Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / IO / Packaging / xmlfixedPageInfo.cs / 1305600 / xmlfixedPageInfo.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Implements a DOM-based subclass of the FixedPageInfo abstract class. // The class functions as an array of XmlGlyphRunInfo's in markup order. // // History: // 05/06/2004: JohnLarc: Initial implementation //--------------------------------------------------------------------------- using System; using System.Windows; // For ExceptionStringTable using System.Xml; // For DOM objects using System.Diagnostics; // For Assert using System.Globalization; // For CultureInfo namespace MS.Internal.IO.Packaging { internal class XmlFixedPageInfo : MS.Internal.FixedPageInfo { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Initialize object from DOM node. /// ////// The DOM node is assumed to be a XAML FixedPage element. Its namespace URI /// is subsequently used to look for its nested Glyphs elements (see private property NodeList). /// internal XmlFixedPageInfo(XmlNode fixedPageNode) { _pageNode = fixedPageNode; Debug.Assert(_pageNode != null); if (_pageNode.LocalName != _fixedPageName || _pageNode.NamespaceURI != ElementTableKey.FixedMarkupNamespace) { throw new ArgumentException(SR.Get(SRID.UnexpectedXmlNodeInXmlFixedPageInfoConstructor, _pageNode.NamespaceURI, _pageNode.LocalName, ElementTableKey.FixedMarkupNamespace, _fixedPageName)); } } #endregion Constructors //------------------------------------------------------ // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Get the glyph run at zero-based position 'position'. /// ////// Returns null for a nonexistent position. No exception raised. /// internal override GlyphRunInfo GlyphRunAtPosition(int position) { if (position < 0 || position >= GlyphRunList.Length) { return null; } if (GlyphRunList[position] == null) { GlyphRunList[position] = new XmlGlyphRunInfo(NodeList[position]); } return GlyphRunList[position]; } #endregion Internal Methods //------------------------------------------------------ // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Indicates the number of glyph runs on the page. /// internal override int GlyphRunCount { get { return GlyphRunList.Length; } } #endregion Internal Properties //----------------------------------------------------- // // Private Properties // //------------------------------------------------------ #region Private Properties ////// Lazily initialize _glyphRunList, an array of XmlGlyphInfo objects, /// using the NodeList private property. /// ////// When using Visual Studio to step through code using this property, make sure the option /// "Allow property evaluation in variables windows" is unchecked. /// private XmlGlyphRunInfo[] GlyphRunList { get { if (_glyphRunList == null) { _glyphRunList = new XmlGlyphRunInfo[NodeList.Count]; } return _glyphRunList; } } ////// Lazily initialize the list of Glyphs elements on the page using XPath. /// ////// When using Visual Studio to step through code using this property, make sure the option /// "Allow property evaluation in variables windows" is unchecked. /// private XmlNodeList NodeList { get { if (_nodeList == null) { string glyphRunQuery = String.Format(CultureInfo.InvariantCulture, ".//*[namespace-uri()='{0}' and local-name()='{1}']", ElementTableKey.FixedMarkupNamespace, _glyphRunName); _nodeList = _pageNode.SelectNodes(glyphRunQuery); } return _nodeList; } } #endregion Private Properties //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields #region Constants private const string _fixedPageName = "FixedPage"; private const string _glyphRunName = "Glyphs"; #endregion Constants private XmlNode _pageNode; private XmlNodeList _nodeList = null; private XmlGlyphRunInfo[] _glyphRunList = null; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
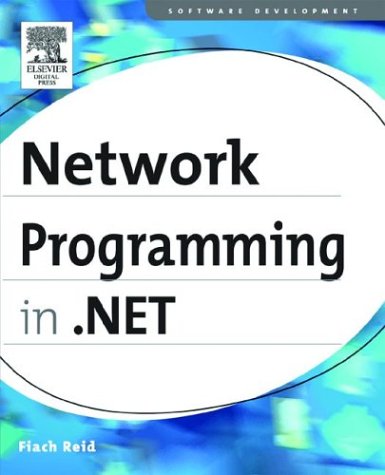
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AutomationElementCollection.cs
- RawUIStateInputReport.cs
- SrgsRulesCollection.cs
- TdsParserSafeHandles.cs
- QilTernary.cs
- Util.cs
- RectAnimationClockResource.cs
- WebScriptEnablingBehavior.cs
- RuntimeHelpers.cs
- TargetException.cs
- CommonXSendMessage.cs
- DeferredRunTextReference.cs
- LowerCaseStringConverter.cs
- SafeHandles.cs
- ProfileGroupSettingsCollection.cs
- HierarchicalDataBoundControl.cs
- ListControl.cs
- ManagementClass.cs
- NativeWindow.cs
- DodSequenceMerge.cs
- TextProviderWrapper.cs
- SafeMILHandleMemoryPressure.cs
- View.cs
- ClientData.cs
- MediaSystem.cs
- DataSourceControl.cs
- DragStartedEventArgs.cs
- WindowsFormsDesignerOptionService.cs
- DataKeyArray.cs
- RegexMatch.cs
- Matrix3DConverter.cs
- OpenCollectionAsyncResult.cs
- SpellerInterop.cs
- NumberAction.cs
- StateDesignerConnector.cs
- Accessible.cs
- SetIndexBinder.cs
- HtmlControl.cs
- BamlResourceSerializer.cs
- LinearGradientBrush.cs
- Component.cs
- ReliableMessagingHelpers.cs
- X509CertificateCollection.cs
- ServiceSecurityContext.cs
- InvalidPrinterException.cs
- SizeChangedEventArgs.cs
- TaiwanCalendar.cs
- MultiBindingExpression.cs
- BindingsCollection.cs
- HwndTarget.cs
- CachedCompositeFamily.cs
- UniqueIdentifierService.cs
- EntityDesignerUtils.cs
- CatalogUtil.cs
- ObjectMemberMapping.cs
- Version.cs
- QuaternionAnimation.cs
- ApplicationManager.cs
- ApplicationId.cs
- WebPartExportVerb.cs
- AuthenticationException.cs
- UnsafeNativeMethods.cs
- unsafenativemethodsother.cs
- SqlTypeSystemProvider.cs
- ColorMap.cs
- TextAutomationPeer.cs
- ListControl.cs
- _TimerThread.cs
- Column.cs
- FormViewUpdateEventArgs.cs
- EventEntry.cs
- CalculatedColumn.cs
- ComboBoxHelper.cs
- SurrogateEncoder.cs
- SqlWriter.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- CodeBinaryOperatorExpression.cs
- SerialStream.cs
- HMACSHA384.cs
- FrameworkTextComposition.cs
- BatchWriter.cs
- FamilyTypeface.cs
- ObjectDataSourceView.cs
- CqlQuery.cs
- ISAPIApplicationHost.cs
- CompilerScope.Storage.cs
- BamlLocalizationDictionary.cs
- GifBitmapEncoder.cs
- RuleConditionDialog.cs
- GlyphTypeface.cs
- NonBatchDirectoryCompiler.cs
- KeyConverter.cs
- TextLineResult.cs
- ContextStaticAttribute.cs
- IDReferencePropertyAttribute.cs
- ClientOptions.cs
- Facet.cs
- DefaultBindingPropertyAttribute.cs
- ControlBuilder.cs
- DataPagerFieldCollection.cs