Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Data / System / Data / SQLTypes / SQLUtility.cs / 1305376 / SQLUtility.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //junfang //[....] //[....] //----------------------------------------------------------------------------- //************************************************************************* // @File: SQLUtility.cs // // Create by: JunFang // // Purpose: Implementation of utilities in COM+ SQL Types Library. // Includes interface INullable, exceptions SqlNullValueException // and SqlTruncateException, and SQLDebug class. // // Notes: // // History: // // 09/17/99 JunFang Created and implemented as first drop. // // @EndHeader@ //************************************************************************* using System; using System.Data.Sql; using System.Data.SqlClient; using System.Diagnostics; using System.Globalization; using System.Runtime.Serialization; namespace System.Data.SqlTypes { internal enum EComparison { LT, LE, EQ, GE, GT, NE } // This enumeration is used to inquire about internal storage of a SqlBytes or SqlChars instance: public enum StorageState { Buffer = 0, Stream = 1, #if WINFSFunctionality UnmanagedBuffer = 2, Delayed = 3 #else UnmanagedBuffer = 2 #endif } [Serializable] public class SqlTypeException : SystemException { public SqlTypeException() : this(Res.GetString(Res.SqlMisc_SqlTypeMessage), null) { // MDAC 82931, 84941 } // Creates a new SqlTypeException with its message string set to message. public SqlTypeException(String message) : this(message, null) { } public SqlTypeException(String message, Exception e) : base(message, e) { // MDAC 82931 HResult = HResults.SqlType; // MDAC 84941 } // runtime will call even if private... //protected SqlTypeException(SerializationInfo si, StreamingContext sc) : base(SqlTypeExceptionSerialization(si, sc), sc) { } static private SerializationInfo SqlTypeExceptionSerialization(SerializationInfo si, StreamingContext sc) { if ((null != si) && (1 == si.MemberCount)) { string message = si.GetString("SqlTypeExceptionMessage"); // WebData 104331 SqlTypeException fakeValue = new SqlTypeException(message); fakeValue.GetObjectData(si, sc); } return si; } } // SqlTypeException [Serializable] public sealed class SqlNullValueException : SqlTypeException { // Creates a new SqlNullValueException with its message string set to the common string. public SqlNullValueException() : this(SQLResource.NullValueMessage, null) { } // Creates a new NullValueException with its message string set to message. public SqlNullValueException(String message) : this(message, null) { } public SqlNullValueException(String message, Exception e) : base(message, e) { // MDAC 82931 HResult = HResults.SqlNullValue; // MDAC 84941 } // runtime will call even if private... // private SqlNullValueException(SerializationInfo si, StreamingContext sc) : base(SqlNullValueExceptionSerialization(si, sc), sc) { } static private SerializationInfo SqlNullValueExceptionSerialization(SerializationInfo si, StreamingContext sc) { if ((null != si) && (1 == si.MemberCount)) { string message = si.GetString("SqlNullValueExceptionMessage"); // WebData 104331 SqlNullValueException fakeValue = new SqlNullValueException(message); fakeValue.GetObjectData(si, sc); } return si; } } // NullValueException [Serializable] public sealed class SqlTruncateException : SqlTypeException { // Creates a new SqlTruncateException with its message string set to the empty string. public SqlTruncateException() : this(SQLResource.TruncationMessage, null) { } // Creates a new SqlTruncateException with its message string set to message. public SqlTruncateException(String message) : this(message, null) { } public SqlTruncateException(String message, Exception e) : base(message, e) { // MDAC 82931 HResult = HResults.SqlTruncate; // MDAC 84941 } // runtime will call even if private... // private SqlTruncateException(SerializationInfo si, StreamingContext sc) : base(SqlTruncateExceptionSerialization(si, sc), sc) { } static private SerializationInfo SqlTruncateExceptionSerialization(SerializationInfo si, StreamingContext sc) { if ((null != si) && (1 == si.MemberCount)) { string message = si.GetString("SqlTruncateExceptionMessage"); // WebData 104331 SqlTruncateException fakeValue = new SqlTruncateException(message); fakeValue.GetObjectData(si, sc); } return si; } } // SqlTruncateException [Serializable] public sealed class SqlNotFilledException : SqlTypeException { // // Creates a new SqlNotFilledException with its message string set to the common string. public SqlNotFilledException() : this(SQLResource.NotFilledMessage, null) { } // Creates a new NullValueException with its message string set to message. public SqlNotFilledException(String message) : this(message, null) { } public SqlNotFilledException(String message, Exception e) : base(message, e) { // MDAC 82931 HResult = HResults.SqlNullValue; // MDAC 84941 } // runtime will call even if private... // private SqlNotFilledException(SerializationInfo si, StreamingContext sc) : base(si, sc) { } } // SqlNotFilledException [Serializable] public sealed class SqlAlreadyFilledException : SqlTypeException { // // Creates a new SqlNotFilledException with its message string set to the common string. public SqlAlreadyFilledException() : this(SQLResource.AlreadyFilledMessage, null) { } // Creates a new NullValueException with its message string set to message. public SqlAlreadyFilledException(String message) : this(message, null) { } public SqlAlreadyFilledException(String message, Exception e) : base(message, e) { // MDAC 82931 HResult = HResults.SqlNullValue; // MDAC 84941 } // runtime will call even if private... // private SqlAlreadyFilledException(SerializationInfo si, StreamingContext sc) : base(si, sc) { } } // SqlNotFilledException internal sealed class SQLDebug { private SQLDebug() { /* prevent utility class from being insantiated*/ } [System.Diagnostics.Conditional("DEBUG")] internal static void Check(bool condition) { Debug.Assert(condition, "", ""); } [System.Diagnostics.Conditional("DEBUG")] internal static void Check(bool condition, String conditionString, string message) { Debug.Assert(condition, conditionString, message); } [System.Diagnostics.Conditional("DEBUG")] internal static void Check(bool condition, String conditionString) { Debug.Assert(condition, conditionString, ""); } /* [System.Diagnostics.Conditional("DEBUG")] internal static void Message(String traceMessage) { Debug.WriteLine(SQLResource.MessageString + ": " + traceMessage); } */ } // SQLDebug #if WINFSFunctionality internal static class SQLUtility { internal static object GetLOBFromCookie(byte[] cookie, SqlConnection connection, SqlTransaction transaction) { // Error checking??? SqlCommand command = connection.CreateCommand(); command.CommandText = "sp_fetchLOBfromcookie"; if (transaction != null) command.Transaction = transaction; command.CommandType = CommandType.StoredProcedure; SqlParameter parameter = command.Parameters.Add("@cookie", SqlDbType.VarBinary); parameter.Direction = ParameterDirection.Input; parameter.Value = cookie; parameter.Size = -1; return command.ExecuteScalar(); } } #endif } // namespace System.Data.SqlTypes // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
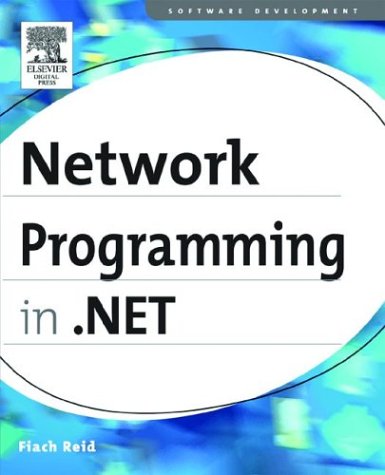
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- JsonWriterDelegator.cs
- Accessible.cs
- InvalidDataException.cs
- CustomTrackingQuery.cs
- View.cs
- SafeHandles.cs
- TextDecorationLocationValidation.cs
- MatrixValueSerializer.cs
- printdlgexmarshaler.cs
- FormatterConverter.cs
- IdentifierCollection.cs
- ErrorStyle.cs
- WebServiceResponse.cs
- CodeSubDirectoriesCollection.cs
- ToolStripItemClickedEventArgs.cs
- StickyNoteHelper.cs
- ModelItemCollection.cs
- XPathDescendantIterator.cs
- AnnotationAuthorChangedEventArgs.cs
- QuadraticBezierSegment.cs
- X509PeerCertificateAuthentication.cs
- Encoding.cs
- GridViewDeletedEventArgs.cs
- InternalBufferManager.cs
- ExpressionLexer.cs
- MobileErrorInfo.cs
- SchemaImporterExtensionsSection.cs
- BitmapCodecInfo.cs
- Ppl.cs
- BinHexDecoder.cs
- SchemaTypeEmitter.cs
- InternalConfigHost.cs
- StrokeFIndices.cs
- FileSystemEventArgs.cs
- ping.cs
- DocumentAutomationPeer.cs
- GenericAuthenticationEventArgs.cs
- FieldMetadata.cs
- XmlSerializerFactory.cs
- _NegotiateClient.cs
- StorageEntitySetMapping.cs
- PermissionRequestEvidence.cs
- PageContent.cs
- BinaryConverter.cs
- WorkflowEnvironment.cs
- BinaryParser.cs
- BrowserCapabilitiesFactory.cs
- MediaTimeline.cs
- IsolatedStorageFile.cs
- ForeignConstraint.cs
- ContainerSelectorActiveEvent.cs
- PagerSettings.cs
- CurrencyManager.cs
- RoutedEventHandlerInfo.cs
- MsmqIntegrationReceiveParameters.cs
- MSAAWinEventWrap.cs
- QueryActivatableWorkflowsCommand.cs
- PartitionerQueryOperator.cs
- DataSourceHelper.cs
- DbConnectionPoolGroupProviderInfo.cs
- ToolStrip.cs
- AssemblyNameEqualityComparer.cs
- SessionStateContainer.cs
- MdImport.cs
- ExpandedProjectionNode.cs
- Journal.cs
- LinkLabelLinkClickedEvent.cs
- CaseInsensitiveComparer.cs
- StylusOverProperty.cs
- SharedPersonalizationStateInfo.cs
- RuntimeConfig.cs
- DBCommand.cs
- TabletDevice.cs
- TextSegment.cs
- DbParameterCollection.cs
- SQLDateTimeStorage.cs
- DbUpdateCommandTree.cs
- CFGGrammar.cs
- CheckPair.cs
- TextTreeTextElementNode.cs
- EntryPointNotFoundException.cs
- InputMethodStateChangeEventArgs.cs
- DbConnectionPoolIdentity.cs
- ControlParameter.cs
- HttpServerChannel.cs
- FSWPathEditor.cs
- Knowncolors.cs
- StylusEventArgs.cs
- CqlParser.cs
- DotExpr.cs
- VarInfo.cs
- ImageSourceValueSerializer.cs
- DataStreamFromComStream.cs
- MetadataResolver.cs
- NetCodeGroup.cs
- PeerNameResolver.cs
- VersionConverter.cs
- ExpressionBuilder.cs
- SqlBuffer.cs
- TableItemPatternIdentifiers.cs