Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Framework / System / Windows / Controls / BorderGapMaskConverter.cs / 1 / BorderGapMaskConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Used to create a Gap in the Border for GroupBox style // //--------------------------------------------------------------------------- using System.Globalization; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Media; using System.Windows.Shapes; namespace System.Windows.Controls { ////// BorderGapMaskConverter class /// public class BorderGapMaskConverter : IMultiValueConverter { ////// Convert a value. /// /// values as produced by source binding /// target type /// converter parameter /// culture information ////// Converted value. /// Visual Brush that is used as the opacity mask for the Border /// in the style for GroupBox. /// public object Convert(object[] values, Type targetType, object parameter, CultureInfo culture) { // // Parameter Validation // Type doubleType = typeof(double); if (parameter == null || values == null || values.Length != 3 || values[0] == null || values[1] == null || values[2] == null || !doubleType.IsAssignableFrom(values[0].GetType()) || !doubleType.IsAssignableFrom(values[1].GetType()) || !doubleType.IsAssignableFrom(values[2].GetType()) ) { return DependencyProperty.UnsetValue; } Type paramType = parameter.GetType(); if (!(doubleType.IsAssignableFrom(paramType) || typeof(string).IsAssignableFrom(paramType))) { return DependencyProperty.UnsetValue; } // // Conversion // double headerWidth = (double)values[0]; double borderWidth = (double)values[1]; double borderHeight = (double)values[2]; // Doesn't make sense to have a Grid // with 0 as width or height if (borderWidth == 0 || borderHeight == 0) { return null; } // Width of the line to the left of the header // to be used to set the width of the first column of the Grid double lineWidth; if (parameter is string) { lineWidth = Double.Parse(((string)parameter), NumberFormatInfo.InvariantInfo); } else { lineWidth = (double)parameter; } Grid grid = new Grid(); grid.Width = borderWidth; grid.Height = borderHeight; ColumnDefinition colDef1 = new ColumnDefinition(); ColumnDefinition colDef2 = new ColumnDefinition(); ColumnDefinition colDef3 = new ColumnDefinition(); colDef1.Width = new GridLength(lineWidth); colDef2.Width = new GridLength(headerWidth); colDef3.Width = new GridLength(1, GridUnitType.Star); grid.ColumnDefinitions.Add(colDef1); grid.ColumnDefinitions.Add(colDef2); grid.ColumnDefinitions.Add(colDef3); RowDefinition rowDef1 = new RowDefinition(); RowDefinition rowDef2 = new RowDefinition(); rowDef1.Height = new GridLength(borderHeight / 2); rowDef2.Height = new GridLength(1, GridUnitType.Star); grid.RowDefinitions.Add(rowDef1); grid.RowDefinitions.Add(rowDef2); Rectangle rectColumn1 = new Rectangle(); Rectangle rectColumn2 = new Rectangle(); Rectangle rectColumn3 = new Rectangle(); rectColumn1.Fill = Brushes.Black; rectColumn2.Fill = Brushes.Black; rectColumn3.Fill = Brushes.Black; Grid.SetRowSpan(rectColumn1, 2); Grid.SetRow(rectColumn1, 0); Grid.SetColumn(rectColumn1, 0); Grid.SetRow(rectColumn2, 1); Grid.SetColumn(rectColumn2, 1); Grid.SetRowSpan(rectColumn3, 2); Grid.SetRow(rectColumn3, 0); Grid.SetColumn(rectColumn3, 2); grid.Children.Add(rectColumn1); grid.Children.Add(rectColumn2); grid.Children.Add(rectColumn3); return (new VisualBrush(grid)); } ////// Not Supported /// /// value, as produced by target /// target types /// converter parameter /// culture information ///Nothing public object[] ConvertBack(object value, Type[] targetTypes, object parameter, CultureInfo culture) { return new object[] { Binding.DoNothing }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
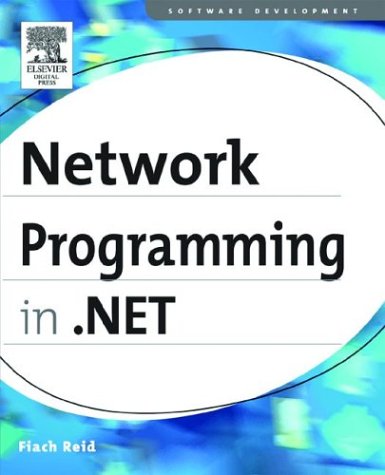
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MimeTypeMapper.cs
- ReferentialConstraint.cs
- Dictionary.cs
- InkCanvasInnerCanvas.cs
- WebPartDisplayModeEventArgs.cs
- UriSectionData.cs
- RectangleGeometry.cs
- StylusTouchDevice.cs
- ParentUndoUnit.cs
- cookie.cs
- DrawingImage.cs
- PerformanceCounterPermissionEntryCollection.cs
- PageTheme.cs
- FlowLayoutPanel.cs
- ExecutionContext.cs
- SchemaAttDef.cs
- DesignerLoader.cs
- ButtonRenderer.cs
- BindingMAnagerBase.cs
- WindowsFormsHostAutomationPeer.cs
- EdgeModeValidation.cs
- CommandConverter.cs
- AttributeQuery.cs
- ExpressionBuilder.cs
- OleDbException.cs
- StorageBasedPackageProperties.cs
- Tracer.cs
- BindingCompleteEventArgs.cs
- Quaternion.cs
- MessageEncodingBindingElementImporter.cs
- FirewallWrapper.cs
- WinHttpWebProxyFinder.cs
- StickyNoteHelper.cs
- AutomationProperty.cs
- DropDownList.cs
- WindowsGraphics2.cs
- ExtensionElement.cs
- VectorKeyFrameCollection.cs
- CreateUserWizard.cs
- ScrollChangedEventArgs.cs
- ProxyFragment.cs
- NullRuntimeConfig.cs
- DtrList.cs
- DetailsViewPagerRow.cs
- InertiaTranslationBehavior.cs
- Lease.cs
- safePerfProviderHandle.cs
- NodeFunctions.cs
- TextParaLineResult.cs
- CopyEncoder.cs
- Rotation3DAnimationUsingKeyFrames.cs
- FixedTextContainer.cs
- XmlSchemaAny.cs
- Preprocessor.cs
- ILGen.cs
- XPathNavigatorKeyComparer.cs
- LocatorPartList.cs
- CodeExpressionRuleDeclaration.cs
- DynamicUpdateCommand.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- RelationshipManager.cs
- PolicyStatement.cs
- PersonalizationDictionary.cs
- PenContext.cs
- ScaleTransform3D.cs
- TypeUsage.cs
- ObjectResult.cs
- CommandBindingCollection.cs
- SemanticResolver.cs
- DefaultDialogButtons.cs
- SaveFileDialogDesigner.cs
- FtpWebRequest.cs
- CompiledIdentityConstraint.cs
- UInt64Converter.cs
- ContentTypeSettingClientMessageFormatter.cs
- CodeGroup.cs
- RegexWorker.cs
- NonBatchDirectoryCompiler.cs
- TimelineClockCollection.cs
- InputProcessorProfilesLoader.cs
- ScrollPattern.cs
- DesignerUtils.cs
- CompModSwitches.cs
- TextRunProperties.cs
- BezierSegment.cs
- WeakReference.cs
- precedingsibling.cs
- RankException.cs
- SmtpLoginAuthenticationModule.cs
- RenamedEventArgs.cs
- Token.cs
- RequestCachingSection.cs
- HtmlTable.cs
- DesignerCalendarAdapter.cs
- SpecialFolderEnumConverter.cs
- RequestQueue.cs
- RegexTree.cs
- Point.cs
- ClientScriptManagerWrapper.cs
- DesignerAdapterUtil.cs