Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / clr / src / BCL / System / Runtime / Remoting / DispatchChannelSink.cs / 1 / DispatchChannelSink.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // File: DispatchChannelSink.cs using System; using System.Collections; using System.IO; using System.Runtime.Remoting; using System.Runtime.Remoting.Messaging; namespace System.Runtime.Remoting.Channels { internal class DispatchChannelSinkProvider : IServerChannelSinkProvider { internal DispatchChannelSinkProvider() { } // DispatchChannelSinkProvider public void GetChannelData(IChannelDataStore channelData) { } public IServerChannelSink CreateSink(IChannelReceiver channel) { return new DispatchChannelSink(); } public IServerChannelSinkProvider Next { get { return null; } set { throw new NotSupportedException(); } } } // class DispatchChannelSinkProvider internal class DispatchChannelSink : IServerChannelSink { internal DispatchChannelSink() { } // DispatchChannelSink public ServerProcessing ProcessMessage(IServerChannelSinkStack sinkStack, IMessage requestMsg, ITransportHeaders requestHeaders, Stream requestStream, out IMessage responseMsg, out ITransportHeaders responseHeaders, out Stream responseStream) { if (requestMsg == null) { throw new ArgumentNullException( "requestMsg", Environment.GetResourceString("Remoting_Channel_DispatchSinkMessageMissing")); } // check arguments if (requestStream != null) { throw new RemotingException( Environment.GetResourceString("Remoting_Channel_DispatchSinkWantsNullRequestStream")); } responseHeaders = null; responseStream = null; return ChannelServices.DispatchMessage(sinkStack, requestMsg, out responseMsg); } // ProcessMessage public void AsyncProcessResponse(IServerResponseChannelSinkStack sinkStack, Object state, IMessage msg, ITransportHeaders headers, Stream stream) { // We never push ourselves to the sink stack, so this won't be called. throw new NotSupportedException(); } // AsyncProcessResponse public Stream GetResponseStream(IServerResponseChannelSinkStack sinkStack, Object state, IMessage msg, ITransportHeaders headers) { // We never push ourselves to the sink stack, so this won't be called. throw new NotSupportedException(); } // GetResponseStream public IServerChannelSink NextChannelSink { get { return null; } } public IDictionary Properties { get { return null; } } } // class DispatchChannelSink } // namespace System.Runtime.Remoting.Channels // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // File: DispatchChannelSink.cs using System; using System.Collections; using System.IO; using System.Runtime.Remoting; using System.Runtime.Remoting.Messaging; namespace System.Runtime.Remoting.Channels { internal class DispatchChannelSinkProvider : IServerChannelSinkProvider { internal DispatchChannelSinkProvider() { } // DispatchChannelSinkProvider public void GetChannelData(IChannelDataStore channelData) { } public IServerChannelSink CreateSink(IChannelReceiver channel) { return new DispatchChannelSink(); } public IServerChannelSinkProvider Next { get { return null; } set { throw new NotSupportedException(); } } } // class DispatchChannelSinkProvider internal class DispatchChannelSink : IServerChannelSink { internal DispatchChannelSink() { } // DispatchChannelSink public ServerProcessing ProcessMessage(IServerChannelSinkStack sinkStack, IMessage requestMsg, ITransportHeaders requestHeaders, Stream requestStream, out IMessage responseMsg, out ITransportHeaders responseHeaders, out Stream responseStream) { if (requestMsg == null) { throw new ArgumentNullException( "requestMsg", Environment.GetResourceString("Remoting_Channel_DispatchSinkMessageMissing")); } // check arguments if (requestStream != null) { throw new RemotingException( Environment.GetResourceString("Remoting_Channel_DispatchSinkWantsNullRequestStream")); } responseHeaders = null; responseStream = null; return ChannelServices.DispatchMessage(sinkStack, requestMsg, out responseMsg); } // ProcessMessage public void AsyncProcessResponse(IServerResponseChannelSinkStack sinkStack, Object state, IMessage msg, ITransportHeaders headers, Stream stream) { // We never push ourselves to the sink stack, so this won't be called. throw new NotSupportedException(); } // AsyncProcessResponse public Stream GetResponseStream(IServerResponseChannelSinkStack sinkStack, Object state, IMessage msg, ITransportHeaders headers) { // We never push ourselves to the sink stack, so this won't be called. throw new NotSupportedException(); } // GetResponseStream public IServerChannelSink NextChannelSink { get { return null; } } public IDictionary Properties { get { return null; } } } // class DispatchChannelSink } // namespace System.Runtime.Remoting.Channels // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
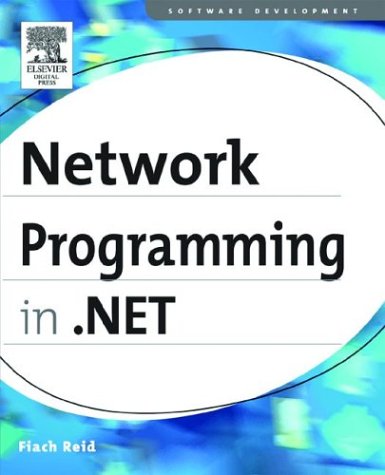
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ServicePoint.cs
- RadioButtonFlatAdapter.cs
- RootBuilder.cs
- MultiTrigger.cs
- DesignerTextWriter.cs
- XmlIgnoreAttribute.cs
- NameValueSectionHandler.cs
- IndicCharClassifier.cs
- PreDigestedSignedInfo.cs
- PathData.cs
- SqlInternalConnectionTds.cs
- ChunkedMemoryStream.cs
- WebException.cs
- InvokeProviderWrapper.cs
- StyleModeStack.cs
- ProfileProvider.cs
- sqlmetadatafactory.cs
- RowsCopiedEventArgs.cs
- TransactionContextValidator.cs
- DataGridView.cs
- ComponentChangingEvent.cs
- DataBinding.cs
- Token.cs
- RemotingHelper.cs
- BamlRecords.cs
- CustomTrackingQuery.cs
- NodeLabelEditEvent.cs
- ParagraphVisual.cs
- SQLGuid.cs
- CodeValidator.cs
- RuntimeHandles.cs
- HttpCacheVary.cs
- EntityDataSourceState.cs
- ConfigsHelper.cs
- TextElementAutomationPeer.cs
- ZipIOCentralDirectoryDigitalSignature.cs
- DataReaderContainer.cs
- SmiXetterAccessMap.cs
- HMACSHA384.cs
- ViewEvent.cs
- ParagraphResult.cs
- GridViewDeleteEventArgs.cs
- OleDbEnumerator.cs
- OleDbRowUpdatedEvent.cs
- PaperSize.cs
- RequestCachePolicy.cs
- InheritanceAttribute.cs
- Bitmap.cs
- ThicknessKeyFrameCollection.cs
- ChtmlTextWriter.cs
- TableItemPatternIdentifiers.cs
- XamlStyleSerializer.cs
- SafeThreadHandle.cs
- DebugView.cs
- CheckBoxField.cs
- RelatedCurrencyManager.cs
- OptimalTextSource.cs
- AggregateNode.cs
- util.cs
- KnownBoxes.cs
- UnSafeCharBuffer.cs
- Code.cs
- TablePattern.cs
- PropertyChangeTracker.cs
- BoundingRectTracker.cs
- PassportPrincipal.cs
- HttpSessionStateWrapper.cs
- ItemMap.cs
- AssertHelper.cs
- Drawing.cs
- BitmapEffectGeneralTransform.cs
- EntitySqlQueryCacheKey.cs
- ProcessHostServerConfig.cs
- UInt16Storage.cs
- SqlMethodTransformer.cs
- WindowsFormsEditorServiceHelper.cs
- DoubleAnimationUsingKeyFrames.cs
- OpenTypeLayout.cs
- mactripleDES.cs
- EntitySqlQueryCacheKey.cs
- Mapping.cs
- SerialPinChanges.cs
- ApplicationHost.cs
- GetPageNumberCompletedEventArgs.cs
- ListViewDeletedEventArgs.cs
- CancelEventArgs.cs
- BamlLocalizableResource.cs
- RoutedUICommand.cs
- DecimalAnimationUsingKeyFrames.cs
- MetadataItemEmitter.cs
- PlatformCulture.cs
- ParameterCollection.cs
- Location.cs
- TypedElement.cs
- SelectionPattern.cs
- Models.cs
- Table.cs
- BinaryCommonClasses.cs
- ObjectStateManager.cs
- GenericsNotImplementedException.cs