Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Input / Keyboard.cs / 1 / Keyboard.cs
using System; using System.Windows; using MS.Win32; using System.Security; using System.Security.Permissions; namespace System.Windows.Input { ////// The Keyboard class represents the mouse device to the /// members of a context. /// ////// The static members of this class simply delegate to the primary /// keyboard device of the calling thread's input manager. /// public static class Keyboard { ////// PreviewKeyDown /// public static readonly RoutedEvent PreviewKeyDownEvent = EventManager.RegisterRoutedEvent("PreviewKeyDown", RoutingStrategy.Tunnel, typeof(KeyEventHandler), typeof(Keyboard)); ////// Adds a handler for the PreviewKeyDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewKeyDownHandler(DependencyObject element, KeyEventHandler handler) { UIElement.AddHandler(element, PreviewKeyDownEvent, handler); } ////// Removes a handler for the PreviewKeyDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewKeyDownHandler(DependencyObject element, KeyEventHandler handler) { UIElement.RemoveHandler(element, PreviewKeyDownEvent, handler); } ////// KeyDown /// public static readonly RoutedEvent KeyDownEvent = EventManager.RegisterRoutedEvent("KeyDown", RoutingStrategy.Bubble, typeof(KeyEventHandler), typeof(Keyboard)); ////// Adds a handler for the KeyDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddKeyDownHandler(DependencyObject element, KeyEventHandler handler) { UIElement.AddHandler(element, KeyDownEvent, handler); } ////// Removes a handler for the KeyDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveKeyDownHandler(DependencyObject element, KeyEventHandler handler) { UIElement.RemoveHandler(element, KeyDownEvent, handler); } ////// PreviewKeyUp /// public static readonly RoutedEvent PreviewKeyUpEvent = EventManager.RegisterRoutedEvent("PreviewKeyUp", RoutingStrategy.Tunnel, typeof(KeyEventHandler), typeof(Keyboard)); ////// Adds a handler for the PreviewKeyUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewKeyUpHandler(DependencyObject element, KeyEventHandler handler) { UIElement.AddHandler(element, PreviewKeyUpEvent, handler); } ////// Removes a handler for the PreviewKeyUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewKeyUpHandler(DependencyObject element, KeyEventHandler handler) { UIElement.RemoveHandler(element, PreviewKeyUpEvent, handler); } ////// KeyUp /// public static readonly RoutedEvent KeyUpEvent = EventManager.RegisterRoutedEvent("KeyUp", RoutingStrategy.Bubble, typeof(KeyEventHandler), typeof(Keyboard)); ////// Adds a handler for the KeyUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddKeyUpHandler(DependencyObject element, KeyEventHandler handler) { UIElement.AddHandler(element, KeyUpEvent, handler); } ////// Removes a handler for the KeyUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void RemoveKeyUpHandler(DependencyObject element, KeyEventHandler handler) { UIElement.RemoveHandler(element, KeyUpEvent, handler); } ////// PreviewGotKeyboardFocus /// public static readonly RoutedEvent PreviewGotKeyboardFocusEvent = EventManager.RegisterRoutedEvent("PreviewGotKeyboardFocus", RoutingStrategy.Tunnel, typeof(KeyboardFocusChangedEventHandler), typeof(Keyboard)); ////// Adds a handler for the PreviewGotKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewGotKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.AddHandler(element, PreviewGotKeyboardFocusEvent, handler); } ////// Removes a handler for the PreviewGotKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void RemovePreviewGotKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.RemoveHandler(element, PreviewGotKeyboardFocusEvent, handler); } ////// GotKeyboardFocus /// public static readonly RoutedEvent GotKeyboardFocusEvent = EventManager.RegisterRoutedEvent("GotKeyboardFocus", RoutingStrategy.Bubble, typeof(KeyboardFocusChangedEventHandler), typeof(Keyboard)); ////// Adds a handler for the GotKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddGotKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.AddHandler(element, GotKeyboardFocusEvent, handler); } ////// Removes a handler for the GotKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveGotKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.RemoveHandler(element, GotKeyboardFocusEvent, handler); } ////// PreviewLostKeyboardFocus /// public static readonly RoutedEvent PreviewLostKeyboardFocusEvent = EventManager.RegisterRoutedEvent("PreviewLostKeyboardFocus", RoutingStrategy.Tunnel, typeof(KeyboardFocusChangedEventHandler), typeof(Keyboard)); ////// Adds a handler for the PreviewLostKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewLostKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.AddHandler(element, PreviewLostKeyboardFocusEvent, handler); } ////// Removes a handler for the PreviewLostKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewLostKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.RemoveHandler(element, PreviewLostKeyboardFocusEvent, handler); } ////// LostKeyboardFocus /// public static readonly RoutedEvent LostKeyboardFocusEvent = EventManager.RegisterRoutedEvent("LostKeyboardFocus", RoutingStrategy.Bubble, typeof(KeyboardFocusChangedEventHandler), typeof(Keyboard)); ////// Adds a handler for the LostKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddLostKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.AddHandler(element, LostKeyboardFocusEvent, handler); } ////// Removes a handler for the LostKeyboardFocus attached event /// /// UIElement or ContentElement that removedto this event /// Event Handler to be removed public static void RemoveLostKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.RemoveHandler(element, LostKeyboardFocusEvent, handler); } ////// Returns the element that the keyboard is focused on. /// public static IInputElement FocusedElement { get { return Keyboard.PrimaryDevice.FocusedElement; } } ////// Focuses the keyboard on a particular element. /// /// /// The element to focus the keyboard on. /// public static IInputElement Focus(IInputElement element) { return Keyboard.PrimaryDevice.Focus(element); } ////// The set of modifier keys currently pressed. /// public static ModifierKeys Modifiers { get { return Keyboard.PrimaryDevice.Modifiers; } } ////// Returns whether or not the specified key is down. /// public static bool IsKeyDown(Key key) { return Keyboard.PrimaryDevice.IsKeyDown(key); } ////// Returns whether or not the specified key is up. /// public static bool IsKeyUp(Key key) { return Keyboard.PrimaryDevice.IsKeyUp(key); } ////// Returns whether or not the specified key is toggled. /// public static bool IsKeyToggled(Key key) { return Keyboard.PrimaryDevice.IsKeyToggled(key); } ////// Returns the state of the specified key. /// public static KeyStates GetKeyStates(Key key) { return Keyboard.PrimaryDevice.GetKeyStates(key); } ////// The primary keyboard device. /// ////// Critical: This code accesses the InputManager which causes an elevation /// PublicOK: It is ok to return the primary device /// public static KeyboardDevice PrimaryDevice { [SecurityCritical] get { KeyboardDevice keyboardDevice = InputManager.UnsecureCurrent.PrimaryKeyboardDevice; return keyboardDevice; } } // Check for Valid enum, as any int can be casted to the enum. internal static bool IsValidKey(Key key) { return ((int)key >= (int)Key.None && (int)key <= (int)Key.OemClear); } ////// Critical: This code accesses critical data(_activeSource) /// TreatAsSafe: Although it accesses critical data it does not modify or expose it, only compares against it. /// [SecurityCritical, SecurityTreatAsSafe] internal static bool IsFocusable(DependencyObject element) { // CODE if(element == null) { return false; } UIElement uie = element as UIElement; if(uie != null) { if(uie.IsVisible == false) { return false; } } if((bool)element.GetValue(UIElement.IsEnabledProperty) == false) { return false; } // CODE bool hasModifiers = false; BaseValueSourceInternal valueSource = element.GetValueSource(UIElement.FocusableProperty, null, out hasModifiers); bool focusable = (bool) element.GetValue(UIElement.FocusableProperty); if(!focusable && valueSource == BaseValueSourceInternal.Default && !hasModifiers) { // The Focusable property was not explicitly set to anything. // The default value is generally false, but true in a few cases. if(FocusManager.GetIsFocusScope(element)) { // Focus scopes are considered focusable, even if // the Focusable property is false. return true; } else if(uie != null && uie.InternalVisualParent == null) { PresentationSource presentationSource = PresentationSource.CriticalFromVisual(uie); if(presentationSource != null) { // A UIElements that is the root of a PresentationSource is considered focusable. return true; } } } return focusable; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Windows; using MS.Win32; using System.Security; using System.Security.Permissions; namespace System.Windows.Input { ////// The Keyboard class represents the mouse device to the /// members of a context. /// ////// The static members of this class simply delegate to the primary /// keyboard device of the calling thread's input manager. /// public static class Keyboard { ////// PreviewKeyDown /// public static readonly RoutedEvent PreviewKeyDownEvent = EventManager.RegisterRoutedEvent("PreviewKeyDown", RoutingStrategy.Tunnel, typeof(KeyEventHandler), typeof(Keyboard)); ////// Adds a handler for the PreviewKeyDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewKeyDownHandler(DependencyObject element, KeyEventHandler handler) { UIElement.AddHandler(element, PreviewKeyDownEvent, handler); } ////// Removes a handler for the PreviewKeyDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewKeyDownHandler(DependencyObject element, KeyEventHandler handler) { UIElement.RemoveHandler(element, PreviewKeyDownEvent, handler); } ////// KeyDown /// public static readonly RoutedEvent KeyDownEvent = EventManager.RegisterRoutedEvent("KeyDown", RoutingStrategy.Bubble, typeof(KeyEventHandler), typeof(Keyboard)); ////// Adds a handler for the KeyDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddKeyDownHandler(DependencyObject element, KeyEventHandler handler) { UIElement.AddHandler(element, KeyDownEvent, handler); } ////// Removes a handler for the KeyDown attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveKeyDownHandler(DependencyObject element, KeyEventHandler handler) { UIElement.RemoveHandler(element, KeyDownEvent, handler); } ////// PreviewKeyUp /// public static readonly RoutedEvent PreviewKeyUpEvent = EventManager.RegisterRoutedEvent("PreviewKeyUp", RoutingStrategy.Tunnel, typeof(KeyEventHandler), typeof(Keyboard)); ////// Adds a handler for the PreviewKeyUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewKeyUpHandler(DependencyObject element, KeyEventHandler handler) { UIElement.AddHandler(element, PreviewKeyUpEvent, handler); } ////// Removes a handler for the PreviewKeyUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewKeyUpHandler(DependencyObject element, KeyEventHandler handler) { UIElement.RemoveHandler(element, PreviewKeyUpEvent, handler); } ////// KeyUp /// public static readonly RoutedEvent KeyUpEvent = EventManager.RegisterRoutedEvent("KeyUp", RoutingStrategy.Bubble, typeof(KeyEventHandler), typeof(Keyboard)); ////// Adds a handler for the KeyUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddKeyUpHandler(DependencyObject element, KeyEventHandler handler) { UIElement.AddHandler(element, KeyUpEvent, handler); } ////// Removes a handler for the KeyUp attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void RemoveKeyUpHandler(DependencyObject element, KeyEventHandler handler) { UIElement.RemoveHandler(element, KeyUpEvent, handler); } ////// PreviewGotKeyboardFocus /// public static readonly RoutedEvent PreviewGotKeyboardFocusEvent = EventManager.RegisterRoutedEvent("PreviewGotKeyboardFocus", RoutingStrategy.Tunnel, typeof(KeyboardFocusChangedEventHandler), typeof(Keyboard)); ////// Adds a handler for the PreviewGotKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewGotKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.AddHandler(element, PreviewGotKeyboardFocusEvent, handler); } ////// Removes a handler for the PreviewGotKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void RemovePreviewGotKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.RemoveHandler(element, PreviewGotKeyboardFocusEvent, handler); } ////// GotKeyboardFocus /// public static readonly RoutedEvent GotKeyboardFocusEvent = EventManager.RegisterRoutedEvent("GotKeyboardFocus", RoutingStrategy.Bubble, typeof(KeyboardFocusChangedEventHandler), typeof(Keyboard)); ////// Adds a handler for the GotKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddGotKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.AddHandler(element, GotKeyboardFocusEvent, handler); } ////// Removes a handler for the GotKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemoveGotKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.RemoveHandler(element, GotKeyboardFocusEvent, handler); } ////// PreviewLostKeyboardFocus /// public static readonly RoutedEvent PreviewLostKeyboardFocusEvent = EventManager.RegisterRoutedEvent("PreviewLostKeyboardFocus", RoutingStrategy.Tunnel, typeof(KeyboardFocusChangedEventHandler), typeof(Keyboard)); ////// Adds a handler for the PreviewLostKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddPreviewLostKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.AddHandler(element, PreviewLostKeyboardFocusEvent, handler); } ////// Removes a handler for the PreviewLostKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be removed public static void RemovePreviewLostKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.RemoveHandler(element, PreviewLostKeyboardFocusEvent, handler); } ////// LostKeyboardFocus /// public static readonly RoutedEvent LostKeyboardFocusEvent = EventManager.RegisterRoutedEvent("LostKeyboardFocus", RoutingStrategy.Bubble, typeof(KeyboardFocusChangedEventHandler), typeof(Keyboard)); ////// Adds a handler for the LostKeyboardFocus attached event /// /// UIElement or ContentElement that listens to this event /// Event Handler to be added public static void AddLostKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.AddHandler(element, LostKeyboardFocusEvent, handler); } ////// Removes a handler for the LostKeyboardFocus attached event /// /// UIElement or ContentElement that removedto this event /// Event Handler to be removed public static void RemoveLostKeyboardFocusHandler(DependencyObject element, KeyboardFocusChangedEventHandler handler) { UIElement.RemoveHandler(element, LostKeyboardFocusEvent, handler); } ////// Returns the element that the keyboard is focused on. /// public static IInputElement FocusedElement { get { return Keyboard.PrimaryDevice.FocusedElement; } } ////// Focuses the keyboard on a particular element. /// /// /// The element to focus the keyboard on. /// public static IInputElement Focus(IInputElement element) { return Keyboard.PrimaryDevice.Focus(element); } ////// The set of modifier keys currently pressed. /// public static ModifierKeys Modifiers { get { return Keyboard.PrimaryDevice.Modifiers; } } ////// Returns whether or not the specified key is down. /// public static bool IsKeyDown(Key key) { return Keyboard.PrimaryDevice.IsKeyDown(key); } ////// Returns whether or not the specified key is up. /// public static bool IsKeyUp(Key key) { return Keyboard.PrimaryDevice.IsKeyUp(key); } ////// Returns whether or not the specified key is toggled. /// public static bool IsKeyToggled(Key key) { return Keyboard.PrimaryDevice.IsKeyToggled(key); } ////// Returns the state of the specified key. /// public static KeyStates GetKeyStates(Key key) { return Keyboard.PrimaryDevice.GetKeyStates(key); } ////// The primary keyboard device. /// ////// Critical: This code accesses the InputManager which causes an elevation /// PublicOK: It is ok to return the primary device /// public static KeyboardDevice PrimaryDevice { [SecurityCritical] get { KeyboardDevice keyboardDevice = InputManager.UnsecureCurrent.PrimaryKeyboardDevice; return keyboardDevice; } } // Check for Valid enum, as any int can be casted to the enum. internal static bool IsValidKey(Key key) { return ((int)key >= (int)Key.None && (int)key <= (int)Key.OemClear); } ////// Critical: This code accesses critical data(_activeSource) /// TreatAsSafe: Although it accesses critical data it does not modify or expose it, only compares against it. /// [SecurityCritical, SecurityTreatAsSafe] internal static bool IsFocusable(DependencyObject element) { // CODE if(element == null) { return false; } UIElement uie = element as UIElement; if(uie != null) { if(uie.IsVisible == false) { return false; } } if((bool)element.GetValue(UIElement.IsEnabledProperty) == false) { return false; } // CODE bool hasModifiers = false; BaseValueSourceInternal valueSource = element.GetValueSource(UIElement.FocusableProperty, null, out hasModifiers); bool focusable = (bool) element.GetValue(UIElement.FocusableProperty); if(!focusable && valueSource == BaseValueSourceInternal.Default && !hasModifiers) { // The Focusable property was not explicitly set to anything. // The default value is generally false, but true in a few cases. if(FocusManager.GetIsFocusScope(element)) { // Focus scopes are considered focusable, even if // the Focusable property is false. return true; } else if(uie != null && uie.InternalVisualParent == null) { PresentationSource presentationSource = PresentationSource.CriticalFromVisual(uie); if(presentationSource != null) { // A UIElements that is the root of a PresentationSource is considered focusable. return true; } } } return focusable; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
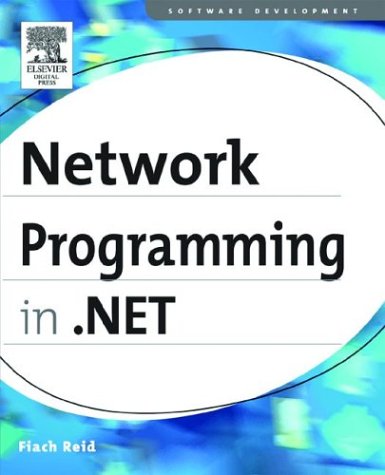
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XmlSchemaAnnotation.cs
- StandardCommands.cs
- Profiler.cs
- FixedPageStructure.cs
- indexingfiltermarshaler.cs
- TableDetailsRow.cs
- TileBrush.cs
- ImageClickEventArgs.cs
- MDIWindowDialog.cs
- WindowsFormsSectionHandler.cs
- ColorConvertedBitmap.cs
- TaskSchedulerException.cs
- HandoffBehavior.cs
- COM2FontConverter.cs
- PrivilegeNotHeldException.cs
- HwndHost.cs
- Util.cs
- LongCountAggregationOperator.cs
- BitmapData.cs
- CaseInsensitiveOrdinalStringComparer.cs
- PrintPageEvent.cs
- TraceHandlerErrorFormatter.cs
- sqlpipe.cs
- EntityModelBuildProvider.cs
- MetadataArtifactLoader.cs
- FastEncoderWindow.cs
- OutputWindow.cs
- WebPartChrome.cs
- SQlBooleanStorage.cs
- FormsAuthenticationUser.cs
- XmlSerializerNamespaces.cs
- SerializationAttributes.cs
- CheckBoxFlatAdapter.cs
- CaseKeyBox.xaml.cs
- HttpHandlersSection.cs
- DecimalConverter.cs
- ParseHttpDate.cs
- PropertyNames.cs
- OdbcCommand.cs
- SharedStatics.cs
- _SingleItemRequestCache.cs
- _BasicClient.cs
- NavigateEvent.cs
- DoWorkEventArgs.cs
- EntityContainerEmitter.cs
- MessageQueueKey.cs
- CellTreeNodeVisitors.cs
- PTProvider.cs
- SpellerInterop.cs
- AggregateNode.cs
- QuaternionRotation3D.cs
- HotCommands.cs
- HostExecutionContextManager.cs
- DoubleStorage.cs
- Properties.cs
- Quaternion.cs
- WindowsAuthenticationEventArgs.cs
- ToolStripItemCollection.cs
- SiteMapNodeCollection.cs
- ContextMarshalException.cs
- SelectionListComponentEditor.cs
- Point3DCollection.cs
- ModelItemKeyValuePair.cs
- ConfigurationSectionCollection.cs
- WebConfigurationManager.cs
- NamespaceInfo.cs
- OptimisticConcurrencyException.cs
- BuildResult.cs
- FrameworkContentElement.cs
- LongPath.cs
- DependencyPropertyConverter.cs
- ClientOptions.cs
- StyleSelector.cs
- HttpCookiesSection.cs
- StylusPoint.cs
- SqlIdentifier.cs
- ScriptControlDescriptor.cs
- JapaneseLunisolarCalendar.cs
- ResolveRequestResponseAsyncResult.cs
- EntityContainerEmitter.cs
- FixedTextPointer.cs
- ObjectFullSpanRewriter.cs
- PropertyGridEditorPart.cs
- WebResourceAttribute.cs
- RequestStatusBarUpdateEventArgs.cs
- VersionedStream.cs
- SqlBinder.cs
- CacheDict.cs
- BamlLocalizationDictionary.cs
- DBCommandBuilder.cs
- TdsParser.cs
- PartialTrustVisibleAssembliesSection.cs
- BrowserCapabilitiesCompiler.cs
- RegexStringValidatorAttribute.cs
- StorageModelBuildProvider.cs
- columnmapfactory.cs
- ValidatorUtils.cs
- UnsupportedPolicyOptionsException.cs
- PeerCollaboration.cs
- ConfigsHelper.cs