Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / ProcessInfo.cs / 1305376 / ProcessInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * ProcessInfo class */ namespace System.Web { using System.Threading; using System.Security.Permissions; ////// public enum ProcessStatus { ///Provides enumerated values representing status of a process. ////// Alive = 1, ///Specifies that the process is running. ////// ShuttingDown = 2, ///Specifies that the process has begun shutting down. ////// ShutDown = 3, ///Specifies the the process has been shut down. ////// Terminated = 4 } ///Specifies that the process has been terminated. ////// public enum ProcessShutdownReason { ///Provides enumerated values representing the reason a process has shut /// down. ////// None = 0, // alive ///Specifies that the process has not been shut down. ////// Unexpected = 1, ///Specifies that the process has been shut down unexpectedly. ////// RequestsLimit = 2, ///Specifies that the process request exceeded the limit on number of /// processes. ////// RequestQueueLimit = 3, ///Specifies that the process request exceeded the limit on number of /// processes in que. ////// Timeout = 4, ///Specifies that the process timed out. ////// IdleTimeout = 5, ///Specifies that the process exceeded the limit on process idle time. ////// MemoryLimitExceeded = 6, PingFailed = 7, DeadlockSuspected = 8 } ///Specifies that the process exceeded the limit of memory available per process. ////// public class ProcessInfo { ///Provides information on processes. ////// public DateTime StartTime { get { return _StartTime;}} ///Indicates the time a process was started. ////// public TimeSpan Age { get { return _Age;}} ///Indicates the length of time the process has been running. ////// public int ProcessID { get { return _ProcessID;}} public int RequestCount { get { return _RequestCount;}} ///Indicates the process id of the process. ////// public ProcessStatus Status { get { return _Status;}} ///Indicates the current status of the process. ////// public ProcessShutdownReason ShutdownReason { get { return _ShutdownReason;}} ///Indicates the reason the process shut down. ////// public int PeakMemoryUsed { get { return _PeakMemoryUsed;}} private DateTime _StartTime; private TimeSpan _Age; private int _ProcessID; private int _RequestCount; private ProcessStatus _Status; private ProcessShutdownReason _ShutdownReason; private int _PeakMemoryUsed; ///Indicates the maximum amount of memory the process has used. ////// public void SetAll (DateTime startTime, TimeSpan age, int processID, int requestCount, ProcessStatus status, ProcessShutdownReason shutdownReason, int peakMemoryUsed) { _StartTime = startTime; _Age = age; _ProcessID = processID; _RequestCount = requestCount; _Status = status; _ShutdownReason = shutdownReason; _PeakMemoryUsed = peakMemoryUsed; } ///Sets internal information indicating the status of the process. ////// public ProcessInfo (DateTime startTime, TimeSpan age, int processID, int requestCount, ProcessStatus status, ProcessShutdownReason shutdownReason, int peakMemoryUsed) { _StartTime = startTime; _Age = age; _ProcessID = processID; _RequestCount = requestCount; _Status = status; _ShutdownReason = shutdownReason; _PeakMemoryUsed = peakMemoryUsed; } public ProcessInfo() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //Initializes a new instance of the ///class and sets internal information /// indicating the status of the process. // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * ProcessInfo class */ namespace System.Web { using System.Threading; using System.Security.Permissions; ////// public enum ProcessStatus { ///Provides enumerated values representing status of a process. ////// Alive = 1, ///Specifies that the process is running. ////// ShuttingDown = 2, ///Specifies that the process has begun shutting down. ////// ShutDown = 3, ///Specifies the the process has been shut down. ////// Terminated = 4 } ///Specifies that the process has been terminated. ////// public enum ProcessShutdownReason { ///Provides enumerated values representing the reason a process has shut /// down. ////// None = 0, // alive ///Specifies that the process has not been shut down. ////// Unexpected = 1, ///Specifies that the process has been shut down unexpectedly. ////// RequestsLimit = 2, ///Specifies that the process request exceeded the limit on number of /// processes. ////// RequestQueueLimit = 3, ///Specifies that the process request exceeded the limit on number of /// processes in que. ////// Timeout = 4, ///Specifies that the process timed out. ////// IdleTimeout = 5, ///Specifies that the process exceeded the limit on process idle time. ////// MemoryLimitExceeded = 6, PingFailed = 7, DeadlockSuspected = 8 } ///Specifies that the process exceeded the limit of memory available per process. ////// public class ProcessInfo { ///Provides information on processes. ////// public DateTime StartTime { get { return _StartTime;}} ///Indicates the time a process was started. ////// public TimeSpan Age { get { return _Age;}} ///Indicates the length of time the process has been running. ////// public int ProcessID { get { return _ProcessID;}} public int RequestCount { get { return _RequestCount;}} ///Indicates the process id of the process. ////// public ProcessStatus Status { get { return _Status;}} ///Indicates the current status of the process. ////// public ProcessShutdownReason ShutdownReason { get { return _ShutdownReason;}} ///Indicates the reason the process shut down. ////// public int PeakMemoryUsed { get { return _PeakMemoryUsed;}} private DateTime _StartTime; private TimeSpan _Age; private int _ProcessID; private int _RequestCount; private ProcessStatus _Status; private ProcessShutdownReason _ShutdownReason; private int _PeakMemoryUsed; ///Indicates the maximum amount of memory the process has used. ////// public void SetAll (DateTime startTime, TimeSpan age, int processID, int requestCount, ProcessStatus status, ProcessShutdownReason shutdownReason, int peakMemoryUsed) { _StartTime = startTime; _Age = age; _ProcessID = processID; _RequestCount = requestCount; _Status = status; _ShutdownReason = shutdownReason; _PeakMemoryUsed = peakMemoryUsed; } ///Sets internal information indicating the status of the process. ////// public ProcessInfo (DateTime startTime, TimeSpan age, int processID, int requestCount, ProcessStatus status, ProcessShutdownReason shutdownReason, int peakMemoryUsed) { _StartTime = startTime; _Age = age; _ProcessID = processID; _RequestCount = requestCount; _Status = status; _ShutdownReason = shutdownReason; _PeakMemoryUsed = peakMemoryUsed; } public ProcessInfo() { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.Initializes a new instance of the ///class and sets internal information /// indicating the status of the process.
Link Menu
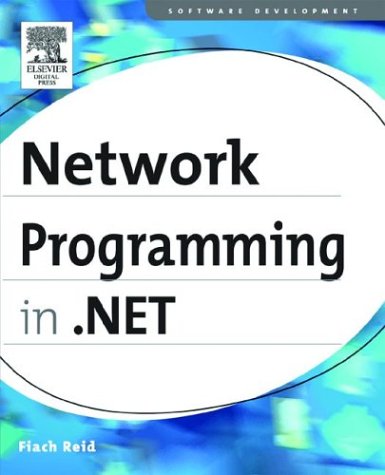
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyValueUIItem.cs
- DataGridViewAccessibleObject.cs
- TableLayoutSettings.cs
- HebrewCalendar.cs
- ByteStream.cs
- MsmqBindingElementBase.cs
- CodeNamespaceCollection.cs
- DataContractSerializerSection.cs
- DrawingVisual.cs
- SplayTreeNode.cs
- MappingException.cs
- AnimatedTypeHelpers.cs
- ProfileInfo.cs
- CheckBox.cs
- DataGridSortingEventArgs.cs
- TableAdapterManagerGenerator.cs
- SpStreamWrapper.cs
- TdsParserStateObject.cs
- WebEncodingValidator.cs
- DEREncoding.cs
- XmlSchemaIdentityConstraint.cs
- EntityTemplateFactory.cs
- UrlMapping.cs
- DecoderBestFitFallback.cs
- Operator.cs
- ProfilePropertySettingsCollection.cs
- CodeBinaryOperatorExpression.cs
- CssTextWriter.cs
- ResponseStream.cs
- UserPreferenceChangingEventArgs.cs
- VerificationAttribute.cs
- SynchronizationLockException.cs
- Effect.cs
- TraversalRequest.cs
- DisposableCollectionWrapper.cs
- HtmlInputRadioButton.cs
- DataControlFieldCell.cs
- Panel.cs
- NullableFloatAverageAggregationOperator.cs
- Form.cs
- DataRecordInternal.cs
- SessionEndedEventArgs.cs
- ReliableRequestSessionChannel.cs
- ComplexLine.cs
- CssTextWriter.cs
- ImageListStreamer.cs
- Link.cs
- DriveNotFoundException.cs
- xmlfixedPageInfo.cs
- COM2TypeInfoProcessor.cs
- XmlSchemaFacet.cs
- ResourceDictionary.cs
- XmlSchemas.cs
- SQLGuid.cs
- X509Extension.cs
- MenuItem.cs
- ToolStripButton.cs
- cookie.cs
- DataSourceControlBuilder.cs
- XmlAtomicValue.cs
- OutputScopeManager.cs
- Math.cs
- XNodeValidator.cs
- InputGestureCollection.cs
- ResourceBinder.cs
- TargetParameterCountException.cs
- ParseChildrenAsPropertiesAttribute.cs
- QuotedPairReader.cs
- TemplateColumn.cs
- HandleValueEditor.cs
- ConfigXmlElement.cs
- MethodBuilderInstantiation.cs
- RpcResponse.cs
- LicenseException.cs
- AsyncContentLoadedEventArgs.cs
- HandlerWithFactory.cs
- DateRangeEvent.cs
- AttachedPropertyBrowsableAttribute.cs
- ServiceDescription.cs
- PlanCompilerUtil.cs
- MarkupObject.cs
- SchemaNotation.cs
- FormattedTextSymbols.cs
- SecurityTokenTypes.cs
- FormsAuthenticationCredentials.cs
- FileClassifier.cs
- BehaviorEditorPart.cs
- ProjectionPruner.cs
- UiaCoreProviderApi.cs
- TemplateField.cs
- TextStore.cs
- Root.cs
- TransformCollection.cs
- ExceptionUtility.cs
- ObjectViewFactory.cs
- ZipIOModeEnforcingStream.cs
- DataGridColumn.cs
- Model3DCollection.cs
- OdbcConnectionStringbuilder.cs
- XmlEntityReference.cs