Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Sys / System / IO / compression / GZipStream.cs / 1 / GZipStream.cs
namespace System.IO.Compression { using System.IO; using System.Diagnostics; using System.Security.Permissions; public class GZipStream : Stream { private DeflateStream deflateStream; public GZipStream(Stream stream, CompressionMode mode) : this( stream, mode, false) { } public GZipStream(Stream stream, CompressionMode mode, bool leaveOpen) { deflateStream = new DeflateStream(stream, mode, leaveOpen, true); } public override bool CanRead { get { if( deflateStream == null) { return false; } return deflateStream.CanRead; } } public override bool CanWrite { get { if( deflateStream == null) { return false; } return deflateStream.CanWrite; } } public override bool CanSeek { get { if( deflateStream == null) { return false; } return deflateStream.CanSeek; } } public override long Length { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override long Position { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } set { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override void Flush() { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Flush(); return; } public override long Seek(long offset, SeekOrigin origin) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } public override void SetLength(long value) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginRead(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginRead(array, offset, count, asyncCallback, asyncState); } public override int EndRead(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.EndRead(asyncResult); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginWrite(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginWrite(array, offset, count, asyncCallback, asyncState); } public override void EndWrite(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.EndWrite(asyncResult); } public override int Read(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.Read(array, offset, count); } public override void Write(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Write(array, offset, count); } protected override void Dispose(bool disposing) { try { if (disposing && deflateStream != null) { deflateStream.Close(); } deflateStream = null; } finally { base.Dispose(disposing); } } public Stream BaseStream { get { if( deflateStream != null) { return deflateStream.BaseStream; } else { return null; } } } } }
Link Menu
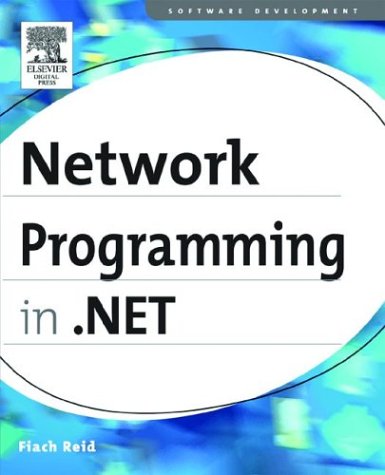
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ZipIOLocalFileDataDescriptor.cs
- CodeBinaryOperatorExpression.cs
- ListControlDesigner.cs
- ObjectDataSourceEventArgs.cs
- ReadOnlyDictionary.cs
- LinqDataSourceSelectEventArgs.cs
- HttpTransportSecurity.cs
- Marshal.cs
- SendOperation.cs
- AnnouncementClient.cs
- FacetChecker.cs
- BaseInfoTable.cs
- FrugalList.cs
- HtmlTernaryTree.cs
- DictionaryEditChange.cs
- WebBrowserBase.cs
- TextBlockAutomationPeer.cs
- XmlDownloadManager.cs
- LayoutInformation.cs
- PenCursorManager.cs
- TableCellAutomationPeer.cs
- HtmlFormWrapper.cs
- BitVector32.cs
- CodeDomSerializerBase.cs
- HandlerMappingMemo.cs
- WebException.cs
- IIS7UserPrincipal.cs
- ReadOnlyDataSourceView.cs
- NoResizeHandleGlyph.cs
- SystemException.cs
- XmlAnyElementAttributes.cs
- CqlQuery.cs
- RIPEMD160.cs
- ServiceX509SecurityTokenProvider.cs
- OdbcTransaction.cs
- HebrewCalendar.cs
- RectAnimationUsingKeyFrames.cs
- WCFServiceClientProxyGenerator.cs
- DataServiceEntityAttribute.cs
- CommandDevice.cs
- ExpressionHelper.cs
- EncodingTable.cs
- GenericIdentity.cs
- EntityModelBuildProvider.cs
- DoubleMinMaxAggregationOperator.cs
- DocumentViewerAutomationPeer.cs
- DataPagerField.cs
- XmlAttributeAttribute.cs
- IQueryable.cs
- StylusButtonCollection.cs
- FixedSchema.cs
- PerformanceCountersElement.cs
- DbCommandDefinition.cs
- XPathNavigator.cs
- LogoValidationException.cs
- MergeExecutor.cs
- ComboBox.cs
- PropertyPushdownHelper.cs
- RawKeyboardInputReport.cs
- PathSegment.cs
- Int32Converter.cs
- PasswordBoxAutomationPeer.cs
- ReadOnlyHierarchicalDataSourceView.cs
- SessionStateModule.cs
- TypeConverterAttribute.cs
- PackWebRequestFactory.cs
- FormViewInsertEventArgs.cs
- DocumentPage.cs
- CultureSpecificStringDictionary.cs
- HostingEnvironmentSection.cs
- CqlQuery.cs
- InvariantComparer.cs
- Propagator.cs
- SharedDp.cs
- ProofTokenCryptoHandle.cs
- Processor.cs
- IntSecurity.cs
- Method.cs
- PrintPreviewGraphics.cs
- ValidatorCollection.cs
- OdbcStatementHandle.cs
- TranslateTransform3D.cs
- ResolveNameEventArgs.cs
- precedingsibling.cs
- ResourceDisplayNameAttribute.cs
- CommonServiceBehaviorElement.cs
- HttpStreamXmlDictionaryReader.cs
- ImageInfo.cs
- ServiceConfigurationTraceRecord.cs
- TokenBasedSet.cs
- ZeroOpNode.cs
- DataGridItem.cs
- CatchDesigner.xaml.cs
- FilterEventArgs.cs
- SeverityFilter.cs
- XmlIterators.cs
- RTTrackingProfile.cs
- SharedStatics.cs
- Roles.cs
- XmlSerializationReader.cs