Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ImageIndexConverter.cs / 1305376 / ImageIndexConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.Drawing; using System.Diagnostics; using System.Globalization; using System.Reflection; ////// /// ImageIndexConverter is a class that can be used to convert /// image index values one data type to another. /// public class ImageIndexConverter : Int32Converter { private string parentImageListProperty = "Parent"; ///protected virtual bool IncludeNoneAsStandardValue { get { return true; } } /// /// this is the property to look at when there is no ImageList property /// on the current object. For example, in ToolBarButton - the ImageList is /// on the ToolBarButton.Parent property. In WinBarItem, the ImageList is on /// the WinBarItem.Owner property. /// internal string ParentImageListProperty { get { return parentImageListProperty; } set { parentImageListProperty = value; } } ////// /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { String stringValue = value as String; if (stringValue != null && String.Compare(stringValue, SR.GetString(SR.toStringNone), true, culture) == 0) { return -1; } return base.ConvertFrom(context, culture, value); } ////// Converts the given value object to a 32-bit signed integer object. /// ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string) && value is int && ((int)value) == -1) { return SR.GetString(SR.toStringNone); } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Retrieves a collection containing a set of standard values /// for the data type this validator is designed for. This /// will return null if the data type does not support a /// standard set of values. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (context != null && context.Instance != null) { object instance = context.Instance; PropertyDescriptor imageListProp = ImageListUtils.GetImageListProperty(context.PropertyDescriptor, ref instance); while (instance != null && imageListProp == null) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(instance); foreach (PropertyDescriptor prop in props) { if (typeof(ImageList).IsAssignableFrom(prop.PropertyType)) { imageListProp = prop; break; } } if (imageListProp == null) { // We didn't find the image list in this component. See if the // component has a "parent" property. If so, walk the tree... // PropertyDescriptor parentProp = props[ParentImageListProperty]; if (parentProp != null) { instance = parentProp.GetValue(instance); } else { // Stick a fork in us, we're done. // instance = null; } } } if (imageListProp != null) { ImageList imageList = (ImageList)imageListProp.GetValue(instance); if (imageList != null) { // Create array to contain standard values // object[] values; int nImages = imageList.Images.Count; if (IncludeNoneAsStandardValue) { values = new object[nImages + 1]; values[nImages] = -1; } else { values = new object[nImages]; } // Fill in the array // for (int i = 0; i < nImages; i++) { values[i] = i; } return new StandardValuesCollection(values); } } } if (IncludeNoneAsStandardValue) { return new StandardValuesCollection(new object[] {-1}); } else { return new StandardValuesCollection(new object[0]); } } ////// /// Determines if the list of standard values returned from /// GetStandardValues is an exclusive list. If the list /// is exclusive, then no other values are valid, such as /// in an enum data type. If the list is not exclusive, /// then there are other valid values besides the list of /// standard values GetStandardValues provides. /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// /// Determines if this object supports a standard set of values /// that can be picked from a list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.Drawing; using System.Diagnostics; using System.Globalization; using System.Reflection; ////// /// ImageIndexConverter is a class that can be used to convert /// image index values one data type to another. /// public class ImageIndexConverter : Int32Converter { private string parentImageListProperty = "Parent"; ///protected virtual bool IncludeNoneAsStandardValue { get { return true; } } /// /// this is the property to look at when there is no ImageList property /// on the current object. For example, in ToolBarButton - the ImageList is /// on the ToolBarButton.Parent property. In WinBarItem, the ImageList is on /// the WinBarItem.Owner property. /// internal string ParentImageListProperty { get { return parentImageListProperty; } set { parentImageListProperty = value; } } ////// /// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { String stringValue = value as String; if (stringValue != null && String.Compare(stringValue, SR.GetString(SR.toStringNone), true, culture) == 0) { return -1; } return base.ConvertFrom(context, culture, value); } ////// Converts the given value object to a 32-bit signed integer object. /// ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(string) && value is int && ((int)value) == -1) { return SR.GetString(SR.toStringNone); } return base.ConvertTo(context, culture, value, destinationType); } ////// /// Retrieves a collection containing a set of standard values /// for the data type this validator is designed for. This /// will return null if the data type does not support a /// standard set of values. /// public override StandardValuesCollection GetStandardValues(ITypeDescriptorContext context) { if (context != null && context.Instance != null) { object instance = context.Instance; PropertyDescriptor imageListProp = ImageListUtils.GetImageListProperty(context.PropertyDescriptor, ref instance); while (instance != null && imageListProp == null) { PropertyDescriptorCollection props = TypeDescriptor.GetProperties(instance); foreach (PropertyDescriptor prop in props) { if (typeof(ImageList).IsAssignableFrom(prop.PropertyType)) { imageListProp = prop; break; } } if (imageListProp == null) { // We didn't find the image list in this component. See if the // component has a "parent" property. If so, walk the tree... // PropertyDescriptor parentProp = props[ParentImageListProperty]; if (parentProp != null) { instance = parentProp.GetValue(instance); } else { // Stick a fork in us, we're done. // instance = null; } } } if (imageListProp != null) { ImageList imageList = (ImageList)imageListProp.GetValue(instance); if (imageList != null) { // Create array to contain standard values // object[] values; int nImages = imageList.Images.Count; if (IncludeNoneAsStandardValue) { values = new object[nImages + 1]; values[nImages] = -1; } else { values = new object[nImages]; } // Fill in the array // for (int i = 0; i < nImages; i++) { values[i] = i; } return new StandardValuesCollection(values); } } } if (IncludeNoneAsStandardValue) { return new StandardValuesCollection(new object[] {-1}); } else { return new StandardValuesCollection(new object[0]); } } ////// /// Determines if the list of standard values returned from /// GetStandardValues is an exclusive list. If the list /// is exclusive, then no other values are valid, such as /// in an enum data type. If the list is not exclusive, /// then there are other valid values besides the list of /// standard values GetStandardValues provides. /// public override bool GetStandardValuesExclusive(ITypeDescriptorContext context) { return false; } ////// /// Determines if this object supports a standard set of values /// that can be picked from a list. /// public override bool GetStandardValuesSupported(ITypeDescriptorContext context) { return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
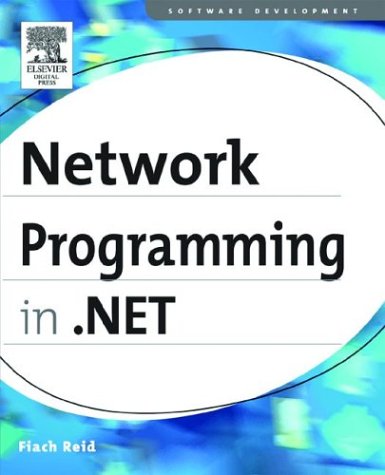
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StructuredTypeInfo.cs
- HwndSubclass.cs
- TemplatedControlDesigner.cs
- EntityProviderFactory.cs
- SmiConnection.cs
- RowCache.cs
- QueryCreatedEventArgs.cs
- Intellisense.cs
- ExecutionContext.cs
- ConnectionManagementSection.cs
- BigInt.cs
- HtmlElementEventArgs.cs
- OleDbStruct.cs
- GacUtil.cs
- FormViewInsertEventArgs.cs
- Soap12ProtocolImporter.cs
- MasterPage.cs
- StoragePropertyMapping.cs
- DateTimeOffsetConverter.cs
- BamlCollectionHolder.cs
- GatewayIPAddressInformationCollection.cs
- TimeSpan.cs
- XmlUtil.cs
- TableNameAttribute.cs
- MembershipAdapter.cs
- DbDeleteCommandTree.cs
- FormViewUpdateEventArgs.cs
- ActivationServices.cs
- basenumberconverter.cs
- Exceptions.cs
- SslStream.cs
- XmlSchemaAttributeGroup.cs
- EncodingTable.cs
- PinnedBufferMemoryStream.cs
- UrlParameterWriter.cs
- LookupBindingPropertiesAttribute.cs
- SettingsProviderCollection.cs
- SortedList.cs
- PngBitmapDecoder.cs
- _Connection.cs
- QueryCacheManager.cs
- SystemIPAddressInformation.cs
- OracleInfoMessageEventArgs.cs
- MasterPageBuildProvider.cs
- CoTaskMemHandle.cs
- ClaimComparer.cs
- KeyConverter.cs
- CorrelationResolver.cs
- FullTrustAssembly.cs
- ImmutableAssemblyCacheEntry.cs
- WrappedIUnknown.cs
- ExcludePathInfo.cs
- ScrollEventArgs.cs
- EdmType.cs
- RotateTransform3D.cs
- DetailsViewPageEventArgs.cs
- LocatorGroup.cs
- NamedObject.cs
- TimeManager.cs
- CacheAxisQuery.cs
- DataGridViewCellFormattingEventArgs.cs
- DataListCommandEventArgs.cs
- WindowPattern.cs
- Axis.cs
- ToolStripContainer.cs
- IssuedTokensHeader.cs
- EnumMember.cs
- __TransparentProxy.cs
- BaseTemplateCodeDomTreeGenerator.cs
- RepeaterCommandEventArgs.cs
- PersonalizationProviderHelper.cs
- StructuralType.cs
- AnnotationAuthorChangedEventArgs.cs
- MasterPageCodeDomTreeGenerator.cs
- AuthenticationManager.cs
- UrlPath.cs
- ActionFrame.cs
- PreviewPrintController.cs
- PreProcessor.cs
- AlternateView.cs
- Parameter.cs
- RegularExpressionValidator.cs
- PathParser.cs
- BamlVersionHeader.cs
- TraceSwitch.cs
- GridViewCommandEventArgs.cs
- InheritanceUI.cs
- LoadRetryHandler.cs
- Pens.cs
- CheckPair.cs
- EntityCodeGenerator.cs
- LinearQuaternionKeyFrame.cs
- x509utils.cs
- Attributes.cs
- PingReply.cs
- QueryOperationResponseOfT.cs
- ConfigXmlWhitespace.cs
- InvokeMethodActivityDesigner.cs
- FlowNode.cs
- RequestContextBase.cs