } private static uint ToUInt32 (OciErrorHandle errorHandle, byte[] value) { uint result; int rc = UnsafeNativeMethods.OCINumberToInt( errorHandle, // err value, // number 4, // rsl_length OCI.SIGN.OCI_NUMBER_UNSIGNED,//rsl_flag out result // rsl ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } private static long ToInt64 (OciErrorHandle errorHandle, byte[] value) { long result; int rc = UnsafeNativeMethods.OCINumberToInt( errorHandle, // err value, // number 8, // rsl_length OCI.SIGN.OCI_NUMBER_SIGNED, // rsl_flag out result // rsl ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } private static ulong ToUInt64 (OciErrorHandle errorHandle, byte[] value) { ulong result; int rc = UnsafeNativeMethods.OCINumberToInt( errorHandle, // err value, // number 8, // rsl_length OCI.SIGN.OCI_NUMBER_UNSIGNED,//rsl_flag out result // rsl ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } static private string ToString (OciErrorHandle errorHandle, byte[] value) { byte[] buffer = new byte[64]; uint bufferLen = unchecked((uint)buffer.Length); int rc = UnsafeNativeMethods.OCINumberToText( errorHandle, // err value, // number "TM9", // fmt 3, // fmt_length IntPtr.Zero, // nls_params 0, // nls_p_length ref bufferLen, // buf_size buffer // buf ); if (0 != rc) { OracleException.Check(errorHandle, rc); } // Wonder of wonders, Oracle has a problem with the value -999999999999999999999999.9999 // where it seems that has trailing ':' characters if you their translator // method; we deal with it by removing the ':' characters. int realBufferLen = Array.IndexOf(buffer, (byte)58); // Oracle doesn't set the buffer length correctly; they include the null bytes // that trail the actual value. That means it's up to us to remove them. realBufferLen = (realBufferLen > 0) ? realBufferLen : Array.LastIndexOf(buffer, 0); // Technically, we should use Oracle's conversion OCICharsetToUnicode, but since numeric // digits are ANSI digits, we're safe here. string retval = System.Text.Encoding.Default.GetString(buffer, 0, (realBufferLen > 0) ? realBufferLen : checked((int)bufferLen)); return retval; } public override string ToString() { if (IsNull){ return ADP.NullString; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); string retval = ToString(errorHandle, _value); return retval; } public static OracleBoolean operator== (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) == 0); } public static OracleBoolean operator> (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) > 0); } public static OracleBoolean operator>= (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) >= 0); } public static OracleBoolean operator< (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) < 0); } public static OracleBoolean operator<= (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) <= 0); } public static OracleBoolean operator!= (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) != 0); } public static OracleNumber operator- (OracleNumber x) { if (x.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalNeg(errorHandle, x._value, result._value); return result; } public static OracleNumber operator+ (OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalAdd(errorHandle,x._value,y._value,result._value); return result; } public static OracleNumber operator- (OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalSub(errorHandle,x._value,y._value,result._value); return result; } public static OracleNumber operator* (OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalMul(errorHandle, x._value, y._value, result._value); return result; } public static OracleNumber operator/ (OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalDiv(errorHandle, x._value, y._value, result._value); return result; } public static OracleNumber operator% (OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalMod(errorHandle, x._value, y._value, result._value); return result; } public static explicit operator Decimal(OracleNumber x) { if (x.IsNull) { throw ADP.DataIsNull(); } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); Decimal result = ToDecimal(errorHandle, x._value); return result; } public static explicit operator Double(OracleNumber x) { if (x.IsNull) { throw ADP.DataIsNull(); } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); double result; int rc = UnsafeNativeMethods.OCINumberToReal( errorHandle, // err x._value, // number 8, // rsl_length out result // rsl ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } public static explicit operator Int32(OracleNumber x) { if (x.IsNull) { throw ADP.DataIsNull(); } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); Int32 result = ToInt32(errorHandle, x._value); return result; } public static explicit operator Int64(OracleNumber x) { if (x.IsNull) { throw ADP.DataIsNull(); } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); Int64 result = ToInt64(errorHandle, x._value); return result; } public static explicit operator OracleNumber(Decimal x) { return new OracleNumber(x); } public static explicit operator OracleNumber(double x) { return new OracleNumber(x); } public static explicit operator OracleNumber(int x) { return new OracleNumber(x); } public static explicit operator OracleNumber(long x) { return new OracleNumber(x); } public static explicit operator OracleNumber(string x) { return new OracleNumber(x); } //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Built In Functions // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// //---------------------------------------------------------------------- // Abs - absolute value // public static OracleNumber Abs(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberAbs( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Acos - Get the Arc Cosine of the number // public static OracleNumber Acos(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberArcCos( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Add - Alternative method for operator + // public static OracleNumber Add(OracleNumber x, OracleNumber y) { return x + y; } //---------------------------------------------------------------------- // Asin - Get the Arc Sine of the number // public static OracleNumber Asin(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberArcSin( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Atan - Get the Arc Tangent of the number // public static OracleNumber Atan(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberArcTan( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Atan2 - Get the Arc Tangent of twi numbers // public static OracleNumber Atan2(OracleNumber y, OracleNumber x) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberArcTan2( errorHandle, // err y._value, // number x._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Ceiling - next smallest integer greater than or equal to the numeric // public static OracleNumber Ceiling(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberCeil( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Cos - Get the Cosine of the number // public static OracleNumber Cos(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberCos( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Cosh - Get the Hyperbolic Cosine of the number // public static OracleNumber Cosh(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberHypCos( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Divide - Alternative method for operator / // public static OracleNumber Divide(OracleNumber x, OracleNumber y) { return x / y; } //---------------------------------------------------------------------- // Equals - Alternative method for operator == // public static OracleBoolean Equals(OracleNumber x, OracleNumber y) { return (x == y); } //--------------------------------------------------------------------- // Exp - raise e to the specified power // public static OracleNumber Exp(OracleNumber p) { if (p.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberExp( errorHandle, // err p._value, // base result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Floor - next largest integer smaller or equal to the numeric // public static OracleNumber Floor(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberFloor( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // GreaterThan - Alternative method for operator > // public static OracleBoolean GreaterThan(OracleNumber x, OracleNumber y) { return (x > y); } //--------------------------------------------------------------------- // GreaterThanOrEqual - Alternative method for operator >= // public static OracleBoolean GreaterThanOrEqual(OracleNumber x, OracleNumber y) { return (x >= y); } //--------------------------------------------------------------------- // LessThan - Alternative method for operator < // public static OracleBoolean LessThan(OracleNumber x, OracleNumber y) { return (x < y); } //---------------------------------------------------------------------- // LessThanOrEqual - Alternative method for operator <= // public static OracleBoolean LessThanOrEqual(OracleNumber x, OracleNumber y) { return (x <= y); } //--------------------------------------------------------------------- // Log - Compute the natural logarithm (base e) // public static OracleNumber Log(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberLn( errorHandle, // err n._value, // base result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Log - Compute the logarithm (any base) // public static OracleNumber Log(OracleNumber n, int newBase) { return Log(n, new OracleNumber(newBase)); } public static OracleNumber Log(OracleNumber n, OracleNumber newBase) { if (n.IsNull || newBase.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberLog( errorHandle, // err newBase._value, // base n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Log10 - Compute the logarithm (base 10) // public static OracleNumber Log10(OracleNumber n) { return Log(n, new OracleNumber(10)); } //--------------------------------------------------------------------- // Max - Get the maximum value of two Oracle Numbers // public static OracleNumber Max(OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } return (x > y) ? x : y; } //---------------------------------------------------------------------- // Min - Get the minimum value of two Oracle Numbers // public static OracleNumber Min(OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } return (x < y) ? x : y; } //--------------------------------------------------------------------- // Modulo - Alternative method for operator % // public static OracleNumber Modulo(OracleNumber x, OracleNumber y) { return x % y; } //--------------------------------------------------------------------- // Multiply - Alternative method for operator * // public static OracleNumber Multiply(OracleNumber x, OracleNumber y) { return x * y; } //--------------------------------------------------------------------- // Negate - Alternative method for unary operator - // public static OracleNumber Negate(OracleNumber x) { return -x; } //---------------------------------------------------------------------- // NotEquals - Alternative method for operator != // public static OracleBoolean NotEquals(OracleNumber x, OracleNumber y) { return (x != y); } //--------------------------------------------------------------------- // Pow - Compute the power of a numeric // public static OracleNumber Pow(OracleNumber x, Int32 y) { if (x.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberIntPower( errorHandle, // err x._value, // base y, // exp result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } public static OracleNumber Pow(OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberPower( errorHandle, // err x._value, // base y._value, // exp result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Round - Round the numeric to a specific digit // public static OracleNumber Round(OracleNumber n, int position) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberRound( errorHandle, // err n._value, // number position, // decplace result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Shift - Shift the number of digits // public static OracleNumber Shift(OracleNumber n, int digits) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalShift(errorHandle, n._value, digits, result._value); return result; } //--------------------------------------------------------------------- // Sign - 1 if positive, -1 if negative // public static OracleNumber Sign(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); int sign = InternalSign(errorHandle, n._value); OracleNumber result = (sign > 0) ? One : MinusOne; return result; } //---------------------------------------------------------------------- // Sin - Get the Sine of the number // public static OracleNumber Sin(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberSin( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Sinh - Get the Hyperbolic Sine of the number // public static OracleNumber Sinh(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberHypSin( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Sqrt - Get the Square Root of the number // public static OracleNumber Sqrt(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberSqrt( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Subtract - Alternative method for operator - // public static OracleNumber Subtract(OracleNumber x, OracleNumber y) { return x - y; } //---------------------------------------------------------------------- // Tan - Get the Tangent of the number // public static OracleNumber Tan(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberTan( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Tanh - Get the Hyperbolic Tangent of the number // public static OracleNumber Tanh(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberHypTan( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Truncate - Truncate the numeric to a specific digit // public static OracleNumber Truncate(OracleNumber n, int position) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalTrunc(errorHandle, n._value, position, result._value); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data.SqlTypes; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Text; //--------------------------------------------------------------------- // OracleNumber // // Contains all the information about a single column in a result set, // and implements the methods necessary to describe column to Oracle // and to extract the column data from the native buffer used to fetch // it. // [StructLayout(LayoutKind.Sequential, Pack=1)] public struct OracleNumber : IComparable, INullable { // Used to bracket the range of double precision values that a number can be constructed from static private double doubleMinValue = -9.99999999999999E+125; static private double doubleMaxValue = 9.99999999999999E+125; // DEVNOTE: the following constants are derived by uncommenting out the // code below and running the OCITest program. The code below // will compute the correct constant values and will print them // to the console output, where you can cut and past back into // this file. private static readonly byte[] OciNumberValue_DecimalMaxValue= { 0x10, 0xcf, 0x08, 0x5d, 0x1d, 0x11, 0x1a, 0x0f, 0x1b, 0x2c, 0x26, 0x3b, 0x5d, 0x31, 0x63, 0x1f, 0x28 }; private static readonly byte[] OciNumberValue_DecimalMinValue= { 0x11, 0x30, 0x5e, 0x09, 0x49, 0x55, 0x4c, 0x57, 0x4b, 0x3a, 0x40, 0x2b, 0x09, 0x35, 0x03, 0x47, 0x3e, 0x66 }; private static readonly byte[] OciNumberValue_E = { 0x15, 0xc1, 0x03, 0x48, 0x53, 0x52, 0x53, 0x55, 0x3c, 0x05, 0x35, 0x24, 0x25, 0x03, 0x58, 0x30, 0x0e, 0x35, 0x43, 0x19, 0x62, 0x4d }; private static readonly byte[] OciNumberValue_MaxValue = { 0x14, 0xff, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64, 0x64 }; private static readonly byte[] OciNumberValue_MinValue = { 0x15, 0x00, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x02, 0x66 }; private static readonly byte[] OciNumberValue_MinusOne = { 0x03, 0x3e, 0x64, 0x66 }; private static readonly byte[] OciNumberValue_One = { 0x02, 0xc1, 0x02 }; private static readonly byte[] OciNumberValue_Pi = { 0x15, 0xc1, 0x04, 0x0f, 0x10, 0x5d, 0x42, 0x24, 0x5a, 0x50, 0x21, 0x27, 0x2f, 0x1b, 0x2c, 0x27, 0x21, 0x50, 0x33, 0x1d, 0x55, 0x15 }; private static readonly byte[] OciNumberValue_TwoPow64 = { 0x0b, 0xca, 0x13, 0x2d, 0x44, 0x2d, 0x08, 0x26, 0x0a, 0x38, 0x11, 0x11 }; private static readonly byte[] OciNumberValue_Zero = { 0x01, 0x80 }; #if GENERATENUMBERCONSTANTS // This is the place that computes the static byte array constant values for the // various constants this class exposes; run this and capture the output to construct // the values that are used above. static OracleNumber() { OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); byte[] result = new byte[22]; //----------------------------------------------------------------- FromDecimal(errorHandle, Decimal.MaxValue, result); PrintByteConstant("OciNumberValue_DecimalMaxValue", result); //------------------------------------------------------------------ FromDecimal(errorHandle, Decimal.MinValue, result); PrintByteConstant("OciNumberValue_DecimalMinValue", result); //----------------------------------------------------------------- FromInt32(errorHandle, 1, result); UnsafeNativeMethods.OCINumberExp( errorHandle, // err result, // p result // result ); PrintByteConstant("OciNumberValue_E", result); //------------------------------------------------------------------ OracleNumber MaxValue = new OracleNumber("9.99999999999999999999999999999999999999E+125"); PrintByteConstant("OciNumberValue_MaxValue", MaxValue._value); //------------------------------------------------------------------ OracleNumber MinValue = new OracleNumber("-9.99999999999999999999999999999999999999E+125"); PrintByteConstant("OciNumberValue_MinValue", MinValue._value); //----------------------------------------------------------------- FromInt32(errorHandle, -1, result); PrintByteConstant("OciNumberValue_MinusOne", result); //------------------------------------------------------------------ FromInt32(errorHandle, 1, result); PrintByteConstant("OciNumberValue_One", result); //----------------------------------------------------------------- UnsafeNativeMethods.OCINumberSetPi( errorHandle, // err result // result ); PrintByteConstant("OciNumberValue_Pi", result); //----------------------------------------------------------------- FromInt32(errorHandle, 2, result); PrintByteConstant("OciNumberValue_Two", result); //----------------------------------------------------------------- FromInt32(errorHandle, 2, result); UnsafeNativeMethods.OCINumberIntPower( errorHandle, // err result, // base 64, // exp result // result ); PrintByteConstant("OciNumberValue_TwoPow64", result); //------------------------------------------------------------------ UnsafeNativeMethods.OCINumberSetZero( errorHandle, // err result // result ); PrintByteConstant("OciNumberValue_Zero", result); //----------------------------------------------------------------- } static void PrintByteConstant(string name, byte[] value) { Console.Write(String.Format("private static readonly byte[] {0,-25}= {{ 0x{1,-2:x2}", name, value[0])); for (int i = 1; i <= value[0]; i++) { Console.Write(String.Format(", 0x{0,-2:x2}", value[i])); } Console.WriteLine(" };"); } #endif //GENERATENUMBERCONSTANTS private byte[] _value; // null == value is null public static readonly OracleNumber E = new OracleNumber(OciNumberValue_E); public static readonly Int32 MaxPrecision = 38; public static readonly Int32 MaxScale = 127; public static readonly Int32 MinScale = -84; public static readonly OracleNumber MaxValue = new OracleNumber(OciNumberValue_MaxValue); public static readonly OracleNumber MinValue = new OracleNumber(OciNumberValue_MinValue); public static readonly OracleNumber MinusOne = new OracleNumber(OciNumberValue_MinusOne); public static readonly OracleNumber Null= new OracleNumber(true); public static readonly OracleNumber One = new OracleNumber(OciNumberValue_One); public static readonly OracleNumber PI = new OracleNumber(OciNumberValue_Pi); public static readonly OracleNumber Zero= new OracleNumber(OciNumberValue_Zero); // (internal) Construct from nothing private OracleNumber(bool isNull) { _value = (isNull) ? null : new byte[22]; } // (internal) Construct from a constant byte array private OracleNumber(byte[] bits) { _value = bits; } // Construct from System.Decimal public OracleNumber (Decimal decValue) : this (false) { OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); FromDecimal(errorHandle, decValue, _value); } // Construct from System.Double public OracleNumber (double dblValue) : this (false) { OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); FromDouble(errorHandle, dblValue, _value); } // Construct from System.Int32 public OracleNumber (Int32 intValue) : this (false) { OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); FromInt32(errorHandle, intValue, _value); } // Construct from System.Int64 public OracleNumber (Int64 longValue) : this (false) { OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); FromInt64(errorHandle, longValue, _value); } // Copy constructor public OracleNumber (OracleNumber from) { byte[] fromvalue = from._value; if (null != fromvalue) _value = (byte[])fromvalue.Clone(); else _value = null; } // (internal) Construct from System.String internal OracleNumber (string s) : this (false) { OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); FromString(errorHandle, s, _value); } // (internal) construct from a row/parameter binding internal OracleNumber (NativeBuffer buffer, int valueOffset) : this (false) { buffer.ReadBytes(valueOffset, _value, 0, 22); } public bool IsNull { get { return (null == _value); } } public Decimal Value { get { return (Decimal)this; } } public int CompareTo (object obj) { if (obj.GetType() == typeof(OracleNumber)) { OracleNumber value2 = (OracleNumber)obj; // If both values are Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) { return value2.IsNull ? 0 : -1; } if (value2.IsNull) { return 1; } // Neither value is null, do the comparison. OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); int result = InternalCmp(errorHandle, _value, value2._value); return result; } throw ADP.WrongType(obj.GetType(), typeof(OracleNumber)); } public override bool Equals(object value) { if (value is OracleNumber) return (this == (OracleNumber)value).Value; else return false; } public override int GetHashCode() { return IsNull ? 0 : _value.GetHashCode(); } static internal decimal MarshalToDecimal (NativeBuffer buffer, int valueOffset, OracleConnection connection) { byte[] value = buffer.ReadBytes(valueOffset, 22); OciErrorHandle errorHandle = connection.ErrorHandle; decimal result = ToDecimal(errorHandle, value); return result; } static internal int MarshalToInt32 (NativeBuffer buffer, int valueOffset, OracleConnection connection) { byte[] value = buffer.ReadBytes(valueOffset, 22); OciErrorHandle errorHandle = connection.ErrorHandle; int result = ToInt32(errorHandle, value); return result; } static internal long MarshalToInt64 (NativeBuffer buffer, int valueOffset, OracleConnection connection) { byte[] value = buffer.ReadBytes(valueOffset, 22); OciErrorHandle errorHandle = connection.ErrorHandle; long result = ToInt64(errorHandle, value); return result; } static internal int MarshalToNative (object value, NativeBuffer buffer, int offset, OracleConnection connection) { byte[] from; if ( value is OracleNumber ) { from = ((OracleNumber)value)._value; } else { OciErrorHandle errorHandle = connection.ErrorHandle; from = new byte[22]; if ( value is Decimal ) { FromDecimal(errorHandle, (decimal)value, from); } else if ( value is int ) { FromInt32(errorHandle, (int)value, from); } else if ( value is long ) { FromInt64(errorHandle, (long)value, from); } else { //if ( value is double ) FromDouble(errorHandle, (double)value, from); } } buffer.WriteBytes(offset, from, 0, 22); return 22; } public static OracleNumber Parse(string s) { if (null == s) { throw ADP.ArgumentNull("s"); } return new OracleNumber(s); } //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Internal Operators (used in the conversion routines) // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// private static void InternalAdd (OciErrorHandle errorHandle, byte[] x, byte[] y, byte[] result) { int rc = UnsafeNativeMethods.OCINumberAdd( errorHandle, // err x, // number1 y, // number2 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static int InternalCmp (OciErrorHandle errorHandle, byte[] value1, byte[] value2) { int result; int rc = UnsafeNativeMethods.OCINumberCmp( errorHandle, // err value1, // number1 value2, // number2 out result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } private static void InternalDiv (OciErrorHandle errorHandle, byte[] x, byte[] y, byte[] result) { int rc = UnsafeNativeMethods.OCINumberDiv( errorHandle, // err x, // number1 y, // number2 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static bool InternalIsInt (OciErrorHandle errorHandle, byte[] n) { int isInt; int rc = UnsafeNativeMethods.OCINumberIsInt( errorHandle, // err n, // number out isInt // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return (0 != isInt); } private static void InternalMod (OciErrorHandle errorHandle, byte[] x, byte[] y, byte[] result) { int rc = UnsafeNativeMethods.OCINumberMod( errorHandle, // err x, // number1 y, // number2 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void InternalMul (OciErrorHandle errorHandle, byte[] x, byte[] y, byte[] result) { int rc = UnsafeNativeMethods.OCINumberMul( errorHandle, // err x, // number1 y, // number2 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void InternalNeg (OciErrorHandle errorHandle, byte[] x, byte[] result) { int rc = UnsafeNativeMethods.OCINumberNeg( errorHandle, // err x, // number1 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static int InternalSign (OciErrorHandle errorHandle, byte[] n) { int sign; int rc = UnsafeNativeMethods.OCINumberSign( errorHandle, // err n, // number out sign // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return sign; } private static void InternalShift (OciErrorHandle errorHandle, byte[] n, int digits, byte[] result) { int rc = UnsafeNativeMethods.OCINumberShift( errorHandle, // err n, // nDig digits, // nDig result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void InternalSub (OciErrorHandle errorHandle, byte[] x, byte[] y, byte[] result) { int rc = UnsafeNativeMethods.OCINumberSub( errorHandle, // err x, // number1 y, // number2 result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void InternalTrunc (OciErrorHandle errorHandle, byte[] n, int position, byte[] result) { int rc = UnsafeNativeMethods.OCINumberTrunc( errorHandle, // err n, // number position, // decplace result // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void FromDecimal (OciErrorHandle errorHandle, decimal decimalValue, byte[] result) { int[] unpackedDecimal = Decimal.GetBits(decimalValue); ulong lowMidPart = ((ulong)((uint)unpackedDecimal[1]) << 32) | (ulong)((uint)unpackedDecimal[0]); uint highPart = (uint)unpackedDecimal[2]; int sign = (unpackedDecimal[3] >> 31); int scale = ((unpackedDecimal[3] >> 16) & 0x7f); FromUInt64(errorHandle, lowMidPart, result); if (0 != highPart) { // Bummer, the value is larger than an Int64... byte[] temp = new byte[22]; FromUInt32 (errorHandle, highPart, temp); InternalMul (errorHandle, temp, OciNumberValue_TwoPow64, temp); InternalAdd (errorHandle, result, temp, result); } // If the sign bit indicates negative, negate the result; if (0 != sign) { InternalNeg (errorHandle, result, result); } // Adjust for a scale value. if (0 != scale) { InternalShift(errorHandle, result, -scale, result); } } private static void FromDouble (OciErrorHandle errorHandle, double dblValue, byte[] result) { if (dblValue < doubleMinValue || dblValue > doubleMaxValue) { throw ADP.OperationResultedInOverflow(); } int rc = UnsafeNativeMethods.OCINumberFromReal( errorHandle, // err ref dblValue, // rnum 8, // rnum_length result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void FromInt32 (OciErrorHandle errorHandle, int intValue, byte[] result) { int rc = UnsafeNativeMethods.OCINumberFromInt( errorHandle, // err ref intValue, // inum 4, // inum_length OCI.SIGN.OCI_NUMBER_SIGNED, // inum_s_flag result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void FromUInt32 (OciErrorHandle errorHandle, UInt32 uintValue, byte[] result) { int rc = UnsafeNativeMethods.OCINumberFromInt( errorHandle, // err ref uintValue, // inum 4, // inum_length OCI.SIGN.OCI_NUMBER_UNSIGNED,//inum_s_flag result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void FromInt64 (OciErrorHandle errorHandle, long longValue, byte[] result) { int rc = UnsafeNativeMethods.OCINumberFromInt( errorHandle, // err ref longValue, // inum 8, // inum_length OCI.SIGN.OCI_NUMBER_SIGNED, // inum_s_flag result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } private static void FromUInt64 (OciErrorHandle errorHandle, UInt64 ulongValue, byte[] result) { int rc = UnsafeNativeMethods.OCINumberFromInt( errorHandle, // err ref ulongValue, // inum 8, // inum_length OCI.SIGN.OCI_NUMBER_UNSIGNED,//inum_s_flag result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } //3 NOTE: While Oracle will dish out up to 64 characters if you use the //3 "TM9" format string, they don't accept the "TM9" format string when //3 they're unformatting and they'll only accept 63 characters in the format //3 string you pass to OCINumberFromText. // "....+....1....+....2....+....3....+....4....+....5....+....6...." private const string WholeDigitPattern = "999999999999999999999999999999999999999999999999999999999999999"; private const int WholeDigitPattern_Length = 63; private void FromStringOfDigits(OciErrorHandle errorHandle, string s, byte[] result) { if (s.Length <= WholeDigitPattern_Length) { int rc = UnsafeNativeMethods.OCINumberFromText( errorHandle, // err s, // str unchecked((uint)s.Length), // str_length WholeDigitPattern, // fmt WholeDigitPattern_Length, // fmt_length IntPtr.Zero, // nls_params 0, // nls_p_length result // number ); if (0 != rc) { OracleException.Check(errorHandle, rc); } } else { byte[] temp = new byte[22]; string leftPart = s.Substring(0,WholeDigitPattern_Length); string rightPart = s.Substring(WholeDigitPattern_Length); FromStringOfDigits(errorHandle, leftPart, temp); FromStringOfDigits(errorHandle, rightPart, result); InternalShift(errorHandle, temp, rightPart.Length, temp); InternalAdd(errorHandle, result, temp, result); } } private void FromString (OciErrorHandle errorHandle, string s, byte[] result) { // DEVNOTE: this only supports the format [{+/-}]digits.digits[E[{+/-}]exponent], // that is all that SQLNumeric supports, so that's all I'll support. It // is also all that the ToString method on this class will produce... byte[] temp = new byte[22]; int exponent = 0; s = s.Trim(); int exponentAt = s.IndexOfAny("eE".ToCharArray()); if (exponentAt > 0) { exponent = Int32.Parse(s.Substring(exponentAt+1), CultureInfo.InvariantCulture); s = s.Substring(0,exponentAt); } bool isNegative = false; if ('-' == s[0]) { isNegative = true; s = s.Substring(1); } else if ('+' == s[0]) { s = s.Substring(1); } int decimalPointAt = s.IndexOf('.'); if (0 <= decimalPointAt) { string fractionalDigits = s.Substring(decimalPointAt+1); FromStringOfDigits(errorHandle, fractionalDigits, result); InternalShift(errorHandle, result, -fractionalDigits.Length, result); if (0 != decimalPointAt) { FromStringOfDigits(errorHandle, s.Substring(0,decimalPointAt), temp); InternalAdd(errorHandle, result, temp, result); } } else { FromStringOfDigits(errorHandle, s, result); } if (0 != exponent) { InternalShift(errorHandle, result, exponent, result); } if (isNegative) { InternalNeg(errorHandle, result, result); } GC.KeepAlive(s); } private static Decimal ToDecimal (OciErrorHandle errorHandle, byte[] value) { byte[] temp1 = (byte[])value.Clone(); byte[] temp2 = new byte[22]; byte scale = 0; int sign = InternalSign(errorHandle, temp1); if (sign < 0) { InternalNeg(errorHandle, temp1, temp1); } if ( !InternalIsInt(errorHandle, temp1) ) { // DEVNOTE: I happen to know how to figure out how many decimal places the value has, // but it means cracking the value. Oracle doesn't provide API support to // tell us how many decimal digits there are in a nubmer int decimalShift = 2 * (temp1[0] - ((temp1[1] & 0x7f)-64) - 1); InternalShift(errorHandle, temp1, decimalShift, temp1); scale += (byte)decimalShift; while( !InternalIsInt(errorHandle, temp1) ) { InternalShift(errorHandle, temp1, 1, temp1); scale++; } } InternalMod(errorHandle, temp1, OciNumberValue_TwoPow64, temp2); ulong loMid = ToUInt64(errorHandle, temp2); InternalDiv(errorHandle, temp1, OciNumberValue_TwoPow64, temp2); InternalTrunc(errorHandle, temp2, 0, temp2); uint hi = ToUInt32(errorHandle, temp2); Decimal decimalValue = new Decimal( (int)(loMid & 0xffffffff),// lo (int)(loMid >> 32), // mid (int)hi, // hi (sign < 0), // isNegative scale // scale ); return decimalValue; } private static int ToInt32 (OciErrorHandle errorHandle, byte[] value) { int result; int rc = UnsafeNativeMethods.OCINumberToInt( errorHandle, // err value, // number 4, // rsl_length OCI.SIGN.OCI_NUMBER_SIGNED, // rsl_flag out result // rsl ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } private static uint ToUInt32 (OciErrorHandle errorHandle, byte[] value) { uint result; int rc = UnsafeNativeMethods.OCINumberToInt( errorHandle, // err value, // number 4, // rsl_length OCI.SIGN.OCI_NUMBER_UNSIGNED,//rsl_flag out result // rsl ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } private static long ToInt64 (OciErrorHandle errorHandle, byte[] value) { long result; int rc = UnsafeNativeMethods.OCINumberToInt( errorHandle, // err value, // number 8, // rsl_length OCI.SIGN.OCI_NUMBER_SIGNED, // rsl_flag out result // rsl ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } private static ulong ToUInt64 (OciErrorHandle errorHandle, byte[] value) { ulong result; int rc = UnsafeNativeMethods.OCINumberToInt( errorHandle, // err value, // number 8, // rsl_length OCI.SIGN.OCI_NUMBER_UNSIGNED,//rsl_flag out result // rsl ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } static private string ToString (OciErrorHandle errorHandle, byte[] value) { byte[] buffer = new byte[64]; uint bufferLen = unchecked((uint)buffer.Length); int rc = UnsafeNativeMethods.OCINumberToText( errorHandle, // err value, // number "TM9", // fmt 3, // fmt_length IntPtr.Zero, // nls_params 0, // nls_p_length ref bufferLen, // buf_size buffer // buf ); if (0 != rc) { OracleException.Check(errorHandle, rc); } // Wonder of wonders, Oracle has a problem with the value -999999999999999999999999.9999 // where it seems that has trailing ':' characters if you their translator // method; we deal with it by removing the ':' characters. int realBufferLen = Array.IndexOf(buffer, (byte)58); // Oracle doesn't set the buffer length correctly; they include the null bytes // that trail the actual value. That means it's up to us to remove them. realBufferLen = (realBufferLen > 0) ? realBufferLen : Array.LastIndexOf(buffer, 0); // Technically, we should use Oracle's conversion OCICharsetToUnicode, but since numeric // digits are ANSI digits, we're safe here. string retval = System.Text.Encoding.Default.GetString(buffer, 0, (realBufferLen > 0) ? realBufferLen : checked((int)bufferLen)); return retval; } public override string ToString() { if (IsNull){ return ADP.NullString; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); string retval = ToString(errorHandle, _value); return retval; } public static OracleBoolean operator== (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) == 0); } public static OracleBoolean operator> (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) > 0); } public static OracleBoolean operator>= (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) >= 0); } public static OracleBoolean operator< (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) < 0); } public static OracleBoolean operator<= (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) <= 0); } public static OracleBoolean operator!= (OracleNumber x, OracleNumber y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) != 0); } public static OracleNumber operator- (OracleNumber x) { if (x.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalNeg(errorHandle, x._value, result._value); return result; } public static OracleNumber operator+ (OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalAdd(errorHandle,x._value,y._value,result._value); return result; } public static OracleNumber operator- (OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalSub(errorHandle,x._value,y._value,result._value); return result; } public static OracleNumber operator* (OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalMul(errorHandle, x._value, y._value, result._value); return result; } public static OracleNumber operator/ (OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalDiv(errorHandle, x._value, y._value, result._value); return result; } public static OracleNumber operator% (OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalMod(errorHandle, x._value, y._value, result._value); return result; } public static explicit operator Decimal(OracleNumber x) { if (x.IsNull) { throw ADP.DataIsNull(); } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); Decimal result = ToDecimal(errorHandle, x._value); return result; } public static explicit operator Double(OracleNumber x) { if (x.IsNull) { throw ADP.DataIsNull(); } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); double result; int rc = UnsafeNativeMethods.OCINumberToReal( errorHandle, // err x._value, // number 8, // rsl_length out result // rsl ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } public static explicit operator Int32(OracleNumber x) { if (x.IsNull) { throw ADP.DataIsNull(); } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); Int32 result = ToInt32(errorHandle, x._value); return result; } public static explicit operator Int64(OracleNumber x) { if (x.IsNull) { throw ADP.DataIsNull(); } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); Int64 result = ToInt64(errorHandle, x._value); return result; } public static explicit operator OracleNumber(Decimal x) { return new OracleNumber(x); } public static explicit operator OracleNumber(double x) { return new OracleNumber(x); } public static explicit operator OracleNumber(int x) { return new OracleNumber(x); } public static explicit operator OracleNumber(long x) { return new OracleNumber(x); } public static explicit operator OracleNumber(string x) { return new OracleNumber(x); } //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Built In Functions // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// //---------------------------------------------------------------------- // Abs - absolute value // public static OracleNumber Abs(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberAbs( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Acos - Get the Arc Cosine of the number // public static OracleNumber Acos(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberArcCos( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Add - Alternative method for operator + // public static OracleNumber Add(OracleNumber x, OracleNumber y) { return x + y; } //---------------------------------------------------------------------- // Asin - Get the Arc Sine of the number // public static OracleNumber Asin(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberArcSin( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Atan - Get the Arc Tangent of the number // public static OracleNumber Atan(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberArcTan( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Atan2 - Get the Arc Tangent of twi numbers // public static OracleNumber Atan2(OracleNumber y, OracleNumber x) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberArcTan2( errorHandle, // err y._value, // number x._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Ceiling - next smallest integer greater than or equal to the numeric // public static OracleNumber Ceiling(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberCeil( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Cos - Get the Cosine of the number // public static OracleNumber Cos(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberCos( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Cosh - Get the Hyperbolic Cosine of the number // public static OracleNumber Cosh(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberHypCos( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Divide - Alternative method for operator / // public static OracleNumber Divide(OracleNumber x, OracleNumber y) { return x / y; } //---------------------------------------------------------------------- // Equals - Alternative method for operator == // public static OracleBoolean Equals(OracleNumber x, OracleNumber y) { return (x == y); } //--------------------------------------------------------------------- // Exp - raise e to the specified power // public static OracleNumber Exp(OracleNumber p) { if (p.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberExp( errorHandle, // err p._value, // base result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Floor - next largest integer smaller or equal to the numeric // public static OracleNumber Floor(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberFloor( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // GreaterThan - Alternative method for operator > // public static OracleBoolean GreaterThan(OracleNumber x, OracleNumber y) { return (x > y); } //--------------------------------------------------------------------- // GreaterThanOrEqual - Alternative method for operator >= // public static OracleBoolean GreaterThanOrEqual(OracleNumber x, OracleNumber y) { return (x >= y); } //--------------------------------------------------------------------- // LessThan - Alternative method for operator < // public static OracleBoolean LessThan(OracleNumber x, OracleNumber y) { return (x < y); } //---------------------------------------------------------------------- // LessThanOrEqual - Alternative method for operator <= // public static OracleBoolean LessThanOrEqual(OracleNumber x, OracleNumber y) { return (x <= y); } //--------------------------------------------------------------------- // Log - Compute the natural logarithm (base e) // public static OracleNumber Log(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberLn( errorHandle, // err n._value, // base result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Log - Compute the logarithm (any base) // public static OracleNumber Log(OracleNumber n, int newBase) { return Log(n, new OracleNumber(newBase)); } public static OracleNumber Log(OracleNumber n, OracleNumber newBase) { if (n.IsNull || newBase.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberLog( errorHandle, // err newBase._value, // base n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Log10 - Compute the logarithm (base 10) // public static OracleNumber Log10(OracleNumber n) { return Log(n, new OracleNumber(10)); } //--------------------------------------------------------------------- // Max - Get the maximum value of two Oracle Numbers // public static OracleNumber Max(OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } return (x > y) ? x : y; } //---------------------------------------------------------------------- // Min - Get the minimum value of two Oracle Numbers // public static OracleNumber Min(OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } return (x < y) ? x : y; } //--------------------------------------------------------------------- // Modulo - Alternative method for operator % // public static OracleNumber Modulo(OracleNumber x, OracleNumber y) { return x % y; } //--------------------------------------------------------------------- // Multiply - Alternative method for operator * // public static OracleNumber Multiply(OracleNumber x, OracleNumber y) { return x * y; } //--------------------------------------------------------------------- // Negate - Alternative method for unary operator - // public static OracleNumber Negate(OracleNumber x) { return -x; } //---------------------------------------------------------------------- // NotEquals - Alternative method for operator != // public static OracleBoolean NotEquals(OracleNumber x, OracleNumber y) { return (x != y); } //--------------------------------------------------------------------- // Pow - Compute the power of a numeric // public static OracleNumber Pow(OracleNumber x, Int32 y) { if (x.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberIntPower( errorHandle, // err x._value, // base y, // exp result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } public static OracleNumber Pow(OracleNumber x, OracleNumber y) { if (x.IsNull || y.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberPower( errorHandle, // err x._value, // base y._value, // exp result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Round - Round the numeric to a specific digit // public static OracleNumber Round(OracleNumber n, int position) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberRound( errorHandle, // err n._value, // number position, // decplace result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Shift - Shift the number of digits // public static OracleNumber Shift(OracleNumber n, int digits) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalShift(errorHandle, n._value, digits, result._value); return result; } //--------------------------------------------------------------------- // Sign - 1 if positive, -1 if negative // public static OracleNumber Sign(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); int sign = InternalSign(errorHandle, n._value); OracleNumber result = (sign > 0) ? One : MinusOne; return result; } //---------------------------------------------------------------------- // Sin - Get the Sine of the number // public static OracleNumber Sin(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberSin( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Sinh - Get the Hyperbolic Sine of the number // public static OracleNumber Sinh(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberHypSin( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Sqrt - Get the Square Root of the number // public static OracleNumber Sqrt(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberSqrt( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Subtract - Alternative method for operator - // public static OracleNumber Subtract(OracleNumber x, OracleNumber y) { return x - y; } //---------------------------------------------------------------------- // Tan - Get the Tangent of the number // public static OracleNumber Tan(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberTan( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //--------------------------------------------------------------------- // Tanh - Get the Hyperbolic Tangent of the number // public static OracleNumber Tanh(OracleNumber n) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); int rc = UnsafeNativeMethods.OCINumberHypTan( errorHandle, // err n._value, // number result._value // result ); if (0 != rc) { OracleException.Check(errorHandle, rc); } return result; } //---------------------------------------------------------------------- // Truncate - Truncate the numeric to a specific digit // public static OracleNumber Truncate(OracleNumber n, int position) { if (n.IsNull) { return Null; } OracleConnection.ExecutePermission.Demand(); OciErrorHandle errorHandle = TempEnvironment.GetErrorHandle(); OracleNumber result = new OracleNumber(false); InternalTrunc(errorHandle, n._value, position, result._value); return result; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
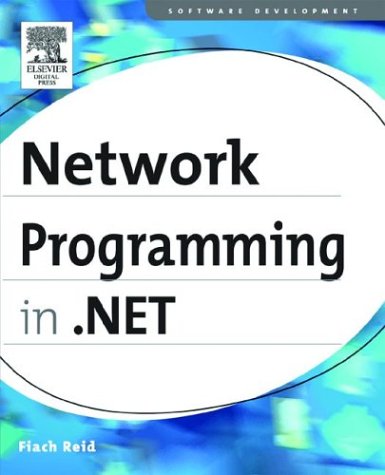
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WebConfigurationHost.cs
- RawStylusInputCustomData.cs
- ListBox.cs
- RequestBringIntoViewEventArgs.cs
- regiisutil.cs
- ClientFormsIdentity.cs
- DataGridViewTextBoxColumn.cs
- CryptoStream.cs
- PersonalizablePropertyEntry.cs
- FormViewModeEventArgs.cs
- ELinqQueryState.cs
- PerfService.cs
- ExpressionParser.cs
- Module.cs
- ListSourceHelper.cs
- HttpSysSettings.cs
- ResolveNameEventArgs.cs
- UncommonField.cs
- XmlAnyElementAttributes.cs
- ObjectDataSource.cs
- DataControlFieldCollection.cs
- DataSvcMapFile.cs
- Calendar.cs
- WebPartConnectionsDisconnectVerb.cs
- PathGradientBrush.cs
- LinqDataSourceSelectEventArgs.cs
- DataRelation.cs
- XPathExpr.cs
- FilterElement.cs
- System.Data_BID.cs
- XamlTreeBuilder.cs
- LastQueryOperator.cs
- ArrayElementGridEntry.cs
- PriorityQueue.cs
- ExpressionVisitor.cs
- WorkflowLayouts.cs
- WorkItem.cs
- HtmlLink.cs
- ConsumerConnectionPointCollection.cs
- Popup.cs
- ComplexType.cs
- Latin1Encoding.cs
- CreateUserWizard.cs
- OdbcException.cs
- SerializeAbsoluteContext.cs
- ExtendedPropertyCollection.cs
- X509Certificate2.cs
- ObjectSecurity.cs
- ControlCollection.cs
- ModelPerspective.cs
- bindurihelper.cs
- SimpleHandlerFactory.cs
- BitmapCodecInfo.cs
- BridgeDataRecord.cs
- sortedlist.cs
- ItemType.cs
- CalendarDataBindingHandler.cs
- BitHelper.cs
- QilTypeChecker.cs
- XNameConverter.cs
- NativeMethods.cs
- HttpCachePolicy.cs
- FormsIdentity.cs
- AsnEncodedData.cs
- OleDbFactory.cs
- SettingsPropertyNotFoundException.cs
- HtmlString.cs
- SqlBinder.cs
- RequestNavigateEventArgs.cs
- DataServiceQueryException.cs
- RunInstallerAttribute.cs
- RectangleConverter.cs
- WebAdminConfigurationHelper.cs
- RtfToXamlLexer.cs
- SingleAnimation.cs
- TextDecoration.cs
- Matrix.cs
- PathFigureCollection.cs
- AutoCompleteStringCollection.cs
- HtmlValidationSummaryAdapter.cs
- AutoResizedEvent.cs
- UmAlQuraCalendar.cs
- BuildProvidersCompiler.cs
- Type.cs
- WebPartDisplayMode.cs
- XmlNavigatorFilter.cs
- HelpProvider.cs
- SequentialOutput.cs
- EventProviderWriter.cs
- AttributeCollection.cs
- SiteMapNode.cs
- NullableFloatAverageAggregationOperator.cs
- RawStylusInput.cs
- FormViewInsertEventArgs.cs
- ContextMenu.cs
- ChtmlMobileTextWriter.cs
- SmiRequestExecutor.cs
- SafeSecurityHelper.cs
- ObjectStateFormatter.cs
- InputScope.cs