Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Base / System / Windows / AttachedPropertyBrowsableWhenAttributePresentAttribute.cs / 1305600 / AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
namespace System.Windows { using System; using System.ComponentModel; ////// This attribute declares that an attached property can only be attached /// to an object that defines the given attribute on its class. /// [AttributeUsage(AttributeTargets.Method, AllowMultiple = false)] public sealed class AttachedPropertyBrowsableWhenAttributePresentAttribute : AttachedPropertyBrowsableAttribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Creates a new AttachedPropertyBrowsableWhenAttributePresentAttribute. Provide /// the type of attribute that, when present on a dependency object, /// should make the property browsable. /// public AttachedPropertyBrowsableWhenAttributePresentAttribute(Type attributeType) { if (attributeType == null) throw new ArgumentNullException("attributeType"); _attributeType = attributeType; } //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// Returns the attribute type passed into the constructor. /// public Type AttributeType { get { return _attributeType; } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Overrides Object.Equals to implement correct equality semantics for this /// attribute. /// public override bool Equals(object obj) { AttachedPropertyBrowsableWhenAttributePresentAttribute other = obj as AttachedPropertyBrowsableWhenAttributePresentAttribute; if (other == null) return false; return _attributeType == other._attributeType; } ////// Overrides Object.GetHashCode to implement correct hashing semantics. /// public override int GetHashCode() { return _attributeType.GetHashCode(); } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ ////// Returns true if the dependency object class defines an attribute /// of the same type contained in this attribute. The attribute must /// differ from the "default" state of the attribute. /// internal override bool IsBrowsable(DependencyObject d, DependencyProperty dp) { if (d == null) throw new ArgumentNullException("d"); if (dp == null) throw new ArgumentNullException("dp"); Attribute a = TypeDescriptor.GetAttributes(d)[_attributeType]; return (a != null && !a.IsDefaultAttribute()); } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Type _attributeType; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Windows { using System; using System.ComponentModel; ////// This attribute declares that an attached property can only be attached /// to an object that defines the given attribute on its class. /// [AttributeUsage(AttributeTargets.Method, AllowMultiple = false)] public sealed class AttachedPropertyBrowsableWhenAttributePresentAttribute : AttachedPropertyBrowsableAttribute { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- ////// Creates a new AttachedPropertyBrowsableWhenAttributePresentAttribute. Provide /// the type of attribute that, when present on a dependency object, /// should make the property browsable. /// public AttachedPropertyBrowsableWhenAttributePresentAttribute(Type attributeType) { if (attributeType == null) throw new ArgumentNullException("attributeType"); _attributeType = attributeType; } //----------------------------------------------------- // // Public Properties // //----------------------------------------------------- ////// Returns the attribute type passed into the constructor. /// public Type AttributeType { get { return _attributeType; } } //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- ////// Overrides Object.Equals to implement correct equality semantics for this /// attribute. /// public override bool Equals(object obj) { AttachedPropertyBrowsableWhenAttributePresentAttribute other = obj as AttachedPropertyBrowsableWhenAttributePresentAttribute; if (other == null) return false; return _attributeType == other._attributeType; } ////// Overrides Object.GetHashCode to implement correct hashing semantics. /// public override int GetHashCode() { return _attributeType.GetHashCode(); } //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ ////// Returns true if the dependency object class defines an attribute /// of the same type contained in this attribute. The attribute must /// differ from the "default" state of the attribute. /// internal override bool IsBrowsable(DependencyObject d, DependencyProperty dp) { if (d == null) throw new ArgumentNullException("d"); if (dp == null) throw new ArgumentNullException("dp"); Attribute a = TypeDescriptor.GetAttributes(d)[_attributeType]; return (a != null && !a.IsDefaultAttribute()); } //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ private Type _attributeType; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
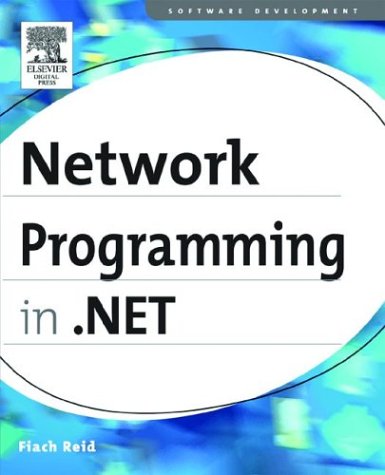
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StylusShape.cs
- ResourceDescriptionAttribute.cs
- DataGridViewColumnHeaderCell.cs
- AttributeEmitter.cs
- UriScheme.cs
- NegotiateStream.cs
- RequestValidator.cs
- UnauthorizedWebPart.cs
- DebugHandleTracker.cs
- SecUtil.cs
- DataSourceControl.cs
- ConsoleKeyInfo.cs
- VideoDrawing.cs
- NativeCompoundFileAPIs.cs
- ToolStripItemImageRenderEventArgs.cs
- SynchronizedInputHelper.cs
- keycontainerpermission.cs
- WebConfigManager.cs
- __TransparentProxy.cs
- VScrollProperties.cs
- UriTemplateClientFormatter.cs
- bindurihelper.cs
- PathFigureCollectionValueSerializer.cs
- RolePrincipal.cs
- AuthenticateEventArgs.cs
- CalendarKeyboardHelper.cs
- ThreadStartException.cs
- EasingFunctionBase.cs
- IndexerNameAttribute.cs
- XdrBuilder.cs
- SafeIUnknown.cs
- Freezable.cs
- QueryTask.cs
- StringConcat.cs
- DependencyPropertyHelper.cs
- OdbcEnvironmentHandle.cs
- XmlDataProvider.cs
- SubclassTypeValidator.cs
- ListParaClient.cs
- TextHintingModeValidation.cs
- OperatingSystem.cs
- Activator.cs
- EmptyElement.cs
- RayHitTestParameters.cs
- CurrentChangingEventArgs.cs
- PointAnimationBase.cs
- WebPartConnectionsEventArgs.cs
- DesignerWithHeader.cs
- XmlILCommand.cs
- SHA256Managed.cs
- SiteMapDataSourceView.cs
- SqlNodeAnnotation.cs
- PngBitmapDecoder.cs
- StyleConverter.cs
- AssemblyCollection.cs
- DataList.cs
- QilParameter.cs
- AuthenticationServiceManager.cs
- ProgressBarRenderer.cs
- Function.cs
- PointHitTestResult.cs
- HttpPostedFile.cs
- PositiveTimeSpanValidatorAttribute.cs
- EventProviderWriter.cs
- InputBuffer.cs
- CommandBinding.cs
- DocumentPageViewAutomationPeer.cs
- TextElement.cs
- AQNBuilder.cs
- WebPartMenu.cs
- TextTreeInsertElementUndoUnit.cs
- EarlyBoundInfo.cs
- SqlProvider.cs
- ArrayExtension.cs
- ScriptRegistrationManager.cs
- ScriptingRoleServiceSection.cs
- SqlNodeAnnotations.cs
- webproxy.cs
- DataGridItemAttachedStorage.cs
- StatusBarItem.cs
- PrimitiveXmlSerializers.cs
- BinaryHeap.cs
- LogEntryHeaderv1Deserializer.cs
- FontCollection.cs
- XmlIlTypeHelper.cs
- DataKeyArray.cs
- HandlerMappingMemo.cs
- TabControl.cs
- COM2PropertyDescriptor.cs
- InternalUserCancelledException.cs
- _TLSstream.cs
- DesignTimeSiteMapProvider.cs
- WebPartConnectionsCancelVerb.cs
- TraceSource.cs
- MessageDirection.cs
- GPRECT.cs
- DeviceFiltersSection.cs
- WebPartEditVerb.cs
- BStrWrapper.cs
- XmlCustomFormatter.cs