Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Objects / Internal / EntityWithChangeTrackerStrategy.cs / 1305376 / EntityWithChangeTrackerStrategy.cs
using System; using System.Collections.Generic; using System.Text; using System.Data.Objects.DataClasses; using System.Diagnostics; namespace System.Data.Objects.Internal { ////// Implementation of the change tracking strategy for entities that support change trackers. /// These are typically entities that implement IEntityWithChangeTracker. /// internal sealed class EntityWithChangeTrackerStrategy : IChangeTrackingStrategy { private IEntityWithChangeTracker _entity; ////// Constructs a strategy object that will cause the change tracker to be set onto the /// given object. /// /// The object onto which a change tracker will be set public EntityWithChangeTrackerStrategy(IEntityWithChangeTracker entity) { _entity = entity; } // See IChangeTrackingStrategy documentation public void SetChangeTracker(IEntityChangeTracker changeTracker) { _entity.SetChangeTracker(changeTracker); } // See IChangeTrackingStrategy documentation public void TakeSnapshot(EntityEntry entry) { if (entry != null && entry.WrappedEntity.RequiresComplexChangeTracking) { entry.TakeSnapshot(true); } } // See IChangeTrackingStrategy documentation public void SetCurrentValue(EntityEntry entry, StateManagerMemberMetadata member, int ordinal, object target, object value) { member.SetValue(target, value); } // See IChangeTrackingStrategy documentation public void UpdateCurrentValueRecord(object value, EntityEntry entry) { // Has change tracker, but may or may not be a proxy bool isProxy = entry.WrappedEntity.IdentityType != _entity.GetType(); entry.UpdateRecordWithoutSetModified(value, entry.CurrentValues); if (isProxy) { entry.DetectChangesInProperties(true); // detect only complex property changes } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
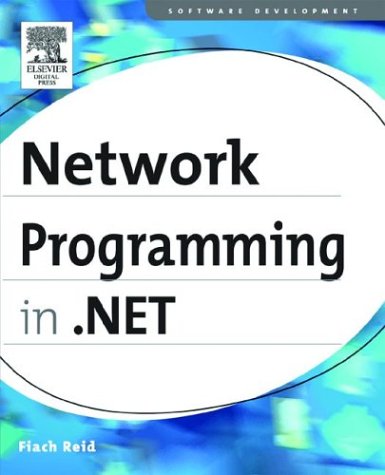
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HybridDictionary.cs
- UserNamePasswordValidator.cs
- SafeEventLogReadHandle.cs
- TreeNodeClickEventArgs.cs
- ToolStripHighContrastRenderer.cs
- StylusEventArgs.cs
- TextRangeEditTables.cs
- EnumValAlphaComparer.cs
- ImageAnimator.cs
- DataServiceRequestException.cs
- DropDownButton.cs
- odbcmetadatacolumnnames.cs
- InputLanguageManager.cs
- WebPartEditorOkVerb.cs
- AnchorEditor.cs
- TextDecoration.cs
- TransactionScope.cs
- ExpressionBuilderContext.cs
- DelegateSerializationHolder.cs
- ApplicationDirectoryMembershipCondition.cs
- Compiler.cs
- DataGridSortCommandEventArgs.cs
- UnsafeNativeMethods.cs
- ExpressionBuilder.cs
- Control.cs
- MetadataCacheItem.cs
- MediaCommands.cs
- ListViewItem.cs
- Classification.cs
- WindowsPen.cs
- DataBoundControlAdapter.cs
- DbModificationClause.cs
- Attributes.cs
- ViewLoader.cs
- TimeSpanSecondsOrInfiniteConverter.cs
- ProfessionalColors.cs
- Queue.cs
- ControlValuePropertyAttribute.cs
- ResXDataNode.cs
- ScriptingWebServicesSectionGroup.cs
- MemberPath.cs
- PropertySet.cs
- CfgParser.cs
- FormsAuthentication.cs
- MessageBox.cs
- CatalogZone.cs
- TdsValueSetter.cs
- KeyGesture.cs
- PopOutPanel.cs
- DictionaryKeyPropertyAttribute.cs
- GeneralTransform.cs
- BasicCommandTreeVisitor.cs
- CodeDirectionExpression.cs
- ConsumerConnectionPointCollection.cs
- TTSEngineProxy.cs
- OdbcEnvironmentHandle.cs
- Domain.cs
- XmlSchemaAttributeGroupRef.cs
- sqlinternaltransaction.cs
- StateManagedCollection.cs
- Rule.cs
- SafeSecurityHandles.cs
- SqlGenericUtil.cs
- TypefaceMap.cs
- FrameworkElement.cs
- PowerStatus.cs
- AppDomainGrammarProxy.cs
- LocationSectionRecord.cs
- XmlIncludeAttribute.cs
- ThemeableAttribute.cs
- PerformanceCounter.cs
- GorillaCodec.cs
- PeerOutputChannel.cs
- EventSourceCreationData.cs
- StickyNoteContentControl.cs
- XpsFixedDocumentSequenceReaderWriter.cs
- SymbolType.cs
- ReadOnlyTernaryTree.cs
- ListenerConstants.cs
- ButtonField.cs
- WebBrowsableAttribute.cs
- HandlerBase.cs
- WindowsStatic.cs
- EncryptedData.cs
- Native.cs
- SpecialNameAttribute.cs
- SystemResourceHost.cs
- XPathNodeIterator.cs
- _IPv6Address.cs
- ContractReference.cs
- HttpBrowserCapabilitiesBase.cs
- SafeEventLogReadHandle.cs
- SiteMembershipCondition.cs
- DefaultAssemblyResolver.cs
- IChannel.cs
- DataBoundLiteralControl.cs
- HwndSourceParameters.cs
- Size.cs
- MessageAction.cs
- SmtpMail.cs