Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / clr / src / ManagedLibraries / SoapSerializer / SoapFormatter.cs / 1 / SoapFormatter.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //============================================================ // // Class: SoapFormatter //// Author: Peter de Jong ([....]) // // Purpose: Soap XML Formatter // // Date: June 10, 1999 // //=========================================================== #if FEATURE_COMINTEROP // These two attributes are for supporting side-by-side of COM-visible // objects with NDP 1.0 RTM. This needs to be set on all assemblies that // expose COM-visible types to be made Side by Side with NDP 1.0 RTM. // This declaration covers System.Runtime.Serialization.Formatters.Soap.dll [assembly:System.Runtime.InteropServices.ComCompatibleVersion(1,0,3300,0)] #endif // FEATURE_COMINTEROP namespace System.Runtime.Serialization.Formatters.Soap { using System; using System.Runtime.Serialization.Formatters; using System.IO; using System.Reflection; using System.Globalization; using System.Collections; using System.Runtime.Serialization; using System.Runtime.Remoting; using System.Runtime.Remoting.Messaging; using System.Text; sealed public class SoapFormatter : IRemotingFormatter { private SoapParser soapParser = null; private ISurrogateSelector m_surrogates; private StreamingContext m_context; private FormatterTypeStyle m_typeFormat = FormatterTypeStyle.TypesWhenNeeded; private ISoapMessage m_topObject = null; //private FormatterAssemblyStyle m_assemblyFormat = FormatterAssemblyStyle.Simple; private FormatterAssemblyStyle m_assemblyFormat = FormatterAssemblyStyle.Full; private TypeFilterLevel m_securityLevel = TypeFilterLevel.Full; private SerializationBinder m_binder; private Stream currentStream = null; // Property which specifies an object of type ISoapMessage into which // the SoapTop object is serialized. Should only be used if the Soap // top record is a methodCall or methodResponse element. public ISoapMessage TopObject { get {return m_topObject;} set {m_topObject = value;} } // Property which specifies how types are serialized, // FormatterTypeStyle Enum specifies options public FormatterTypeStyle TypeFormat { get {return m_typeFormat;} set { // Reset the value if TypesWhenNeeded // Or the value for TypesAlways and XsdString if (value == FormatterTypeStyle.TypesWhenNeeded) m_typeFormat = FormatterTypeStyle.TypesWhenNeeded; else m_typeFormat |= value; } } // Property which specifies how types are serialized, // FormatterAssemblyStyle Enum specifies options public FormatterAssemblyStyle AssemblyFormat { get {return m_assemblyFormat;} set {m_assemblyFormat = value;} } // Property which specifies the security level of formatter // TypeFilterLevel Enum specifies options public TypeFilterLevel FilterLevel { get {return m_securityLevel;} set {m_securityLevel = value;} } // Constructor public SoapFormatter() { m_surrogates=null; m_context = new StreamingContext(StreamingContextStates.All); } // Constructor public SoapFormatter(ISurrogateSelector selector, StreamingContext context) { m_surrogates = selector; m_context = context; } // Deserialize the stream into an object graph. public Object Deserialize(Stream serializationStream) { return Deserialize(serializationStream, null); } // Deserialize the stream into an object graph. public Object Deserialize(Stream serializationStream, HeaderHandler handler) { InternalST.InfoSoap("Enter SoapFormatter.Deserialize "); if (serializationStream==null) { throw new ArgumentNullException("serializationStream"); } if (serializationStream.CanSeek && (serializationStream.Length == 0)) throw new SerializationException(SoapUtil.GetResourceString("Serialization_Stream")); InternalST.Soap( this, "Deserialize Entry"); InternalFE formatterEnums = new InternalFE(); formatterEnums.FEtypeFormat = m_typeFormat; formatterEnums.FEtopObject = m_topObject; formatterEnums.FEserializerTypeEnum = InternalSerializerTypeE.Soap; formatterEnums.FEassemblyFormat = m_assemblyFormat; formatterEnums.FEsecurityLevel = m_securityLevel; ObjectReader sor = new ObjectReader(serializationStream, m_surrogates, m_context, formatterEnums, m_binder); // If this is the first call, or a new stream is being used a new Soap parser is created. // If this is a continuing call, then the existing SoapParser is used. // One stream can contains multiple Soap XML documents. The XMLParser buffers the XML so // that the same XMLParser has to be used to continue a stream. if ((soapParser == null) || (serializationStream != currentStream)) { soapParser = new SoapParser(serializationStream); currentStream = serializationStream; } soapParser.Init(sor); Object obj = sor.Deserialize(handler, soapParser); InternalST.InfoSoap("Leave SoapFormatter.Deserialize "); return obj; } public void Serialize(Stream serializationStream, Object graph) { Serialize(serializationStream, graph, null); } // Commences the process of serializing the entire graph. All of the data (in the appropriate format) // is emitted onto the stream. public void Serialize(Stream serializationStream, Object graph, Header[] headers) { InternalST.InfoSoap("Enter SoapFormatter.Serialize "); if (serializationStream==null) { throw new ArgumentNullException("serializationStream"); } InternalST.Soap( this, "Serialize Entry"); InternalFE formatterEnums = new InternalFE(); formatterEnums.FEtypeFormat = m_typeFormat; formatterEnums.FEtopObject = m_topObject; formatterEnums.FEserializerTypeEnum = InternalSerializerTypeE.Soap; formatterEnums.FEassemblyFormat = m_assemblyFormat; ObjectWriter sow = new ObjectWriter(serializationStream, m_surrogates, m_context, formatterEnums); sow.Serialize(graph, headers, new SoapWriter(serializationStream)); InternalST.InfoSoap("Leave SoapFormatter.Serialize "); } public ISurrogateSelector SurrogateSelector { get { return m_surrogates; } set { m_surrogates = value; } } public SerializationBinder Binder { get { return m_binder; } set { m_binder=value; } } public StreamingContext Context { get { return m_context; } set { m_context = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //============================================================ // // Class: SoapFormatter //// Author: Peter de Jong ([....]) // // Purpose: Soap XML Formatter // // Date: June 10, 1999 // //=========================================================== #if FEATURE_COMINTEROP // These two attributes are for supporting side-by-side of COM-visible // objects with NDP 1.0 RTM. This needs to be set on all assemblies that // expose COM-visible types to be made Side by Side with NDP 1.0 RTM. // This declaration covers System.Runtime.Serialization.Formatters.Soap.dll [assembly:System.Runtime.InteropServices.ComCompatibleVersion(1,0,3300,0)] #endif // FEATURE_COMINTEROP namespace System.Runtime.Serialization.Formatters.Soap { using System; using System.Runtime.Serialization.Formatters; using System.IO; using System.Reflection; using System.Globalization; using System.Collections; using System.Runtime.Serialization; using System.Runtime.Remoting; using System.Runtime.Remoting.Messaging; using System.Text; sealed public class SoapFormatter : IRemotingFormatter { private SoapParser soapParser = null; private ISurrogateSelector m_surrogates; private StreamingContext m_context; private FormatterTypeStyle m_typeFormat = FormatterTypeStyle.TypesWhenNeeded; private ISoapMessage m_topObject = null; //private FormatterAssemblyStyle m_assemblyFormat = FormatterAssemblyStyle.Simple; private FormatterAssemblyStyle m_assemblyFormat = FormatterAssemblyStyle.Full; private TypeFilterLevel m_securityLevel = TypeFilterLevel.Full; private SerializationBinder m_binder; private Stream currentStream = null; // Property which specifies an object of type ISoapMessage into which // the SoapTop object is serialized. Should only be used if the Soap // top record is a methodCall or methodResponse element. public ISoapMessage TopObject { get {return m_topObject;} set {m_topObject = value;} } // Property which specifies how types are serialized, // FormatterTypeStyle Enum specifies options public FormatterTypeStyle TypeFormat { get {return m_typeFormat;} set { // Reset the value if TypesWhenNeeded // Or the value for TypesAlways and XsdString if (value == FormatterTypeStyle.TypesWhenNeeded) m_typeFormat = FormatterTypeStyle.TypesWhenNeeded; else m_typeFormat |= value; } } // Property which specifies how types are serialized, // FormatterAssemblyStyle Enum specifies options public FormatterAssemblyStyle AssemblyFormat { get {return m_assemblyFormat;} set {m_assemblyFormat = value;} } // Property which specifies the security level of formatter // TypeFilterLevel Enum specifies options public TypeFilterLevel FilterLevel { get {return m_securityLevel;} set {m_securityLevel = value;} } // Constructor public SoapFormatter() { m_surrogates=null; m_context = new StreamingContext(StreamingContextStates.All); } // Constructor public SoapFormatter(ISurrogateSelector selector, StreamingContext context) { m_surrogates = selector; m_context = context; } // Deserialize the stream into an object graph. public Object Deserialize(Stream serializationStream) { return Deserialize(serializationStream, null); } // Deserialize the stream into an object graph. public Object Deserialize(Stream serializationStream, HeaderHandler handler) { InternalST.InfoSoap("Enter SoapFormatter.Deserialize "); if (serializationStream==null) { throw new ArgumentNullException("serializationStream"); } if (serializationStream.CanSeek && (serializationStream.Length == 0)) throw new SerializationException(SoapUtil.GetResourceString("Serialization_Stream")); InternalST.Soap( this, "Deserialize Entry"); InternalFE formatterEnums = new InternalFE(); formatterEnums.FEtypeFormat = m_typeFormat; formatterEnums.FEtopObject = m_topObject; formatterEnums.FEserializerTypeEnum = InternalSerializerTypeE.Soap; formatterEnums.FEassemblyFormat = m_assemblyFormat; formatterEnums.FEsecurityLevel = m_securityLevel; ObjectReader sor = new ObjectReader(serializationStream, m_surrogates, m_context, formatterEnums, m_binder); // If this is the first call, or a new stream is being used a new Soap parser is created. // If this is a continuing call, then the existing SoapParser is used. // One stream can contains multiple Soap XML documents. The XMLParser buffers the XML so // that the same XMLParser has to be used to continue a stream. if ((soapParser == null) || (serializationStream != currentStream)) { soapParser = new SoapParser(serializationStream); currentStream = serializationStream; } soapParser.Init(sor); Object obj = sor.Deserialize(handler, soapParser); InternalST.InfoSoap("Leave SoapFormatter.Deserialize "); return obj; } public void Serialize(Stream serializationStream, Object graph) { Serialize(serializationStream, graph, null); } // Commences the process of serializing the entire graph. All of the data (in the appropriate format) // is emitted onto the stream. public void Serialize(Stream serializationStream, Object graph, Header[] headers) { InternalST.InfoSoap("Enter SoapFormatter.Serialize "); if (serializationStream==null) { throw new ArgumentNullException("serializationStream"); } InternalST.Soap( this, "Serialize Entry"); InternalFE formatterEnums = new InternalFE(); formatterEnums.FEtypeFormat = m_typeFormat; formatterEnums.FEtopObject = m_topObject; formatterEnums.FEserializerTypeEnum = InternalSerializerTypeE.Soap; formatterEnums.FEassemblyFormat = m_assemblyFormat; ObjectWriter sow = new ObjectWriter(serializationStream, m_surrogates, m_context, formatterEnums); sow.Serialize(graph, headers, new SoapWriter(serializationStream)); InternalST.InfoSoap("Leave SoapFormatter.Serialize "); } public ISurrogateSelector SurrogateSelector { get { return m_surrogates; } set { m_surrogates = value; } } public SerializationBinder Binder { get { return m_binder; } set { m_binder=value; } } public StreamingContext Context { get { return m_context; } set { m_context = value; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
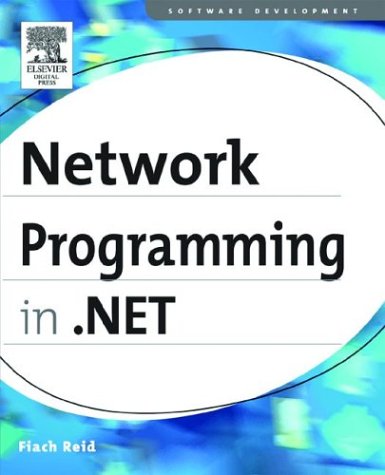
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AsymmetricCryptoHandle.cs
- ClientRuntimeConfig.cs
- FormViewInsertEventArgs.cs
- AttributeData.cs
- MexServiceChannelBuilder.cs
- MailHeaderInfo.cs
- LicenseException.cs
- HttpHandlersInstallComponent.cs
- GridProviderWrapper.cs
- FileClassifier.cs
- ClientTargetCollection.cs
- StylusCaptureWithinProperty.cs
- ListBoxAutomationPeer.cs
- Substitution.cs
- CodeValidator.cs
- InstanceDataCollection.cs
- TemplateBindingExtensionConverter.cs
- SecurityContext.cs
- Calendar.cs
- StylusCaptureWithinProperty.cs
- EmptyControlCollection.cs
- FilterEventArgs.cs
- OrderByQueryOptionExpression.cs
- ExpressionSelection.cs
- HwndHost.cs
- StrongNameIdentityPermission.cs
- DefaultAsyncDataDispatcher.cs
- IdentityNotMappedException.cs
- XmlElementAttributes.cs
- Hash.cs
- ConfigurationStrings.cs
- DoubleAnimationUsingKeyFrames.cs
- _ListenerResponseStream.cs
- EntitySqlQueryCacheKey.cs
- DrawingCollection.cs
- CollectionViewProxy.cs
- EmbeddedMailObject.cs
- NaturalLanguageHyphenator.cs
- CaseExpr.cs
- GlyphRunDrawing.cs
- HwndProxyElementProvider.cs
- SystemIPInterfaceProperties.cs
- TransactionInformation.cs
- NamespaceMapping.cs
- PlainXmlSerializer.cs
- DesignTimeXamlWriter.cs
- KnownTypes.cs
- HtmlValidatorAdapter.cs
- BuildResultCache.cs
- DebuggerService.cs
- Random.cs
- DataSourceCache.cs
- HtmlInputText.cs
- ClientRuntimeConfig.cs
- InnerItemCollectionView.cs
- RowSpanVector.cs
- XPathDocumentIterator.cs
- LinkUtilities.cs
- BaseWebProxyFinder.cs
- StrokeIntersection.cs
- SerializeAbsoluteContext.cs
- WriteTimeStream.cs
- XamlSerializationHelper.cs
- EpmSyndicationContentDeSerializer.cs
- DataControlFieldCell.cs
- IIS7WorkerRequest.cs
- ListViewDataItem.cs
- LocalizationComments.cs
- TextModifierScope.cs
- AuthenticationService.cs
- ClientTarget.cs
- XmlExtensionFunction.cs
- CombinedHttpChannel.cs
- ReceiveActivityDesignerTheme.cs
- DataGridViewSortCompareEventArgs.cs
- Control.cs
- ValidationErrorEventArgs.cs
- HtmlContainerControl.cs
- WebPartTracker.cs
- SqlAggregateChecker.cs
- QuaternionKeyFrameCollection.cs
- ConfigurationManagerInternal.cs
- XmlTextWriter.cs
- ContextMenuAutomationPeer.cs
- InkCanvasAutomationPeer.cs
- BuildManager.cs
- QuerySettings.cs
- SvcMapFile.cs
- XmlSchemaComplexType.cs
- Calendar.cs
- DataMisalignedException.cs
- ServiceElement.cs
- StylusButtonEventArgs.cs
- ZipPackagePart.cs
- StandardCommands.cs
- StyleCollection.cs
- HtmlTableRow.cs
- BasePattern.cs
- Bits.cs
- DbDataSourceEnumerator.cs