Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Threading / Tasks / TaskResultSetter.cs / 1305376 / TaskResultSetter.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // TaskCompletionSource.cs // //[....] // // TaskCompletionSourceis the producer end of an unbound future. Its // Task member may be distributed as the consumer end of the future. // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System; using System.Security.Permissions; using System.Threading; using System.Diagnostics.Contracts; using System.Collections.Generic; // Disable the "reference to volatile field not treated as volatile" error. #pragma warning disable 0420 namespace System.Threading.Tasks { /// /// Represents the producer side of a ///unbound to a /// delegate, providing access to the consumer side through the property. /// /// ////// It is often the case that a ///is desired to /// represent another asynchronous operation. /// TaskCompletionSource is provided for this purpose. It enables /// the creation of a task that can be handed out to consumers, and those consumers can use the members /// of the task as they would any other. However, unlike most tasks, the state of a task created by a /// TaskCompletionSource is controlled explicitly by the methods on TaskCompletionSource. This enables the /// completion of the external asynchronous operation to be propagated to the underlying Task. The /// separation also ensures that consumers are not able to transition the state without access to the /// corresponding TaskCompletionSource. ////// All members of ///are thread-safe /// and may be used from multiple threads concurrently. /// The type of the result value assocatied with this [HostProtection(SecurityAction.LinkDemand, Synchronization = true, ExternalThreading = true)] public class TaskCompletionSource. { private Task m_task; /// /// Creates a public TaskCompletionSource() : this(null, TaskCreationOptions.None) { } ///. /// /// Creates a ////// with the specified options. /// /// The /// The options to use when creating the underlying ///created /// by this instance and accessible through its property /// will be instantiated using the specified . /// . /// /// The public TaskCompletionSource(TaskCreationOptions creationOptions) : this(null, creationOptions) { } ///represent options invalid for use /// with a . /// /// Creates a /// The state to use as the underlying ////// with the specified state. /// 's AsyncState. public TaskCompletionSource(object state) : this(state, TaskCreationOptions.None) { } /// /// Creates a /// The options to use when creating the underlying ///with /// the specified state and options. /// . /// The state to use as the underlying /// 's AsyncState. /// /// The public TaskCompletionSource(object state, TaskCreationOptions creationOptions) { m_task = new Taskrepresent options invalid for use /// with a . /// (state, CancellationToken.None, creationOptions, InternalTaskOptions.PromiseTask); } /// /// Gets the ///created /// by this . /// /// This property enables a consumer access to the public Taskthat is controlled by this instance. /// The , , /// , and /// methods (and their "Try" variants) on this instance all result in the relevant state /// transitions on this underlying Task. /// Task { get { return m_task; } } /// /// Attempts to transition the underlying /// /// The exception to bind to thisinto the /// Faulted /// state. ///. /// True if the operation was successful; otherwise, false. ///This operation will return false if the /// ///is already in one /// of the three final states: /// RanToCompletion , ///Faulted , or ///Canceled . ///The ///argument is null. The public bool TrySetException(Exception exception) { if (exception == null) throw new ArgumentNullException("exception"); bool rval = m_task.TrySetException(exception); if (!rval && !m_task.IsCompleted) { // The only way that m_task is not completed is if it is in a COMPLETION_RESERVED state. // Just wait a bit until the completion is finalized. SpinWait sw = new SpinWait(); while (!m_task.IsCompleted) sw.SpinOnce(); } return rval; } ///was disposed. /// Attempts to transition the underlying /// /// The collection of exceptions to bind to thisinto the /// Faulted /// state. ///. /// True if the operation was successful; otherwise, false. ///This operation will return false if the /// ///is already in one /// of the three final states: /// RanToCompletion , ///Faulted , or ///Canceled . ///The ///argument is null. There are one or more null elements in ///. The ///collection is empty. The public bool TrySetException(IEnumerablewas disposed. exceptions) { if (exceptions == null) throw new ArgumentNullException("exceptions"); List defensiveCopy = new List (); foreach (Exception e in exceptions) { if (e == null) throw new ArgumentException(Environment.GetResourceString("TaskCompletionSourceT_TrySetException_NullException"), "exceptions"); defensiveCopy.Add(e); } if (defensiveCopy.Count == 0) throw new ArgumentException(Environment.GetResourceString("TaskCompletionSourceT_TrySetException_NoExceptions"), "exceptions"); bool rval = m_task.TrySetException(defensiveCopy); if (!rval && !m_task.IsCompleted) { // The only way that m_task is not completed is if it is in a COMPLETION_RESERVED state. // Just wait a bit until the completion is finalized. SpinWait sw = new SpinWait(); while (!m_task.IsCompleted) sw.SpinOnce(); } return rval; } /// /// Transitions the underlying /// /// The exception to bind to thisinto the /// Faulted /// state. ///. /// The ///argument is null. /// The underlying ///is already in one /// of the three final states: /// RanToCompletion , ///Faulted , or ///Canceled . ///The public void SetException(Exception exception) { if (exception == null) throw new ArgumentNullException("exception"); if (!TrySetException(exception)) { throw new InvalidOperationException(Environment.GetResourceString("TaskT_TransitionToFinal_AlreadyCompleted")); } } ///was disposed. /// Transitions the underlying /// /// The collection of exceptions to bind to thisinto the /// Faulted /// state. ///. /// The ///argument is null. There are one or more null elements in ///. /// The underlying ///is already in one /// of the three final states: /// RanToCompletion , ///Faulted , or ///Canceled . ///The public void SetException(IEnumerablewas disposed. exceptions) { if (!TrySetException(exceptions)) { throw new InvalidOperationException(Environment.GetResourceString("TaskT_TransitionToFinal_AlreadyCompleted")); } } /// /// Attempts to transition the underlying /// /// The result value to bind to thisinto the /// RanToCompletion /// state. ///. /// True if the operation was successful; otherwise, false. ///This operation will return false if the /// ///is already in one /// of the three final states: /// RanToCompletion , ///Faulted , or ///Canceled . ///The public bool TrySetResult(TResult result) { // Attempt to quickly recognize failure. if (m_task.IsCompleted) { return false; } bool rval = m_task.TrySetResult(result); if(!rval && !m_task.IsCompleted) { // The only way that m_task is not completed is if it is in a COMPLETION_RESERVED state. // Just wait a bit until the completion is finalized. SpinWait sw = new SpinWait(); while (!m_task.IsCompleted) sw.SpinOnce(); } return rval; } ///was disposed. /// Transitions the underlying /// /// The result value to bind to thisinto the /// RanToCompletion /// state. ///. /// /// The underlying ///is already in one /// of the three final states: /// RanToCompletion , ///Faulted , or ///Canceled . ///The public void SetResult(TResult result) { m_task.Result = result; } ///was disposed. /// Transitions the underlying /// ///into the /// Canceled /// state. ////// The underlying ///is already in one /// of the three final states: /// RanToCompletion , ///Faulted , or ///Canceled . ///The public void SetCanceled() { if(!TrySetCanceled()) throw new InvalidOperationException(Environment.GetResourceString("TaskT_TransitionToFinal_AlreadyCompleted")); } ///was disposed. /// Attempts to transition the underlying /// ///into the /// Canceled /// state. ///True if the operation was successful; otherwise, false. ///This operation will return false if the /// ///is already in one /// of the three final states: /// RanToCompletion , ///Faulted , or ///Canceled . ///The public bool TrySetCanceled() { bool returnValue = false; // "Reserve" the completion for this task, while making sure that: (1) No prior reservation // has been made, (2) The result has not already been set, (3) An exception has not previously // been recorded, and (4) Cancellation has not been requested. // // If the reservation is successful, then record the cancellation and finish completion processing. // // Note: I had to access static Task variables through Taskwas disposed.
Link Menu
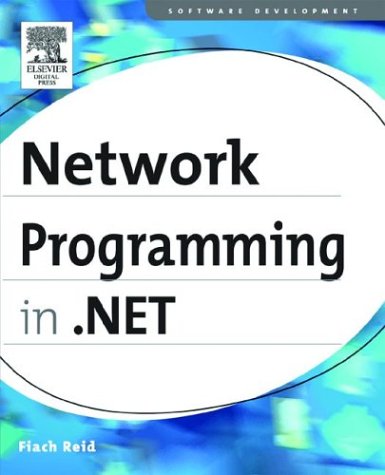
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewColumnDesigner.cs
- PageCache.cs
- RadioButtonStandardAdapter.cs
- ListViewGroup.cs
- UInt16Converter.cs
- ArrayTypeMismatchException.cs
- CodeDelegateInvokeExpression.cs
- XmlSchemaParticle.cs
- MessageQueue.cs
- ObjectAssociationEndMapping.cs
- DesignerVerbCollection.cs
- designeractionlistschangedeventargs.cs
- EntityObject.cs
- DetailsView.cs
- LinqDataSourceInsertEventArgs.cs
- OdbcConnectionHandle.cs
- DoubleCollectionConverter.cs
- CodeGotoStatement.cs
- X509IssuerSerialKeyIdentifierClause.cs
- TypeElement.cs
- RegexGroup.cs
- MetadataItemCollectionFactory.cs
- ConvertTextFrag.cs
- Label.cs
- DomNameTable.cs
- PropertyGridView.cs
- Converter.cs
- TimeoutException.cs
- KeyGesture.cs
- BitConverter.cs
- PropertyValueChangedEvent.cs
- HtmlTextArea.cs
- _NegotiateClient.cs
- ExitEventArgs.cs
- SpellerHighlightLayer.cs
- LabelTarget.cs
- SessionStateUtil.cs
- ObjectTokenCategory.cs
- Interlocked.cs
- OleDbPropertySetGuid.cs
- ListViewCancelEventArgs.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- WebPartHelpVerb.cs
- Keyboard.cs
- EmptyTextWriter.cs
- DataGrid.cs
- OleDbCommandBuilder.cs
- ImageMetadata.cs
- SecurityPolicySection.cs
- FileNotFoundException.cs
- SelectionProviderWrapper.cs
- SqlConnectionString.cs
- SpellerStatusTable.cs
- SqlFileStream.cs
- ProofTokenCryptoHandle.cs
- MemberAccessException.cs
- WhitespaceRuleLookup.cs
- CompensatableTransactionScopeActivityDesigner.cs
- HttpMethodAttribute.cs
- Version.cs
- MarkupObject.cs
- Pen.cs
- Geometry3D.cs
- RNGCryptoServiceProvider.cs
- ProfilePropertySettings.cs
- MessageQueuePermission.cs
- BuildProviderCollection.cs
- RowBinding.cs
- StyleModeStack.cs
- PrivilegeNotHeldException.cs
- RelationshipEndCollection.cs
- dtdvalidator.cs
- EntitySqlQueryState.cs
- FrameworkElementAutomationPeer.cs
- GroupBoxRenderer.cs
- DocobjHost.cs
- PeerToPeerException.cs
- HotCommands.cs
- SecurityPolicySection.cs
- InsufficientMemoryException.cs
- MouseEvent.cs
- Subtree.cs
- ConfigurationManagerInternal.cs
- FileSystemWatcher.cs
- UnsafeNativeMethods.cs
- GeometryHitTestResult.cs
- TreeNodeBindingCollection.cs
- TagNameToTypeMapper.cs
- StatusBar.cs
- SqlCacheDependencyDatabaseCollection.cs
- ObjectItemNoOpAssemblyLoader.cs
- SQLRoleProvider.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- ContractReference.cs
- FixedSOMSemanticBox.cs
- PeerApplicationLaunchInfo.cs
- XmlSchemaGroup.cs
- RSAOAEPKeyExchangeFormatter.cs
- WebPartConnectionsConnectVerb.cs
- ISessionStateStore.cs