Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / MS / Internal / Ink / HighContrastHelper.cs / 1 / HighContrastHelper.cs
//---------------------------------------------------------------------------- // // File: HighContrastHelper.cs // // Description: // A helper class for tracking the change of the system high contrast setting. // // Features: // // History: // 06/15/2005 waynezen: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Media; using System.Windows.Threading; namespace MS.Internal.Ink { ////// HighContrastCallback Classs - An abstract helper class /// internal abstract class HighContrastCallback { ////// TurnHighContrastOn /// /// internal abstract void TurnHighContrastOn(Color highContrastColor); ////// TurnHighContrastOff /// internal abstract void TurnHighContrastOff(); ////// Returns the dispatcher if the object is associated to a UIContext. /// internal abstract Dispatcher Dispatcher { get; } } ////// StylusEditingBehavior - a base class for all stylus related editing behaviors /// internal static class HighContrastHelper { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors ////// Constructor /// static HighContrastHelper() { __highContrastCallbackList = new List(); __increaseCount = 0; } #endregion Constructors //-------------------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------------------- #region Internal Methods /// /// Register the weak references for HighContrastCallback /// /// internal static void RegisterHighContrastCallback(HighContrastCallback highContrastCallback) { lock ( __lock ) { int count = __highContrastCallbackList.Count; int i = 0; int j = 0; // Every 100 items, We go through the list to remove the references // which have been collected by GC. if ( __increaseCount > CleanTolerance ) { while ( i < count ) { WeakReference weakRef = __highContrastCallbackList[j]; if ( weakRef.IsAlive ) { j++; } else { // Remove the unavaliable reference from the list __highContrastCallbackList.RemoveAt(j); } i++; } // Reset the count __increaseCount = 0; } __highContrastCallbackList.Add(new WeakReference(highContrastCallback)); __increaseCount++; } } ////// The method is called from SystemResources.SystemThemeFilterMessage /// internal static void OnSettingChanged() { UpdateHighContrast(); } #endregion Internal Methods //-------------------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------------------- #region Private Methods ////// UpdateHighContrast which calls out all the registered callbacks. /// private static void UpdateHighContrast() { lock ( __lock ) { int count = __highContrastCallbackList.Count; int i = 0; int j = 0; // Now go through the list, // And we will notify the alive callbacks // or remove the references which have been collected by GC. while ( i < count ) { WeakReference weakRef = __highContrastCallbackList[j]; if ( weakRef.IsAlive ) { HighContrastCallback highContrastCallback = weakRef.Target as HighContrastCallback; if ( highContrastCallback.Dispatcher != null ) { highContrastCallback.Dispatcher.BeginInvoke(DispatcherPriority.Background, new UpdateHighContrastCallback(OnUpdateHighContrast), highContrastCallback); } else { OnUpdateHighContrast(highContrastCallback); } j++; } else { // Remove the dead ones __highContrastCallbackList.RemoveAt(j); } i++; } // Reset the count __increaseCount = 0; } } private delegate void UpdateHighContrastCallback(HighContrastCallback highContrastCallback); ////// Invoke the callback /// /// private static void OnUpdateHighContrast(HighContrastCallback highContrastCallback) { // Get the current setting. bool isHighContrast = SystemParameters.HighContrast; Color windowTextColor = SystemColors.WindowTextColor; if ( isHighContrast ) { highContrastCallback.TurnHighContrastOn(windowTextColor); } else { highContrastCallback.TurnHighContrastOff(); } } #endregion Private Methods //------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields private static object __lock = new object(); private static List__highContrastCallbackList; private static int __increaseCount; private const int CleanTolerance = 100; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: HighContrastHelper.cs // // Description: // A helper class for tracking the change of the system high contrast setting. // // Features: // // History: // 06/15/2005 waynezen: Created // // Copyright (C) 2001 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Diagnostics; using System.Security; using System.Security.Permissions; using System.Windows; using System.Windows.Media; using System.Windows.Threading; namespace MS.Internal.Ink { /// /// HighContrastCallback Classs - An abstract helper class /// internal abstract class HighContrastCallback { ////// TurnHighContrastOn /// /// internal abstract void TurnHighContrastOn(Color highContrastColor); ////// TurnHighContrastOff /// internal abstract void TurnHighContrastOff(); ////// Returns the dispatcher if the object is associated to a UIContext. /// internal abstract Dispatcher Dispatcher { get; } } ////// StylusEditingBehavior - a base class for all stylus related editing behaviors /// internal static class HighContrastHelper { //------------------------------------------------------------------------------- // // Constructors // //------------------------------------------------------------------------------- #region Constructors ////// Constructor /// static HighContrastHelper() { __highContrastCallbackList = new List(); __increaseCount = 0; } #endregion Constructors //-------------------------------------------------------------------------------- // // Internal Methods // //------------------------------------------------------------------------------- #region Internal Methods /// /// Register the weak references for HighContrastCallback /// /// internal static void RegisterHighContrastCallback(HighContrastCallback highContrastCallback) { lock ( __lock ) { int count = __highContrastCallbackList.Count; int i = 0; int j = 0; // Every 100 items, We go through the list to remove the references // which have been collected by GC. if ( __increaseCount > CleanTolerance ) { while ( i < count ) { WeakReference weakRef = __highContrastCallbackList[j]; if ( weakRef.IsAlive ) { j++; } else { // Remove the unavaliable reference from the list __highContrastCallbackList.RemoveAt(j); } i++; } // Reset the count __increaseCount = 0; } __highContrastCallbackList.Add(new WeakReference(highContrastCallback)); __increaseCount++; } } ////// The method is called from SystemResources.SystemThemeFilterMessage /// internal static void OnSettingChanged() { UpdateHighContrast(); } #endregion Internal Methods //-------------------------------------------------------------------------------- // // Private Methods // //-------------------------------------------------------------------------------- #region Private Methods ////// UpdateHighContrast which calls out all the registered callbacks. /// private static void UpdateHighContrast() { lock ( __lock ) { int count = __highContrastCallbackList.Count; int i = 0; int j = 0; // Now go through the list, // And we will notify the alive callbacks // or remove the references which have been collected by GC. while ( i < count ) { WeakReference weakRef = __highContrastCallbackList[j]; if ( weakRef.IsAlive ) { HighContrastCallback highContrastCallback = weakRef.Target as HighContrastCallback; if ( highContrastCallback.Dispatcher != null ) { highContrastCallback.Dispatcher.BeginInvoke(DispatcherPriority.Background, new UpdateHighContrastCallback(OnUpdateHighContrast), highContrastCallback); } else { OnUpdateHighContrast(highContrastCallback); } j++; } else { // Remove the dead ones __highContrastCallbackList.RemoveAt(j); } i++; } // Reset the count __increaseCount = 0; } } private delegate void UpdateHighContrastCallback(HighContrastCallback highContrastCallback); ////// Invoke the callback /// /// private static void OnUpdateHighContrast(HighContrastCallback highContrastCallback) { // Get the current setting. bool isHighContrast = SystemParameters.HighContrast; Color windowTextColor = SystemColors.WindowTextColor; if ( isHighContrast ) { highContrastCallback.TurnHighContrastOn(windowTextColor); } else { highContrastCallback.TurnHighContrastOff(); } } #endregion Private Methods //------------------------------------------------------------------------------- // // Private Fields // //-------------------------------------------------------------------------------- #region Private Fields private static object __lock = new object(); private static List__highContrastCallbackList; private static int __increaseCount; private const int CleanTolerance = 100; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
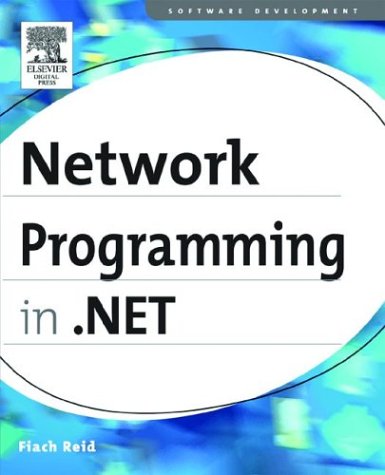
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataTableExtensions.cs
- DeferredTextReference.cs
- TextServicesPropertyRanges.cs
- BitmapMetadataEnumerator.cs
- graph.cs
- HttpRequestTraceRecord.cs
- ComponentResourceKeyConverter.cs
- ProxyHelper.cs
- DocumentPage.cs
- WmlValidationSummaryAdapter.cs
- Typography.cs
- MultiView.cs
- StaticExtensionConverter.cs
- ReachObjectContext.cs
- DbConnectionStringBuilder.cs
- BoundsDrawingContextWalker.cs
- BinaryObjectInfo.cs
- AbstractExpressions.cs
- NumericUpDownAcceleration.cs
- mediaclock.cs
- LineProperties.cs
- ServerValidateEventArgs.cs
- SEHException.cs
- TableRow.cs
- TdsParserStaticMethods.cs
- Stopwatch.cs
- CurrencyManager.cs
- ObjectDataSourceStatusEventArgs.cs
- EventMappingSettings.cs
- connectionpool.cs
- CommentAction.cs
- HttpCookieCollection.cs
- SmtpClient.cs
- MiniParameterInfo.cs
- EmitterCache.cs
- PackageFilter.cs
- Soap.cs
- Sql8ExpressionRewriter.cs
- TextTrailingCharacterEllipsis.cs
- HttpCapabilitiesEvaluator.cs
- FlowLayoutPanel.cs
- Int16AnimationBase.cs
- CategoryList.cs
- KeyboardInputProviderAcquireFocusEventArgs.cs
- LoadMessageLogger.cs
- CachedFontFamily.cs
- DetailsViewUpdateEventArgs.cs
- DBCSCodePageEncoding.cs
- ECDiffieHellmanCngPublicKey.cs
- OlePropertyStructs.cs
- DataGridViewColumnHeaderCell.cs
- PropertyInformationCollection.cs
- Geometry3D.cs
- FaultContractInfo.cs
- DataRecordInternal.cs
- XComponentModel.cs
- GZipStream.cs
- ManagedIStream.cs
- InstalledFontCollection.cs
- DataBindingExpressionBuilder.cs
- DeflateStream.cs
- ToolboxDataAttribute.cs
- ServiceProviders.cs
- Image.cs
- ContainerControl.cs
- DecoratedNameAttribute.cs
- TransformCollection.cs
- GroupQuery.cs
- SelectionProcessor.cs
- MimeMultiPart.cs
- WebPartDescriptionCollection.cs
- HttpRequestWrapper.cs
- Normalization.cs
- GuidelineCollection.cs
- SortedList.cs
- ImpersonateTokenRef.cs
- DrawingContext.cs
- SqlRowUpdatingEvent.cs
- ConstraintManager.cs
- ExpressionConverter.cs
- WindowsListViewGroupHelper.cs
- DeferredTextReference.cs
- InstallerTypeAttribute.cs
- ColumnClickEvent.cs
- SegmentInfo.cs
- FormClosedEvent.cs
- StylusPointPropertyUnit.cs
- Privilege.cs
- TextBoxAutomationPeer.cs
- SplitterEvent.cs
- GridViewColumnCollectionChangedEventArgs.cs
- MappableObjectManager.cs
- CodeDefaultValueExpression.cs
- TypeUnloadedException.cs
- VectorValueSerializer.cs
- SqlProviderManifest.cs
- Compilation.cs
- SHA256.cs
- AutomationElementCollection.cs
- InspectionWorker.cs