Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / SapiInterop / SpAudioStreamWrapper.cs / 1 / SpAudioStreamWrapper.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // Maps an audio stream the SAPI ISpStreamFormat // // History: // 7/10/2005 jeanfp //----------------------------------------------------------------- using System; using System.IO; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Speech.AudioFormat; using System.Speech.Internal.SapiInterop; using System.Speech.Internal.Synthesis; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. using STATSTG = System.Runtime.InteropServices.ComTypes.STATSTG; namespace System.Speech.Internal.SapiInterop { internal class SpAudioStreamWrapper : SpStreamWrapper, ISpStreamFormat { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal SpAudioStreamWrapper (Stream stream, SpeechAudioFormatInfo audioFormat) : base (stream) { // Assume PCM to start with _formatType = SAPIGuids.SPDFID_WaveFormatEx; if (audioFormat != null) { WAVEFORMATEX wfx = new WAVEFORMATEX (); wfx.wFormatTag = (short) audioFormat.EncodingFormat; wfx.nChannels = (short) audioFormat.ChannelCount; wfx.nSamplesPerSec = audioFormat.SamplesPerSecond; wfx.nAvgBytesPerSec = audioFormat.AverageBytesPerSecond; wfx.nBlockAlign = (short) audioFormat.BlockAlign; wfx.wBitsPerSample = (short) audioFormat.BitsPerSample; wfx.cbSize = (short) audioFormat.FormatSpecificData ().Length; _wfx = wfx.ToBytes (); if (wfx.cbSize == 0) { byte [] wfxTemp = new byte [_wfx.Length + wfx.cbSize]; Array.Copy (_wfx, wfxTemp, _wfx.Length); Array.Copy (audioFormat.FormatSpecificData (), 0, wfxTemp, _wfx.Length, wfx.cbSize); _wfx = wfxTemp; } } else { try { GetStreamOffsets (stream); } catch (IOException) { throw new FormatException (SR.Get (SRID.SynthesizerInvalidWaveFile)); } } } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region public Methods #region ISpStreamFormat interface implementation void ISpStreamFormat.GetFormat (out Guid guid, out IntPtr format) { guid = _formatType; format = Marshal.AllocCoTaskMem (_wfx.Length); Marshal.Copy (_wfx, 0, format, _wfx.Length); } #endregion #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Methods #pragma warning disable 56518 // The Binary reader cannot be disposed or it would close the underlying stream ////// Builds the /// /// internal void GetStreamOffsets (Stream stream) { BinaryReader br = new BinaryReader (stream); // Read the riff Header RIFFHDR riff = new RIFFHDR (); riff._id = br.ReadUInt32 (); riff._len = br.ReadInt32 (); riff._type = br.ReadUInt32 (); if (riff._id != RIFF_MARKER && riff._type != WAVE_MARKER) { throw new FormatException (); } BLOCKHDR block = new BLOCKHDR (); block._id = br.ReadUInt32 (); block._len = br.ReadInt32 (); if (block._id != FMT_MARKER) { throw new FormatException (); } // If the format is of type WAVEFORMAT then fake a cbByte with a length of zero _wfx = br.ReadBytes (block._len); // Hardcode the value of the size for the structure element // as the C# compiler pads the structure to the closest 4 or 8 bytes if (block._len == 16) { byte [] wfxTemp = new byte [18]; Array.Copy (_wfx, wfxTemp, 16); _wfx = wfxTemp; } while (true) { DATAHDR dataHdr = new DATAHDR (); // check for the end of file (+8 for the 2 DWORD) if (stream.Position + 8 >= stream.Length) { break; } dataHdr._id = br.ReadUInt32 (); dataHdr._len = br.ReadInt32 (); // Is this the WAVE data? if (dataHdr._id == DATA_MARKER) { _endOfStreamPosition = stream.Position + dataHdr._len; break; } else { // Skip this RIFF fragment. stream.Seek (dataHdr._len, SeekOrigin.Current); } } } #pragma warning restore 56518 // The Binary reader cannot be disposed or it would close the underlying stream #endregion //******************************************************************* // // Private Types // //******************************************************************** #region Private Types private const UInt32 RIFF_MARKER = 0x46464952; private const UInt32 WAVE_MARKER = 0x45564157; private const UInt32 FMT_MARKER = 0x20746d66; private const UInt32 DATA_MARKER = 0x61746164; [StructLayout (LayoutKind.Sequential)] private struct RIFFHDR { internal UInt32 _id; internal Int32 _len; /* file length less header */ internal UInt32 _type; /* should be "WAVE" */ } [StructLayout (LayoutKind.Sequential)] private struct BLOCKHDR { internal UInt32 _id; /* should be "fmt " or "data" */ internal Int32 _len; /* block size less header */ }; [StructLayout (LayoutKind.Sequential)] private struct DATAHDR { internal UInt32 _id; /* should be "fmt " or "data" */ internal Int32 _len; /* block size less header */ } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region Private Fields private byte [] _wfx; private Guid _formatType; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // // Description: // Maps an audio stream the SAPI ISpStreamFormat // // History: // 7/10/2005 jeanfp //----------------------------------------------------------------- using System; using System.IO; using System.Runtime.InteropServices; using System.Runtime.InteropServices.ComTypes; using System.Speech.AudioFormat; using System.Speech.Internal.SapiInterop; using System.Speech.Internal.Synthesis; #pragma warning disable 1634, 1691 // Allows suppression of certain PreSharp messages. using STATSTG = System.Runtime.InteropServices.ComTypes.STATSTG; namespace System.Speech.Internal.SapiInterop { internal class SpAudioStreamWrapper : SpStreamWrapper, ISpStreamFormat { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal SpAudioStreamWrapper (Stream stream, SpeechAudioFormatInfo audioFormat) : base (stream) { // Assume PCM to start with _formatType = SAPIGuids.SPDFID_WaveFormatEx; if (audioFormat != null) { WAVEFORMATEX wfx = new WAVEFORMATEX (); wfx.wFormatTag = (short) audioFormat.EncodingFormat; wfx.nChannels = (short) audioFormat.ChannelCount; wfx.nSamplesPerSec = audioFormat.SamplesPerSecond; wfx.nAvgBytesPerSec = audioFormat.AverageBytesPerSecond; wfx.nBlockAlign = (short) audioFormat.BlockAlign; wfx.wBitsPerSample = (short) audioFormat.BitsPerSample; wfx.cbSize = (short) audioFormat.FormatSpecificData ().Length; _wfx = wfx.ToBytes (); if (wfx.cbSize == 0) { byte [] wfxTemp = new byte [_wfx.Length + wfx.cbSize]; Array.Copy (_wfx, wfxTemp, _wfx.Length); Array.Copy (audioFormat.FormatSpecificData (), 0, wfxTemp, _wfx.Length, wfx.cbSize); _wfx = wfxTemp; } } else { try { GetStreamOffsets (stream); } catch (IOException) { throw new FormatException (SR.Get (SRID.SynthesizerInvalidWaveFile)); } } } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region public Methods #region ISpStreamFormat interface implementation void ISpStreamFormat.GetFormat (out Guid guid, out IntPtr format) { guid = _formatType; format = Marshal.AllocCoTaskMem (_wfx.Length); Marshal.Copy (_wfx, 0, format, _wfx.Length); } #endregion #endregion //******************************************************************** // // Internal Properties // //******************************************************************** #region Internal Methods #pragma warning disable 56518 // The Binary reader cannot be disposed or it would close the underlying stream ////// Builds the /// /// internal void GetStreamOffsets (Stream stream) { BinaryReader br = new BinaryReader (stream); // Read the riff Header RIFFHDR riff = new RIFFHDR (); riff._id = br.ReadUInt32 (); riff._len = br.ReadInt32 (); riff._type = br.ReadUInt32 (); if (riff._id != RIFF_MARKER && riff._type != WAVE_MARKER) { throw new FormatException (); } BLOCKHDR block = new BLOCKHDR (); block._id = br.ReadUInt32 (); block._len = br.ReadInt32 (); if (block._id != FMT_MARKER) { throw new FormatException (); } // If the format is of type WAVEFORMAT then fake a cbByte with a length of zero _wfx = br.ReadBytes (block._len); // Hardcode the value of the size for the structure element // as the C# compiler pads the structure to the closest 4 or 8 bytes if (block._len == 16) { byte [] wfxTemp = new byte [18]; Array.Copy (_wfx, wfxTemp, 16); _wfx = wfxTemp; } while (true) { DATAHDR dataHdr = new DATAHDR (); // check for the end of file (+8 for the 2 DWORD) if (stream.Position + 8 >= stream.Length) { break; } dataHdr._id = br.ReadUInt32 (); dataHdr._len = br.ReadInt32 (); // Is this the WAVE data? if (dataHdr._id == DATA_MARKER) { _endOfStreamPosition = stream.Position + dataHdr._len; break; } else { // Skip this RIFF fragment. stream.Seek (dataHdr._len, SeekOrigin.Current); } } } #pragma warning restore 56518 // The Binary reader cannot be disposed or it would close the underlying stream #endregion //******************************************************************* // // Private Types // //******************************************************************** #region Private Types private const UInt32 RIFF_MARKER = 0x46464952; private const UInt32 WAVE_MARKER = 0x45564157; private const UInt32 FMT_MARKER = 0x20746d66; private const UInt32 DATA_MARKER = 0x61746164; [StructLayout (LayoutKind.Sequential)] private struct RIFFHDR { internal UInt32 _id; internal Int32 _len; /* file length less header */ internal UInt32 _type; /* should be "WAVE" */ } [StructLayout (LayoutKind.Sequential)] private struct BLOCKHDR { internal UInt32 _id; /* should be "fmt " or "data" */ internal Int32 _len; /* block size less header */ }; [StructLayout (LayoutKind.Sequential)] private struct DATAHDR { internal UInt32 _id; /* should be "fmt " or "data" */ internal Int32 _len; /* block size less header */ } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region Private Fields private byte [] _wfx; private Guid _formatType; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
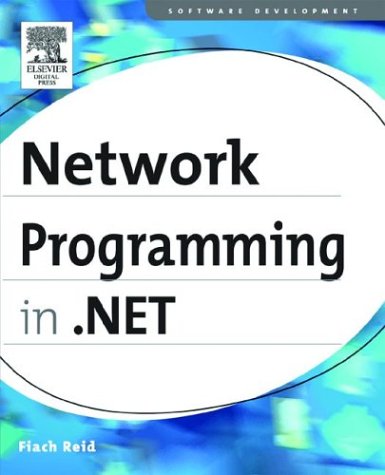
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ExpressionBindingsDialog.cs
- IdentityManager.cs
- SchemaImporterExtensionsSection.cs
- RecommendedAsConfigurableAttribute.cs
- ReflectEventDescriptor.cs
- InternalDuplexChannelListener.cs
- TdsRecordBufferSetter.cs
- DataTableMappingCollection.cs
- mediaclock.cs
- HttpCacheVary.cs
- DateTimeOffset.cs
- BasicHttpSecurityElement.cs
- MemoryFailPoint.cs
- RowToParametersTransformer.cs
- MessageBox.cs
- SpotLight.cs
- AbstractExpressions.cs
- MessagePartProtectionMode.cs
- ThreadPoolTaskScheduler.cs
- lengthconverter.cs
- DocumentReferenceCollection.cs
- Label.cs
- Site.cs
- ListViewGroup.cs
- SecurityElement.cs
- DataServiceQueryOfT.cs
- EntityDesignerDataSourceView.cs
- PngBitmapEncoder.cs
- ToolboxCategory.cs
- ComPlusContractBehavior.cs
- _FixedSizeReader.cs
- RegionInfo.cs
- WindowsGraphics.cs
- CoreSwitches.cs
- MultiplexingFormatMapping.cs
- ManagementScope.cs
- StructuredTypeInfo.cs
- Application.cs
- HttpModule.cs
- BaseDataList.cs
- XmlCustomFormatter.cs
- SchemaNamespaceManager.cs
- Cursor.cs
- DragDropManager.cs
- ListItemConverter.cs
- BuilderInfo.cs
- OpenFileDialog.cs
- ConnectionsZone.cs
- FontStretch.cs
- UserControlFileEditor.cs
- HostingEnvironmentException.cs
- GridEntry.cs
- CookieProtection.cs
- XmlSerializerSection.cs
- HuffModule.cs
- MDIControlStrip.cs
- TrackBarDesigner.cs
- Evaluator.cs
- HostedBindingBehavior.cs
- Funcletizer.cs
- RelativeSource.cs
- ReadOnlyDataSourceView.cs
- DataGridColumnFloatingHeader.cs
- CompoundFileReference.cs
- NegationPusher.cs
- Version.cs
- DelegateTypeInfo.cs
- ConnectionProviderAttribute.cs
- ToolBarButtonDesigner.cs
- InvalidDataException.cs
- GrowingArray.cs
- FixedSOMTable.cs
- XmlSerializerImportOptions.cs
- Model3D.cs
- StringFreezingAttribute.cs
- FileDialogPermission.cs
- PersonalizationAdministration.cs
- DataControlLinkButton.cs
- OrthographicCamera.cs
- OrderPreservingPipeliningMergeHelper.cs
- ZipPackage.cs
- ReferencedCollectionType.cs
- DomNameTable.cs
- LayoutTable.cs
- DatePickerAutomationPeer.cs
- TreeNodeConverter.cs
- RequestNavigateEventArgs.cs
- CurrentTimeZone.cs
- UInt16Storage.cs
- TextLine.cs
- WebPartUserCapability.cs
- PermissionSet.cs
- OleDbParameter.cs
- DataGridTable.cs
- AlternateView.cs
- DefaultDialogButtons.cs
- DocumentPageViewAutomationPeer.cs
- UntrustedRecipientException.cs
- BitmapEffectGroup.cs
- BuildResultCache.cs