Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Base / System / Windows / Input / KeyConverter.cs / 3 / KeyConverter.cs
//---------------------------------------------------------------------------- // // File: KeyConverter.cs // // Description: // // KeyConverter : Converts a key string to the *Type* that the string represents and vice-versa // // Features: // // History: // 05/28/2003 created: Chandrasekhar Rentachintala // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; // for TypeConverter using System.Globalization; // for CultureInfo using System.Reflection; using System.Windows; using System.Windows.Input; using System.Windows.Markup; using System.Security.Permissions; using MS.Utility; namespace System.Windows.Input { ////// Key Converter class for converting between a string and the Type of a Key /// ///public class KeyConverter : TypeConverter { /// /// CanConvertFrom() /// /// /// ////// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // We can convert to a string. // We can convert to an InstanceDescriptor or to a string. if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for known type if (context != null && context.Instance != null) { Key key = (Key)context.Instance; return ((int)key >= (int)Key.None && (int)key <= (int)Key.DeadCharProcessed); } } return false; } ////// ConvertFrom() /// /// /// /// ////// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { if (source is string) { string fullName = ((string)source).Trim(); object key = GetKey(fullName, CultureInfo.InvariantCulture); if (key != null) { return ((Key)key); } else { throw new NotSupportedException(SR.Get(SRID.Unsupported_Key, fullName)); } } throw GetConvertFromException(source); } /// /// ConvertTo() /// /// /// /// /// ////// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) throw new ArgumentNullException("destinationType"); if (destinationType == typeof(string) && value != null) { Key key = (Key)value; if (key == Key.None) { return String.Empty; } if (key >= Key.D0 && key <= Key.D9) { return Char.ToString((char)(int)(key - Key.D0 + '0')); } if (key >= Key.A && key <= Key.Z) { return Char.ToString((char)(int)(key - Key.A + 'A')); } String strKey = MatchKey(key, culture); if (strKey != null && (strKey.Length != 0 || strKey == String.Empty)) { return strKey; } } throw GetConvertToException(value, destinationType); } private object GetKey(string keyToken, CultureInfo culture) { if (keyToken == String.Empty) { return Key.None; } else { keyToken = keyToken.ToUpper(culture); if (keyToken.Length == 1 && Char.IsLetterOrDigit(keyToken[0])) { if (Char.IsDigit(keyToken[0]) && (keyToken[0] >= '0' && keyToken[0] <= '9')) { return ((int)(Key)(Key.D0 + keyToken[0] - '0')); } else if (Char.IsLetter(keyToken[0]) && (keyToken[0] >= 'A' && keyToken[0] <= 'Z')) { return ((int)(Key)(Key.A + keyToken[0] - 'A')); } else { throw new ArgumentException(SR.Get(SRID.CannotConvertStringToType, keyToken, typeof(Key))); } } else { Key keyFound = (Key)(-1); switch (keyToken) { case "ENTER": keyFound = Key.Return; break; case "ESC": keyFound = Key.Escape; break; case "PGUP": keyFound = Key.PageUp; break; case "PGDN": keyFound = Key.PageDown; break; case "PRTSC": keyFound = Key.PrintScreen; break; case "INS": keyFound = Key.Insert; break; case "DEL": keyFound = Key.Delete; break; case "WINDOWS": keyFound = Key.LWin; break; case "WIN": keyFound = Key.LWin; break; case "LEFTWINDOWS": keyFound = Key.LWin; break; case "RIGHTWINDOWS": keyFound = Key.RWin; break; case "APPS": keyFound = Key.Apps; break; case "APPLICATION": keyFound = Key.Apps; break; case "BREAK": keyFound = Key.Cancel; break; case "BACKSPACE": keyFound = Key.Back; break; case "BKSP": keyFound = Key.Back; break; case "BS": keyFound = Key.Back; break; case "SHIFT": keyFound = Key.LeftShift; break; case "LEFTSHIFT": keyFound = Key.LeftShift; break; case "RIGHTSHIFT": keyFound = Key.RightShift; break; case "CONTROL": keyFound = Key.LeftCtrl; break; case "CTRL": keyFound = Key.LeftCtrl; break; case "LEFTCTRL": keyFound = Key.LeftCtrl; break; case "RIGHTCTRL": keyFound = Key.RightCtrl; break; case "ALT": keyFound = Key.LeftAlt; break; case "LEFTALT": keyFound = Key.LeftAlt; break; case "RIGHTALT": keyFound = Key.RightAlt; break; case "SEMICOLON": keyFound = Key.OemSemicolon; break; case "PLUS": keyFound = Key.OemPlus; break; case "COMMA": keyFound = Key.OemComma; break; case "MINUS": keyFound = Key.OemMinus; break; case "PERIOD": keyFound = Key.OemPeriod; break; case "QUESTION": keyFound = Key.OemQuestion; break; case "TILDE": keyFound = Key.OemTilde; break; case "OPENBRACKETS": keyFound = Key.OemOpenBrackets; break; case "PIPE": keyFound = Key.OemPipe; break; case "CLOSEBRACKETS": keyFound = Key.OemCloseBrackets; break; case "QUOTES": keyFound = Key.OemQuotes; break; case "BACKSLASH": keyFound = Key.OemBackslash; break; case "FINISH": keyFound = Key.OemFinish; break; case "ATTN": keyFound = Key.Attn; break; case "CRSEL": keyFound = Key.CrSel; break; case "EXSEL": keyFound = Key.ExSel; break; case "ERASEEOF": keyFound = Key.EraseEof; break; case "PLAY": keyFound = Key.Play; break; case "ZOOM": keyFound = Key.Zoom; break; case "PA1": keyFound = Key.Pa1; break; default: keyFound = (Key)Enum.Parse(typeof(Key), keyToken, true); break; } if ((int)keyFound != -1) { return keyFound; } return null; } } } private static string MatchKey(Key key, CultureInfo culture) { if (key == Key.None) return String.Empty; else { switch (key) { case Key.Back: return "Backspace"; case Key.LineFeed: return "Clear"; case Key.Escape: return "Esc"; } } if ((int)key >= (int)Key.None && (int)key <= (int)Key.DeadCharProcessed) return key.ToString(); else return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: KeyConverter.cs // // Description: // // KeyConverter : Converts a key string to the *Type* that the string represents and vice-versa // // Features: // // History: // 05/28/2003 created: Chandrasekhar Rentachintala // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; // for TypeConverter using System.Globalization; // for CultureInfo using System.Reflection; using System.Windows; using System.Windows.Input; using System.Windows.Markup; using System.Security.Permissions; using MS.Utility; namespace System.Windows.Input { /// /// Key Converter class for converting between a string and the Type of a Key /// ///public class KeyConverter : TypeConverter { /// /// CanConvertFrom() /// /// /// ////// public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // We can convert to a string. // We can convert to an InstanceDescriptor or to a string. if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for known type if (context != null && context.Instance != null) { Key key = (Key)context.Instance; return ((int)key >= (int)Key.None && (int)key <= (int)Key.DeadCharProcessed); } } return false; } ////// ConvertFrom() /// /// /// /// ////// public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { if (source is string) { string fullName = ((string)source).Trim(); object key = GetKey(fullName, CultureInfo.InvariantCulture); if (key != null) { return ((Key)key); } else { throw new NotSupportedException(SR.Get(SRID.Unsupported_Key, fullName)); } } throw GetConvertFromException(source); } /// /// ConvertTo() /// /// /// /// /// ////// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) throw new ArgumentNullException("destinationType"); if (destinationType == typeof(string) && value != null) { Key key = (Key)value; if (key == Key.None) { return String.Empty; } if (key >= Key.D0 && key <= Key.D9) { return Char.ToString((char)(int)(key - Key.D0 + '0')); } if (key >= Key.A && key <= Key.Z) { return Char.ToString((char)(int)(key - Key.A + 'A')); } String strKey = MatchKey(key, culture); if (strKey != null && (strKey.Length != 0 || strKey == String.Empty)) { return strKey; } } throw GetConvertToException(value, destinationType); } private object GetKey(string keyToken, CultureInfo culture) { if (keyToken == String.Empty) { return Key.None; } else { keyToken = keyToken.ToUpper(culture); if (keyToken.Length == 1 && Char.IsLetterOrDigit(keyToken[0])) { if (Char.IsDigit(keyToken[0]) && (keyToken[0] >= '0' && keyToken[0] <= '9')) { return ((int)(Key)(Key.D0 + keyToken[0] - '0')); } else if (Char.IsLetter(keyToken[0]) && (keyToken[0] >= 'A' && keyToken[0] <= 'Z')) { return ((int)(Key)(Key.A + keyToken[0] - 'A')); } else { throw new ArgumentException(SR.Get(SRID.CannotConvertStringToType, keyToken, typeof(Key))); } } else { Key keyFound = (Key)(-1); switch (keyToken) { case "ENTER": keyFound = Key.Return; break; case "ESC": keyFound = Key.Escape; break; case "PGUP": keyFound = Key.PageUp; break; case "PGDN": keyFound = Key.PageDown; break; case "PRTSC": keyFound = Key.PrintScreen; break; case "INS": keyFound = Key.Insert; break; case "DEL": keyFound = Key.Delete; break; case "WINDOWS": keyFound = Key.LWin; break; case "WIN": keyFound = Key.LWin; break; case "LEFTWINDOWS": keyFound = Key.LWin; break; case "RIGHTWINDOWS": keyFound = Key.RWin; break; case "APPS": keyFound = Key.Apps; break; case "APPLICATION": keyFound = Key.Apps; break; case "BREAK": keyFound = Key.Cancel; break; case "BACKSPACE": keyFound = Key.Back; break; case "BKSP": keyFound = Key.Back; break; case "BS": keyFound = Key.Back; break; case "SHIFT": keyFound = Key.LeftShift; break; case "LEFTSHIFT": keyFound = Key.LeftShift; break; case "RIGHTSHIFT": keyFound = Key.RightShift; break; case "CONTROL": keyFound = Key.LeftCtrl; break; case "CTRL": keyFound = Key.LeftCtrl; break; case "LEFTCTRL": keyFound = Key.LeftCtrl; break; case "RIGHTCTRL": keyFound = Key.RightCtrl; break; case "ALT": keyFound = Key.LeftAlt; break; case "LEFTALT": keyFound = Key.LeftAlt; break; case "RIGHTALT": keyFound = Key.RightAlt; break; case "SEMICOLON": keyFound = Key.OemSemicolon; break; case "PLUS": keyFound = Key.OemPlus; break; case "COMMA": keyFound = Key.OemComma; break; case "MINUS": keyFound = Key.OemMinus; break; case "PERIOD": keyFound = Key.OemPeriod; break; case "QUESTION": keyFound = Key.OemQuestion; break; case "TILDE": keyFound = Key.OemTilde; break; case "OPENBRACKETS": keyFound = Key.OemOpenBrackets; break; case "PIPE": keyFound = Key.OemPipe; break; case "CLOSEBRACKETS": keyFound = Key.OemCloseBrackets; break; case "QUOTES": keyFound = Key.OemQuotes; break; case "BACKSLASH": keyFound = Key.OemBackslash; break; case "FINISH": keyFound = Key.OemFinish; break; case "ATTN": keyFound = Key.Attn; break; case "CRSEL": keyFound = Key.CrSel; break; case "EXSEL": keyFound = Key.ExSel; break; case "ERASEEOF": keyFound = Key.EraseEof; break; case "PLAY": keyFound = Key.Play; break; case "ZOOM": keyFound = Key.Zoom; break; case "PA1": keyFound = Key.Pa1; break; default: keyFound = (Key)Enum.Parse(typeof(Key), keyToken, true); break; } if ((int)keyFound != -1) { return keyFound; } return null; } } } private static string MatchKey(Key key, CultureInfo culture) { if (key == Key.None) return String.Empty; else { switch (key) { case Key.Back: return "Backspace"; case Key.LineFeed: return "Clear"; case Key.Escape: return "Esc"; } } if ((int)key >= (int)Key.None && (int)key <= (int)Key.DeadCharProcessed) return key.ToString(); else return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
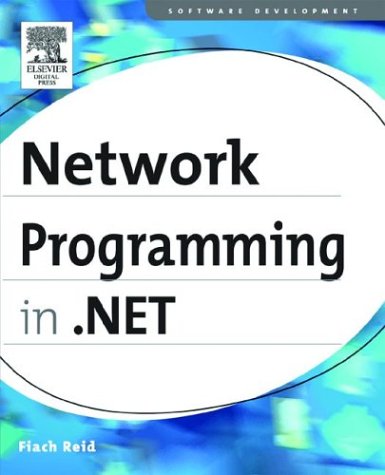
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TreeBuilder.cs
- WindowsTitleBar.cs
- LeafCellTreeNode.cs
- ListDictionary.cs
- RangeBase.cs
- JoinGraph.cs
- CodePageUtils.cs
- UIAgentAsyncEndRequest.cs
- Transform3DCollection.cs
- SubstitutionList.cs
- Tablet.cs
- CodeMemberField.cs
- TargetConverter.cs
- HttpGetProtocolReflector.cs
- OdbcCommandBuilder.cs
- SchemaTableOptionalColumn.cs
- CustomWebEventKey.cs
- ContainerParagraph.cs
- UrlParameterReader.cs
- DetailsViewDeletedEventArgs.cs
- AutomationPropertyInfo.cs
- DictionaryGlobals.cs
- ObjectDataSourceStatusEventArgs.cs
- CommandPlan.cs
- RectAnimationBase.cs
- MailMessageEventArgs.cs
- ZipIOLocalFileDataDescriptor.cs
- TableLayoutStyle.cs
- CorePropertiesFilter.cs
- SemanticBasicElement.cs
- UpdateRecord.cs
- DefaultAssemblyResolver.cs
- HeaderUtility.cs
- Frame.cs
- ApplicationException.cs
- ThreadStateException.cs
- ConfigurationSchemaErrors.cs
- WpfKnownTypeInvoker.cs
- Tool.cs
- Interlocked.cs
- SqlProcedureAttribute.cs
- MethodResolver.cs
- BufferBuilder.cs
- DecoderExceptionFallback.cs
- IntPtr.cs
- IERequestCache.cs
- CompositeKey.cs
- DataSourceView.cs
- ChooseAction.cs
- RadioButtonAutomationPeer.cs
- CheckBox.cs
- CodeIdentifier.cs
- TextParagraphView.cs
- CultureMapper.cs
- HitTestWithGeometryDrawingContextWalker.cs
- ContainerFilterService.cs
- ByteRangeDownloader.cs
- InvalidOleVariantTypeException.cs
- SocketInformation.cs
- WindowsImpersonationContext.cs
- DBNull.cs
- PrtCap_Reader.cs
- DeclarativeCatalogPart.cs
- DataConnectionHelper.cs
- ResXResourceSet.cs
- SqlClientWrapperSmiStreamChars.cs
- StructuralType.cs
- CompositionCommandSet.cs
- UnsafeNativeMethods.cs
- Logging.cs
- SystemWebExtensionsSectionGroup.cs
- CheckBox.cs
- FixUpCollection.cs
- PolyLineSegmentFigureLogic.cs
- TextServicesCompartment.cs
- XmlQueryCardinality.cs
- StructuralObject.cs
- SignatureDescription.cs
- DynamicRouteExpression.cs
- Image.cs
- DescendantQuery.cs
- TextServicesCompartmentEventSink.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- ConnectionPoint.cs
- DeclaredTypeValidatorAttribute.cs
- As.cs
- SqlConnection.cs
- NumericUpDownAcceleration.cs
- TextElementCollectionHelper.cs
- NamespaceQuery.cs
- AxParameterData.cs
- StringResourceManager.cs
- CngProvider.cs
- MatrixCamera.cs
- DateTimeOffsetStorage.cs
- EditorBrowsableAttribute.cs
- LayoutTable.cs
- ToolStripRenderEventArgs.cs
- ScrollEvent.cs
- NetworkAddressChange.cs