Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / Regex / System / Text / RegularExpressions / RegexGroup.cs / 1 / RegexGroup.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // Group represents the substring or substrings that // are captured by a single capturing group after one // regular expression match. namespace System.Text.RegularExpressions { using System.Security.Permissions; ////// Group /// represents the results from a single capturing group. A capturing group can /// capture zero, one, or more strings in a single match because of quantifiers, so /// Group supplies a collection of Capture objects. /// [ Serializable() ] public class Group : Capture { // the empty group object internal static Group _emptygroup = new Group(String.Empty, new int[0], 0); internal int[] _caps; internal int _capcount; internal CaptureCollection _capcoll; internal Group(String text, int[] caps, int capcount) : base(text, capcount == 0 ? 0 : caps[(capcount - 1) * 2], capcount == 0 ? 0 : caps[(capcount * 2) - 1]) { _caps = caps; _capcount = capcount; } /* * True if the match was successful */ ////// public bool Success { get { return _capcount != 0; } } /* * The collection of all captures for this group */ ///Indicates whether the match is successful. ////// public CaptureCollection Captures { get { if (_capcoll == null) _capcoll = new CaptureCollection(this); return _capcoll; } } /* * Convert to a thread-safe object by precomputing cache contents */ ////// Returns a collection of all the captures matched by the capturing /// group, in innermost-leftmost-first order (or innermost-rightmost-first order if /// compiled with the "r" option). The collection may have zero or more items. /// ////// [HostProtection(Synchronization=true)] static public Group Synchronized(Group inner) { if (inner == null) throw new ArgumentNullException("inner"); // force Captures to be computed. CaptureCollection capcoll; Capture dummy; capcoll = inner.Captures; if (inner._capcount > 0) dummy = capcoll[0]; return inner; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Returns /// a Group object equivalent to the one supplied that is safe to share between /// multiple threads. ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- // Group represents the substring or substrings that // are captured by a single capturing group after one // regular expression match. namespace System.Text.RegularExpressions { using System.Security.Permissions; ////// Group /// represents the results from a single capturing group. A capturing group can /// capture zero, one, or more strings in a single match because of quantifiers, so /// Group supplies a collection of Capture objects. /// [ Serializable() ] public class Group : Capture { // the empty group object internal static Group _emptygroup = new Group(String.Empty, new int[0], 0); internal int[] _caps; internal int _capcount; internal CaptureCollection _capcoll; internal Group(String text, int[] caps, int capcount) : base(text, capcount == 0 ? 0 : caps[(capcount - 1) * 2], capcount == 0 ? 0 : caps[(capcount * 2) - 1]) { _caps = caps; _capcount = capcount; } /* * True if the match was successful */ ////// public bool Success { get { return _capcount != 0; } } /* * The collection of all captures for this group */ ///Indicates whether the match is successful. ////// public CaptureCollection Captures { get { if (_capcoll == null) _capcoll = new CaptureCollection(this); return _capcoll; } } /* * Convert to a thread-safe object by precomputing cache contents */ ////// Returns a collection of all the captures matched by the capturing /// group, in innermost-leftmost-first order (or innermost-rightmost-first order if /// compiled with the "r" option). The collection may have zero or more items. /// ////// [HostProtection(Synchronization=true)] static public Group Synchronized(Group inner) { if (inner == null) throw new ArgumentNullException("inner"); // force Captures to be computed. CaptureCollection capcoll; Capture dummy; capcoll = inner.Captures; if (inner._capcount > 0) dummy = capcoll[0]; return inner; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Returns /// a Group object equivalent to the one supplied that is safe to share between /// multiple threads. ///
Link Menu
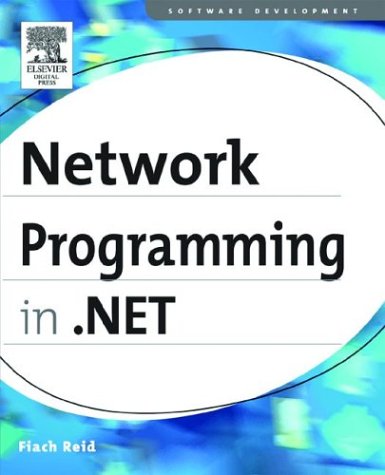
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StorageTypeMapping.cs
- DateTimeOffset.cs
- UIPropertyMetadata.cs
- CodePageEncoding.cs
- BaseEntityWrapper.cs
- ReferenceConverter.cs
- CodeEntryPointMethod.cs
- ByteAnimationBase.cs
- NamespaceEmitter.cs
- LoaderAllocator.cs
- TableItemStyle.cs
- SqlCachedBuffer.cs
- FormViewRow.cs
- ItemsChangedEventArgs.cs
- XPathItem.cs
- KerberosSecurityTokenProvider.cs
- CollectionViewProxy.cs
- SchemaObjectWriter.cs
- PersianCalendar.cs
- WindowClosedEventArgs.cs
- PersistChildrenAttribute.cs
- basevalidator.cs
- FragmentQuery.cs
- PackagePartCollection.cs
- TimeSpanParse.cs
- DataGridViewLayoutData.cs
- EntityKeyElement.cs
- FontEditor.cs
- DataListDesigner.cs
- DesignSurface.cs
- ColorMap.cs
- HttpApplicationFactory.cs
- PermissionAttributes.cs
- UserInitiatedNavigationPermission.cs
- SafeFileMapViewHandle.cs
- DesignerForm.cs
- OdbcCommandBuilder.cs
- Subtree.cs
- CompilerInfo.cs
- DataGridHeaderBorder.cs
- HttpRuntime.cs
- Header.cs
- UserPreferenceChangedEventArgs.cs
- PassportAuthentication.cs
- RootBrowserWindowProxy.cs
- Button.cs
- SystemException.cs
- ConnectionPointCookie.cs
- ErrorFormatter.cs
- TCPClient.cs
- Crc32Helper.cs
- WebControlsSection.cs
- Converter.cs
- UrlMapping.cs
- TableRowCollection.cs
- Module.cs
- RegexBoyerMoore.cs
- UrlPath.cs
- WebPartVerbCollection.cs
- Point3DCollectionValueSerializer.cs
- DesignTimeResourceProviderFactoryAttribute.cs
- TextProperties.cs
- PersonalizationDictionary.cs
- SqlOuterApplyReducer.cs
- HostedElements.cs
- NestPullup.cs
- StateManager.cs
- X509CertificateCollection.cs
- TabControlEvent.cs
- TypeResolvingOptions.cs
- DataAdapter.cs
- RangeBaseAutomationPeer.cs
- TextSpanModifier.cs
- XmlQueryRuntime.cs
- StateInitializationDesigner.cs
- CounterCreationData.cs
- ResourceBinder.cs
- ValidationManager.cs
- NamedElement.cs
- TextContainerChangedEventArgs.cs
- CodeMemberProperty.cs
- LingerOption.cs
- TransformDescriptor.cs
- DataKeyCollection.cs
- SoapFormatterSinks.cs
- OdbcCommand.cs
- PluralizationServiceUtil.cs
- WindowVisualStateTracker.cs
- XDRSchema.cs
- bidPrivateBase.cs
- FixedPageStructure.cs
- FunctionImportMapping.cs
- Baml2006Reader.cs
- InputLanguageProfileNotifySink.cs
- WebPartZoneBase.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- RSACryptoServiceProvider.cs
- CommandDevice.cs
- RightsManagementEncryptedStream.cs
- App.cs