Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Caching / MetadataCacheItem.cs / 1305376 / MetadataCacheItem.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class to cache metadata information. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Caching { using System; using System.Collections.Generic; using System.Data.Services.Common; using System.Data.Services.Providers; using System.Diagnostics; ///Use this class to cache metadata for providers. internal class MetadataCacheItem { #region Private fields. ///list of top level entity sets private readonly DictionaryentitySets; /// Collection of service operations, keyed by name. private readonly DictionaryserviceOperations; /// Target type for the data provider. private readonly Type type; ///Cache of resource properties per type. private readonly DictionarytypeCache; /// Cache of immediate derived types per type. private readonly Dictionary> childTypesCache; /// Service configuration information. private DataServiceConfiguration configuration; ///Whether all EPM properties serialize in an Astoria V1-compatible way. private bool epmIsV1Compatible; ////// Keep track of the calculated visibility of resource types. /// private DictionaryvisibleTypeCache; /// /// Maps resource set names to ResourceSetWrappers. /// private DictionaryresourceSetWrapperCache; /// /// Maps service operation names to ServiceOperationWrappers. /// private DictionaryserviceOperationWrapperCache; /// /// Maps names to ResourceAssociationSets. /// private DictionaryresourceAssociationSetCache; /// /// Mapes "resourceSetName_resourceTypeName" to the list of visible properties from the set. /// private Dictionary> resourcePropertyCache; /// /// Mapes "resourceSetName_resourceTypeName" to boolean of whether resourceType is allowed for resourceSet /// private DictionaryentityTypeDisallowedForSet; #endregion Private fields. /// Initializes a new /// Type of data context for which metadata will be generated. internal MetadataCacheItem(Type type) { Debug.Assert(type != null, "type != null"); this.serviceOperations = new Dictionaryinstance. (EqualityComparer .Default); this.typeCache = new Dictionary (EqualityComparer .Default); this.childTypesCache = new Dictionary >(ReferenceEqualityComparer .Instance); this.entitySets = new Dictionary (EqualityComparer .Default); this.epmIsV1Compatible = true; this.resourceSetWrapperCache = new Dictionary (EqualityComparer .Default); this.serviceOperationWrapperCache = new Dictionary (EqualityComparer .Default); this.visibleTypeCache = new Dictionary (EqualityComparer .Default); this.resourceAssociationSetCache = new Dictionary (EqualityComparer .Default); this.resourcePropertyCache = new Dictionary >(EqualityComparer .Default); this.entityTypeDisallowedForSet = new Dictionary (EqualityComparer .Default); this.type = type; this.EdmSchemaVersion = MetadataEdmSchemaVersion.Version1Dot0; } #region Properties. /// Service configuration information. internal DataServiceConfiguration Configuration { [DebuggerStepThrough] get { return this.configuration; } set { Debug.Assert(value != null, "value != null"); Debug.Assert(this.configuration == null, "this.configuration == null -- otherwise it's being set more than once"); this.configuration = value; } } ////// Keep track of the calculated visibility of resource types. /// internal DictionaryVisibleTypeCache { [DebuggerStepThrough] get { return this.visibleTypeCache; } } /// /// Maps resource set names to ResourceSetWrappers. /// internal DictionaryResourceSetWrapperCache { [DebuggerStepThrough] get { return this.resourceSetWrapperCache; } } /// /// Maps service operation names to ServiceOperationWrappers. /// internal DictionaryServiceOperationWrapperCache { [DebuggerStepThrough] get { return this.serviceOperationWrapperCache; } } /// /// Maps names to ResourceAssociationSets. /// internal DictionaryResourceAssociationSetCache { [DebuggerStepThrough] get { return this.resourceAssociationSetCache; } } /// /// Mapes "resourceSetName_resourceTypeName" to the list of visible properties from the set. /// internal Dictionary> ResourcePropertyCache { [DebuggerStepThrough] get { return this.resourcePropertyCache; } } /// /// Mapes "resourceSetName_resourceTypeName" to boolean of whether resourceType is allowed for resourceSet /// internal DictionaryEntityTypeDisallowedForSet { [DebuggerStepThrough] get { return this.entityTypeDisallowedForSet; } } /// Collection of service operations, keyed by name. internal DictionaryServiceOperations { [DebuggerStepThrough] get { return this.serviceOperations; } } /// Cache of resource properties per type. internal DictionaryTypeCache { [DebuggerStepThrough] get { return this.typeCache; } } /// Cache of immediate derived types per type. internal Dictionary> ChildTypesCache { [DebuggerStepThrough] get { return this.childTypesCache; } } /// list of top level entity sets internal DictionaryEntitySets { [DebuggerStepThrough] get { return this.entitySets; } } /// Target type for the data provider. internal Type Type { [DebuggerStepThrough] get { return this.type; } } ///EDM version to which metadata is compatible. ////// For example, a service operation of type Void is not acceptable 1.0 CSDL, /// so it should use 1.1 CSDL instead. Similarly, OpenTypes are supported /// in 1.2 and not before. /// internal MetadataEdmSchemaVersion EdmSchemaVersion { get; set; } ///Whether all EPM properties serialize in an Astoria V1-compatible way. ////// This property is false if any property has KeepInContent set to false. /// internal bool EpmIsV1Compatible { get { return this.epmIsV1Compatible; } set { this.epmIsV1Compatible = value; } } #endregion Properties. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
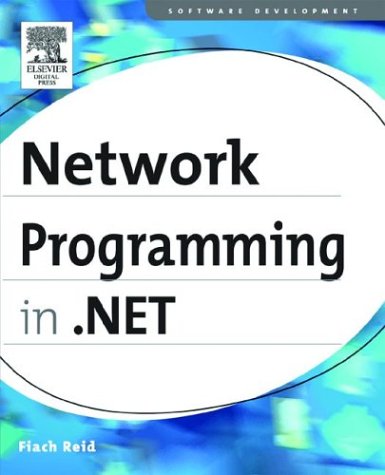
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DynamicValueConverter.cs
- TransferMode.cs
- DataColumnCollection.cs
- Funcletizer.cs
- RadioButton.cs
- ParameterToken.cs
- ObjectViewQueryResultData.cs
- VersionedStream.cs
- VectorCollection.cs
- AnimationStorage.cs
- DocumentViewer.cs
- UnsafeNativeMethodsPenimc.cs
- Resources.Designer.cs
- ControlCachePolicy.cs
- Graphics.cs
- SecurityTokenException.cs
- versioninfo.cs
- AvTraceDetails.cs
- DataViewListener.cs
- DataSourceCacheDurationConverter.cs
- ZipIOExtraField.cs
- DataRowComparer.cs
- StorageBasedPackageProperties.cs
- WebBrowserSiteBase.cs
- SurrogateChar.cs
- FreeFormDesigner.cs
- typedescriptorpermissionattribute.cs
- LinearGradientBrush.cs
- Rotation3DAnimation.cs
- GiveFeedbackEvent.cs
- BindingGroup.cs
- DetailsViewRow.cs
- ThrowHelper.cs
- DataTableTypeConverter.cs
- EdmType.cs
- SqlConnectionString.cs
- MembershipUser.cs
- SecurityUniqueId.cs
- HttpDebugHandler.cs
- ReflectionPermission.cs
- MenuItemStyle.cs
- RegexNode.cs
- RenderContext.cs
- Roles.cs
- ImageConverter.cs
- LocalizationComments.cs
- SQLUtility.cs
- PersonalizationProvider.cs
- TimerEventSubscriptionCollection.cs
- ProcessStartInfo.cs
- UnmanagedMemoryStream.cs
- SkewTransform.cs
- KeysConverter.cs
- OleDbParameter.cs
- Deflater.cs
- X509Certificate.cs
- URL.cs
- GeometryConverter.cs
- TrackingCondition.cs
- RtfToXamlLexer.cs
- ClientUrlResolverWrapper.cs
- DataGridTemplateColumn.cs
- Semaphore.cs
- DynamicContractTypeBuilder.cs
- CodeIdentifier.cs
- ExecutorLocksHeldException.cs
- tibetanshape.cs
- ObjectNavigationPropertyMapping.cs
- WebBrowsableAttribute.cs
- TransformerInfo.cs
- MimeParameter.cs
- PointAnimation.cs
- UpdateException.cs
- RectangleF.cs
- FilteredDataSetHelper.cs
- CollectionView.cs
- Parameter.cs
- OperatingSystem.cs
- SecurityUtils.cs
- ClientTargetCollection.cs
- DesignerToolboxInfo.cs
- DSASignatureDeformatter.cs
- SoapAttributeAttribute.cs
- TimeSpanParse.cs
- FakeModelPropertyImpl.cs
- Brush.cs
- ContentValidator.cs
- Button.cs
- ClosableStream.cs
- DSASignatureDeformatter.cs
- __ConsoleStream.cs
- LinqDataSourceInsertEventArgs.cs
- CommandManager.cs
- DataListItemCollection.cs
- TextTreeTextElementNode.cs
- Rule.cs
- RunInstallerAttribute.cs
- NavigationProperty.cs
- IUnknownConstantAttribute.cs
- Message.cs