Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ListItemConverter.cs / 1305376 / ListItemConverter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms { using System.Runtime.Serialization.Formatters; using System.Runtime.Remoting; using System.Runtime.InteropServices; using Microsoft.Win32; using System.Collections; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Drawing; using System.Diagnostics; using System.Globalization; using System.Reflection; ////// /// ListViewItemConverter is a class that can be used to convert /// ListViewItem objects from one data type to another. Access this /// class through the TypeDescriptor. /// public class ListViewItemConverter : ExpandableObjectConverter { ////// /// public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } ///Gets a value indicating whether this converter can /// convert an object to the given destination type using the context. ////// /// Converts the given object to another type. The most common types to convert /// are to and from a string object. The default implementation will make a call /// to ToString on the object if the object is valid and if the destination /// type is string. If this cannot convert to the desitnation type, this will /// throw a NotSupportedException. /// public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(InstanceDescriptor) && value is ListViewItem) { ListViewItem item = (ListViewItem)value; ConstructorInfo ctor; // Should we use the subitem constructor? // for(int i=1; i < item.SubItems.Count; ++i) { if (item.SubItems[i].CustomStyle) { if (!String.IsNullOrEmpty(item.ImageKey)) { ctor = typeof(ListViewItem).GetConstructor(new Type[] { typeof(ListViewItem.ListViewSubItem[]), typeof(string)}); if (ctor != null) { ListViewItem.ListViewSubItem[] subItemArray = new ListViewItem.ListViewSubItem[item.SubItems.Count]; ((ICollection)item.SubItems).CopyTo(subItemArray, 0); return new InstanceDescriptor(ctor, new object[] {subItemArray, item.ImageKey}, false); } else { break; } } else { ctor = typeof(ListViewItem).GetConstructor(new Type[] { typeof(ListViewItem.ListViewSubItem[]), typeof(int)}); if (ctor != null) { ListViewItem.ListViewSubItem[] subItemArray = new ListViewItem.ListViewSubItem[item.SubItems.Count]; ((ICollection)item.SubItems).CopyTo(subItemArray, 0); return new InstanceDescriptor(ctor, new object[] {subItemArray, item.ImageIndex}, false); } else { break; } } } } // Convert SubItem array to string array // string[] subItems = new string[item.SubItems.Count]; for(int i=0; i < subItems.Length; ++i) { subItems[i] = item.SubItems[i].Text; } // ForeColor, BackColor or ItemFont set // if (item.SubItems[0].CustomStyle) { if (!String.IsNullOrEmpty(item.ImageKey)) { ctor = typeof(ListViewItem).GetConstructor(new Type[] { typeof(string[]), typeof(string), typeof(Color), typeof(Color), typeof(Font)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] { subItems, item.ImageKey, item.SubItems[0].CustomForeColor ? item.ForeColor : Color.Empty, item.SubItems[0].CustomBackColor ? item.BackColor : Color.Empty, item.SubItems[0].CustomFont ? item.Font : null }, false); } } else { ctor = typeof(ListViewItem).GetConstructor(new Type[] { typeof(string[]), typeof(int), typeof(Color), typeof(Color), typeof(Font)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] { subItems, item.ImageIndex, item.SubItems[0].CustomForeColor ? item.ForeColor : Color.Empty, item.SubItems[0].CustomBackColor ? item.BackColor : Color.Empty, item.SubItems[0].CustomFont ? item.Font : null }, false); } } } // Text // if (item.ImageIndex == -1 && String.IsNullOrEmpty(item.ImageKey) && item.SubItems.Count <= 1) { ctor = typeof(ListViewItem).GetConstructor(new Type[] {typeof(string)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] {item.Text}, false); } } // Text and Image // if (item.SubItems.Count <= 1) { if (!String.IsNullOrEmpty(item.ImageKey)) { ctor = typeof(ListViewItem).GetConstructor(new Type[] { typeof(string), typeof(string)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] {item.Text, item.ImageKey}, false); } } else { ctor = typeof(ListViewItem).GetConstructor(new Type[] { typeof(string), typeof(int)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] {item.Text, item.ImageIndex}, false); } } } // Text, Image and SubItems // if (!String.IsNullOrEmpty(item.ImageKey)) { ctor = typeof(ListViewItem).GetConstructor(new Type[] { typeof(string[]), typeof(string)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] {subItems, item.ImageKey}, false); } } else { ctor = typeof(ListViewItem).GetConstructor(new Type[] { typeof(string[]), typeof(int)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] {subItems, item.ImageIndex}, false); } } } return base.ConvertTo(context, culture, value, destinationType); } } internal class ListViewSubItemConverter : ExpandableObjectConverter { public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(InstanceDescriptor)) { return true; } return base.CanConvertTo(context, destinationType); } public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType == null) { throw new ArgumentNullException("destinationType"); } if (destinationType == typeof(InstanceDescriptor) && value is ListViewItem.ListViewSubItem) { ListViewItem.ListViewSubItem item = (ListViewItem.ListViewSubItem)value; ConstructorInfo ctor; // Subitem has custom style // if (item.CustomStyle) { ctor = typeof(ListViewItem.ListViewSubItem).GetConstructor(new Type[] { typeof(ListViewItem), typeof(string), typeof(Color), typeof(Color), typeof(Font)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] { null, item.Text, item.ForeColor, item.BackColor, item.Font}, true); } } // Otherwise, just use the text constructor // ctor = typeof(ListViewItem.ListViewSubItem).GetConstructor(new Type[] {typeof(ListViewItem), typeof(string)}); if (ctor != null) { return new InstanceDescriptor(ctor, new object[] {null, item.Text}, true); } } return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
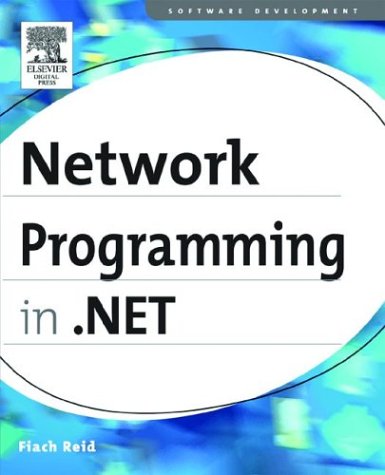
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectContext.cs
- SqlDataSourceQuery.cs
- ToolStripLabel.cs
- ClaimTypeRequirement.cs
- Peer.cs
- infer.cs
- PointCollection.cs
- WasNotInstalledException.cs
- Encoder.cs
- __ConsoleStream.cs
- EncryptedType.cs
- ExecutorLocksHeldException.cs
- Identity.cs
- WmlTextViewAdapter.cs
- MachineKeySection.cs
- StandardCommands.cs
- CqlLexerHelpers.cs
- RepeaterItemEventArgs.cs
- Lease.cs
- TagPrefixAttribute.cs
- IndicFontClient.cs
- SyntaxCheck.cs
- ImpersonateTokenRef.cs
- PostBackOptions.cs
- AlphabeticalEnumConverter.cs
- LinearKeyFrames.cs
- TiffBitmapEncoder.cs
- NetworkStream.cs
- CryptographicAttribute.cs
- DataTemplate.cs
- XmlNamespaceDeclarationsAttribute.cs
- ExpressionCopier.cs
- BmpBitmapEncoder.cs
- SqlDeflator.cs
- XmlDownloadManager.cs
- Translator.cs
- SiteMapNodeItem.cs
- XmlSubtreeReader.cs
- BinHexDecoder.cs
- EntityClassGenerator.cs
- CapacityStreamGeometryContext.cs
- TransformerInfo.cs
- EventHandlers.cs
- HashRepartitionStream.cs
- ArgumentNullException.cs
- XmlILOptimizerVisitor.cs
- RegistryPermission.cs
- Transform3DGroup.cs
- GetWinFXPath.cs
- InternalCache.cs
- OdbcInfoMessageEvent.cs
- DataGridViewControlCollection.cs
- XmlWhitespace.cs
- SqlErrorCollection.cs
- SignerInfo.cs
- HandlerMappingMemo.cs
- StringAnimationBase.cs
- ExpressionBindingCollection.cs
- VScrollBar.cs
- Helpers.cs
- OleDbWrapper.cs
- HostExecutionContextManager.cs
- Aggregates.cs
- DbProviderFactoriesConfigurationHandler.cs
- DataSourceCache.cs
- TextBox.cs
- PermissionListSet.cs
- SqlProviderServices.cs
- InstanceData.cs
- FullTextLine.cs
- BrowsableAttribute.cs
- ObjectConverter.cs
- ProfessionalColorTable.cs
- NavigationFailedEventArgs.cs
- While.cs
- ForEachAction.cs
- SizeAnimation.cs
- brushes.cs
- SafePEFileHandle.cs
- AdCreatedEventArgs.cs
- DataSourceXmlElementAttribute.cs
- TextViewSelectionProcessor.cs
- UrlMapping.cs
- Sql8ConformanceChecker.cs
- CorrelationActionMessageFilter.cs
- BinHexEncoding.cs
- SmtpReplyReaderFactory.cs
- AssertSection.cs
- AttachInfo.cs
- TextEmbeddedObject.cs
- Types.cs
- EDesignUtil.cs
- DataControlCommands.cs
- ReceiveActivityDesignerTheme.cs
- UrlMappingCollection.cs
- ClientSettingsStore.cs
- DecimalConstantAttribute.cs
- FacetEnabledSchemaElement.cs
- ConnectionPointConverter.cs
- CommunicationObject.cs