Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Controls / ComboBoxItem.cs / 1 / ComboBoxItem.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ComboBoxItem.cs // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using System; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Media; using System.Windows.Input; using System.Windows.Controls.Primitives; using System.Windows.Shapes; namespace System.Windows.Controls { ////// Control that implements a selectable item inside a ComboBox. /// [Localizability(LocalizationCategory.ComboBox)] #if OLD_AUTOMATION [Automation(AccessibilityControlType = "ListItem")] #endif public class ComboBoxItem : ListBoxItem { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default DependencyObject constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public ComboBoxItem() : base() { } static ComboBoxItem() { DefaultStyleKeyProperty.OverrideMetadata(typeof(ComboBoxItem), new FrameworkPropertyMetadata(typeof(ComboBoxItem))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(ComboBoxItem)); } #endregion #region Public Properties ////// The key needed set a read-only property. /// private static readonly DependencyPropertyKey IsHighlightedPropertyKey = DependencyProperty.RegisterReadOnly("IsHighlighted", typeof(bool), typeof(ComboBoxItem), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); ////// DependencyProperty for the IsHighlighted property /// public static readonly DependencyProperty IsHighlightedProperty = IsHighlightedPropertyKey.DependencyProperty; ////// Indicates if the item is highlighted or not. Styles that want to /// show a highlight for selection should trigger off of this value. /// ///public bool IsHighlighted { get { return (bool)GetValue(IsHighlightedProperty); } protected set { SetValue(IsHighlightedPropertyKey, BooleanBoxes.Box(value)); } } #endregion //-------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------- #region Protected Methods /// /// This is the method that responds to the MouseButtonEvent event. /// /// Event arguments protected override void OnMouseLeftButtonDown(MouseButtonEventArgs e) { e.Handled = true; ComboBox parent = ParentComboBox; if (parent != null) { parent.NotifyComboBoxItemMouseDown(this); } base.OnMouseLeftButtonDown(e); } ////// /// /// protected override void OnMouseLeftButtonUp(MouseButtonEventArgs e) { e.Handled = true; ComboBox parent = ParentComboBox; if (parent != null) { parent.NotifyComboBoxItemMouseUp(this); } base.OnMouseLeftButtonUp(e); } ////// /// /// protected override void OnMouseEnter(MouseEventArgs e) { e.Handled = true; ComboBox parent = ParentComboBox; if (parent != null) { parent.NotifyComboBoxItemEnter(this); } base.OnMouseEnter(e); } ////// Called when Content property has been changed. /// /// /// protected override void OnContentChanged(object oldContent, object newContent) { base.OnContentChanged(oldContent, newContent); // If this is selected, we need to update ParentComboBox.Text // Scenario: //// // In this case ComboBox will try to update Text property as soon as it get // SelectionChanged event. However, at that time ComboBoxItem.Content is not // parse yet. So, Content is null. This causes ComboBox.Text to be "". // ComboBox parent; if (IsSelected && (null != (parent = ParentComboBox))) { parent.SelectedItemUpdated(); } // When the content of the combobox item is a UIElement, // combobox will create a visual clone of the item which needs // to update even when the combobox is closed SetFlags(newContent is UIElement, VisualFlags.IsLayoutIslandRoot); } ///item1 //Item2 //item3 ///// Called when this element gets focus. /// /// protected override void OnGotKeyboardFocus(KeyboardFocusChangedEventArgs e) { e.Handled = true; ComboBox parent = ParentComboBox; if (parent != null) { parent.NotifyComboBoxItemEnter(this); } base.OnGotKeyboardFocus(e); } #endregion //-------------------------------------------------------------------- // // Implementation // //-------------------------------------------------------------------- #region Implementation private ComboBox ParentComboBox { get { return ParentSelector as ComboBox; } } internal void SetIsHighlighted(bool isHighlighted) { IsHighlighted = isHighlighted; } #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // // File: ComboBoxItem.cs // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using System; using System.ComponentModel; using System.Diagnostics; using System.Windows.Threading; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Media; using System.Windows.Input; using System.Windows.Controls.Primitives; using System.Windows.Shapes; namespace System.Windows.Controls { ////// Control that implements a selectable item inside a ComboBox. /// [Localizability(LocalizationCategory.ComboBox)] #if OLD_AUTOMATION [Automation(AccessibilityControlType = "ListItem")] #endif public class ComboBoxItem : ListBoxItem { //------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------- #region Constructors ////// Default DependencyObject constructor /// ////// Automatic determination of current Dispatcher. Use alternative constructor /// that accepts a Dispatcher for best performance. /// public ComboBoxItem() : base() { } static ComboBoxItem() { DefaultStyleKeyProperty.OverrideMetadata(typeof(ComboBoxItem), new FrameworkPropertyMetadata(typeof(ComboBoxItem))); _dType = DependencyObjectType.FromSystemTypeInternal(typeof(ComboBoxItem)); } #endregion #region Public Properties ////// The key needed set a read-only property. /// private static readonly DependencyPropertyKey IsHighlightedPropertyKey = DependencyProperty.RegisterReadOnly("IsHighlighted", typeof(bool), typeof(ComboBoxItem), new FrameworkPropertyMetadata(BooleanBoxes.FalseBox)); ////// DependencyProperty for the IsHighlighted property /// public static readonly DependencyProperty IsHighlightedProperty = IsHighlightedPropertyKey.DependencyProperty; ////// Indicates if the item is highlighted or not. Styles that want to /// show a highlight for selection should trigger off of this value. /// ///public bool IsHighlighted { get { return (bool)GetValue(IsHighlightedProperty); } protected set { SetValue(IsHighlightedPropertyKey, BooleanBoxes.Box(value)); } } #endregion //-------------------------------------------------------------------- // // Protected Methods // //------------------------------------------------------------------- #region Protected Methods /// /// This is the method that responds to the MouseButtonEvent event. /// /// Event arguments protected override void OnMouseLeftButtonDown(MouseButtonEventArgs e) { e.Handled = true; ComboBox parent = ParentComboBox; if (parent != null) { parent.NotifyComboBoxItemMouseDown(this); } base.OnMouseLeftButtonDown(e); } ////// /// /// protected override void OnMouseLeftButtonUp(MouseButtonEventArgs e) { e.Handled = true; ComboBox parent = ParentComboBox; if (parent != null) { parent.NotifyComboBoxItemMouseUp(this); } base.OnMouseLeftButtonUp(e); } ////// /// /// protected override void OnMouseEnter(MouseEventArgs e) { e.Handled = true; ComboBox parent = ParentComboBox; if (parent != null) { parent.NotifyComboBoxItemEnter(this); } base.OnMouseEnter(e); } ////// Called when Content property has been changed. /// /// /// protected override void OnContentChanged(object oldContent, object newContent) { base.OnContentChanged(oldContent, newContent); // If this is selected, we need to update ParentComboBox.Text // Scenario: //// // In this case ComboBox will try to update Text property as soon as it get // SelectionChanged event. However, at that time ComboBoxItem.Content is not // parse yet. So, Content is null. This causes ComboBox.Text to be "". // ComboBox parent; if (IsSelected && (null != (parent = ParentComboBox))) { parent.SelectedItemUpdated(); } // When the content of the combobox item is a UIElement, // combobox will create a visual clone of the item which needs // to update even when the combobox is closed SetFlags(newContent is UIElement, VisualFlags.IsLayoutIslandRoot); } ///item1 //Item2 //item3 ///// Called when this element gets focus. /// /// protected override void OnGotKeyboardFocus(KeyboardFocusChangedEventArgs e) { e.Handled = true; ComboBox parent = ParentComboBox; if (parent != null) { parent.NotifyComboBoxItemEnter(this); } base.OnGotKeyboardFocus(e); } #endregion //-------------------------------------------------------------------- // // Implementation // //-------------------------------------------------------------------- #region Implementation private ComboBox ParentComboBox { get { return ParentSelector as ComboBox; } } internal void SetIsHighlighted(bool isHighlighted) { IsHighlighted = isHighlighted; } #endregion #region DTypeThemeStyleKey // Returns the DependencyObjectType for the registered ThemeStyleKey's default // value. Controls will override this method to return approriate types. internal override DependencyObjectType DTypeThemeStyleKey { get { return _dType; } } private static DependencyObjectType _dType; #endregion DTypeThemeStyleKey } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
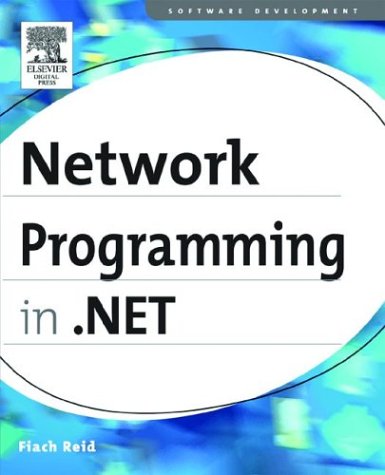
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExpressionBuilder.cs
- basecomparevalidator.cs
- DependencyObject.cs
- ObjectSet.cs
- SmtpTransport.cs
- FrameworkElementAutomationPeer.cs
- MaskedTextBoxDesignerActionList.cs
- TextSelectionHelper.cs
- RemoteWebConfigurationHostServer.cs
- StandardBindingCollectionElement.cs
- DataGridViewLayoutData.cs
- DataGridRow.cs
- PolicyException.cs
- XPathBuilder.cs
- CfgParser.cs
- SecurityElement.cs
- DataKeyArray.cs
- SqlError.cs
- ExpressionBuilder.cs
- EventWaitHandle.cs
- UIHelper.cs
- EncoderParameter.cs
- RuntimeCompatibilityAttribute.cs
- SafeArrayTypeMismatchException.cs
- SymLanguageVendor.cs
- XmlMapping.cs
- CommentEmitter.cs
- ResolvePPIDRequest.cs
- TextTreeObjectNode.cs
- FrameworkTextComposition.cs
- XmlUtil.cs
- EntityUtil.cs
- ImageKeyConverter.cs
- CacheDependency.cs
- MultiView.cs
- ClockGroup.cs
- CreateParams.cs
- BitmapEffectGroup.cs
- MenuBase.cs
- OdbcException.cs
- SerialPort.cs
- ObjectIDGenerator.cs
- FixedHyperLink.cs
- ActivityDefaults.cs
- PageParser.cs
- ISSmlParser.cs
- AsymmetricAlgorithm.cs
- XmlStringTable.cs
- EncoderReplacementFallback.cs
- X509UI.cs
- XmlnsDictionary.cs
- BlobPersonalizationState.cs
- HttpProfileBase.cs
- DataChangedEventManager.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- UIElementParagraph.cs
- SchemaType.cs
- TaskFormBase.cs
- ChannelServices.cs
- GatewayDefinition.cs
- CharStorage.cs
- Source.cs
- DisposableCollectionWrapper.cs
- TemplateField.cs
- NameTable.cs
- MeasureItemEvent.cs
- Convert.cs
- ReferenceList.cs
- PenContext.cs
- ConfigurationSection.cs
- PerformanceCounterPermissionAttribute.cs
- WorkflowInstanceRecord.cs
- DragDrop.cs
- MethodImplAttribute.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- UnitySerializationHolder.cs
- Helpers.cs
- PropertyValue.cs
- TemplatedWizardStep.cs
- List.cs
- XNodeNavigator.cs
- Soap.cs
- XmlEventCache.cs
- ColumnHeader.cs
- SocketInformation.cs
- MappingModelBuildProvider.cs
- NamedPipeTransportBindingElement.cs
- Identity.cs
- InputReport.cs
- RenderingEventArgs.cs
- StateWorkerRequest.cs
- Control.cs
- BindingManagerDataErrorEventArgs.cs
- CalendarDataBindingHandler.cs
- GridViewDeletedEventArgs.cs
- Visual3DCollection.cs
- PermissionAttributes.cs
- TextUtf8RawTextWriter.cs
- InheritanceContextChangedEventManager.cs
- SessionStateContainer.cs