Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ComponentModel / COM2Interop / COM2FontConverter.cs / 1305376 / COM2FontConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Collections; using Microsoft.Win32; using System.Runtime.Versioning; ////// /// This class maps an OLE_COLOR to a managed Color editor. /// internal class Com2FontConverter : Com2DataTypeToManagedDataTypeConverter { private IntPtr lastHandle = IntPtr.Zero; private Font lastFont = null; public override bool AllowExpand { get { return true; } } ////// /// Returns the managed type that this editor maps the property type to. /// public override Type ManagedType { get { return typeof(Font); } } ////// /// Converts the native value into a managed value /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public override object ConvertNativeToManaged(object nativeValue, Com2PropertyDescriptor pd) { // we're getting an IFont thing here UnsafeNativeMethods.IFont nativeFont = nativeValue as UnsafeNativeMethods.IFont; if (nativeFont == null) { lastHandle = IntPtr.Zero; lastFont = Control.DefaultFont; return lastFont; } IntPtr fontHandle = nativeFont.GetHFont(); // see if we have this guy cached if (fontHandle == lastHandle && lastFont != null) { return lastFont; } lastHandle = fontHandle; try { // this wasn't working because it was converting everything to // world units. // Font font = Font.FromHfont(lastHandle); try { lastFont = ControlPaint.FontInPoints(font); } finally { font.Dispose(); } } catch(ArgumentException) { // we will fail on non-truetype fonts, so // just use the default font. lastFont = Control.DefaultFont; } return lastFont; } ////// /// Converts the managed value into a native value /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public override object ConvertManagedToNative(object managedValue, Com2PropertyDescriptor pd, ref bool cancelSet) { // we default to black. // if (managedValue == null) { managedValue = Control.DefaultFont; } cancelSet = true; if (lastFont != null && lastFont.Equals(managedValue)) { // don't do anything here. return null; } lastFont = (Font)managedValue; UnsafeNativeMethods.IFont nativeFont = (UnsafeNativeMethods.IFont)pd.GetNativeValue(pd.TargetObject); // now, push all the values into the native side if (nativeFont != null) { bool changed = ControlPaint.FontToIFont(lastFont, nativeFont); if (changed) { // here, we want to pick up a new font from the handle lastFont = null; ConvertNativeToManaged(nativeFont, pd); } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms.ComponentModel.Com2Interop { using System.Runtime.Serialization.Formatters; using System.Runtime.InteropServices; using System.ComponentModel; using System.Diagnostics; using System; using System.Drawing; using System.Collections; using Microsoft.Win32; using System.Runtime.Versioning; ////// /// This class maps an OLE_COLOR to a managed Color editor. /// internal class Com2FontConverter : Com2DataTypeToManagedDataTypeConverter { private IntPtr lastHandle = IntPtr.Zero; private Font lastFont = null; public override bool AllowExpand { get { return true; } } ////// /// Returns the managed type that this editor maps the property type to. /// public override Type ManagedType { get { return typeof(Font); } } ////// /// Converts the native value into a managed value /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public override object ConvertNativeToManaged(object nativeValue, Com2PropertyDescriptor pd) { // we're getting an IFont thing here UnsafeNativeMethods.IFont nativeFont = nativeValue as UnsafeNativeMethods.IFont; if (nativeFont == null) { lastHandle = IntPtr.Zero; lastFont = Control.DefaultFont; return lastFont; } IntPtr fontHandle = nativeFont.GetHFont(); // see if we have this guy cached if (fontHandle == lastHandle && lastFont != null) { return lastFont; } lastHandle = fontHandle; try { // this wasn't working because it was converting everything to // world units. // Font font = Font.FromHfont(lastHandle); try { lastFont = ControlPaint.FontInPoints(font); } finally { font.Dispose(); } } catch(ArgumentException) { // we will fail on non-truetype fonts, so // just use the default font. lastFont = Control.DefaultFont; } return lastFont; } ////// /// Converts the managed value into a native value /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public override object ConvertManagedToNative(object managedValue, Com2PropertyDescriptor pd, ref bool cancelSet) { // we default to black. // if (managedValue == null) { managedValue = Control.DefaultFont; } cancelSet = true; if (lastFont != null && lastFont.Equals(managedValue)) { // don't do anything here. return null; } lastFont = (Font)managedValue; UnsafeNativeMethods.IFont nativeFont = (UnsafeNativeMethods.IFont)pd.GetNativeValue(pd.TargetObject); // now, push all the values into the native side if (nativeFont != null) { bool changed = ControlPaint.FontToIFont(lastFont, nativeFont); if (changed) { // here, we want to pick up a new font from the handle lastFont = null; ConvertNativeToManaged(nativeFont, pd); } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
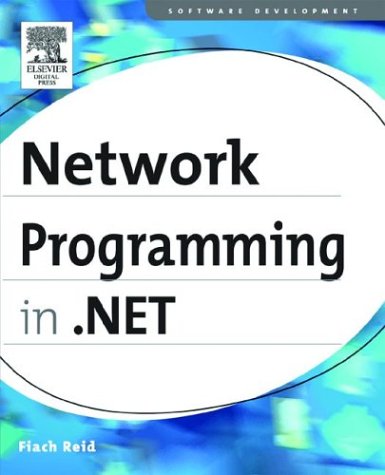
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- _DisconnectOverlappedAsyncResult.cs
- CustomErrorCollection.cs
- ParserHooks.cs
- AutomationProperty.cs
- Single.cs
- TextureBrush.cs
- CodePageUtils.cs
- CharConverter.cs
- UxThemeWrapper.cs
- XpsFilter.cs
- SrgsDocument.cs
- LogSwitch.cs
- SubMenuStyle.cs
- CallbackDebugBehavior.cs
- WriteFileContext.cs
- LocatorPartList.cs
- UnionCodeGroup.cs
- FormViewDeleteEventArgs.cs
- TextLineBreak.cs
- TableRowsCollectionEditor.cs
- OracleColumn.cs
- BulletChrome.cs
- CodeDelegateCreateExpression.cs
- AlphabeticalEnumConverter.cs
- DependencyPropertyKind.cs
- PrimitiveSchema.cs
- NotSupportedException.cs
- InstanceLockedException.cs
- RelationshipDetailsCollection.cs
- EventManager.cs
- ContextMenu.cs
- FileSecurity.cs
- DynamicHyperLink.cs
- ImageInfo.cs
- ApplicationHost.cs
- SwitchAttribute.cs
- DataListCommandEventArgs.cs
- CustomCredentialPolicy.cs
- StyleHelper.cs
- TextOnlyOutput.cs
- LoadedOrUnloadedOperation.cs
- StringUtil.cs
- COM2PropertyBuilderUITypeEditor.cs
- ProcessInfo.cs
- SubMenuStyleCollection.cs
- RegexTree.cs
- GridViewUpdatedEventArgs.cs
- MachineSettingsSection.cs
- XmlNavigatorFilter.cs
- InvariantComparer.cs
- Simplifier.cs
- EncodingNLS.cs
- WpfGeneratedKnownProperties.cs
- Hex.cs
- Point3DValueSerializer.cs
- DeploymentExceptionMapper.cs
- DelegatingTypeDescriptionProvider.cs
- Positioning.cs
- WizardPanelChangingEventArgs.cs
- WebBaseEventKeyComparer.cs
- GridEntry.cs
- XmlSerializerFactory.cs
- ScriptControlManager.cs
- PingOptions.cs
- ByteConverter.cs
- DirectionalLight.cs
- TextBlock.cs
- NoneExcludedImageIndexConverter.cs
- WebPartAuthorizationEventArgs.cs
- SyndicationItemFormatter.cs
- WorkflowViewManager.cs
- Compensate.cs
- PasswordBoxAutomationPeer.cs
- CatalogPartChrome.cs
- PrintPreviewGraphics.cs
- CategoryAttribute.cs
- updateconfighost.cs
- ResourceAssociationTypeEnd.cs
- WCFBuildProvider.cs
- DiagnosticEventProvider.cs
- KeyGestureConverter.cs
- DispatcherHooks.cs
- SHA384CryptoServiceProvider.cs
- TransferMode.cs
- SRef.cs
- FreezableOperations.cs
- ConcurrencyMode.cs
- GB18030Encoding.cs
- RectAnimationClockResource.cs
- ArrayTypeMismatchException.cs
- UriTemplatePathSegment.cs
- XamlPointCollectionSerializer.cs
- RotateTransform3D.cs
- NavigationPropertyEmitter.cs
- InvalidDataException.cs
- ProcessInfo.cs
- RuntimeConfigLKG.cs
- AuthenticationManager.cs
- TemplatePartAttribute.cs
- InternalResources.cs