Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Shared / MS / Internal / XmlHelper.cs / 1 / XmlHelper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements some helper functions for Xml nodes. // //--------------------------------------------------------------------------- using System; using System.Xml; #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif namespace MS.Internal { [FriendAccessAllowed] static class XmlHelper { ////// Return true if the given item is an XML node. /// internal static bool IsXmlNode(object item) { if (item != null) { Type type = item.GetType(); return type.FullName.StartsWith("System.Xml", StringComparison.Ordinal) && IsXmlNodeHelper(item); } else return false; } // separate function to avoid JIT-ing System.Xml until we have a good reason private static bool IsXmlNodeHelper(object item) { return item is System.Xml.XmlNode; } ////// Return a string by applying an XPath query to an XmlNode. /// internal static string SelectStringValue(XmlNode node, string query) { return SelectStringValue(node, query, null); } ////// Return a string by applying an XPath query to an XmlNode. /// internal static string SelectStringValue(XmlNode node, string query, XmlNamespaceManager namespaceManager) { string strValue; XmlNode result; result = node.SelectSingleNode(query, namespaceManager); if (result != null) { strValue = XmlHelper.ExtractString(result); } else { strValue = String.Empty; } return strValue; } ////// Get a string from an XmlNode (of any kind: element, attribute, etc.) /// internal static string ExtractString(XmlNode node) { string value = ""; if (node.NodeType == XmlNodeType.Element) { for (int i = 0; i < node.ChildNodes.Count; i++) { if (node.ChildNodes[i].NodeType == XmlNodeType.Text) { value += node.ChildNodes[i].Value; } } } else { value = node.Value; } return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements some helper functions for Xml nodes. // //--------------------------------------------------------------------------- using System; using System.Xml; #if WINDOWS_BASE using MS.Internal.WindowsBase; #elif PRESENTATION_CORE using MS.Internal.PresentationCore; #elif PRESENTATIONFRAMEWORK using MS.Internal.PresentationFramework; #elif DRT using MS.Internal.Drt; #else #error Attempt to use FriendAccessAllowedAttribute from an unknown assembly. using MS.Internal.YourAssemblyName; #endif namespace MS.Internal { [FriendAccessAllowed] static class XmlHelper { ////// Return true if the given item is an XML node. /// internal static bool IsXmlNode(object item) { if (item != null) { Type type = item.GetType(); return type.FullName.StartsWith("System.Xml", StringComparison.Ordinal) && IsXmlNodeHelper(item); } else return false; } // separate function to avoid JIT-ing System.Xml until we have a good reason private static bool IsXmlNodeHelper(object item) { return item is System.Xml.XmlNode; } ////// Return a string by applying an XPath query to an XmlNode. /// internal static string SelectStringValue(XmlNode node, string query) { return SelectStringValue(node, query, null); } ////// Return a string by applying an XPath query to an XmlNode. /// internal static string SelectStringValue(XmlNode node, string query, XmlNamespaceManager namespaceManager) { string strValue; XmlNode result; result = node.SelectSingleNode(query, namespaceManager); if (result != null) { strValue = XmlHelper.ExtractString(result); } else { strValue = String.Empty; } return strValue; } ////// Get a string from an XmlNode (of any kind: element, attribute, etc.) /// internal static string ExtractString(XmlNode node) { string value = ""; if (node.NodeType == XmlNodeType.Element) { for (int i = 0; i < node.ChildNodes.Count; i++) { if (node.ChildNodes[i].NodeType == XmlNodeType.Text) { value += node.ChildNodes[i].Value; } } } else { value = node.Value; } return value; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
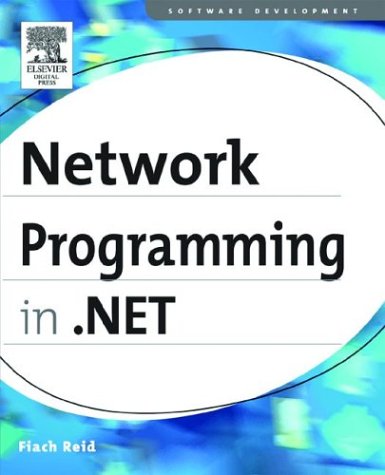
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OracleParameter.cs
- SecurityTokenReferenceStyle.cs
- TempFiles.cs
- XmlSerializationReader.cs
- FactoryRecord.cs
- XmlSchemaIdentityConstraint.cs
- Form.cs
- TemplateEditingVerb.cs
- Nullable.cs
- WindowsGraphicsWrapper.cs
- PathGeometry.cs
- ColumnCollection.cs
- ConfigXmlComment.cs
- HwndSubclass.cs
- ExpressionVisitor.cs
- ComponentEditorForm.cs
- lengthconverter.cs
- CustomError.cs
- ObjectParameterCollection.cs
- PackUriHelper.cs
- SchemaImporter.cs
- SelectionRange.cs
- CacheChildrenQuery.cs
- PathData.cs
- SqlConnectionString.cs
- UpDownEvent.cs
- HttpModulesSection.cs
- TaskResultSetter.cs
- StorageModelBuildProvider.cs
- TextRunCache.cs
- RelatedPropertyManager.cs
- SymbolTable.cs
- ReplyAdapterChannelListener.cs
- FunctionUpdateCommand.cs
- PropertyFilterAttribute.cs
- PropertyGridView.cs
- WindowsScrollBarBits.cs
- DeleteWorkflowOwnerCommand.cs
- ZipIOBlockManager.cs
- TreeNode.cs
- MinimizableAttributeTypeConverter.cs
- DeferredBinaryDeserializerExtension.cs
- OdbcEnvironmentHandle.cs
- MimeXmlReflector.cs
- precedingquery.cs
- EventLogWatcher.cs
- RedBlackList.cs
- SqlParameter.cs
- SqlXmlStorage.cs
- TimelineGroup.cs
- GeneralTransform3DGroup.cs
- MatrixTransform.cs
- MemoryRecordBuffer.cs
- mediapermission.cs
- Int32CollectionConverter.cs
- Input.cs
- ActivityInterfaces.cs
- AttachedAnnotationChangedEventArgs.cs
- COM2TypeInfoProcessor.cs
- GridViewSortEventArgs.cs
- Win32.cs
- ListControl.cs
- CacheChildrenQuery.cs
- WsatTransactionFormatter.cs
- DataGridViewCellMouseEventArgs.cs
- NetMsmqSecurityMode.cs
- DynamicFilterExpression.cs
- XhtmlTextWriter.cs
- CompiledIdentityConstraint.cs
- HttpCacheVaryByContentEncodings.cs
- ValidatingCollection.cs
- StylusPointCollection.cs
- WhitespaceRuleReader.cs
- ComplexTypeEmitter.cs
- XmlSchemaObject.cs
- SqlInternalConnectionSmi.cs
- GZipStream.cs
- HttpCookieCollection.cs
- DesignerWebPartChrome.cs
- Utility.cs
- VariableQuery.cs
- StrokeNode.cs
- precedingquery.cs
- Literal.cs
- Ray3DHitTestResult.cs
- Int16Converter.cs
- TransactionFilter.cs
- SystemException.cs
- NativeWindow.cs
- SHA1Managed.cs
- TextTreeTextElementNode.cs
- XamlBrushSerializer.cs
- SubpageParagraph.cs
- WizardPanel.cs
- XmlEntity.cs
- KeyMatchBuilder.cs
- BinaryMethodMessage.cs
- XAMLParseException.cs
- DataControlField.cs
- SQLInt16Storage.cs