Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / Utils / Util.cs / 1305376 / Util.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // Util.cs // //[....] // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System.Collections.Generic; namespace System.Linq.Parallel { ////// Common miscellaneous utility methods used throughout the code-base. /// internal static class Util { //------------------------------------------------------------------------------------ // Simple helper that returns a constant depending on the sign of the argument. I.e. // if the argument is negative, the result is -1; if it's positive, the result is 1; // otherwise, if it's zero, the result is 0. // internal static int Sign(int x) { return x < 0 ? -1 : x == 0 ? 0 : 1; } //----------------------------------------------------------------------------------- // This is a temporary workaround for a VSWhidbey bug 601998 in the X64 JIT compiler. // Unlike the X86 JIT, null checks on value types aren't optimized away in Whidbey. // That means using the GenericComparerinfrastructure results in boxing value // types. This kills performance all over the place. This bug has been fixed in // Orcas (2.0 SP1), so once we're on the SP1 runtime, this can be removed. // internal static Comparer GetDefaultComparer () { if (typeof(TKey) == typeof(int)) { return (Comparer )(object)s_fastIntComparer; } else if (typeof(TKey) == typeof(long)) { return (Comparer )(object)s_fastLongComparer; } else if (typeof(TKey) == typeof(float)) { return (Comparer )(object)s_fastFloatComparer; } else if (typeof(TKey) == typeof(double)) { return (Comparer )(object)s_fastDoubleComparer; } else if (typeof(TKey) == typeof(DateTime)) { return (Comparer )(object)s_fastDateTimeComparer; } return Comparer .Default; } private static FastIntComparer s_fastIntComparer = new FastIntComparer(); class FastIntComparer : Comparer { public override int Compare(int x, int y) { return x.CompareTo(y); } } private static FastLongComparer s_fastLongComparer = new FastLongComparer(); class FastLongComparer : Comparer { public override int Compare(long x, long y) { return x.CompareTo(y); } } private static FastFloatComparer s_fastFloatComparer = new FastFloatComparer(); class FastFloatComparer : Comparer { public override int Compare(float x, float y) { return x.CompareTo(y); } } private static FastDoubleComparer s_fastDoubleComparer = new FastDoubleComparer(); class FastDoubleComparer : Comparer { public override int Compare(double x, double y) { return x.CompareTo(y); } } private static FastDateTimeComparer s_fastDateTimeComparer = new FastDateTimeComparer(); class FastDateTimeComparer : Comparer { public override int Compare(DateTime x, DateTime y) { return x.CompareTo(y); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
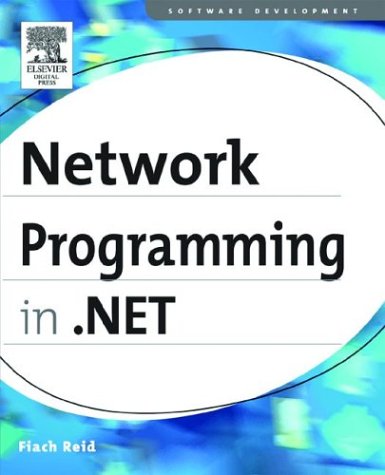
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LogReservationCollection.cs
- BCLDebug.cs
- InvocationExpression.cs
- TypeExtension.cs
- RegexMatchCollection.cs
- ProviderBase.cs
- DataSourceCache.cs
- DocumentViewerHelper.cs
- __Error.cs
- EntityType.cs
- Dynamic.cs
- TransactionContextValidator.cs
- SQLMembershipProvider.cs
- KeyTime.cs
- Point.cs
- Module.cs
- SQLChars.cs
- DeviceFiltersSection.cs
- MulticastNotSupportedException.cs
- NullExtension.cs
- BindingWorker.cs
- PageCatalogPart.cs
- RangeEnumerable.cs
- IisTraceWebEventProvider.cs
- Configuration.cs
- ClientSettingsSection.cs
- ConfigXmlText.cs
- AxImporter.cs
- CodeAttributeArgumentCollection.cs
- Model3DCollection.cs
- ComponentDesigner.cs
- EditorPartChrome.cs
- SqlUserDefinedTypeAttribute.cs
- XmlDictionaryString.cs
- WindowProviderWrapper.cs
- IntranetCredentialPolicy.cs
- ReadingWritingEntityEventArgs.cs
- ProcessStartInfo.cs
- TrustSection.cs
- HtmlShimManager.cs
- Select.cs
- UdpTransportSettings.cs
- DesignerRegionMouseEventArgs.cs
- ContentValidator.cs
- HandlerBase.cs
- AssemblyUtil.cs
- CfgParser.cs
- HtmlImage.cs
- OperatingSystem.cs
- CollectionViewSource.cs
- WinEventHandler.cs
- ConfigurationLocationCollection.cs
- StringStorage.cs
- ProvidersHelper.cs
- HiddenField.cs
- RawStylusSystemGestureInputReport.cs
- TextBlockAutomationPeer.cs
- GridViewUpdateEventArgs.cs
- TreeViewEvent.cs
- IndependentAnimationStorage.cs
- _FixedSizeReader.cs
- RenderContext.cs
- WindowsEditBoxRange.cs
- DiscardableAttribute.cs
- XmlText.cs
- panel.cs
- APCustomTypeDescriptor.cs
- ProxyHelper.cs
- SelfIssuedAuthRSACryptoProvider.cs
- XhtmlBasicObjectListAdapter.cs
- ServiceDescriptionSerializer.cs
- XPathParser.cs
- WebProxyScriptElement.cs
- HtmlImage.cs
- SQLDecimal.cs
- login.cs
- FunctionDefinition.cs
- DataColumnChangeEvent.cs
- CursorConverter.cs
- Help.cs
- XmlTextAttribute.cs
- HMACRIPEMD160.cs
- NamedPermissionSet.cs
- ObjectPropertyMapping.cs
- InteropEnvironment.cs
- QueueProcessor.cs
- WebPartManagerInternals.cs
- ConfigurationConverterBase.cs
- UserPreferenceChangedEventArgs.cs
- BitmapCacheBrush.cs
- GridViewSelectEventArgs.cs
- ConfigurationStrings.cs
- TextTreeTextBlock.cs
- Msec.cs
- WsdlBuildProvider.cs
- DataList.cs
- ClientApiGenerator.cs
- UnmanagedMemoryStream.cs
- JsonStringDataContract.cs
- OdbcReferenceCollection.cs