Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / CqlGeneration / AliasedSlot.cs / 1305376 / AliasedSlot.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Data.Mapping.ViewGeneration.Structures; using System.Text; using System.Diagnostics; using System.Data.Common.Utils; using System.Collections.Generic; namespace System.Data.Mapping.ViewGeneration.CqlGeneration { // A slot that encapsulates a slot in a particular CqlBlock internal class AliasedSlot : ProjectedSlot { #region Constructor // effects: Creates an alias slot "block.slot" whose output member // path is memberPath - this can be null for boolean slots. slotNum // is the slot number in block to which this slot refers to internal AliasedSlot(CqlBlock block, ProjectedSlot slot, MemberPath memberPath, int slotNum) { Debug.Assert(block != null && slot != null, "Null input to AliasedSlot constructor"); Debug.Assert(slot is AliasedSlot || (slot is BooleanProjectedSlot) == (memberPath == null), "If slot is boolean slot, there is no member path for it and vice-versa"); m_block = block; // Note slot can be another aliased slot m_slot = slot; m_memberPath = memberPath; // Make sure that the aliased slot is projected Debug.Assert(block.IsProjected(slotNum), "Aliased slot " + this + " not being projected " + "in child block "); } #endregion #region Fields private CqlBlock m_block; private ProjectedSlot m_slot; private MemberPath m_memberPath; #endregion #region Properties internal MemberPath MemberPath { get { return m_memberPath; } } internal CqlBlock Block { get { return m_block; } } internal ProjectedSlot InnerSlot { get { return m_slot; } } #endregion #region Methods internal override ProjectedSlot MakeAliasedSlot(CqlBlock block, MemberPath outputPath, int slotNum) { // We take the slot inside this and change the block Debug.Assert(MemberPath.EqualityComparer.Equals(m_memberPath, outputPath), "Different output paths for aliased slot and next use of it higher up in tree"); AliasedSlot result = new AliasedSlot(block, m_slot, outputPath, slotNum); return result; } internal string FullCqlAlias() { return CqlWriter.GetQualifiedName(m_block.CqlAlias, CqlFieldAlias(m_memberPath)); } internal override string CqlFieldAlias(MemberPath outputMember) { // effects: Returns The alias corresponding to this slot, e.g., // _from0 or CPerson1_pid Debug.Assert(MemberPath.EqualityComparer.Equals(outputMember, m_memberPath), "Aliased slot's idea of what the output is different from the Cql block's idea"); ProjectedSlot slot = m_slot; // Keep looking inside the aliased slot till we find a // non-aliased slot and then get the alias name for it // For cycle detection SetslotSet = new Set (); while (true) { Debug.Assert(false == slotSet.Contains(slot), "Cycle detected in aliased slot"); slotSet.Add(slot); AliasedSlot aliasedSlot = slot as AliasedSlot; if (aliasedSlot == null) { break; } slot = aliasedSlot.m_slot; } string result = slot.CqlFieldAlias(m_memberPath); return result; } internal override StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias, int indentLevel) { // Ignore table alias and just get the full alias name builder.Append(FullCqlAlias()); return builder; } internal override void ToCompactString(StringBuilder builder) { StringUtil.FormatStringBuilder(builder, "{0} ", m_block.CqlAlias); m_slot.ToCompactString(builder); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
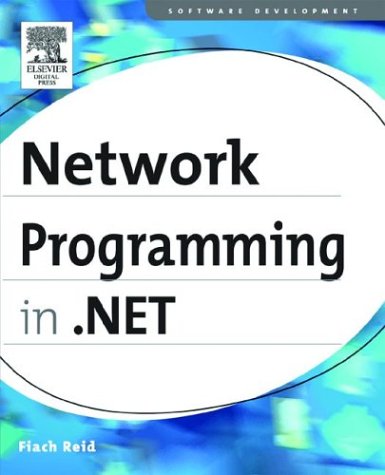
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LoginView.cs
- UdpMessageProperty.cs
- PerformanceCounterManager.cs
- UiaCoreTypesApi.cs
- KeyedCollection.cs
- HMACSHA384.cs
- UnauthorizedWebPart.cs
- Bidi.cs
- UrlMappingCollection.cs
- ConfigXmlSignificantWhitespace.cs
- SecurityDocument.cs
- MouseGesture.cs
- ManagedIStream.cs
- UserUseLicenseDictionaryLoader.cs
- Vector3DConverter.cs
- EngineSite.cs
- ExpressionConverter.cs
- TextEditorSelection.cs
- NetSectionGroup.cs
- EventRouteFactory.cs
- BulletDecorator.cs
- ScriptMethodAttribute.cs
- MimeTypePropertyAttribute.cs
- AssemblyCollection.cs
- TextRangeEdit.cs
- WeakReference.cs
- DataGridViewHeaderCell.cs
- JapaneseLunisolarCalendar.cs
- HMACMD5.cs
- GeneralTransformCollection.cs
- BaseValidator.cs
- DSASignatureFormatter.cs
- XmlSerializerAssemblyAttribute.cs
- DataFormat.cs
- RefExpr.cs
- EnumValidator.cs
- LinqDataSourceSelectEventArgs.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- EdmScalarPropertyAttribute.cs
- ToolStripItemClickedEventArgs.cs
- ExpanderAutomationPeer.cs
- MachineKeySection.cs
- PathGradientBrush.cs
- DataGridColumn.cs
- IsolatedStorage.cs
- SoapIgnoreAttribute.cs
- DataObjectPastingEventArgs.cs
- Trace.cs
- PermissionToken.cs
- SemanticResultKey.cs
- HtmlToClrEventProxy.cs
- PaintEvent.cs
- CodeAttributeArgumentCollection.cs
- AdapterDictionary.cs
- XamlClipboardData.cs
- httpserverutility.cs
- ImpersonateTokenRef.cs
- XmlFormatReaderGenerator.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- ImageSource.cs
- COM2Properties.cs
- TypeForwardedToAttribute.cs
- SqlConnectionManager.cs
- HashRepartitionStream.cs
- TableRowGroupCollection.cs
- ToolTipService.cs
- ValidationResult.cs
- AuthenticationServiceManager.cs
- RemoteArgument.cs
- BuilderPropertyEntry.cs
- XmlSchemaObject.cs
- ECDiffieHellman.cs
- Graph.cs
- Point3DCollectionValueSerializer.cs
- XmlILModule.cs
- PageCache.cs
- DataGridViewCheckBoxCell.cs
- SqlNodeAnnotations.cs
- DynamicEntity.cs
- JapaneseCalendar.cs
- CodeDomDecompiler.cs
- NavigationProperty.cs
- ComEventsMethod.cs
- ProfileProvider.cs
- userdatakeys.cs
- UniformGrid.cs
- ParameterDataSourceExpression.cs
- GiveFeedbackEventArgs.cs
- XmlObjectSerializerReadContext.cs
- UnauthorizedWebPart.cs
- IPEndPointCollection.cs
- IntegerCollectionEditor.cs
- ComboBoxItem.cs
- panel.cs
- If.cs
- ActivationWorker.cs
- FolderBrowserDialog.cs
- List.cs
- VideoDrawing.cs
- DataSvcMapFile.cs