Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / UI / HtmlControls / HtmlImage.cs / 1 / HtmlImage.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlImage.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.Globalization; using System.Collections; using System.ComponentModel; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ ControlBuilderAttribute(typeof(HtmlEmptyTagControlBuilder)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlImage : HtmlControl { /* * Creates an intrinsic Html IMG control. */ ////// The ////// class defines the methods, properties, and events /// for the HtmlImage server control. /// This class provides programmatic access on the server to /// the HTML <img> element. /// /// public HtmlImage() : base("img") { } /* * Alt property */ ///Initializes a new instance of the ///class. /// [ WebCategory("Appearance"), Localizable(true), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Alt { get { string s = Attributes["alt"]; return((s != null) ? s : String.Empty); } set { Attributes["alt"] = MapStringAttributeToString(value); } } /* * Align property */ ////// Gets or sets the alternative caption that the /// browser displays if image is either unavailable or has not been downloaded yet. /// ////// [ WebCategory("Appearance"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Align { get { string s = Attributes["align"]; return((s != null) ? s : String.Empty); } set { Attributes["align"] = MapStringAttributeToString(value); } } /* * Border property, size of border in pixels. */ ///Gets or sets the alignment of the image with /// surrounding text. ////// [ WebCategory("Appearance"), DefaultValue(0), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Border { get { string s = Attributes["border"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["border"] = MapIntegerAttributeToString(value); } } /* * Height property */ ////// Gets or sets the width of image border, in pixels. /// ////// [ WebCategory("Layout"), DefaultValue(100), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Height { get { string s = Attributes["height"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["height"] = MapIntegerAttributeToString(value); } } /* * Src property. */ ////// Gets or sets /// the height of the image. By default, this is expressed in /// pixels, /// but can be a expressed as a percentage. /// ////// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), UrlProperty() ] public string Src { get { string s = Attributes["src"]; return((s != null) ? s : String.Empty); } set { Attributes["src"] = MapStringAttributeToString(value); } } /* * Width property */ ////// Gets or sets the name of and path to the /// image file to be displayed. This can be an absolute or /// relative path. /// ////// [ WebCategory("Layout"), DefaultValue(100), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Width { get { string s = Attributes["width"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["width"] = MapIntegerAttributeToString(value); } } /* * Override to render unique name attribute. * The name attribute is owned by the framework. */ ////// Gets or sets the width of the image. By default, this is /// expressed in pixels, /// but can be a expressed as a percentage. /// ////// /// protected override void RenderAttributes(HtmlTextWriter writer) { PreProcessRelativeReferenceAttribute(writer, "src"); base.RenderAttributes(writer); writer.Write(" /"); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* * HtmlImage.cs * * Copyright (c) 2000 Microsoft Corporation */ namespace System.Web.UI.HtmlControls { using System; using System.Globalization; using System.Collections; using System.ComponentModel; using System.Web; using System.Web.UI; using System.Security.Permissions; ////// [ ControlBuilderAttribute(typeof(HtmlEmptyTagControlBuilder)) ] [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] [AspNetHostingPermission(SecurityAction.InheritanceDemand, Level=AspNetHostingPermissionLevel.Minimal)] public class HtmlImage : HtmlControl { /* * Creates an intrinsic Html IMG control. */ ////// The ////// class defines the methods, properties, and events /// for the HtmlImage server control. /// This class provides programmatic access on the server to /// the HTML <img> element. /// /// public HtmlImage() : base("img") { } /* * Alt property */ ///Initializes a new instance of the ///class. /// [ WebCategory("Appearance"), Localizable(true), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Alt { get { string s = Attributes["alt"]; return((s != null) ? s : String.Empty); } set { Attributes["alt"] = MapStringAttributeToString(value); } } /* * Align property */ ////// Gets or sets the alternative caption that the /// browser displays if image is either unavailable or has not been downloaded yet. /// ////// [ WebCategory("Appearance"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public string Align { get { string s = Attributes["align"]; return((s != null) ? s : String.Empty); } set { Attributes["align"] = MapStringAttributeToString(value); } } /* * Border property, size of border in pixels. */ ///Gets or sets the alignment of the image with /// surrounding text. ////// [ WebCategory("Appearance"), DefaultValue(0), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Border { get { string s = Attributes["border"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["border"] = MapIntegerAttributeToString(value); } } /* * Height property */ ////// Gets or sets the width of image border, in pixels. /// ////// [ WebCategory("Layout"), DefaultValue(100), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Height { get { string s = Attributes["height"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["height"] = MapIntegerAttributeToString(value); } } /* * Src property. */ ////// Gets or sets /// the height of the image. By default, this is expressed in /// pixels, /// but can be a expressed as a percentage. /// ////// [ WebCategory("Behavior"), DefaultValue(""), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), UrlProperty() ] public string Src { get { string s = Attributes["src"]; return((s != null) ? s : String.Empty); } set { Attributes["src"] = MapStringAttributeToString(value); } } /* * Width property */ ////// Gets or sets the name of and path to the /// image file to be displayed. This can be an absolute or /// relative path. /// ////// [ WebCategory("Layout"), DefaultValue(100), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden) ] public int Width { get { string s = Attributes["width"]; return((s != null) ? Int32.Parse(s, CultureInfo.InvariantCulture) : -1); } set { Attributes["width"] = MapIntegerAttributeToString(value); } } /* * Override to render unique name attribute. * The name attribute is owned by the framework. */ ////// Gets or sets the width of the image. By default, this is /// expressed in pixels, /// but can be a expressed as a percentage. /// ////// /// protected override void RenderAttributes(HtmlTextWriter writer) { PreProcessRelativeReferenceAttribute(writer, "src"); base.RenderAttributes(writer); writer.Write(" /"); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
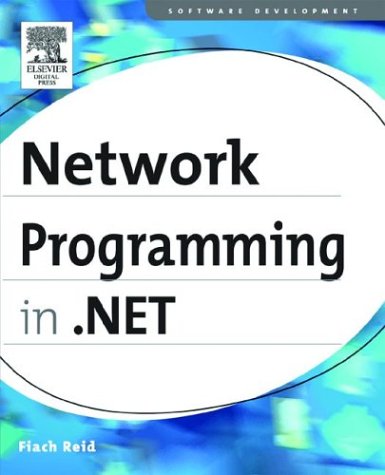
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ClientSideQueueItem.cs
- ValuePattern.cs
- TransformBlockRequest.cs
- RangeValidator.cs
- StylusPointProperty.cs
- FlatButtonAppearance.cs
- PowerModeChangedEventArgs.cs
- WebPartEventArgs.cs
- ServiceParser.cs
- MouseGestureConverter.cs
- DesignerTransactionCloseEvent.cs
- DirectoryObjectSecurity.cs
- Schedule.cs
- SafeRegistryKey.cs
- XamlReader.cs
- FormsAuthenticationUser.cs
- DataServiceRequest.cs
- ItemContainerPattern.cs
- AccessedThroughPropertyAttribute.cs
- TableCellCollection.cs
- X509ThumbprintKeyIdentifierClause.cs
- DocumentViewerConstants.cs
- LexicalChunk.cs
- TextAutomationPeer.cs
- FrameDimension.cs
- SQLInt16Storage.cs
- AnnotationComponentManager.cs
- Imaging.cs
- TcpAppDomainProtocolHandler.cs
- DocobjHost.cs
- CodeMethodReturnStatement.cs
- AutoResizedEvent.cs
- Simplifier.cs
- List.cs
- XmlWrappingReader.cs
- AttributeEmitter.cs
- hebrewshape.cs
- AutomationPeer.cs
- Content.cs
- ArgumentValue.cs
- Blend.cs
- RuntimeConfigLKG.cs
- XmlDocument.cs
- HttpModulesSection.cs
- DescendantBaseQuery.cs
- ToolStripDesignerAvailabilityAttribute.cs
- Currency.cs
- pingexception.cs
- PageRequestManager.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- RankException.cs
- DebuggerAttributes.cs
- CacheModeConverter.cs
- RowToParametersTransformer.cs
- SimpleTypesSurrogate.cs
- ServerReliableChannelBinder.cs
- autovalidator.cs
- PriorityQueue.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- cryptoapiTransform.cs
- Graphics.cs
- InputScopeConverter.cs
- DelegatingConfigHost.cs
- FrameworkElement.cs
- SignedXml.cs
- QuaternionAnimationBase.cs
- DataGridColumnReorderingEventArgs.cs
- XamlStream.cs
- StringFunctions.cs
- EdmValidator.cs
- CodeSubDirectory.cs
- AuthorizationSection.cs
- TreeNodeStyleCollection.cs
- BitmapPalettes.cs
- XXXOnTypeBuilderInstantiation.cs
- ComProxy.cs
- SqlUtils.cs
- PageCatalogPart.cs
- Int32.cs
- IndexedString.cs
- TagMapCollection.cs
- TraceFilter.cs
- BufferManager.cs
- ByteRangeDownloader.cs
- MenuTracker.cs
- OrderedDictionary.cs
- TransformValueSerializer.cs
- SpeechRecognitionEngine.cs
- WebPartTransformer.cs
- EventLogEntry.cs
- GlyphRun.cs
- BeginSelectCardRequest.cs
- FrameAutomationPeer.cs
- AssemblyBuilderData.cs
- Compiler.cs
- DomainUpDown.cs
- PropertyContainer.cs
- CompositeActivityTypeDescriptorProvider.cs
- _SslSessionsCache.cs
- BoolExpr.cs