Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Input / Command / MouseGestureConverter.cs / 1305600 / MouseGestureConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: MouseGestureConverter - Converts a MouseGesture string // to the *Type* that the string represents // // // History: // 05/01/2003 : Chandrasekhar Rentachintala - Created // //--------------------------------------------------------------------------- using System; using System.ComponentModel; // for TypeConverter using System.Globalization; // for CultureInfo using System.Reflection; using MS.Internal; using System.Windows; using System.Windows.Input; using MS.Utility; namespace System.Windows.Input { ////// MouseGesture - Converter class for converting between a string and the Type of a MouseGesture /// public class MouseGestureConverter : TypeConverter { private const char MODIFIERS_DELIMITER = '+' ; ////// CanConvertFrom() /// ///ITypeDescriptorContext ///type to convert from ///true if the given type can be converted, false otherwise public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { // We can only handle string. if (sourceType == typeof(string)) { return true; } else { return false; } } ////// ConvertFrom /// /// Parser Context /// Culture Info /// MouseGesture String ///public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { if (source is string && source != null) { string fullName = ((string)source).Trim(); string mouseActionToken; string modifiersToken; if (fullName == String.Empty) return new MouseGesture(MouseAction.None, ModifierKeys.None); ; // break apart LocalName and Prefix int Offset = fullName.LastIndexOf(MODIFIERS_DELIMITER); if (Offset >= 0) { // modifiers exists modifiersToken = fullName.Substring(0,Offset); mouseActionToken = fullName.Substring(Offset + 1); } else { modifiersToken = String.Empty; mouseActionToken = fullName; } TypeConverter mouseActionConverter = TypeDescriptor.GetConverter(typeof(System.Windows.Input.MouseAction)); if (null != mouseActionConverter ) { object mouseAction = mouseActionConverter.ConvertFrom(context, culture, mouseActionToken); // mouseAction Converter will throw Exception, if it fails, // so we don't need to check once more for bogus // MouseAction values if (mouseAction != null) { if (modifiersToken != String.Empty) { TypeConverter modifierKeysConverter = TypeDescriptor.GetConverter(typeof(System.Windows.Input.ModifierKeys)); if (null != modifierKeysConverter) { object modifierKeys = modifierKeysConverter.ConvertFrom(context, culture, modifiersToken); if (modifierKeys != null && modifierKeys is ModifierKeys) { return new MouseGesture((MouseAction)mouseAction, (ModifierKeys)modifierKeys); } } } else { return new MouseGesture((MouseAction)mouseAction); } } } } throw GetConvertFromException(source); } /// ///TypeConverter method override. /// ///ITypeDescriptorContext ///Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for known type if (context != null && context.Instance != null) { MouseGesture mouseGesture = context.Instance as MouseGesture; if (mouseGesture != null) { return (ModifierKeysConverter.IsDefinedModifierKeys(mouseGesture.Modifiers) && MouseActionConverter.IsDefinedMouseAction(mouseGesture.MouseAction)); } } } return false; } ////// ConvertTo() /// /// Serialization Context /// Culture Info /// MouseGesture value /// Type to Convert ///string if parameter is a MouseGesture public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) throw new ArgumentNullException("destinationType"); if (destinationType == typeof(string)) { if (value == null) return String.Empty; MouseGesture mouseGesture = value as MouseGesture; if (mouseGesture != null) { string strGesture = ""; TypeConverter modifierKeysConverter = TypeDescriptor.GetConverter(typeof(System.Windows.Input.ModifierKeys)); if (null != modifierKeysConverter) { strGesture += modifierKeysConverter.ConvertTo(context, culture, mouseGesture.Modifiers, destinationType) as string; if (strGesture != String.Empty) { strGesture += MODIFIERS_DELIMITER ; } } TypeConverter mouseActionConverter = TypeDescriptor.GetConverter(typeof(System.Windows.Input.MouseAction)); if (null != mouseActionConverter) { strGesture += mouseActionConverter.ConvertTo(context, culture, mouseGesture.MouseAction, destinationType) as string; } return strGesture; } } throw GetConvertToException(value,destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: MouseGestureConverter - Converts a MouseGesture string // to the *Type* that the string represents // // // History: // 05/01/2003 : Chandrasekhar Rentachintala - Created // //--------------------------------------------------------------------------- using System; using System.ComponentModel; // for TypeConverter using System.Globalization; // for CultureInfo using System.Reflection; using MS.Internal; using System.Windows; using System.Windows.Input; using MS.Utility; namespace System.Windows.Input { ////// MouseGesture - Converter class for converting between a string and the Type of a MouseGesture /// public class MouseGestureConverter : TypeConverter { private const char MODIFIERS_DELIMITER = '+' ; ////// CanConvertFrom() /// ///ITypeDescriptorContext ///type to convert from ///true if the given type can be converted, false otherwise public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { // We can only handle string. if (sourceType == typeof(string)) { return true; } else { return false; } } ////// ConvertFrom /// /// Parser Context /// Culture Info /// MouseGesture String ///public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object source) { if (source is string && source != null) { string fullName = ((string)source).Trim(); string mouseActionToken; string modifiersToken; if (fullName == String.Empty) return new MouseGesture(MouseAction.None, ModifierKeys.None); ; // break apart LocalName and Prefix int Offset = fullName.LastIndexOf(MODIFIERS_DELIMITER); if (Offset >= 0) { // modifiers exists modifiersToken = fullName.Substring(0,Offset); mouseActionToken = fullName.Substring(Offset + 1); } else { modifiersToken = String.Empty; mouseActionToken = fullName; } TypeConverter mouseActionConverter = TypeDescriptor.GetConverter(typeof(System.Windows.Input.MouseAction)); if (null != mouseActionConverter ) { object mouseAction = mouseActionConverter.ConvertFrom(context, culture, mouseActionToken); // mouseAction Converter will throw Exception, if it fails, // so we don't need to check once more for bogus // MouseAction values if (mouseAction != null) { if (modifiersToken != String.Empty) { TypeConverter modifierKeysConverter = TypeDescriptor.GetConverter(typeof(System.Windows.Input.ModifierKeys)); if (null != modifierKeysConverter) { object modifierKeys = modifierKeysConverter.ConvertFrom(context, culture, modifiersToken); if (modifierKeys != null && modifierKeys is ModifierKeys) { return new MouseGesture((MouseAction)mouseAction, (ModifierKeys)modifierKeys); } } } else { return new MouseGesture((MouseAction)mouseAction); } } } } throw GetConvertFromException(source); } /// ///TypeConverter method override. /// ///ITypeDescriptorContext ///Type to convert to ///true if conversion is possible public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { // We can convert to an InstanceDescriptor or to a string. if (destinationType == typeof(string)) { // When invoked by the serialization engine we can convert to string only for known type if (context != null && context.Instance != null) { MouseGesture mouseGesture = context.Instance as MouseGesture; if (mouseGesture != null) { return (ModifierKeysConverter.IsDefinedModifierKeys(mouseGesture.Modifiers) && MouseActionConverter.IsDefinedMouseAction(mouseGesture.MouseAction)); } } } return false; } ////// ConvertTo() /// /// Serialization Context /// Culture Info /// MouseGesture value /// Type to Convert ///string if parameter is a MouseGesture public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) throw new ArgumentNullException("destinationType"); if (destinationType == typeof(string)) { if (value == null) return String.Empty; MouseGesture mouseGesture = value as MouseGesture; if (mouseGesture != null) { string strGesture = ""; TypeConverter modifierKeysConverter = TypeDescriptor.GetConverter(typeof(System.Windows.Input.ModifierKeys)); if (null != modifierKeysConverter) { strGesture += modifierKeysConverter.ConvertTo(context, culture, mouseGesture.Modifiers, destinationType) as string; if (strGesture != String.Empty) { strGesture += MODIFIERS_DELIMITER ; } } TypeConverter mouseActionConverter = TypeDescriptor.GetConverter(typeof(System.Windows.Input.MouseAction)); if (null != mouseActionConverter) { strGesture += mouseActionConverter.ConvertTo(context, culture, mouseGesture.MouseAction, destinationType) as string; } return strGesture; } } throw GetConvertToException(value,destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
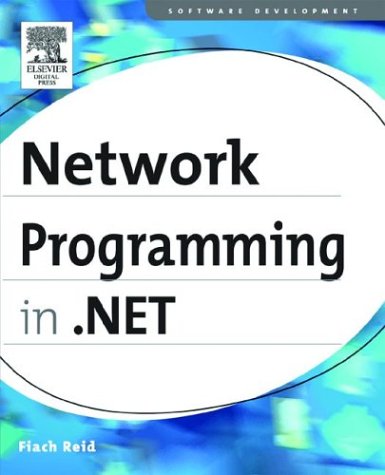
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ellipse.cs
- RijndaelManagedTransform.cs
- FontCacheUtil.cs
- SerializationEventsCache.cs
- RoutedEventArgs.cs
- ResXResourceWriter.cs
- X509Utils.cs
- WasHostedComPlusFactory.cs
- ActivityInstanceReference.cs
- XmlCDATASection.cs
- InlinedAggregationOperator.cs
- BitmapEffectDrawingContextWalker.cs
- RichTextBox.cs
- SystemBrushes.cs
- EventLogEntry.cs
- SymLanguageVendor.cs
- AppDomainFactory.cs
- _emptywebproxy.cs
- RoutedUICommand.cs
- DataView.cs
- SoapFormatExtensions.cs
- _Rfc2616CacheValidators.cs
- ProviderMetadataCachedInformation.cs
- EdmRelationshipRoleAttribute.cs
- SystemTcpConnection.cs
- ConfigXmlAttribute.cs
- XmlHierarchicalEnumerable.cs
- ProfileGroupSettings.cs
- MsmqChannelListenerBase.cs
- FileDialogCustomPlace.cs
- Hash.cs
- SqlBulkCopyColumnMapping.cs
- ResourceReader.cs
- ToolStripItemEventArgs.cs
- DirectionalLight.cs
- SqlUserDefinedTypeAttribute.cs
- RuleElement.cs
- Popup.cs
- TdsParserStateObject.cs
- RectangleGeometry.cs
- SpellCheck.cs
- CookieParameter.cs
- WinFormsUtils.cs
- EnumerableRowCollectionExtensions.cs
- _ProxyChain.cs
- GetLastErrorDetailsRequest.cs
- AnnotationDocumentPaginator.cs
- GridEntry.cs
- MenuItemBindingCollection.cs
- DbMetaDataColumnNames.cs
- ReadonlyMessageFilter.cs
- RoutedUICommand.cs
- LayoutExceptionEventArgs.cs
- MissingMemberException.cs
- RootAction.cs
- DataSourceView.cs
- DocumentOrderComparer.cs
- UniqueTransportManagerRegistration.cs
- MsdtcWrapper.cs
- Size.cs
- HatchBrush.cs
- TagPrefixCollection.cs
- SamlConditions.cs
- EncryptedType.cs
- DependencyPropertyAttribute.cs
- ContainerControl.cs
- SmtpNtlmAuthenticationModule.cs
- DefaultPropertyAttribute.cs
- CopyAttributesAction.cs
- Int32Converter.cs
- unsafeIndexingFilterStream.cs
- CanonicalXml.cs
- MenuItem.cs
- WebEventTraceProvider.cs
- UnsafeNativeMethods.cs
- DataBindingCollectionEditor.cs
- EntityTypeBase.cs
- DescendantOverDescendantQuery.cs
- PtsCache.cs
- DbConnectionPoolOptions.cs
- RelatedCurrencyManager.cs
- MarginsConverter.cs
- DataSourceCacheDurationConverter.cs
- Journal.cs
- DesignOnlyAttribute.cs
- KerberosRequestorSecurityToken.cs
- RefreshInfo.cs
- TextRangeBase.cs
- QueryOptionExpression.cs
- TargetException.cs
- XmlEventCache.cs
- TextAction.cs
- VisemeEventArgs.cs
- SynchronizationContext.cs
- EntityDataSourceState.cs
- XamlPointCollectionSerializer.cs
- UrlAuthorizationModule.cs
- ThrowHelper.cs
- SemaphoreSecurity.cs
- AppDomainManager.cs