Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Policy / Site.cs / 1305376 / Site.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // Site.cs // // Site is an IIdentity representing internet sites. // using System; using System.Diagnostics.Contracts; using System.Globalization; using System.Security.Permissions; using System.Security.Util; namespace System.Security.Policy { [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public sealed class Site : EvidenceBase, IIdentityPermissionFactory { private SiteString m_name; public Site(String name) { if (name == null) throw new ArgumentNullException("name"); Contract.EndContractBlock(); m_name = new SiteString( name ); } private Site(SiteString name) { Contract.Assert(name != null); m_name = name; } public static Site CreateFromUrl( String url ) { return new Site(ParseSiteFromUrl(url)); } private static SiteString ParseSiteFromUrl( String name ) { URLString urlString = new URLString( name ); if (String.Compare( urlString.Scheme, "file", StringComparison.OrdinalIgnoreCase) == 0) throw new ArgumentException( Environment.GetResourceString( "Argument_InvalidSite" ) ); return new SiteString( new URLString( name ).Host ); } public String Name { get { return m_name.ToString(); } } internal SiteString GetSiteString() { return m_name; } public IPermission CreateIdentityPermission( Evidence evidence ) { return new SiteIdentityPermission( Name ); } public override bool Equals(Object o) { Site other = o as Site; if (other == null) { return false; } return String.Equals(Name, other.Name, StringComparison.OrdinalIgnoreCase); } public override int GetHashCode() { return Name.GetHashCode(); } public override EvidenceBase Clone() { return new Site(m_name); } public Object Copy() { return Clone(); } #if FEATURE_CAS_POLICY internal SecurityElement ToXml() { SecurityElement elem = new SecurityElement( "System.Security.Policy.Site" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. Contract.Assert( this.GetType().FullName.Equals( "System.Security.Policy.Site" ), "Class name changed!" ); elem.AddAttribute( "version", "1" ); if(m_name != null) elem.AddChild( new SecurityElement( "Name", m_name.ToString() ) ); return elem; } #endif // FEATURE_CAS_POLICY #if FEATURE_CAS_POLICY public override String ToString() { return ToXml().ToString(); } #endif // FEATURE_CAS_POLICY // INormalizeForIsolatedStorage is not implemented for startup perf // equivalent to INormalizeForIsolatedStorage.Normalize() internal Object Normalize() { return m_name.ToString().ToUpper(CultureInfo.InvariantCulture); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // Site.cs // // Site is an IIdentity representing internet sites. // using System; using System.Diagnostics.Contracts; using System.Globalization; using System.Security.Permissions; using System.Security.Util; namespace System.Security.Policy { [Serializable] [System.Runtime.InteropServices.ComVisible(true)] public sealed class Site : EvidenceBase, IIdentityPermissionFactory { private SiteString m_name; public Site(String name) { if (name == null) throw new ArgumentNullException("name"); Contract.EndContractBlock(); m_name = new SiteString( name ); } private Site(SiteString name) { Contract.Assert(name != null); m_name = name; } public static Site CreateFromUrl( String url ) { return new Site(ParseSiteFromUrl(url)); } private static SiteString ParseSiteFromUrl( String name ) { URLString urlString = new URLString( name ); if (String.Compare( urlString.Scheme, "file", StringComparison.OrdinalIgnoreCase) == 0) throw new ArgumentException( Environment.GetResourceString( "Argument_InvalidSite" ) ); return new SiteString( new URLString( name ).Host ); } public String Name { get { return m_name.ToString(); } } internal SiteString GetSiteString() { return m_name; } public IPermission CreateIdentityPermission( Evidence evidence ) { return new SiteIdentityPermission( Name ); } public override bool Equals(Object o) { Site other = o as Site; if (other == null) { return false; } return String.Equals(Name, other.Name, StringComparison.OrdinalIgnoreCase); } public override int GetHashCode() { return Name.GetHashCode(); } public override EvidenceBase Clone() { return new Site(m_name); } public Object Copy() { return Clone(); } #if FEATURE_CAS_POLICY internal SecurityElement ToXml() { SecurityElement elem = new SecurityElement( "System.Security.Policy.Site" ); // If you hit this assert then most likely you are trying to change the name of this class. // This is ok as long as you change the hard coded string above and change the assert below. Contract.Assert( this.GetType().FullName.Equals( "System.Security.Policy.Site" ), "Class name changed!" ); elem.AddAttribute( "version", "1" ); if(m_name != null) elem.AddChild( new SecurityElement( "Name", m_name.ToString() ) ); return elem; } #endif // FEATURE_CAS_POLICY #if FEATURE_CAS_POLICY public override String ToString() { return ToXml().ToString(); } #endif // FEATURE_CAS_POLICY // INormalizeForIsolatedStorage is not implemented for startup perf // equivalent to INormalizeForIsolatedStorage.Normalize() internal Object Normalize() { return m_name.ToString().ToUpper(CultureInfo.InvariantCulture); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
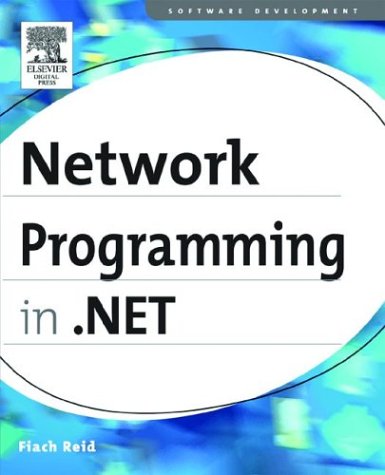
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextProperties.cs
- arc.cs
- GradientStopCollection.cs
- LeftCellWrapper.cs
- OleDbConnection.cs
- VisualTreeHelper.cs
- ComponentEditorForm.cs
- BamlVersionHeader.cs
- CustomAttributeFormatException.cs
- OracleMonthSpan.cs
- Keyboard.cs
- SqlDataSourceQueryEditor.cs
- SamlDelegatingWriter.cs
- TextEditorCharacters.cs
- ExtensionSimplifierMarkupObject.cs
- ColumnMapProcessor.cs
- RegionData.cs
- InputElement.cs
- X509Certificate.cs
- NavigatingCancelEventArgs.cs
- ZoneMembershipCondition.cs
- WebPartTransformerAttribute.cs
- MediaTimeline.cs
- LinqDataSourceStatusEventArgs.cs
- UndoManager.cs
- DragDeltaEventArgs.cs
- PropertyCollection.cs
- PointCollection.cs
- CachedPathData.cs
- TextDecorationLocationValidation.cs
- Win32SafeHandles.cs
- SafeLibraryHandle.cs
- Paragraph.cs
- SourceSwitch.cs
- LinqDataSource.cs
- TextAnchor.cs
- XmlCustomFormatter.cs
- ColorPalette.cs
- DataObjectMethodAttribute.cs
- Typography.cs
- SignHashRequest.cs
- TableLayoutRowStyleCollection.cs
- SqlTrackingWorkflowInstance.cs
- WebPartDisplayModeCollection.cs
- WsiProfilesElement.cs
- EntityViewContainer.cs
- ResumeStoryboard.cs
- ForceCopyBuildProvider.cs
- Rijndael.cs
- SHA512Managed.cs
- LocalValueEnumerator.cs
- IisTraceWebEventProvider.cs
- NavigateEvent.cs
- ALinqExpressionVisitor.cs
- StrokeNodeData.cs
- ItemsPresenter.cs
- WebServiceTypeData.cs
- SecurityCapabilities.cs
- _DigestClient.cs
- DeviceContexts.cs
- PropagatorResult.cs
- TreeView.cs
- DataGridSortingEventArgs.cs
- DispatcherSynchronizationContext.cs
- SqlBuffer.cs
- SqlUDTStorage.cs
- ObjectList.cs
- ISessionStateStore.cs
- Nodes.cs
- PeerNeighborManager.cs
- NetTcpSecurity.cs
- ReadOnlyDataSource.cs
- MethodCallTranslator.cs
- ClientUrlResolverWrapper.cs
- DataGridColumnHeadersPresenter.cs
- WindowsSolidBrush.cs
- OptimizedTemplateContent.cs
- MexTcpBindingCollectionElement.cs
- DescriptionAttribute.cs
- DesignTimeValidationFeature.cs
- DocumentViewerConstants.cs
- WindowsIdentity.cs
- XsltArgumentList.cs
- BamlMapTable.cs
- ValidationService.cs
- XmlSerializerAssemblyAttribute.cs
- LicenseException.cs
- StandardCommands.cs
- ObsoleteAttribute.cs
- PersonalizationDictionary.cs
- SamlAudienceRestrictionCondition.cs
- SafePEFileHandle.cs
- ListViewEditEventArgs.cs
- MetadataCacheItem.cs
- SqlCachedBuffer.cs
- CipherData.cs
- HttpResponse.cs
- PrinterUnitConvert.cs
- GridItemPattern.cs
- TrackingMemoryStreamFactory.cs