Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Expressions / As.cs / 1305376 / As.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Expressions { using System.Activities; using System.Activities.Statements; using System.Activities.Validation; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Linq.Expressions; using System.Runtime; [SuppressMessage(FxCop.Category.Naming, FxCop.Rule.IdentifiersShouldNotMatchKeywords, Justification = "Optimizing for XAML naming. VB imperative users will [] qualify (e.g. New [As])")] public sealed class As: CodeActivity { //Lock is not needed for operationFunction here. The reason is that delegates for a given As are the same. //It's possible that 2 threads are assigning the operationFucntion at the same time. But it's okay because the compiled codes are the same. static Func operationFunction; [RequiredArgument] [DefaultValue(null)] public InArgument Operand { get; set; } protected override void CacheMetadata(CodeActivityMetadata metadata) { UnaryExpressionHelper.OnGetArguments(metadata, this.Operand); if (operationFunction == null) { ValidationError validationError; if (!UnaryExpressionHelper.TryGenerateLinqDelegate(ExpressionType.TypeAs, out operationFunction, out validationError)) { metadata.AddValidationError(validationError); } } } protected override TResult Execute(CodeActivityContext context) { Fx.Assert(operationFunction != null, "OperationFunction must exist."); TOperand operandValue = this.Operand.Get(context); return operationFunction(operandValue); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
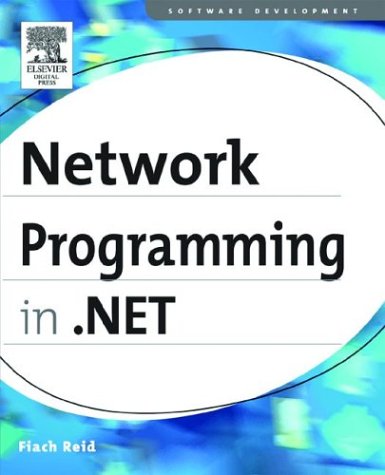
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlConnectionPoolProviderInfo.cs
- SettingsPropertyValue.cs
- DrawingAttributesDefaultValueFactory.cs
- WindowsAuthenticationModule.cs
- MessageRpc.cs
- Variable.cs
- HttpGetClientProtocol.cs
- RichTextBoxContextMenu.cs
- DecoratedNameAttribute.cs
- ReferenceEqualityComparer.cs
- MgmtConfigurationRecord.cs
- StorageEndPropertyMapping.cs
- DataServiceRequestException.cs
- StateMachineWorkflowDesigner.cs
- HwndStylusInputProvider.cs
- AssertSection.cs
- PackageDigitalSignatureManager.cs
- FtpCachePolicyElement.cs
- DesignTimeParseData.cs
- BulletedListEventArgs.cs
- listitem.cs
- ArraySet.cs
- validationstate.cs
- AliasedSlot.cs
- PageAdapter.cs
- TokenBasedSet.cs
- EnvironmentPermission.cs
- NotFiniteNumberException.cs
- LowerCaseStringConverter.cs
- FixedSOMContainer.cs
- EventRouteFactory.cs
- StateDesigner.Helpers.cs
- TreeViewDesigner.cs
- EventData.cs
- TableRow.cs
- AlphaSortedEnumConverter.cs
- AttributedMetaModel.cs
- ConstrainedGroup.cs
- MimeReturn.cs
- DesignerCommandSet.cs
- PrintEvent.cs
- FormView.cs
- JsonWriterDelegator.cs
- WizardPanelChangingEventArgs.cs
- RolePrincipal.cs
- DescendantQuery.cs
- SafeProcessHandle.cs
- MimeXmlImporter.cs
- GatewayDefinition.cs
- RunWorkerCompletedEventArgs.cs
- RowType.cs
- BindingNavigatorDesigner.cs
- PathSegmentCollection.cs
- BindableAttribute.cs
- XmlSchemaDatatype.cs
- CodeMemberField.cs
- TraceSection.cs
- NumberSubstitution.cs
- CompiledRegexRunnerFactory.cs
- StateChangeEvent.cs
- SQLBoolean.cs
- TranslateTransform.cs
- TemplateBindingExtension.cs
- ClientData.cs
- RootBuilder.cs
- SerializationEventsCache.cs
- Block.cs
- _NetworkingPerfCounters.cs
- Pen.cs
- DataServiceOperationContext.cs
- GridSplitter.cs
- EmptyEnumerator.cs
- HideDisabledControlAdapter.cs
- ArgumentOutOfRangeException.cs
- HMACSHA384.cs
- ImplicitInputBrush.cs
- TypedTableBase.cs
- ColumnHeader.cs
- Wildcard.cs
- SiteOfOriginContainer.cs
- BufferedWebEventProvider.cs
- EntitySqlQueryBuilder.cs
- UnsafeNativeMethods.cs
- ToolStripPanelSelectionBehavior.cs
- SectionRecord.cs
- AggregateException.cs
- InvokeGenerator.cs
- HotSpot.cs
- Vector3D.cs
- TextElementEnumerator.cs
- QilGenerator.cs
- XmlAnyElementAttributes.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- CatalogPart.cs
- TextEditorMouse.cs
- OdbcError.cs
- DbConnectionHelper.cs
- AttachmentCollection.cs
- PointValueSerializer.cs
- ProgressBarRenderer.cs