Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / RetriableClipboard.cs / 1305376 / RetriableClipboard.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation { using System.Threading; using System.Windows; using System.Windows.Media.Imaging; using System.Runtime.InteropServices; using System.Runtime; using System.Diagnostics; // The clipboard may be accessed by other processes. // RetriableClipboard retries several times before giving up. static class RetriableClipboard { const int retryCount = 10; const int sleepTime = 50; internal static IDataObject GetDataObject() { for (int i = 0; i < retryCount; i++) { try { return Clipboard.GetDataObject(); } catch (Exception err) { Trace.WriteLine(err.ToString()); if (Fx.IsFatal(err)) { throw; } Thread.Sleep(sleepTime); } } return null; } internal static void SetDataObject(object data, bool copy) { for (int i = 0; i < retryCount; i++) { try { Clipboard.SetDataObject(data, copy); return; } catch (Exception err) { Trace.WriteLine(err.ToString()); if (Fx.IsFatal(err)) { throw; } Thread.Sleep(sleepTime); } } } internal static void SetImage(BitmapSource image) { for (int i = 0; i < retryCount; i++) { try { Clipboard.SetImage(image); return; } catch (Exception err) { Trace.WriteLine(err.ToString()); if (Fx.IsFatal(err)) { throw; } Thread.Sleep(sleepTime); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation { using System.Threading; using System.Windows; using System.Windows.Media.Imaging; using System.Runtime.InteropServices; using System.Runtime; using System.Diagnostics; // The clipboard may be accessed by other processes. // RetriableClipboard retries several times before giving up. static class RetriableClipboard { const int retryCount = 10; const int sleepTime = 50; internal static IDataObject GetDataObject() { for (int i = 0; i < retryCount; i++) { try { return Clipboard.GetDataObject(); } catch (Exception err) { Trace.WriteLine(err.ToString()); if (Fx.IsFatal(err)) { throw; } Thread.Sleep(sleepTime); } } return null; } internal static void SetDataObject(object data, bool copy) { for (int i = 0; i < retryCount; i++) { try { Clipboard.SetDataObject(data, copy); return; } catch (Exception err) { Trace.WriteLine(err.ToString()); if (Fx.IsFatal(err)) { throw; } Thread.Sleep(sleepTime); } } } internal static void SetImage(BitmapSource image) { for (int i = 0; i < retryCount; i++) { try { Clipboard.SetImage(image); return; } catch (Exception err) { Trace.WriteLine(err.ToString()); if (Fx.IsFatal(err)) { throw; } Thread.Sleep(sleepTime); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
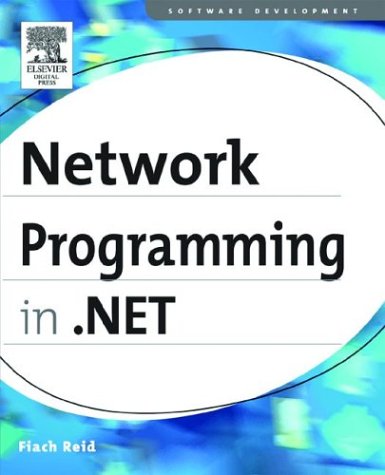
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BasePropertyDescriptor.cs
- TextBlockAutomationPeer.cs
- CopyAction.cs
- MultipartIdentifier.cs
- namescope.cs
- HostSecurityManager.cs
- DefaultMemberAttribute.cs
- WebReferenceCollection.cs
- StyleBamlRecordReader.cs
- DBCSCodePageEncoding.cs
- DriveInfo.cs
- HtmlGenericControl.cs
- RowParagraph.cs
- CharEnumerator.cs
- COM2IProvidePropertyBuilderHandler.cs
- StorageConditionPropertyMapping.cs
- DelimitedListTraceListener.cs
- DtrList.cs
- ExpressionBindings.cs
- CodeArgumentReferenceExpression.cs
- Site.cs
- BitmapEffect.cs
- Char.cs
- SelectionEditingBehavior.cs
- EventProviderWriter.cs
- GeneralTransform2DTo3D.cs
- RelationshipEndCollection.cs
- HostingEnvironmentSection.cs
- ReadOnlyHierarchicalDataSource.cs
- WebPartZoneCollection.cs
- HttpHeaderCollection.cs
- PrePrepareMethodAttribute.cs
- SynchronizationValidator.cs
- HttpEncoderUtility.cs
- OleDbPermission.cs
- NameNode.cs
- ComboBox.cs
- DbParameterCollection.cs
- LightweightCodeGenerator.cs
- SqlRowUpdatedEvent.cs
- StorageInfo.cs
- ObjectViewQueryResultData.cs
- GridViewDeletedEventArgs.cs
- TiffBitmapEncoder.cs
- StateItem.cs
- Resources.Designer.cs
- TimelineGroup.cs
- LogWriteRestartAreaState.cs
- SiteMapNodeCollection.cs
- TrailingSpaceComparer.cs
- NumericExpr.cs
- SqlClientPermission.cs
- PopupRootAutomationPeer.cs
- TransportElement.cs
- SmtpReplyReaderFactory.cs
- RemotingConfiguration.cs
- Compiler.cs
- DataControlPagerLinkButton.cs
- EmptyArray.cs
- XmlWriterTraceListener.cs
- ExceptionValidationRule.cs
- TextPointerBase.cs
- ColorConvertedBitmap.cs
- SamlSerializer.cs
- WindowsAuthenticationEventArgs.cs
- DetailsViewUpdateEventArgs.cs
- ArgumentOutOfRangeException.cs
- HttpFileCollectionWrapper.cs
- XmlDeclaration.cs
- EntityModelSchemaGenerator.cs
- DurableInstance.cs
- DataBindingHandlerAttribute.cs
- FrameSecurityDescriptor.cs
- Atom10FeedFormatter.cs
- SemanticKeyElement.cs
- XamlGridLengthSerializer.cs
- ScaleTransform3D.cs
- UTF32Encoding.cs
- PipelineModuleStepContainer.cs
- ToolStripControlHost.cs
- CodeEventReferenceExpression.cs
- EntityPropertyMappingAttribute.cs
- ProtocolViolationException.cs
- FixedPageStructure.cs
- ReadOnlyDictionary.cs
- XmlAttributeCollection.cs
- EventProxy.cs
- ObjectSerializerFactory.cs
- ParseChildrenAsPropertiesAttribute.cs
- AssemblyAttributesGoHere.cs
- Vector3DIndependentAnimationStorage.cs
- SettingsAttributeDictionary.cs
- ExternalException.cs
- WindowsRebar.cs
- ExceptQueryOperator.cs
- ImageMap.cs
- TwoPhaseCommitProxy.cs
- MetricEntry.cs
- MembershipValidatePasswordEventArgs.cs
- CodeStatementCollection.cs