Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Interaction / Model / ModelItemCollection.cs / 1305376 / ModelItemCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System.Activities.Presentation.Internal.Properties; using System.Activities.Presentation; using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Globalization; using System.Windows; ////// ModelItemCollection derives from ModelItem and implements /// support for a collection of items. /// /// ModelItemCollection defines a static attached property called /// Item. This property is returned from the Properties /// enumeration of the collection, in addition to any properties /// defined on the collection. The Item property represents all /// the items in the collection and is defined as type /// IEnumerable of ModelItem. All items in the collection have /// their Source property set to this property. The property’s metadata /// marks it non browsable and non-serializable. The Item property is a /// “pseudo” property because it is not actually set on the model. The /// value it points to is the ModelItemCollection itself. /// public abstract class ModelItemCollection : ModelItem, IList, IList, INotifyCollectionChanged { /// /// Creates a new ModelItemCollection. /// protected ModelItemCollection() { } ////// Returns the item at the given index. Sets the item at the /// given index to the given value. /// /// The zero-based index into the collection. ////// if value is null. ///if index is less than 0 or greater than or equal to count. public abstract ModelItem this[int index] { get; set; } ////// Returns the count of items in the collection. /// public abstract int Count { get; } ////// Returns true if the collection is a fixed size. /// The default implementation returns true if the /// collection is read only. /// protected virtual bool IsFixedSize { get { return IsReadOnly; } } ////// Returns true if the collection cannot be modified. /// public abstract bool IsReadOnly { get; } ////// Protected access to ICollection.IsSynchronized. /// protected virtual bool IsSynchronized { get { return false; } } ////// Protected access to the SyncRoot object used to synchronize /// this collection. The default value returns "this". /// protected virtual object SyncRoot { get { return this; } } ////// This event is raised when the contents of this collection change. /// public abstract event NotifyCollectionChangedEventHandler CollectionChanged; ////// Adds the item to the collection. /// /// ///if item is null. ///if the collection is read only. public abstract void Add(ModelItem item); ////// Adds the given value to the collection. This will create an item /// for the value. It returns the newly created item. /// /// ///an item representing the value ///if value is null. ///if the collection is read only. public abstract ModelItem Add(object value); ////// Clears the contents of the collection. /// ///if the collection is read only. public abstract void Clear(); ////// Returns true if the collection contains the given item. /// /// ////// if item is null. public abstract bool Contains(ModelItem item); ////// Returns true if the collection contains the given value. /// /// ////// if value is null. public abstract bool Contains(object value); // // Helper method that verifies that objects can be upcast to // the correct type. // private static ModelItem ConvertType(object value) { try { return (ModelItem)value; } catch (InvalidCastException) { throw FxTrace.Exception.AsError(new ArgumentException( string.Format(CultureInfo.CurrentCulture, Resources.Error_ArgIncorrectType, "value", typeof(ModelItem).FullName))); } } ////// Copies the contents of the collection into the given array. /// ///if array is null. ////// if arrayIndex is outside the bounds of the items array or if there is /// insuffient space in the array to hold the collection. /// public abstract void CopyTo(ModelItem[] array, int arrayIndex); ////// Returns an enumerator for the items in the collection. /// ///public abstract IEnumerator GetEnumerator(); /// /// Returns the index of the given item or -1 if the item does not exist. /// /// ////// if item is null. public abstract int IndexOf(ModelItem item); ////// Inserts the item at the given location. To /// move an item, use Move. If index is == Count this will insert the item /// at the end. If it is zero it will insert at the beginning. /// /// /// ///if item is null. ///if index is less than 0 or greater than count. public abstract void Insert(int index, ModelItem item); ////// Inserts the item at the given location. To /// move an item, use Move. If index is == Count this will insert the item /// at the end. If it is zero it will insert at the beginning. /// /// /// ///an item representing the value ///if value is null. ///if index is less than 0 or greater than count. public abstract ModelItem Insert(int index, object value); ////// Moves the item at fromIndex to toIndex. The value for toIndex is /// always where you want the item to be according to how the collection /// currently sits. This means that if you are moving an item to a higher /// index you don’t have to account for the fact that the indexes will /// shuffle when the item is removed from its current location. /// /// /// The index of the item to move. /// /// /// The index to move it to. /// ////// if fromIndex or toIndex is less than zero or greater than or /// equal to Count. /// public abstract void Move(int fromIndex, int toIndex); ////// Removes the item from the collection. This does nothing if the /// item does not exist in the collection. /// /// ///if item is null. public abstract bool Remove(ModelItem item); ////// Removes the value from the collection. This does nothing if the /// value does not exist in the collection. /// /// ///if value is null. public abstract bool Remove(object value); ////// Removes the item at the given index. /// /// ///if index is less than 0 or greater than or equal to count. public abstract void RemoveAt(int index); ////// This property is returned from the Properties enumeration of /// the collection, in addition to any properties defined on the /// collection. The Item property represents all the items in /// the collection and is defined as type IEnumerable of ModelItem. /// All items in the collection have their Source property set to /// this property. The property’s metadata marks it non browsable /// and non-serializable. The Item property is a “pseudo” property /// because it is not actually set on the model. The value it points /// to is the ModelItemCollection itself. /// public static readonly DependencyProperty ItemProperty = DependencyProperty.RegisterAttachedReadOnly( "Item", typeof(IEnumerable), typeof(ModelItemCollection), null).DependencyProperty; #region IList Members /// /// IList Implementation maps back to public API. /// int IList.Add(object value) { Add(value); return Count - 1; } ////// IList Implementation maps back to public API. /// void IList.Clear() { Clear(); } ////// IList Implementation maps back to public API. /// bool IList.Contains(object value) { return Contains(value); } ////// IList Implementation maps back to public API. /// int IList.IndexOf(object value) { return IndexOf(ConvertType(value)); } ////// IList Implementation maps back to public API. /// void IList.Insert(int index, object value) { Insert(index, value); } ////// IList Implementation maps back to public API. /// bool IList.IsFixedSize { get { return IsFixedSize; } } ////// IList Implementation maps back to public API. /// bool IList.IsReadOnly { get { return IsReadOnly; } } ////// IList Implementation maps back to public API. /// void IList.Remove(object value) { Remove(value); } ////// IList Implementation maps back to public API. /// void IList.RemoveAt(int index) { RemoveAt(index); } ////// IList Implementation maps back to public API. /// object IList.this[int index] { get { return this[index]; } set { this[index] = ConvertType(value); } } #endregion #region ICollection Members ////// ICollection Implementation maps back to public API. /// void ICollection.CopyTo(Array array, int index) { for (int idx = 0; idx < Count; idx++) { array.SetValue(this[idx], idx + index); } } ////// ICollection Implementation maps back to public API. /// int ICollection.Count { get { return Count; } } ////// ICollection Implementation maps back to public API. /// bool ICollection.IsSynchronized { get { return IsSynchronized; } } ////// ICollection Implementation maps back to public API. /// object ICollection.SyncRoot { get { return SyncRoot; } } #endregion #region IEnumerable Members ////// IEnumerable Implementation maps back to public API. /// IEnumerator IEnumerable.GetEnumerator() { foreach (object o in this) { yield return o; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System.Activities.Presentation.Internal.Properties; using System.Activities.Presentation; using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Globalization; using System.Windows; ////// ModelItemCollection derives from ModelItem and implements /// support for a collection of items. /// /// ModelItemCollection defines a static attached property called /// Item. This property is returned from the Properties /// enumeration of the collection, in addition to any properties /// defined on the collection. The Item property represents all /// the items in the collection and is defined as type /// IEnumerable of ModelItem. All items in the collection have /// their Source property set to this property. The property’s metadata /// marks it non browsable and non-serializable. The Item property is a /// “pseudo” property because it is not actually set on the model. The /// value it points to is the ModelItemCollection itself. /// public abstract class ModelItemCollection : ModelItem, IList, IList, INotifyCollectionChanged { /// /// Creates a new ModelItemCollection. /// protected ModelItemCollection() { } ////// Returns the item at the given index. Sets the item at the /// given index to the given value. /// /// The zero-based index into the collection. ////// if value is null. ///if index is less than 0 or greater than or equal to count. public abstract ModelItem this[int index] { get; set; } ////// Returns the count of items in the collection. /// public abstract int Count { get; } ////// Returns true if the collection is a fixed size. /// The default implementation returns true if the /// collection is read only. /// protected virtual bool IsFixedSize { get { return IsReadOnly; } } ////// Returns true if the collection cannot be modified. /// public abstract bool IsReadOnly { get; } ////// Protected access to ICollection.IsSynchronized. /// protected virtual bool IsSynchronized { get { return false; } } ////// Protected access to the SyncRoot object used to synchronize /// this collection. The default value returns "this". /// protected virtual object SyncRoot { get { return this; } } ////// This event is raised when the contents of this collection change. /// public abstract event NotifyCollectionChangedEventHandler CollectionChanged; ////// Adds the item to the collection. /// /// ///if item is null. ///if the collection is read only. public abstract void Add(ModelItem item); ////// Adds the given value to the collection. This will create an item /// for the value. It returns the newly created item. /// /// ///an item representing the value ///if value is null. ///if the collection is read only. public abstract ModelItem Add(object value); ////// Clears the contents of the collection. /// ///if the collection is read only. public abstract void Clear(); ////// Returns true if the collection contains the given item. /// /// ////// if item is null. public abstract bool Contains(ModelItem item); ////// Returns true if the collection contains the given value. /// /// ////// if value is null. public abstract bool Contains(object value); // // Helper method that verifies that objects can be upcast to // the correct type. // private static ModelItem ConvertType(object value) { try { return (ModelItem)value; } catch (InvalidCastException) { throw FxTrace.Exception.AsError(new ArgumentException( string.Format(CultureInfo.CurrentCulture, Resources.Error_ArgIncorrectType, "value", typeof(ModelItem).FullName))); } } ////// Copies the contents of the collection into the given array. /// ///if array is null. ////// if arrayIndex is outside the bounds of the items array or if there is /// insuffient space in the array to hold the collection. /// public abstract void CopyTo(ModelItem[] array, int arrayIndex); ////// Returns an enumerator for the items in the collection. /// ///public abstract IEnumerator GetEnumerator(); /// /// Returns the index of the given item or -1 if the item does not exist. /// /// ////// if item is null. public abstract int IndexOf(ModelItem item); ////// Inserts the item at the given location. To /// move an item, use Move. If index is == Count this will insert the item /// at the end. If it is zero it will insert at the beginning. /// /// /// ///if item is null. ///if index is less than 0 or greater than count. public abstract void Insert(int index, ModelItem item); ////// Inserts the item at the given location. To /// move an item, use Move. If index is == Count this will insert the item /// at the end. If it is zero it will insert at the beginning. /// /// /// ///an item representing the value ///if value is null. ///if index is less than 0 or greater than count. public abstract ModelItem Insert(int index, object value); ////// Moves the item at fromIndex to toIndex. The value for toIndex is /// always where you want the item to be according to how the collection /// currently sits. This means that if you are moving an item to a higher /// index you don’t have to account for the fact that the indexes will /// shuffle when the item is removed from its current location. /// /// /// The index of the item to move. /// /// /// The index to move it to. /// ////// if fromIndex or toIndex is less than zero or greater than or /// equal to Count. /// public abstract void Move(int fromIndex, int toIndex); ////// Removes the item from the collection. This does nothing if the /// item does not exist in the collection. /// /// ///if item is null. public abstract bool Remove(ModelItem item); ////// Removes the value from the collection. This does nothing if the /// value does not exist in the collection. /// /// ///if value is null. public abstract bool Remove(object value); ////// Removes the item at the given index. /// /// ///if index is less than 0 or greater than or equal to count. public abstract void RemoveAt(int index); ////// This property is returned from the Properties enumeration of /// the collection, in addition to any properties defined on the /// collection. The Item property represents all the items in /// the collection and is defined as type IEnumerable of ModelItem. /// All items in the collection have their Source property set to /// this property. The property’s metadata marks it non browsable /// and non-serializable. The Item property is a “pseudo” property /// because it is not actually set on the model. The value it points /// to is the ModelItemCollection itself. /// public static readonly DependencyProperty ItemProperty = DependencyProperty.RegisterAttachedReadOnly( "Item", typeof(IEnumerable), typeof(ModelItemCollection), null).DependencyProperty; #region IList Members /// /// IList Implementation maps back to public API. /// int IList.Add(object value) { Add(value); return Count - 1; } ////// IList Implementation maps back to public API. /// void IList.Clear() { Clear(); } ////// IList Implementation maps back to public API. /// bool IList.Contains(object value) { return Contains(value); } ////// IList Implementation maps back to public API. /// int IList.IndexOf(object value) { return IndexOf(ConvertType(value)); } ////// IList Implementation maps back to public API. /// void IList.Insert(int index, object value) { Insert(index, value); } ////// IList Implementation maps back to public API. /// bool IList.IsFixedSize { get { return IsFixedSize; } } ////// IList Implementation maps back to public API. /// bool IList.IsReadOnly { get { return IsReadOnly; } } ////// IList Implementation maps back to public API. /// void IList.Remove(object value) { Remove(value); } ////// IList Implementation maps back to public API. /// void IList.RemoveAt(int index) { RemoveAt(index); } ////// IList Implementation maps back to public API. /// object IList.this[int index] { get { return this[index]; } set { this[index] = ConvertType(value); } } #endregion #region ICollection Members ////// ICollection Implementation maps back to public API. /// void ICollection.CopyTo(Array array, int index) { for (int idx = 0; idx < Count; idx++) { array.SetValue(this[idx], idx + index); } } ////// ICollection Implementation maps back to public API. /// int ICollection.Count { get { return Count; } } ////// ICollection Implementation maps back to public API. /// bool ICollection.IsSynchronized { get { return IsSynchronized; } } ////// ICollection Implementation maps back to public API. /// object ICollection.SyncRoot { get { return SyncRoot; } } #endregion #region IEnumerable Members ////// IEnumerable Implementation maps back to public API. /// IEnumerator IEnumerable.GetEnumerator() { foreach (object o in this) { yield return o; } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
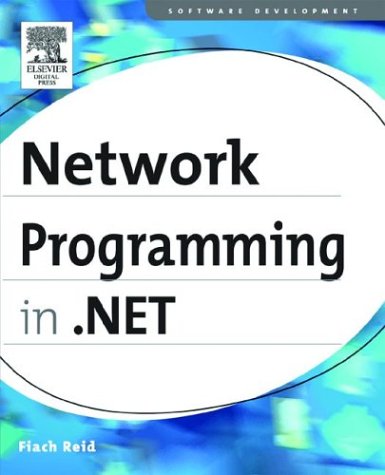
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SimpleTypeResolver.cs
- StreamGeometry.cs
- DbBuffer.cs
- DesignerAttribute.cs
- HiddenFieldPageStatePersister.cs
- validationstate.cs
- StateManagedCollection.cs
- SupportedAddressingMode.cs
- CustomTrackingRecord.cs
- StatusBarAutomationPeer.cs
- QualificationDataItem.cs
- XmlSerializationGeneratedCode.cs
- RegexBoyerMoore.cs
- SafeMarshalContext.cs
- HelpEvent.cs
- MenuCommand.cs
- OdbcUtils.cs
- ZoneMembershipCondition.cs
- DtcInterfaces.cs
- SafeRightsManagementPubHandle.cs
- XmlDocument.cs
- AspNetPartialTrustHelpers.cs
- IProvider.cs
- ActivityInstance.cs
- DesignBindingPicker.cs
- StringBuilder.cs
- DataControlImageButton.cs
- Translator.cs
- ToolStripGripRenderEventArgs.cs
- TransportBindingElement.cs
- HttpCacheVaryByContentEncodings.cs
- ProfileService.cs
- ColorDialog.cs
- IssuanceLicense.cs
- ValueTypeFixupInfo.cs
- ActivityCodeDomReferenceService.cs
- SmiEventSink.cs
- FrameDimension.cs
- DbMetaDataColumnNames.cs
- FieldTemplateFactory.cs
- RelationshipConstraintValidator.cs
- SpAudioStreamWrapper.cs
- SHA384Cng.cs
- CompilationUtil.cs
- SingleStorage.cs
- WebControl.cs
- QuaternionConverter.cs
- Header.cs
- OleDbWrapper.cs
- UserUseLicenseDictionaryLoader.cs
- DataGridViewLayoutData.cs
- XhtmlBasicValidatorAdapter.cs
- AsyncOperation.cs
- WebConfigurationFileMap.cs
- EdmTypeAttribute.cs
- WebPartCatalogCloseVerb.cs
- TypeContext.cs
- EdmComplexTypeAttribute.cs
- HandleExceptionArgs.cs
- SpAudioStreamWrapper.cs
- hebrewshape.cs
- LiteralControl.cs
- DesignerForm.cs
- TableRowCollection.cs
- SoapIgnoreAttribute.cs
- SetStoryboardSpeedRatio.cs
- XPathQilFactory.cs
- TableCellCollection.cs
- DrawToolTipEventArgs.cs
- LayoutDump.cs
- ConfigXmlCDataSection.cs
- StubHelpers.cs
- StringSource.cs
- ZipPackagePart.cs
- SystemPens.cs
- InputScopeNameConverter.cs
- ProcessDesigner.cs
- Adorner.cs
- DSASignatureDeformatter.cs
- Line.cs
- CodeAttributeDeclaration.cs
- PropertyEmitterBase.cs
- WindowsScrollBarBits.cs
- XmlDocumentSchema.cs
- DropAnimation.xaml.cs
- FullTextState.cs
- LowerCaseStringConverter.cs
- VectorCollectionValueSerializer.cs
- WeakEventTable.cs
- CursorInteropHelper.cs
- XmlWhitespace.cs
- DesigntimeLicenseContextSerializer.cs
- ReadWriteControlDesigner.cs
- ParseChildrenAsPropertiesAttribute.cs
- ProfilePropertySettingsCollection.cs
- PersonalizablePropertyEntry.cs
- ProtocolsConfigurationEntry.cs
- CodeDirectionExpression.cs
- SrgsRule.cs
- XmlHierarchicalEnumerable.cs