Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Automation / Peers / ExpanderAutomationPeer.cs / 1305600 / ExpanderAutomationPeer.cs
using System; using System.Collections; using System.Collections.Generic; using System.Runtime.InteropServices; using System.Security; using System.Text; using System.Windows; using System.Windows.Automation.Provider; using System.Windows.Controls; using System.Windows.Controls.Primitives; using System.Windows.Interop; using System.Windows.Media; using MS.Internal; using MS.Win32; namespace System.Windows.Automation.Peers { /// public class ExpanderAutomationPeer : FrameworkElementAutomationPeer, IExpandCollapseProvider { /// public ExpanderAutomationPeer(Expander owner): base(owner) {} /// override protected string GetClassNameCore() { return "Expander"; } /// override protected AutomationControlType GetAutomationControlTypeCore() { return AutomationControlType.Group; } /// override public object GetPattern(PatternInterface pattern) { object iface = null; if(pattern == PatternInterface.ExpandCollapse) { iface = this; } else { iface = base.GetPattern(pattern); } return iface; } #region ExpandCollapse ////// Blocking method that returns after the element has been expanded. /// ///true if the node was successfully expanded void IExpandCollapseProvider.Expand() { if (!IsEnabled()) throw new ElementNotEnabledException(); Expander owner = (Expander)((ExpanderAutomationPeer)this).Owner; owner.IsExpanded = true; } ////// Blocking method that returns after the element has been collapsed. /// ///true if the node was successfully collapsed void IExpandCollapseProvider.Collapse() { if (!IsEnabled()) throw new ElementNotEnabledException(); Expander owner = (Expander)((ExpanderAutomationPeer)this).Owner; owner.IsExpanded = false; } ///indicates an element's current Collapsed or Expanded state ExpandCollapseState IExpandCollapseProvider.ExpandCollapseState { get { Expander owner = (Expander)((ExpanderAutomationPeer)this).Owner; return owner.IsExpanded ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed; } } // [System.Runtime.CompilerServices.MethodImpl(System.Runtime.CompilerServices.MethodImplOptions.NoInlining)] internal void RaiseExpandCollapseAutomationEvent(bool oldValue, bool newValue) { RaisePropertyChangedEvent( ExpandCollapsePatternIdentifiers.ExpandCollapseStateProperty, oldValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed, newValue ? ExpandCollapseState.Expanded : ExpandCollapseState.Collapsed); } #endregion ExpandCollapse } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
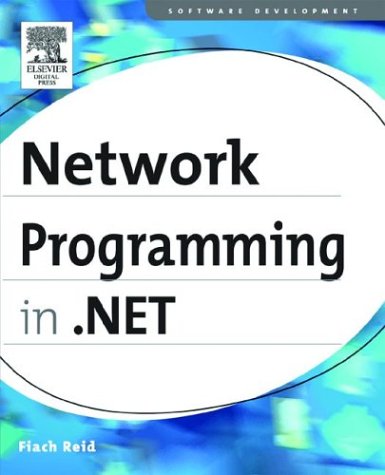
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Pointer.cs
- SchemaAttDef.cs
- SecurityElement.cs
- UTF32Encoding.cs
- HtmlInputText.cs
- ReferenceEqualityComparer.cs
- userdatakeys.cs
- PageHandlerFactory.cs
- GenericsInstances.cs
- Stack.cs
- GridItemProviderWrapper.cs
- DataContractSerializerServiceBehavior.cs
- CopyOnWriteList.cs
- TileBrush.cs
- securitycriticaldata.cs
- ReferencedAssembly.cs
- ScrollProperties.cs
- EntityDataSourceSelectedEventArgs.cs
- CodeMemberMethod.cs
- DesignerProperties.cs
- FontFamilyConverter.cs
- ArraySegment.cs
- CustomValidator.cs
- FailedToStartupUIException.cs
- DeobfuscatingStream.cs
- CheckBoxField.cs
- FileCodeGroup.cs
- RectangleHotSpot.cs
- XNameConverter.cs
- AssemblyBuilder.cs
- indexingfiltermarshaler.cs
- TimeZoneNotFoundException.cs
- ResourceReader.cs
- Vector3dCollection.cs
- XmlWhitespace.cs
- RegexGroupCollection.cs
- VirtualPath.cs
- DateTimeSerializationSection.cs
- DecoderFallbackWithFailureFlag.cs
- BulletedListEventArgs.cs
- TextTreeTextBlock.cs
- ModelItem.cs
- Converter.cs
- CharEnumerator.cs
- RegexCapture.cs
- QilTypeChecker.cs
- CurrentTimeZone.cs
- InputLanguageSource.cs
- ParameterReplacerVisitor.cs
- OraclePermissionAttribute.cs
- COMException.cs
- System.Data.OracleClient_BID.cs
- XPathMultyIterator.cs
- ConversionContext.cs
- OverflowException.cs
- CardSpaceSelector.cs
- XmlSchemaComplexContentExtension.cs
- __Filters.cs
- DesignerActionVerbItem.cs
- AlignmentXValidation.cs
- SessionEndingCancelEventArgs.cs
- DateTimeParse.cs
- PrintingPermission.cs
- SynthesizerStateChangedEventArgs.cs
- SqlFlattener.cs
- wmiprovider.cs
- DataSourceControl.cs
- ProxyGenerationError.cs
- SchemaLookupTable.cs
- RightsManagementProvider.cs
- FlagsAttribute.cs
- StringConverter.cs
- ScriptControlManager.cs
- XmlUtilWriter.cs
- CommandField.cs
- Registry.cs
- JsonWriterDelegator.cs
- DataServiceQuery.cs
- WorkflowServiceBehavior.cs
- ListItemParagraph.cs
- Themes.cs
- SortDescriptionCollection.cs
- SmtpTransport.cs
- CellNormalizer.cs
- LoginCancelEventArgs.cs
- CanonicalFontFamilyReference.cs
- ProgressChangedEventArgs.cs
- Formatter.cs
- HtmlEncodedRawTextWriter.cs
- IsolatedStoragePermission.cs
- SQLRoleProvider.cs
- DataServiceRequest.cs
- ConfigurationCollectionAttribute.cs
- SoapDocumentServiceAttribute.cs
- AnnotationStore.cs
- GregorianCalendar.cs
- ObjectResult.cs
- LogSwitch.cs
- Codec.cs
- SystemIcmpV4Statistics.cs