Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / CompMod / System / Collections / Generic / DebugView.cs / 1 / DebugView.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** ** ** Purpose: DebugView class for generic collections ** ** Date: Mar 09, 2004 ** =============================================================================*/ namespace System.Collections.Generic { using System; using System.Security.Permissions; using System.Diagnostics; internal sealed class System_CollectionDebugView{ private ICollection collection; public System_CollectionDebugView(ICollection collection) { if (collection == null) { throw new ArgumentNullException("collection"); } this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class System_QueueDebugView { private Queue queue; public System_QueueDebugView(Queue queue) { if (queue == null) { throw new ArgumentNullException("queue"); } this.queue = queue; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { return queue.ToArray(); } } } internal sealed class System_StackDebugView { private Stack stack; public System_StackDebugView(Stack stack) { if (stack == null) { throw new ArgumentNullException("stack"); } this.stack = stack; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { return stack.ToArray(); } } } internal sealed class System_DictionaryDebugView { private IDictionary dict; public System_DictionaryDebugView(IDictionary dictionary) { if (dictionary == null) throw new ArgumentNullException("dictionary"); this.dict = dictionary; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public KeyValuePair [] Items { get { KeyValuePair [] items = new KeyValuePair [dict.Count]; dict.CopyTo(items, 0); return items; } } } internal sealed class System_DictionaryKeyCollectionDebugView { private ICollection collection; public System_DictionaryKeyCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TKey[] Items { get { TKey[] items = new TKey[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class System_DictionaryValueCollectionDebugView { private ICollection collection; public System_DictionaryValueCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TValue[] Items { get { TValue[] items = new TValue[collection.Count]; collection.CopyTo(items, 0); return items; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================================== ** ** ** ** Purpose: DebugView class for generic collections ** ** Date: Mar 09, 2004 ** =============================================================================*/ namespace System.Collections.Generic { using System; using System.Security.Permissions; using System.Diagnostics; internal sealed class System_CollectionDebugView { private ICollection collection; public System_CollectionDebugView(ICollection collection) { if (collection == null) { throw new ArgumentNullException("collection"); } this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { T[] items = new T[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class System_QueueDebugView { private Queue queue; public System_QueueDebugView(Queue queue) { if (queue == null) { throw new ArgumentNullException("queue"); } this.queue = queue; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { return queue.ToArray(); } } } internal sealed class System_StackDebugView { private Stack stack; public System_StackDebugView(Stack stack) { if (stack == null) { throw new ArgumentNullException("stack"); } this.stack = stack; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public T[] Items { get { return stack.ToArray(); } } } internal sealed class System_DictionaryDebugView { private IDictionary dict; public System_DictionaryDebugView(IDictionary dictionary) { if (dictionary == null) throw new ArgumentNullException("dictionary"); this.dict = dictionary; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public KeyValuePair [] Items { get { KeyValuePair [] items = new KeyValuePair [dict.Count]; dict.CopyTo(items, 0); return items; } } } internal sealed class System_DictionaryKeyCollectionDebugView { private ICollection collection; public System_DictionaryKeyCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TKey[] Items { get { TKey[] items = new TKey[collection.Count]; collection.CopyTo(items, 0); return items; } } } internal sealed class System_DictionaryValueCollectionDebugView { private ICollection collection; public System_DictionaryValueCollectionDebugView(ICollection collection) { if (collection == null) ThrowHelper.ThrowArgumentNullException(ExceptionArgument.collection); this.collection = collection; } [DebuggerBrowsable(DebuggerBrowsableState.RootHidden)] public TValue[] Items { get { TValue[] items = new TValue[collection.Count]; collection.CopyTo(items, 0); return items; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
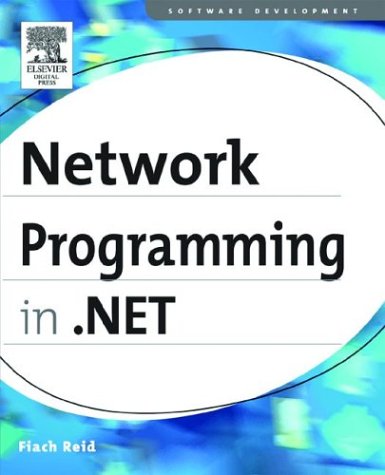
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CharAnimationBase.cs
- ExpressionEditorAttribute.cs
- HttpResponseInternalWrapper.cs
- PropertyTabAttribute.cs
- CellParaClient.cs
- SoapEnumAttribute.cs
- Preprocessor.cs
- PermissionToken.cs
- ColumnCollection.cs
- DateTimeFormatInfo.cs
- BitmapEffectState.cs
- HandlerBase.cs
- ComboBoxRenderer.cs
- SafeArrayRankMismatchException.cs
- ConfigurationStrings.cs
- Walker.cs
- ReferentialConstraintRoleElement.cs
- MergeFailedEvent.cs
- WebPartZoneBase.cs
- SqlXmlStorage.cs
- ExpressionPrinter.cs
- ToolZone.cs
- UnionCqlBlock.cs
- AsymmetricAlgorithm.cs
- HttpProxyCredentialType.cs
- SpellerError.cs
- ExtentCqlBlock.cs
- AutomationEvent.cs
- CodeSnippetStatement.cs
- SoapSchemaImporter.cs
- WebPartConnectVerb.cs
- PropertyDescriptor.cs
- SessionEndingEventArgs.cs
- XPathNode.cs
- UnSafeCharBuffer.cs
- SamlSecurityToken.cs
- SID.cs
- ImageFormat.cs
- XPathSingletonIterator.cs
- _SslStream.cs
- StrongNameUtility.cs
- RoleGroup.cs
- Vector.cs
- ConfigurationException.cs
- BuilderPropertyEntry.cs
- WebPartAuthorizationEventArgs.cs
- PerformanceCountersElement.cs
- OdbcRowUpdatingEvent.cs
- ListInitExpression.cs
- HtmlToClrEventProxy.cs
- ITextView.cs
- TemplateParser.cs
- TypefaceMetricsCache.cs
- ActivityDesignerLayoutSerializers.cs
- SpellerError.cs
- UrlAuthorizationModule.cs
- ExpressionBuilderContext.cs
- SplitterCancelEvent.cs
- DataGrid.cs
- Button.cs
- StrokeSerializer.cs
- ListDictionaryInternal.cs
- TextProperties.cs
- WindowsClientElement.cs
- NativeMethods.cs
- DescriptionAttribute.cs
- OpenFileDialog.cs
- TemplateControl.cs
- ExpressionBuilder.cs
- ShapingWorkspace.cs
- FrameworkTemplate.cs
- XmlAttributeAttribute.cs
- PolicyUnit.cs
- HTTP_SERVICE_CONFIG_URLACL_PARAM.cs
- DataListItem.cs
- Win32Native.cs
- GradientStop.cs
- MouseOverProperty.cs
- BufferedGraphicsContext.cs
- DerivedKeyCachingSecurityTokenSerializer.cs
- CheckBoxField.cs
- OptimizerPatterns.cs
- SpotLight.cs
- XmlDeclaration.cs
- COM2FontConverter.cs
- Command.cs
- TextModifierScope.cs
- AccessKeyManager.cs
- WorkflowWebHostingModule.cs
- ViewEvent.cs
- ArrayEditor.cs
- FormClosedEvent.cs
- AtlasWeb.Designer.cs
- WebConfigurationHost.cs
- TimeSpanSecondsConverter.cs
- InvalidFilterCriteriaException.cs
- DebugHandleTracker.cs
- ClientBuildManager.cs
- ControlCommandSet.cs
- Walker.cs