Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Web / System / Web / Services / Description / Soap12ProtocolImporter.cs / 1305376 / Soap12ProtocolImporter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Web.Services.Description { using System.Web.Services; using System.Web.Services.Protocols; using System.Xml; using System.Xml.Serialization; using System.Xml.Schema; using System.Collections; using System; using System.Reflection; using System.CodeDom; using System.Web.Services.Configuration; using System.Diagnostics; using System.ComponentModel; using System.Security.Permissions; using System.Globalization; ///[PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] internal class Soap12ProtocolImporter : SoapProtocolImporter { public override string ProtocolName { get { return "Soap12"; } } protected override bool IsBindingSupported() { Soap12Binding soapBinding = (Soap12Binding)Binding.Extensions.Find(typeof(Soap12Binding)); if (soapBinding == null) return false; if (GetTransport(soapBinding.Transport) == null) { UnsupportedBindingWarning(Res.GetString(Res.ThereIsNoSoapTransportImporterThatUnderstands1, soapBinding.Transport)); return false; } return true; } protected override bool IsSoapEncodingPresent(string uriList) { int iStart = 0; do { iStart = uriList.IndexOf(Soap12.Encoding, iStart, StringComparison.Ordinal); if (iStart < 0) break; int iEnd = iStart + Soap12.Encoding.Length; if (iStart == 0 || uriList[iStart-1] == ' ') if (iEnd == uriList.Length || uriList[iEnd] == ' ') return true; iStart = iEnd; } while (iStart < uriList.Length); // not soap 1.2 encoding. let's detect the soap 1.1 encoding and give a better error message. // otherwise just default to the normal "encoding style not supported" error. if (base.IsSoapEncodingPresent(uriList)) UnsupportedOperationBindingWarning(Res.GetString(Res.WebSoap11EncodingStyleNotSupported1, Soap12.Encoding)); return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All Rights Reserved. // Information Contained Herein is Proprietary and Confidential. // //----------------------------------------------------------------------------- namespace System.Web.Services.Description { using System.Web.Services; using System.Web.Services.Protocols; using System.Xml; using System.Xml.Serialization; using System.Xml.Schema; using System.Collections; using System; using System.Reflection; using System.CodeDom; using System.Web.Services.Configuration; using System.Diagnostics; using System.ComponentModel; using System.Security.Permissions; using System.Globalization; ///[PermissionSet(SecurityAction.LinkDemand, Name="FullTrust")] internal class Soap12ProtocolImporter : SoapProtocolImporter { public override string ProtocolName { get { return "Soap12"; } } protected override bool IsBindingSupported() { Soap12Binding soapBinding = (Soap12Binding)Binding.Extensions.Find(typeof(Soap12Binding)); if (soapBinding == null) return false; if (GetTransport(soapBinding.Transport) == null) { UnsupportedBindingWarning(Res.GetString(Res.ThereIsNoSoapTransportImporterThatUnderstands1, soapBinding.Transport)); return false; } return true; } protected override bool IsSoapEncodingPresent(string uriList) { int iStart = 0; do { iStart = uriList.IndexOf(Soap12.Encoding, iStart, StringComparison.Ordinal); if (iStart < 0) break; int iEnd = iStart + Soap12.Encoding.Length; if (iStart == 0 || uriList[iStart-1] == ' ') if (iEnd == uriList.Length || uriList[iEnd] == ' ') return true; iStart = iEnd; } while (iStart < uriList.Length); // not soap 1.2 encoding. let's detect the soap 1.1 encoding and give a better error message. // otherwise just default to the normal "encoding style not supported" error. if (base.IsSoapEncodingPresent(uriList)) UnsupportedOperationBindingWarning(Res.GetString(Res.WebSoap11EncodingStyleNotSupported1, Soap12.Encoding)); return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
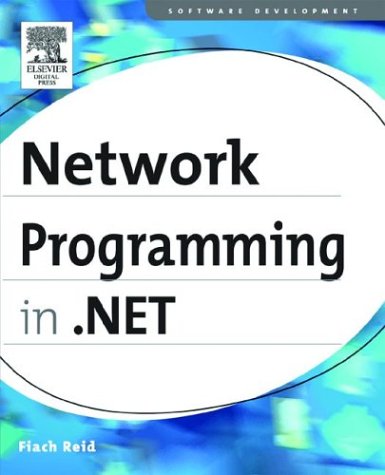
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ValidationSummary.cs
- Model3D.cs
- EdmItemError.cs
- DataControlFieldCell.cs
- SafeCryptHandles.cs
- IgnoreFlushAndCloseStream.cs
- SineEase.cs
- OracleTimeSpan.cs
- baseaxisquery.cs
- GeneralTransform2DTo3D.cs
- SmtpLoginAuthenticationModule.cs
- ComplexObject.cs
- MultilineStringConverter.cs
- StaticResourceExtension.cs
- IndependentAnimationStorage.cs
- OracleConnectionString.cs
- FilterEventArgs.cs
- NonVisualControlAttribute.cs
- DataListItem.cs
- HttpTransportSecurity.cs
- BufferModesCollection.cs
- WindowsListViewItemCheckBox.cs
- ObjectReaderCompiler.cs
- DesignerCapabilities.cs
- Line.cs
- SchemaImporterExtensionElement.cs
- EventManager.cs
- InternalReceiveMessage.cs
- ToolBarButton.cs
- SecureEnvironment.cs
- XmlName.cs
- SQlBooleanStorage.cs
- ProfilePropertySettings.cs
- CharAnimationUsingKeyFrames.cs
- CssTextWriter.cs
- ConnectionManagementSection.cs
- HttpListenerContext.cs
- HttpContext.cs
- SynchronizedInputProviderWrapper.cs
- isolationinterop.cs
- Int64AnimationUsingKeyFrames.cs
- PageRanges.cs
- FloaterParagraph.cs
- InputLanguage.cs
- RepeatButton.cs
- MessageVersionConverter.cs
- DaylightTime.cs
- GroupLabel.cs
- RemotingConfiguration.cs
- DispatcherExceptionEventArgs.cs
- Menu.cs
- ObjectContext.cs
- HtmlGenericControl.cs
- ParameterReplacerVisitor.cs
- EntityContainerAssociationSetEnd.cs
- XmlWriter.cs
- VisualCollection.cs
- CodeThrowExceptionStatement.cs
- TextParaLineResult.cs
- ObjectCloneHelper.cs
- HttpListenerException.cs
- cookie.cs
- UniqueEventHelper.cs
- LocalizationComments.cs
- XmlUtil.cs
- Switch.cs
- XsdCachingReader.cs
- GenericQueueSurrogate.cs
- LogSwitch.cs
- VirtualizedItemPattern.cs
- PersonalizableTypeEntry.cs
- Geometry3D.cs
- GroupItem.cs
- OperationAbortedException.cs
- PopupRootAutomationPeer.cs
- StackBuilderSink.cs
- InvokeHandlers.cs
- SeparatorAutomationPeer.cs
- ListDictionaryInternal.cs
- Compress.cs
- _SslStream.cs
- basenumberconverter.cs
- TemplatePagerField.cs
- RegexStringValidator.cs
- ValidationErrorCollection.cs
- messageonlyhwndwrapper.cs
- GeneratedView.cs
- XmlChildEnumerator.cs
- SynchronousChannel.cs
- MarkupExtensionParser.cs
- HotCommands.cs
- XmlSchemaAttributeGroup.cs
- ContentType.cs
- MethodCallConverter.cs
- StructuralObject.cs
- IRCollection.cs
- AppDomain.cs
- PassportIdentity.cs
- MenuTracker.cs
- TableRowCollection.cs