Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Interaction / Model / ModelItem.cs / 1305376 / ModelItem.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Windows; using System.Collections.Generic; //// The ModelItem class represents a single item in the editing model. An // item can be anything from a Window or Control down to a color or integer. // // You may access the item�s properties through its Properties collection // and make changes to the values of the properties. // // A ModelItem is essentially a wrapper around the designer�s underlying // data model. You can access the underlying model through the // GetCurrentValue method. Note that you should never make any serializable // changes to an object returned from the GetCurrentValue method: it will // not be reflected back in the designer�s serialization or undo systems. // public abstract class ModelItem : INotifyPropertyChanged { //// Creates a new ModelItem. // protected ModelItem() { } //// Implements INotifyPropertyChanged. This can be used to tell when a property // on the model changes. It is also useful because INotifyPropertyChanged can // be used by the data binding features of WPF. // // You should take care to disconnect events from items when you are finished // when them. Otherwise you can prevent the item from garbage collecting. // public abstract event PropertyChangedEventHandler PropertyChanged; //// Returns the attributes declared on this item. // public abstract AttributeCollection Attributes { get; } //// If this item's ItemType declares a ContentPropertyAttribute, // that property will be exposed here. Otherwise, this will // return null. // public abstract ModelProperty Content { get; } //// Returns the type of object the item represents. // public abstract Type ItemType { get; } //// This property represents the name or ID of the item. Not // all items need to have names so this may return null. Also, // depending on the type of item and where it sits in the // hierarchy, it may not always be legal to set the name on // an item. If this item's ItemType declares a // RuntimeNamePropertyAttribute, this Name property will be // a direct mapping to the property dictated by that attribute. // public abstract string Name { get; set; } //// Returns the item that is the parent of this item. // If an item is contained in a collection or dictionary // the collection or dictionary is skipped, returning the // object owns the collection or dictionary. // public abstract ModelItem Parent { get; } //// Returns the al the parents of this item. // public abstract IEnumerableParents { get; } // // Returns the item that is the root of this tree. // If there is no root yet, as this isn't part of a tree // returns null. // public abstract ModelItem Root { get; } //// Returns the public properties on this object. The set of // properties returned may change based on attached // properties or changes to the editing scope. // public abstract ModelPropertyCollection Properties { get; } //// Returns the property that provided this value. If the item represents // the root of the object graph, this will return null. If an item is a // member of a collection or dictionary, the property returned from Source // will be a pseudo-property provided by the collection or dictionary. // For other values, the Source property returns the property where the // value was actually set. Therefore, if a value is being inherited, // Source allows you to find out who originally provided the value. // public abstract ModelProperty Source { get; } //// Returns all the properties that hold this value. // public abstract IEnumerableSources { get; } // // Returns the visual or visual3D representing the UI for this item. This // may return null if there is no view for this object. // public abstract DependencyObject View { get; } //// If you are doing multiple operations on an object or group of objects // you may call BeginEdit. Once an editing scope is open, all changes // across all objects will be saved into the scope. // // Editing scopes are global to the designer. An editing scope may be // created for any item in the designer; you do not need to create an // editing scope for the specific item you are changing. // // Editing scopes can be nested, but must be committed in order. // //// An editing scope that must be either completed or reverted. // public abstract ModelEditingScope BeginEdit(); //// If you are doing multiple operations on an object or group of objects // you may call BeginEdit. Once an editing scope is open, all changes // across all objects will be saved into the scope. // // Editing scopes are global to the designer. An editing scope may be // created for any item in the designer; you do not need to create an // editing scope for the specific item you are changing. // // Editing scopes can be nested, but must be committed in order. // // // An optional description that describes the change. This will be set // into the editing scope�s Description property. // //// An editing scope that must be either completed or reverted. // public abstract ModelEditingScope BeginEdit(string description); //// Returns the current value of the underlying model object the ModelItem // is wrapping. You can inspect this object, but you should not make any // changes to it. Changes made to the object returned will not be incorporated // into the designer. The GetCurrentValue method may return either an existing // or new cloned instance of the object. // //// Returns the current value of the underlying model object the ModelItem is wrapping. // public abstract object GetCurrentValue(); //// Returns string representation of the ModelItem which is the string representation // of underlying object the ModelItem is wrapping. // //// Returns string representation of the ModelItem. // public override string ToString() { object instance = this.GetCurrentValue(); if (instance != null) { return instance.ToString(); } else { return base.ToString(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Windows; using System.Collections.Generic; //// The ModelItem class represents a single item in the editing model. An // item can be anything from a Window or Control down to a color or integer. // // You may access the item�s properties through its Properties collection // and make changes to the values of the properties. // // A ModelItem is essentially a wrapper around the designer�s underlying // data model. You can access the underlying model through the // GetCurrentValue method. Note that you should never make any serializable // changes to an object returned from the GetCurrentValue method: it will // not be reflected back in the designer�s serialization or undo systems. // public abstract class ModelItem : INotifyPropertyChanged { //// Creates a new ModelItem. // protected ModelItem() { } //// Implements INotifyPropertyChanged. This can be used to tell when a property // on the model changes. It is also useful because INotifyPropertyChanged can // be used by the data binding features of WPF. // // You should take care to disconnect events from items when you are finished // when them. Otherwise you can prevent the item from garbage collecting. // public abstract event PropertyChangedEventHandler PropertyChanged; //// Returns the attributes declared on this item. // public abstract AttributeCollection Attributes { get; } //// If this item's ItemType declares a ContentPropertyAttribute, // that property will be exposed here. Otherwise, this will // return null. // public abstract ModelProperty Content { get; } //// Returns the type of object the item represents. // public abstract Type ItemType { get; } //// This property represents the name or ID of the item. Not // all items need to have names so this may return null. Also, // depending on the type of item and where it sits in the // hierarchy, it may not always be legal to set the name on // an item. If this item's ItemType declares a // RuntimeNamePropertyAttribute, this Name property will be // a direct mapping to the property dictated by that attribute. // public abstract string Name { get; set; } //// Returns the item that is the parent of this item. // If an item is contained in a collection or dictionary // the collection or dictionary is skipped, returning the // object owns the collection or dictionary. // public abstract ModelItem Parent { get; } //// Returns the al the parents of this item. // public abstract IEnumerableParents { get; } // // Returns the item that is the root of this tree. // If there is no root yet, as this isn't part of a tree // returns null. // public abstract ModelItem Root { get; } //// Returns the public properties on this object. The set of // properties returned may change based on attached // properties or changes to the editing scope. // public abstract ModelPropertyCollection Properties { get; } //// Returns the property that provided this value. If the item represents // the root of the object graph, this will return null. If an item is a // member of a collection or dictionary, the property returned from Source // will be a pseudo-property provided by the collection or dictionary. // For other values, the Source property returns the property where the // value was actually set. Therefore, if a value is being inherited, // Source allows you to find out who originally provided the value. // public abstract ModelProperty Source { get; } //// Returns all the properties that hold this value. // public abstract IEnumerableSources { get; } // // Returns the visual or visual3D representing the UI for this item. This // may return null if there is no view for this object. // public abstract DependencyObject View { get; } //// If you are doing multiple operations on an object or group of objects // you may call BeginEdit. Once an editing scope is open, all changes // across all objects will be saved into the scope. // // Editing scopes are global to the designer. An editing scope may be // created for any item in the designer; you do not need to create an // editing scope for the specific item you are changing. // // Editing scopes can be nested, but must be committed in order. // //// An editing scope that must be either completed or reverted. // public abstract ModelEditingScope BeginEdit(); //// If you are doing multiple operations on an object or group of objects // you may call BeginEdit. Once an editing scope is open, all changes // across all objects will be saved into the scope. // // Editing scopes are global to the designer. An editing scope may be // created for any item in the designer; you do not need to create an // editing scope for the specific item you are changing. // // Editing scopes can be nested, but must be committed in order. // // // An optional description that describes the change. This will be set // into the editing scope�s Description property. // //// An editing scope that must be either completed or reverted. // public abstract ModelEditingScope BeginEdit(string description); //// Returns the current value of the underlying model object the ModelItem // is wrapping. You can inspect this object, but you should not make any // changes to it. Changes made to the object returned will not be incorporated // into the designer. The GetCurrentValue method may return either an existing // or new cloned instance of the object. // //// Returns the current value of the underlying model object the ModelItem is wrapping. // public abstract object GetCurrentValue(); //// Returns string representation of the ModelItem which is the string representation // of underlying object the ModelItem is wrapping. // //// Returns string representation of the ModelItem. // public override string ToString() { object instance = this.GetCurrentValue(); if (instance != null) { return instance.ToString(); } else { return base.ToString(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
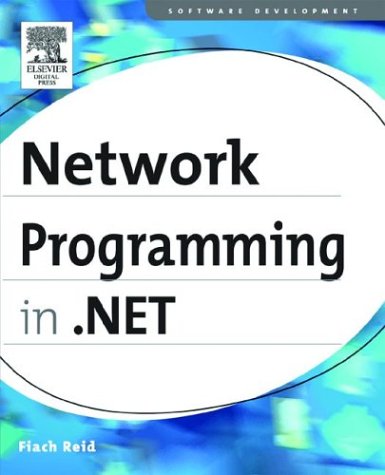
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- InputLanguageCollection.cs
- StylusDownEventArgs.cs
- TextBlock.cs
- OracleCommand.cs
- Encoding.cs
- EarlyBoundInfo.cs
- AsyncCompletedEventArgs.cs
- StructuralObject.cs
- EntitySqlException.cs
- GraphicsPathIterator.cs
- Propagator.cs
- Imaging.cs
- Root.cs
- InternalTransaction.cs
- QualifiedCellIdBoolean.cs
- FixedSOMElement.cs
- cryptoapiTransform.cs
- OracleTimeSpan.cs
- HttpRequestCacheValidator.cs
- Activator.cs
- FastEncoderStatics.cs
- ExtensionQuery.cs
- ExtractCollection.cs
- SafeProcessHandle.cs
- PauseStoryboard.cs
- RayHitTestParameters.cs
- SafeBitVector32.cs
- sqlpipe.cs
- AttributeQuery.cs
- ButtonField.cs
- ReadOnlyHierarchicalDataSource.cs
- Hashtable.cs
- LongValidator.cs
- HandlerWithFactory.cs
- WebPartDisplayModeCancelEventArgs.cs
- AccessDataSource.cs
- AnnotationHighlightLayer.cs
- EntityDataSourceConfigureObjectContext.cs
- MultiSelectRootGridEntry.cs
- Variable.cs
- ConfigurationStrings.cs
- WindowsTokenRoleProvider.cs
- SqlBuilder.cs
- ContentPlaceHolder.cs
- UriSchemeKeyedCollection.cs
- DeploymentSection.cs
- GridViewRow.cs
- AstNode.cs
- AbstractExpressions.cs
- OneOf.cs
- Filter.cs
- srgsitem.cs
- FamilyMapCollection.cs
- HelpKeywordAttribute.cs
- ActiveXHelper.cs
- CodeAttributeArgument.cs
- FieldAccessException.cs
- TableLayoutStyleCollection.cs
- Binding.cs
- SplineQuaternionKeyFrame.cs
- PhoneCallDesigner.cs
- TableCellCollection.cs
- StringDictionaryWithComparer.cs
- Oid.cs
- KeyInfo.cs
- shaperfactory.cs
- ColorPalette.cs
- UTF32Encoding.cs
- ListViewTableRow.cs
- HwndTarget.cs
- LabelAutomationPeer.cs
- SqlNotificationRequest.cs
- ProfileSettingsCollection.cs
- AttributeQuery.cs
- TypeUsageBuilder.cs
- TypeLoadException.cs
- InvalidOperationException.cs
- IndexerNameAttribute.cs
- FloaterParaClient.cs
- PolicyStatement.cs
- EntityCommandDefinition.cs
- WebPartZoneCollection.cs
- GroupQuery.cs
- IntPtr.cs
- MediaPlayerState.cs
- Misc.cs
- X509WindowsSecurityToken.cs
- BorderGapMaskConverter.cs
- CodeDOMProvider.cs
- ScrollPatternIdentifiers.cs
- ValuePattern.cs
- WorkflowInstanceExtensionProvider.cs
- CodeAssignStatement.cs
- BidPrivateBase.cs
- WebPartCollection.cs
- _ProxyRegBlob.cs
- GridLength.cs
- RegexWriter.cs
- ActivityDesigner.cs
- DetailsViewUpdateEventArgs.cs