Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / DefaultTextStoreTextComposition.cs / 1305600 / DefaultTextStoreTextComposition.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DefaultTextStoreTextComposition class is the composition // object for the input in DefaultTextStore. // Cicero's composition injected to DefaulteTextStore is // represent by this DefaultTextStoreTextComposition. // This has custom Complete method to control // Cicero's composiiton. // // History: // 04/01/2004 : yutakas created // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Text; using System.Windows.Threading; using System.Windows; using System.Security; using MS.Win32; namespace System.Windows.Input { ////// DefaultTextStoreTextComposition class implements Complete for /// the composition in DefaultTextStore. /// internal class DefaultTextStoreTextComposition : TextComposition { //----------------------------------------------------- // // ctor // //----------------------------------------------------- ////// ctor /// ////// Critical - calls base ctor - which in turn stores the inputmanager that's critical. /// [SecurityCritical] internal DefaultTextStoreTextComposition(InputManager inputManager, IInputElement source, string text, TextCompositionAutoComplete autoComplete) : base(inputManager, source, text, autoComplete) { } //------------------------------------------------------ // // Public Interface Methods // //----------------------------------------------------- ////// Finalize the composition. /// This does not call base.Complete() because TextComposition.Complete() /// will call TextServicesManager.CompleteComposition() directly to generate TextCompositionEvent. /// We finalize Cicero's composition and DefaultTextStore will automatically /// generate the proper TextComposition events. /// ////// Critical: This completes the composition and in doing so calls GetTransitionaryContext which gives it ITfContext /// TreatAsSafe: The context is not exposed, neither are the other members /// [SecurityCritical,SecurityTreatAsSafe] public override void Complete() { // VerifyAccess(); UnsafeNativeMethods.ITfContext context = GetTransitoryContext(); UnsafeNativeMethods.ITfContextOwnerCompositionServices compositionService = context as UnsafeNativeMethods.ITfContextOwnerCompositionServices; UnsafeNativeMethods.ITfCompositionView composition = GetComposition(context); if (composition != null) { // Terminate composition if there is a composition view. compositionService.TerminateComposition(composition); Marshal.ReleaseComObject(composition); } Marshal.ReleaseComObject(context); } //------------------------------------------------------ // // private Methods // //------------------------------------------------------ ////// Get the base ITfContext of the transitory document. /// ////// Critical: This exposes ITfContext which has unsecure methods /// [SecurityCritical] private UnsafeNativeMethods.ITfContext GetTransitoryContext() { DefaultTextStore defaultTextStore = DefaultTextStore.Current; UnsafeNativeMethods.ITfDocumentMgr doc = defaultTextStore.TransitoryDocumentManager; UnsafeNativeMethods.ITfContext context; doc.GetBase(out context); Marshal.ReleaseComObject(doc); return context; } ////// Get ITfContextView of the context. /// ////// Critical: calls Marshal.ReleaseComObject which has a LinkDemand /// TreatAsSafe: can't pass in arbitrary COM object to release /// [SecurityCritical, SecurityTreatAsSafe] private UnsafeNativeMethods.ITfCompositionView GetComposition(UnsafeNativeMethods.ITfContext context) { UnsafeNativeMethods.ITfContextComposition contextComposition; UnsafeNativeMethods.IEnumITfCompositionView enumCompositionView; UnsafeNativeMethods.ITfCompositionView[] compositionViews = new UnsafeNativeMethods.ITfCompositionView[1]; int fetched; contextComposition = (UnsafeNativeMethods.ITfContextComposition)context; contextComposition.EnumCompositions(out enumCompositionView); enumCompositionView.Next(1, compositionViews, out fetched); Marshal.ReleaseComObject(enumCompositionView); return compositionViews[0]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DefaultTextStoreTextComposition class is the composition // object for the input in DefaultTextStore. // Cicero's composition injected to DefaulteTextStore is // represent by this DefaultTextStoreTextComposition. // This has custom Complete method to control // Cicero's composiiton. // // History: // 04/01/2004 : yutakas created // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Text; using System.Windows.Threading; using System.Windows; using System.Security; using MS.Win32; namespace System.Windows.Input { ////// DefaultTextStoreTextComposition class implements Complete for /// the composition in DefaultTextStore. /// internal class DefaultTextStoreTextComposition : TextComposition { //----------------------------------------------------- // // ctor // //----------------------------------------------------- ////// ctor /// ////// Critical - calls base ctor - which in turn stores the inputmanager that's critical. /// [SecurityCritical] internal DefaultTextStoreTextComposition(InputManager inputManager, IInputElement source, string text, TextCompositionAutoComplete autoComplete) : base(inputManager, source, text, autoComplete) { } //------------------------------------------------------ // // Public Interface Methods // //----------------------------------------------------- ////// Finalize the composition. /// This does not call base.Complete() because TextComposition.Complete() /// will call TextServicesManager.CompleteComposition() directly to generate TextCompositionEvent. /// We finalize Cicero's composition and DefaultTextStore will automatically /// generate the proper TextComposition events. /// ////// Critical: This completes the composition and in doing so calls GetTransitionaryContext which gives it ITfContext /// TreatAsSafe: The context is not exposed, neither are the other members /// [SecurityCritical,SecurityTreatAsSafe] public override void Complete() { // VerifyAccess(); UnsafeNativeMethods.ITfContext context = GetTransitoryContext(); UnsafeNativeMethods.ITfContextOwnerCompositionServices compositionService = context as UnsafeNativeMethods.ITfContextOwnerCompositionServices; UnsafeNativeMethods.ITfCompositionView composition = GetComposition(context); if (composition != null) { // Terminate composition if there is a composition view. compositionService.TerminateComposition(composition); Marshal.ReleaseComObject(composition); } Marshal.ReleaseComObject(context); } //------------------------------------------------------ // // private Methods // //------------------------------------------------------ ////// Get the base ITfContext of the transitory document. /// ////// Critical: This exposes ITfContext which has unsecure methods /// [SecurityCritical] private UnsafeNativeMethods.ITfContext GetTransitoryContext() { DefaultTextStore defaultTextStore = DefaultTextStore.Current; UnsafeNativeMethods.ITfDocumentMgr doc = defaultTextStore.TransitoryDocumentManager; UnsafeNativeMethods.ITfContext context; doc.GetBase(out context); Marshal.ReleaseComObject(doc); return context; } ////// Get ITfContextView of the context. /// ////// Critical: calls Marshal.ReleaseComObject which has a LinkDemand /// TreatAsSafe: can't pass in arbitrary COM object to release /// [SecurityCritical, SecurityTreatAsSafe] private UnsafeNativeMethods.ITfCompositionView GetComposition(UnsafeNativeMethods.ITfContext context) { UnsafeNativeMethods.ITfContextComposition contextComposition; UnsafeNativeMethods.IEnumITfCompositionView enumCompositionView; UnsafeNativeMethods.ITfCompositionView[] compositionViews = new UnsafeNativeMethods.ITfCompositionView[1]; int fetched; contextComposition = (UnsafeNativeMethods.ITfContextComposition)context; contextComposition.EnumCompositions(out enumCompositionView); enumCompositionView.Next(1, compositionViews, out fetched); Marshal.ReleaseComObject(enumCompositionView); return compositionViews[0]; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
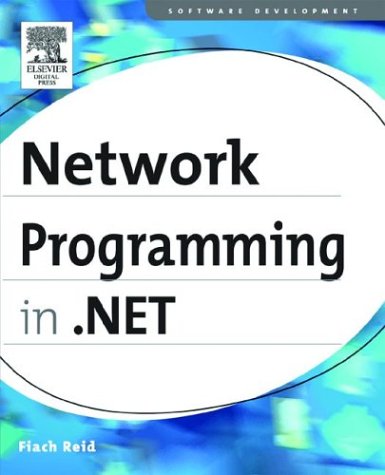
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlVersion.cs
- StrongNamePublicKeyBlob.cs
- ProcessThreadCollection.cs
- ComboBoxRenderer.cs
- _ScatterGatherBuffers.cs
- BitmapScalingModeValidation.cs
- TlsnegoTokenProvider.cs
- EmptyControlCollection.cs
- GC.cs
- DuplexSecurityProtocolFactory.cs
- IdleTimeoutMonitor.cs
- HttpClientCertificate.cs
- DecimalAnimation.cs
- WebPartDisplayModeCollection.cs
- IdentifierService.cs
- ReadOnlyCollectionBase.cs
- RuntimeConfigurationRecord.cs
- SendMailErrorEventArgs.cs
- HtmlElementErrorEventArgs.cs
- CodeTypeDelegate.cs
- ManipulationBoundaryFeedbackEventArgs.cs
- StringAnimationUsingKeyFrames.cs
- SrgsDocument.cs
- DocumentPageTextView.cs
- PackageDigitalSignatureManager.cs
- XmlCharCheckingWriter.cs
- DecoderNLS.cs
- GeneralTransform3D.cs
- ApplicationSettingsBase.cs
- ComplexTypeEmitter.cs
- ExpandableObjectConverter.cs
- PageFunction.cs
- DataGridPageChangedEventArgs.cs
- XhtmlConformanceSection.cs
- arc.cs
- TrackPoint.cs
- ProxySimple.cs
- StyleBamlTreeBuilder.cs
- WorkflowApplicationUnloadedException.cs
- XmlAttributeCache.cs
- BackStopAuthenticationModule.cs
- ImageClickEventArgs.cs
- _IPv6Address.cs
- ThumbAutomationPeer.cs
- Queue.cs
- Timer.cs
- PointCollection.cs
- RelAssertionDirectKeyIdentifierClause.cs
- CommandID.cs
- PreDigestedSignedInfo.cs
- ConfigXmlSignificantWhitespace.cs
- LinqExpressionNormalizer.cs
- ImageIndexConverter.cs
- WorkflowOperationBehavior.cs
- DataGridViewColumnCollection.cs
- TreeBuilder.cs
- dataprotectionpermission.cs
- DataSourceControl.cs
- QueryHandler.cs
- WpfWebRequestHelper.cs
- XPathPatternParser.cs
- DocumentViewerHelper.cs
- ExtensibleClassFactory.cs
- OracleDateTime.cs
- LicenseManager.cs
- FormViewInsertedEventArgs.cs
- InputBinding.cs
- WindowsFormsHelpers.cs
- Bold.cs
- AsyncWaitHandle.cs
- UniqueIdentifierService.cs
- CodeDomDesignerLoader.cs
- TableLayoutColumnStyleCollection.cs
- SqlWebEventProvider.cs
- RuleProcessor.cs
- BaseAddressElement.cs
- AppDomain.cs
- XmlSchemaComplexContent.cs
- StrokeFIndices.cs
- FileSecurity.cs
- NameNode.cs
- DataGridColumnEventArgs.cs
- SqlReferenceCollection.cs
- PerformanceCounterPermissionAttribute.cs
- LineProperties.cs
- XmlNamedNodeMap.cs
- GeneratedCodeAttribute.cs
- BaseParser.cs
- XPathMessageContext.cs
- CodeFieldReferenceExpression.cs
- FullTextBreakpoint.cs
- MetabaseSettings.cs
- BuildResult.cs
- MultipleViewPattern.cs
- Debug.cs
- XamlVector3DCollectionSerializer.cs
- InternalRelationshipCollection.cs
- PageParserFilter.cs
- DateRangeEvent.cs
- StaticDataManager.cs