Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / Odbc / OdbcException.cs / 1305376 / OdbcException.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.ComponentModel; //Component using System.Collections; //ICollection using System.Data; using System.Data.Common; using System.Globalization; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Text; namespace System.Data.Odbc { [Serializable] public sealed class OdbcException : System.Data.Common.DbException { OdbcErrorCollection odbcErrors = new OdbcErrorCollection(); ODBC32.RETCODE _retcode; // DO NOT REMOVE! only needed for serialization purposes, because Everett had it. static internal OdbcException CreateException(OdbcErrorCollection errors, ODBC32.RetCode retcode) { StringBuilder builder = new StringBuilder(); foreach (OdbcError error in errors) { if (builder.Length > 0) { builder.Append(Environment.NewLine); } builder.Append(Res.GetString(Res.Odbc_ExceptionMessage, ODBC32.RetcodeToString(retcode), error.SQLState, error.Message)); // MDAC 68337 } OdbcException exception = new OdbcException(builder.ToString(), errors); return exception; } internal OdbcException(string message, OdbcErrorCollection errors) : base(message) { odbcErrors = errors; HResult = HResults.OdbcException; } // runtime will call even if private... private OdbcException(SerializationInfo si, StreamingContext sc) : base(si, sc) { _retcode = (ODBC32.RETCODE) si.GetValue("odbcRetcode", typeof(ODBC32.RETCODE)); odbcErrors = (OdbcErrorCollection) si.GetValue("odbcErrors", typeof(OdbcErrorCollection)); HResult = HResults.OdbcException; } public OdbcErrorCollection Errors { get { return odbcErrors; } } [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.SerializationFormatter)] override public void GetObjectData(SerializationInfo si, StreamingContext context) { // MDAC 72003 if (null == si) { throw new ArgumentNullException("si"); } si.AddValue("odbcRetcode", _retcode, typeof(ODBC32.RETCODE)); si.AddValue("odbcErrors", odbcErrors, typeof(OdbcErrorCollection)); base.GetObjectData(si, context); } // mdac bug 62559 - if we don't have it return nothing (empty string) override public string Source { get { if (0 < Errors.Count) { string source = Errors[0].Source; return ADP.IsEmpty(source) ? "" : source; // base.Source; } return ""; // base.Source; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //[....] //----------------------------------------------------------------------------- using System; using System.ComponentModel; //Component using System.Collections; //ICollection using System.Data; using System.Data.Common; using System.Globalization; using System.Runtime.InteropServices; using System.Runtime.Serialization; using System.Text; namespace System.Data.Odbc { [Serializable] public sealed class OdbcException : System.Data.Common.DbException { OdbcErrorCollection odbcErrors = new OdbcErrorCollection(); ODBC32.RETCODE _retcode; // DO NOT REMOVE! only needed for serialization purposes, because Everett had it. static internal OdbcException CreateException(OdbcErrorCollection errors, ODBC32.RetCode retcode) { StringBuilder builder = new StringBuilder(); foreach (OdbcError error in errors) { if (builder.Length > 0) { builder.Append(Environment.NewLine); } builder.Append(Res.GetString(Res.Odbc_ExceptionMessage, ODBC32.RetcodeToString(retcode), error.SQLState, error.Message)); // MDAC 68337 } OdbcException exception = new OdbcException(builder.ToString(), errors); return exception; } internal OdbcException(string message, OdbcErrorCollection errors) : base(message) { odbcErrors = errors; HResult = HResults.OdbcException; } // runtime will call even if private... private OdbcException(SerializationInfo si, StreamingContext sc) : base(si, sc) { _retcode = (ODBC32.RETCODE) si.GetValue("odbcRetcode", typeof(ODBC32.RETCODE)); odbcErrors = (OdbcErrorCollection) si.GetValue("odbcErrors", typeof(OdbcErrorCollection)); HResult = HResults.OdbcException; } public OdbcErrorCollection Errors { get { return odbcErrors; } } [System.Security.Permissions.SecurityPermissionAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Flags=System.Security.Permissions.SecurityPermissionFlag.SerializationFormatter)] override public void GetObjectData(SerializationInfo si, StreamingContext context) { // MDAC 72003 if (null == si) { throw new ArgumentNullException("si"); } si.AddValue("odbcRetcode", _retcode, typeof(ODBC32.RETCODE)); si.AddValue("odbcErrors", odbcErrors, typeof(OdbcErrorCollection)); base.GetObjectData(si, context); } // mdac bug 62559 - if we don't have it return nothing (empty string) override public string Source { get { if (0 < Errors.Count) { string source = Errors[0].Source; return ADP.IsEmpty(source) ? "" : source; // base.Source; } return ""; // base.Source; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
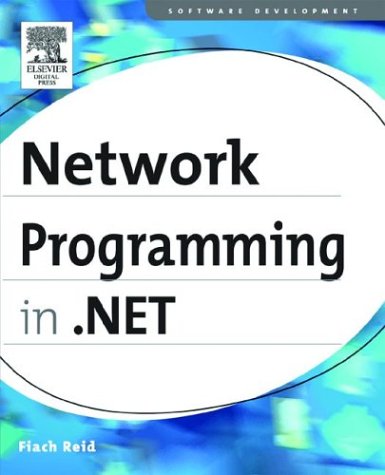
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataRowCollection.cs
- PerformanceCounterPermissionAttribute.cs
- Quad.cs
- OracleCommandBuilder.cs
- _RequestCacheProtocol.cs
- VirtualPathProvider.cs
- HTTPNotFoundHandler.cs
- ParseHttpDate.cs
- PropertyAccessVisitor.cs
- BindingEntityInfo.cs
- ComponentResourceKeyConverter.cs
- ThemeableAttribute.cs
- ItemCheckEvent.cs
- X509Utils.cs
- ListParaClient.cs
- DataGridViewDataErrorEventArgs.cs
- PopupRootAutomationPeer.cs
- SoapFault.cs
- RelationalExpressions.cs
- DecoderNLS.cs
- RequestCacheValidator.cs
- updatecommandorderer.cs
- QueryFunctions.cs
- JoinElimination.cs
- FormViewModeEventArgs.cs
- Scripts.cs
- ModifiableIteratorCollection.cs
- HtmlGenericControl.cs
- HttpHeaderCollection.cs
- SpellerHighlightLayer.cs
- CodeLinePragma.cs
- ThreadStaticAttribute.cs
- EdmComplexTypeAttribute.cs
- PrtCap_Base.cs
- Variant.cs
- TimeSpanSecondsConverter.cs
- EdmItemError.cs
- CachedPathData.cs
- VBCodeProvider.cs
- Site.cs
- XmlIlVisitor.cs
- WizardStepBase.cs
- ObjRef.cs
- SoapProtocolReflector.cs
- OdbcConnectionFactory.cs
- ToolTipService.cs
- _ServiceNameStore.cs
- CalendarDay.cs
- DateTimeConstantAttribute.cs
- CommonObjectSecurity.cs
- ErrorFormatterPage.cs
- ServiceHostingEnvironment.cs
- FixedTextView.cs
- SmtpDateTime.cs
- GridViewDeletedEventArgs.cs
- VariableReference.cs
- MultilineStringConverter.cs
- UserValidatedEventArgs.cs
- FlatButtonAppearance.cs
- XmlSchemaChoice.cs
- ProxyManager.cs
- EpmSyndicationContentSerializer.cs
- _SSPIWrapper.cs
- MatrixStack.cs
- DataServiceQuery.cs
- ShaderEffect.cs
- ADMembershipUser.cs
- UniqueIdentifierService.cs
- _NegotiateClient.cs
- LayoutTableCell.cs
- UInt64Converter.cs
- DiffuseMaterial.cs
- SHA384.cs
- ChannelServices.cs
- _RequestCacheProtocol.cs
- Transform.cs
- SoapInteropTypes.cs
- MdiWindowListItemConverter.cs
- DataGridColumnFloatingHeader.cs
- AttachedAnnotationChangedEventArgs.cs
- SQLByteStorage.cs
- AspNetSynchronizationContext.cs
- SizeConverter.cs
- PrincipalPermission.cs
- ObjectItemCollection.cs
- MULTI_QI.cs
- EntityViewContainer.cs
- RequestCacheValidator.cs
- StringUtil.cs
- ToolTipService.cs
- DateTimeFormat.cs
- ThreadWorkerController.cs
- RijndaelManaged.cs
- CommonDialog.cs
- httpapplicationstate.cs
- CodeAttributeDeclarationCollection.cs
- RoleGroup.cs
- TimeoutHelper.cs
- XmlFileEditor.cs
- Matrix3DConverter.cs