Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Ast / ListInitExpression.cs / 1305376 / ListInitExpression.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Dynamic.Utils; using System.Reflection; using System.Runtime.CompilerServices; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ////// Represents a constructor call that has a collection initializer. /// ////// Use the #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.ListInitExpressionProxy))] #endif public sealed class ListInitExpression : Expression { private readonly NewExpression _newExpression; private readonly ReadOnlyCollectionfactory methods to create a ListInitExpression. /// The value of the NodeType property of a ListInitExpression is ListInit. /// _initializers; internal ListInitExpression(NewExpression newExpression, ReadOnlyCollection initializers) { _newExpression = newExpression; _initializers = initializers; } /// /// Returns the node type of this ///. (Inherited from .) /// The public sealed override ExpressionType NodeType { get { return ExpressionType.ListInit; } } ///that represents this expression. /// Gets the static type of the expression that this ///represents. (Inherited from .) /// The public sealed override Type Type { get { return _newExpression.Type; } } ///that represents the static type of the expression. /// Gets a value that indicates whether the expression tree node can be reduced. /// public override bool CanReduce { get { return true; } } ////// Gets the expression that contains a call to the constructor of a collection type. /// public NewExpression NewExpression { get { return _newExpression; } } ////// Gets the element initializers that are used to initialize a collection. /// public ReadOnlyCollectionInitializers { get { return _initializers; } } /// /// Dispatches to the specific visit method for this node type. /// protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitListInit(this); } ////// Reduces the binary expression node to a simpler expression. /// If CanReduce returns true, this should return a valid expression. /// This method is allowed to return another node which itself /// must be reduced. /// ///The reduced expression. public override Expression Reduce() { return MemberInitExpression.ReduceListInit(_newExpression, _initializers, true); } ////// Creates a new expression that is like this one, but using the /// supplied children. If all of the children are the same, it will /// return this expression. /// /// Theproperty of the result. /// The property of the result. /// This expression if no children changed, or an expression with the updated children. public ListInitExpression Update(NewExpression newExpression, IEnumerableinitializers) { if (newExpression == NewExpression && initializers == Initializers) { return this; } return Expression.ListInit(newExpression, initializers); } } public partial class Expression { /// /// Creates a /// Athat uses a method named "Add" to add elements to a collection. /// to set the property equal to. /// An array of objects to use to populate the collection. /// A public static ListInitExpression ListInit(NewExpression newExpression, params Expression[] initializers) { ContractUtils.RequiresNotNull(newExpression, "newExpression"); ContractUtils.RequiresNotNull(initializers, "initializers"); return ListInit(newExpression, initializers as IEnumerablethat has the property equal to ListInit and the property set to the specified value. ); } /// /// Creates a /// Athat uses a method named "Add" to add elements to a collection. /// to set the property equal to. /// An that contains objects to use to populate the collection. /// A public static ListInitExpression ListInit(NewExpression newExpression, IEnumerablethat has the property equal to ListInit and the property set to the specified value. initializers) { ContractUtils.RequiresNotNull(newExpression, "newExpression"); ContractUtils.RequiresNotNull(initializers, "initializers"); var initializerlist = initializers.ToReadOnly(); if (initializerlist.Count == 0) { throw Error.ListInitializerWithZeroMembers(); } MethodInfo addMethod = FindMethod(newExpression.Type, "Add", null, new Expression[] { initializerlist[0] }, BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic); return ListInit(newExpression, addMethod, initializers); } /// /// Creates a /// Athat uses a specified method to add elements to a collection. /// to set the property equal to. /// A that represents an instance method named "Add" (case insensitive), that adds an element to a collection. /// An array of objects to use to populate the collection. /// A public static ListInitExpression ListInit(NewExpression newExpression, MethodInfo addMethod, params Expression[] initializers) { if (addMethod == null) { return ListInit(newExpression, initializers as IEnumerablethat has the property equal to ListInit and the property set to the specified value. ); } ContractUtils.RequiresNotNull(newExpression, "newExpression"); ContractUtils.RequiresNotNull(initializers, "initializers"); return ListInit(newExpression, addMethod, initializers as IEnumerable ); } /// /// Creates a /// Athat uses a specified method to add elements to a collection. /// to set the property equal to. /// A that represents an instance method named "Add" (case insensitive), that adds an element to a collection. /// An that contains objects to use to populate the Initializers collection. /// A public static ListInitExpression ListInit(NewExpression newExpression, MethodInfo addMethod, IEnumerablethat has the property equal to ListInit and the property set to the specified value. initializers) { if (addMethod == null) { return ListInit(newExpression, initializers); } ContractUtils.RequiresNotNull(newExpression, "newExpression"); ContractUtils.RequiresNotNull(initializers, "initializers"); var initializerlist = initializers.ToReadOnly(); if (initializerlist.Count == 0) { throw Error.ListInitializerWithZeroMembers(); } ElementInit[] initList = new ElementInit[initializerlist.Count]; for (int i = 0; i < initializerlist.Count; i++) { initList[i] = ElementInit(addMethod, initializerlist[i]); } return ListInit(newExpression, new TrueReadOnlyCollection (initList)); } /// /// Creates a /// Athat uses specified objects to initialize a collection. /// to set the property equal to. /// An array that contains objects to use to populate the collection. /// /// A ///that has the property equal to ListInit /// and the and properties set to the specified values. /// /// The public static ListInitExpression ListInit(NewExpression newExpression, params ElementInit[] initializers) { return ListInit(newExpression, (IEnumerableproperty of must represent a type that implements . /// The property of the resulting is equal to newExpression.Type. /// )initializers); } /// /// Creates a /// Athat uses specified objects to initialize a collection. /// to set the property equal to. /// An that contains objects to use to populate the collection. /// An ///that contains objects to use to populate the collection. /// The public static ListInitExpression ListInit(NewExpression newExpression, IEnumerableproperty of must represent a type that implements . /// The property of the resulting is equal to newExpression.Type. /// initializers) { ContractUtils.RequiresNotNull(newExpression, "newExpression"); ContractUtils.RequiresNotNull(initializers, "initializers"); var initializerlist = initializers.ToReadOnly(); if (initializerlist.Count == 0) { throw Error.ListInitializerWithZeroMembers(); } ValidateListInitArgs(newExpression.Type, initializerlist); return new ListInitExpression(newExpression, initializerlist); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics; using System.Dynamic.Utils; using System.Reflection; using System.Runtime.CompilerServices; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { /// /// Represents a constructor call that has a collection initializer. /// ////// Use the #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.ListInitExpressionProxy))] #endif public sealed class ListInitExpression : Expression { private readonly NewExpression _newExpression; private readonly ReadOnlyCollectionfactory methods to create a ListInitExpression. /// The value of the NodeType property of a ListInitExpression is ListInit. /// _initializers; internal ListInitExpression(NewExpression newExpression, ReadOnlyCollection initializers) { _newExpression = newExpression; _initializers = initializers; } /// /// Returns the node type of this ///. (Inherited from .) /// The public sealed override ExpressionType NodeType { get { return ExpressionType.ListInit; } } ///that represents this expression. /// Gets the static type of the expression that this ///represents. (Inherited from .) /// The public sealed override Type Type { get { return _newExpression.Type; } } ///that represents the static type of the expression. /// Gets a value that indicates whether the expression tree node can be reduced. /// public override bool CanReduce { get { return true; } } ////// Gets the expression that contains a call to the constructor of a collection type. /// public NewExpression NewExpression { get { return _newExpression; } } ////// Gets the element initializers that are used to initialize a collection. /// public ReadOnlyCollectionInitializers { get { return _initializers; } } /// /// Dispatches to the specific visit method for this node type. /// protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitListInit(this); } ////// Reduces the binary expression node to a simpler expression. /// If CanReduce returns true, this should return a valid expression. /// This method is allowed to return another node which itself /// must be reduced. /// ///The reduced expression. public override Expression Reduce() { return MemberInitExpression.ReduceListInit(_newExpression, _initializers, true); } ////// Creates a new expression that is like this one, but using the /// supplied children. If all of the children are the same, it will /// return this expression. /// /// Theproperty of the result. /// The property of the result. /// This expression if no children changed, or an expression with the updated children. public ListInitExpression Update(NewExpression newExpression, IEnumerableinitializers) { if (newExpression == NewExpression && initializers == Initializers) { return this; } return Expression.ListInit(newExpression, initializers); } } public partial class Expression { /// /// Creates a /// Athat uses a method named "Add" to add elements to a collection. /// to set the property equal to. /// An array of objects to use to populate the collection. /// A public static ListInitExpression ListInit(NewExpression newExpression, params Expression[] initializers) { ContractUtils.RequiresNotNull(newExpression, "newExpression"); ContractUtils.RequiresNotNull(initializers, "initializers"); return ListInit(newExpression, initializers as IEnumerablethat has the property equal to ListInit and the property set to the specified value. ); } /// /// Creates a /// Athat uses a method named "Add" to add elements to a collection. /// to set the property equal to. /// An that contains objects to use to populate the collection. /// A public static ListInitExpression ListInit(NewExpression newExpression, IEnumerablethat has the property equal to ListInit and the property set to the specified value. initializers) { ContractUtils.RequiresNotNull(newExpression, "newExpression"); ContractUtils.RequiresNotNull(initializers, "initializers"); var initializerlist = initializers.ToReadOnly(); if (initializerlist.Count == 0) { throw Error.ListInitializerWithZeroMembers(); } MethodInfo addMethod = FindMethod(newExpression.Type, "Add", null, new Expression[] { initializerlist[0] }, BindingFlags.Instance | BindingFlags.Public | BindingFlags.NonPublic); return ListInit(newExpression, addMethod, initializers); } /// /// Creates a /// Athat uses a specified method to add elements to a collection. /// to set the property equal to. /// A that represents an instance method named "Add" (case insensitive), that adds an element to a collection. /// An array of objects to use to populate the collection. /// A public static ListInitExpression ListInit(NewExpression newExpression, MethodInfo addMethod, params Expression[] initializers) { if (addMethod == null) { return ListInit(newExpression, initializers as IEnumerablethat has the property equal to ListInit and the property set to the specified value. ); } ContractUtils.RequiresNotNull(newExpression, "newExpression"); ContractUtils.RequiresNotNull(initializers, "initializers"); return ListInit(newExpression, addMethod, initializers as IEnumerable ); } /// /// Creates a /// Athat uses a specified method to add elements to a collection. /// to set the property equal to. /// A that represents an instance method named "Add" (case insensitive), that adds an element to a collection. /// An that contains objects to use to populate the Initializers collection. /// A public static ListInitExpression ListInit(NewExpression newExpression, MethodInfo addMethod, IEnumerablethat has the property equal to ListInit and the property set to the specified value. initializers) { if (addMethod == null) { return ListInit(newExpression, initializers); } ContractUtils.RequiresNotNull(newExpression, "newExpression"); ContractUtils.RequiresNotNull(initializers, "initializers"); var initializerlist = initializers.ToReadOnly(); if (initializerlist.Count == 0) { throw Error.ListInitializerWithZeroMembers(); } ElementInit[] initList = new ElementInit[initializerlist.Count]; for (int i = 0; i < initializerlist.Count; i++) { initList[i] = ElementInit(addMethod, initializerlist[i]); } return ListInit(newExpression, new TrueReadOnlyCollection (initList)); } /// /// Creates a /// Athat uses specified objects to initialize a collection. /// to set the property equal to. /// An array that contains objects to use to populate the collection. /// /// A ///that has the property equal to ListInit /// and the and properties set to the specified values. /// /// The public static ListInitExpression ListInit(NewExpression newExpression, params ElementInit[] initializers) { return ListInit(newExpression, (IEnumerableproperty of must represent a type that implements . /// The property of the resulting is equal to newExpression.Type. /// )initializers); } /// /// Creates a /// Athat uses specified objects to initialize a collection. /// to set the property equal to. /// An that contains objects to use to populate the collection. /// An ///that contains objects to use to populate the collection. /// The public static ListInitExpression ListInit(NewExpression newExpression, IEnumerableproperty of must represent a type that implements . /// The property of the resulting is equal to newExpression.Type. /// initializers) { ContractUtils.RequiresNotNull(newExpression, "newExpression"); ContractUtils.RequiresNotNull(initializers, "initializers"); var initializerlist = initializers.ToReadOnly(); if (initializerlist.Count == 0) { throw Error.ListInitializerWithZeroMembers(); } ValidateListInitArgs(newExpression.Type, initializerlist); return new ListInitExpression(newExpression, initializerlist); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
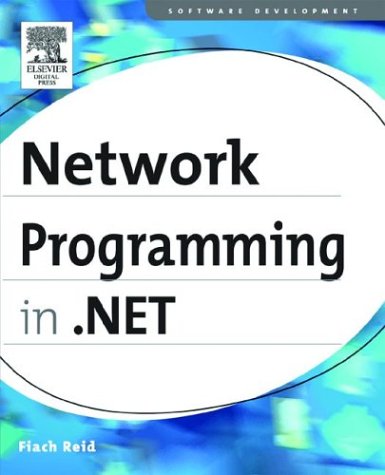
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AdornerHitTestResult.cs
- Deserializer.cs
- ClientConfigPaths.cs
- SoapClientMessage.cs
- CookielessHelper.cs
- Rfc2898DeriveBytes.cs
- ProfileService.cs
- DispatcherEventArgs.cs
- ProcessManager.cs
- ManipulationLogic.cs
- StylusPointDescription.cs
- PagesChangedEventArgs.cs
- TransformDescriptor.cs
- ControlType.cs
- AutoGeneratedFieldProperties.cs
- Preprocessor.cs
- InternalControlCollection.cs
- DrawingGroup.cs
- MD5.cs
- PartitionedStream.cs
- WorkflowTimerService.cs
- SqlDependencyListener.cs
- ObjectDataSourceView.cs
- InheritablePropertyChangeInfo.cs
- NetworkInformationPermission.cs
- Transform.cs
- DataObjectAttribute.cs
- BCLDebug.cs
- XmlNodeComparer.cs
- PageTheme.cs
- MemberProjectionIndex.cs
- CacheDependency.cs
- DbExpressionBuilder.cs
- KeyedCollection.cs
- ProxyHwnd.cs
- StringUtil.cs
- TextSpan.cs
- UnhandledExceptionEventArgs.cs
- MaterialCollection.cs
- WSHttpBindingBase.cs
- NullableDecimalAverageAggregationOperator.cs
- StreamFormatter.cs
- StackSpiller.Bindings.cs
- ListParagraph.cs
- RunWorkerCompletedEventArgs.cs
- SqlCrossApplyToCrossJoin.cs
- securitycriticaldataClass.cs
- UIAgentAsyncBeginRequest.cs
- HostedTransportConfigurationBase.cs
- RegexCapture.cs
- DataChangedEventManager.cs
- HtmlGenericControl.cs
- MultiByteCodec.cs
- thaishape.cs
- ClientTarget.cs
- PageTheme.cs
- CompiledIdentityConstraint.cs
- CodeDomSerializer.cs
- OleDbDataReader.cs
- UnauthorizedWebPart.cs
- HtmlInputCheckBox.cs
- DependentList.cs
- QilPatternFactory.cs
- RequestCachingSection.cs
- ExeConfigurationFileMap.cs
- IntegerFacetDescriptionElement.cs
- TemplateBindingExpressionConverter.cs
- ContainerAction.cs
- Msec.cs
- FloaterBaseParagraph.cs
- HtmlWindow.cs
- MissingFieldException.cs
- SqlCacheDependencyDatabase.cs
- DefaultValueConverter.cs
- ObjectTag.cs
- TrackingProvider.cs
- ConfigurationStrings.cs
- HandledMouseEvent.cs
- ZipIOFileItemStream.cs
- CurrencyManager.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- ZoneIdentityPermission.cs
- DeclaredTypeValidatorAttribute.cs
- GridViewUpdateEventArgs.cs
- ReferencedType.cs
- SizeIndependentAnimationStorage.cs
- RectValueSerializer.cs
- QueryStringParameter.cs
- ObjectCloneHelper.cs
- SimpleBitVector32.cs
- ISFTagAndGuidCache.cs
- ImageInfo.cs
- TaiwanCalendar.cs
- WebControl.cs
- WorkflowNamespace.cs
- DataGridViewComboBoxCell.cs
- SafeMarshalContext.cs
- Soap12ProtocolImporter.cs
- DesignerDeviceConfig.cs
- DocumentXmlWriter.cs