Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / DataOracleClient / System / Data / OracleClient / OracleTimeSpan.cs / 1 / OracleTimeSpan.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data.SqlTypes; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Text; //--------------------------------------------------------------------- // OracleTimeSpan // // This class implements support for the Oracle 9i 'INTERVAL DAY TO SECOND' // internal data type. // [StructLayout(LayoutKind.Sequential, Pack=1)] public struct OracleTimeSpan : IComparable, INullable { private byte[] _value; private const int FractionalSecondsPerTick = 100; public static readonly OracleTimeSpan MaxValue = new OracleTimeSpan(TimeSpan.MaxValue); public static readonly OracleTimeSpan MinValue = new OracleTimeSpan(TimeSpan.MinValue); public static readonly OracleTimeSpan Null = new OracleTimeSpan(true); // Construct from nothing -- the value will be null private OracleTimeSpan(bool isNull) { _value = null; } // Construct from System.TimeSpan type public OracleTimeSpan (TimeSpan ts) { _value = new byte[11]; Pack(_value, ts.Days, ts.Hours, ts.Minutes, ts.Seconds, (int)(ts.Ticks % TimeSpan.TicksPerSecond) * FractionalSecondsPerTick); } public OracleTimeSpan (Int64 ticks) { _value = new byte[11]; TimeSpan ts = new TimeSpan(ticks); Pack(_value, ts.Days, ts.Hours, ts.Minutes, ts.Seconds, (int)(ts.Ticks % TimeSpan.TicksPerSecond) * FractionalSecondsPerTick); } public OracleTimeSpan (Int32 hours, Int32 minutes, Int32 seconds) : this (0, hours, minutes, seconds, 0) {} public OracleTimeSpan (Int32 days, Int32 hours, Int32 minutes, Int32 seconds) : this (days, hours, minutes, seconds, 0) {} public OracleTimeSpan (Int32 days, Int32 hours, Int32 minutes, Int32 seconds, Int32 milliseconds) { _value = new byte[11]; Pack(_value, days, hours, minutes, seconds, (int)(milliseconds * TimeSpan.TicksPerMillisecond) * FractionalSecondsPerTick); } // Copy constructor public OracleTimeSpan (OracleTimeSpan from) { _value = new byte[from._value.Length]; from._value.CopyTo(_value, 0); } // (internal) construct from a row/parameter binding internal OracleTimeSpan (NativeBuffer buffer, int valueOffset) : this (true) { _value = buffer.ReadBytes(valueOffset, 11); } static private void Pack (byte[] spanval, int days, int hours, int minutes, int seconds, int fsecs) { days = (int)((long)(days) + 0x80000000); fsecs = (int)((long)(fsecs) + 0x80000000); // DEVNOTE: undoubtedly, this is Intel byte order specific, but how // do I verify what Oracle needs on a non Intel machine? spanval[0] = (byte)((days >> 24)); spanval[1] = (byte)((days >> 16) & 0xff); spanval[2] = (byte)((days >> 8) & 0xff); spanval[3] = (byte)(days & 0xff); spanval[4] = (byte)(hours + 60); spanval[5] = (byte)(minutes + 60); spanval[6] = (byte)(seconds + 60); spanval[7] = (byte)((fsecs >> 24)); spanval[8] = (byte)((fsecs >> 16) & 0xff); spanval[9] = (byte)((fsecs >> 8) & 0xff); spanval[10]= (byte)(fsecs & 0xff); } static private void Unpack (byte[] spanval, out int days, out int hours, out int minutes, out int seconds, out int fsecs) { // DEVNOTE: undoubtedly, this is Intel byte order specific, but how // do I verify what Oracle needs on a non Intel machine? days = (int)( (long)( (int)spanval[0] << 24 | (int)spanval[1] << 16 | (int)spanval[2] << 8 | (int)spanval[3] ) - 0x80000000); hours = (int)spanval[4] - 60; minutes = (int)spanval[5] - 60; seconds = (int)spanval[6] - 60; fsecs = (int)( (long)( (int)spanval[7] << 24 | (int)spanval[8] << 16 | (int)spanval[9] << 8 | (int)spanval[10] ) - 0x80000000); } public bool IsNull { get { return (null == _value); } } public TimeSpan Value { get { if (IsNull) { throw ADP.DataIsNull(); } TimeSpan result = ToTimeSpan(_value); return result; } } public int Days { get { if (IsNull) { throw ADP.DataIsNull(); } int day, hour, minute, second, fsec; Unpack( _value, out day, out hour, out minute, out second, out fsec); return day; } } public int Hours { get { if (IsNull) { throw ADP.DataIsNull(); } int day, hour, minute, second, fsec; Unpack( _value, out day, out hour, out minute, out second, out fsec); return hour; } } public int Minutes { get { if (IsNull) { throw ADP.DataIsNull(); } int day, hour, minute, second, fsec; Unpack( _value, out day, out hour, out minute, out second, out fsec); return minute; } } public int Seconds { get { if (IsNull) { throw ADP.DataIsNull(); } int day, hour, minute, second, fsec; Unpack( _value, out day, out hour, out minute, out second, out fsec); return second; } } public int Milliseconds { get { if (IsNull) { throw ADP.DataIsNull(); } int day, hour, minute, second, fsec; Unpack( _value, out day, out hour, out minute, out second, out fsec); int milliseconds = (int)((fsec / FractionalSecondsPerTick) / TimeSpan.TicksPerMillisecond); return milliseconds; } } public int CompareTo (object obj) { if (obj.GetType() == typeof(OracleTimeSpan)) { OracleTimeSpan odt = (OracleTimeSpan)obj; // If both values are Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) { return odt.IsNull ? 0 : -1; } if (odt.IsNull) { return 1; } // Neither value is null, do the comparison. int days1, hours1, minutes1, seconds1, fsecs1; int days2, hours2, minutes2, seconds2, fsecs2; Unpack( _value, out days1, out hours1, out minutes1, out seconds1, out fsecs1); Unpack( odt._value, out days2, out hours2, out minutes2, out seconds2, out fsecs2); int delta; delta = (days1 - days2); if (0 != delta) return delta; delta = (hours1 - hours2); if (0 != delta) return delta; delta = (minutes1 - minutes2); if (0 != delta) return delta; delta = (seconds1 - seconds2); if (0 != delta) return delta; delta = (fsecs1 - fsecs2); if (0 != delta) return delta; return 0; } throw ADP.WrongType(obj.GetType(), typeof(OracleTimeSpan)); } public override bool Equals(object value) { if (value is OracleTimeSpan) { return (this == (OracleTimeSpan)value).Value; } else { return false; } } public override int GetHashCode() { return IsNull ? 0 : _value.GetHashCode(); } static internal TimeSpan MarshalToTimeSpan (NativeBuffer buffer, int valueOffset) { byte[] rawValue = buffer.ReadBytes(valueOffset, 11); TimeSpan result = ToTimeSpan(rawValue); return result; } static internal int MarshalToNative (object value, NativeBuffer buffer, int offset) { byte[] from; if ( value is OracleTimeSpan ) { from = ((OracleTimeSpan)value)._value; } else { TimeSpan ts = (TimeSpan)value; from = new byte[11]; Pack(from, ts.Days, ts.Hours, ts.Minutes, ts.Seconds, (int)(ts.Ticks % TimeSpan.TicksPerSecond) * FractionalSecondsPerTick); } buffer.WriteBytes(offset, from, 0, 11); return 11; } public static OracleTimeSpan Parse(string s) { TimeSpan ts = TimeSpan.Parse(s); return new OracleTimeSpan(ts); } public override string ToString() { if (IsNull) { return ADP.NullString; } string retval = Value.ToString(); return retval; } static private TimeSpan ToTimeSpan(byte[] rawValue) { int days, hours, minutes, seconds, fsecs; Unpack( rawValue, out days, out hours, out minutes, out seconds, out fsecs); long tickcount = (days * TimeSpan.TicksPerDay) + (hours * TimeSpan.TicksPerHour) + (minutes * TimeSpan.TicksPerMinute) + (seconds * TimeSpan.TicksPerSecond); if (fsecs < 100 || fsecs > 100) { // DEVNOTE: Yes, there's a mismatch in the precision between Oracle, // (which has 9 digits) and System.TimeSpan (which has 7 // digits); All the other providers truncate the precision, // so we do as well. tickcount += ((long)fsecs / 100); } TimeSpan result = new TimeSpan(tickcount); return result; } //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Operators // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// public static OracleBoolean Equals(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator == return (x == y); } public static OracleBoolean GreaterThan(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator > return (x > y); } public static OracleBoolean GreaterThanOrEqual(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator >= return (x >= y); } public static OracleBoolean LessThan(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator < return (x < y); } public static OracleBoolean LessThanOrEqual(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator <= return (x <= y); } public static OracleBoolean NotEquals(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator != return (x != y); } public static explicit operator TimeSpan(OracleTimeSpan x) { if (x.IsNull) { throw ADP.DataIsNull(); } return x.Value; } public static explicit operator OracleTimeSpan(string x) { return OracleTimeSpan.Parse(x); } public static OracleBoolean operator== (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) == 0); } public static OracleBoolean operator> (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) > 0); } public static OracleBoolean operator>= (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) >= 0); } public static OracleBoolean operator< (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) < 0); } public static OracleBoolean operator<= (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) <= 0); } public static OracleBoolean operator!= (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) != 0); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.Data.SqlTypes; using System.Data.Common; using System.Diagnostics; using System.Globalization; using System.Runtime.InteropServices; using System.Text; //--------------------------------------------------------------------- // OracleTimeSpan // // This class implements support for the Oracle 9i 'INTERVAL DAY TO SECOND' // internal data type. // [StructLayout(LayoutKind.Sequential, Pack=1)] public struct OracleTimeSpan : IComparable, INullable { private byte[] _value; private const int FractionalSecondsPerTick = 100; public static readonly OracleTimeSpan MaxValue = new OracleTimeSpan(TimeSpan.MaxValue); public static readonly OracleTimeSpan MinValue = new OracleTimeSpan(TimeSpan.MinValue); public static readonly OracleTimeSpan Null = new OracleTimeSpan(true); // Construct from nothing -- the value will be null private OracleTimeSpan(bool isNull) { _value = null; } // Construct from System.TimeSpan type public OracleTimeSpan (TimeSpan ts) { _value = new byte[11]; Pack(_value, ts.Days, ts.Hours, ts.Minutes, ts.Seconds, (int)(ts.Ticks % TimeSpan.TicksPerSecond) * FractionalSecondsPerTick); } public OracleTimeSpan (Int64 ticks) { _value = new byte[11]; TimeSpan ts = new TimeSpan(ticks); Pack(_value, ts.Days, ts.Hours, ts.Minutes, ts.Seconds, (int)(ts.Ticks % TimeSpan.TicksPerSecond) * FractionalSecondsPerTick); } public OracleTimeSpan (Int32 hours, Int32 minutes, Int32 seconds) : this (0, hours, minutes, seconds, 0) {} public OracleTimeSpan (Int32 days, Int32 hours, Int32 minutes, Int32 seconds) : this (days, hours, minutes, seconds, 0) {} public OracleTimeSpan (Int32 days, Int32 hours, Int32 minutes, Int32 seconds, Int32 milliseconds) { _value = new byte[11]; Pack(_value, days, hours, minutes, seconds, (int)(milliseconds * TimeSpan.TicksPerMillisecond) * FractionalSecondsPerTick); } // Copy constructor public OracleTimeSpan (OracleTimeSpan from) { _value = new byte[from._value.Length]; from._value.CopyTo(_value, 0); } // (internal) construct from a row/parameter binding internal OracleTimeSpan (NativeBuffer buffer, int valueOffset) : this (true) { _value = buffer.ReadBytes(valueOffset, 11); } static private void Pack (byte[] spanval, int days, int hours, int minutes, int seconds, int fsecs) { days = (int)((long)(days) + 0x80000000); fsecs = (int)((long)(fsecs) + 0x80000000); // DEVNOTE: undoubtedly, this is Intel byte order specific, but how // do I verify what Oracle needs on a non Intel machine? spanval[0] = (byte)((days >> 24)); spanval[1] = (byte)((days >> 16) & 0xff); spanval[2] = (byte)((days >> 8) & 0xff); spanval[3] = (byte)(days & 0xff); spanval[4] = (byte)(hours + 60); spanval[5] = (byte)(minutes + 60); spanval[6] = (byte)(seconds + 60); spanval[7] = (byte)((fsecs >> 24)); spanval[8] = (byte)((fsecs >> 16) & 0xff); spanval[9] = (byte)((fsecs >> 8) & 0xff); spanval[10]= (byte)(fsecs & 0xff); } static private void Unpack (byte[] spanval, out int days, out int hours, out int minutes, out int seconds, out int fsecs) { // DEVNOTE: undoubtedly, this is Intel byte order specific, but how // do I verify what Oracle needs on a non Intel machine? days = (int)( (long)( (int)spanval[0] << 24 | (int)spanval[1] << 16 | (int)spanval[2] << 8 | (int)spanval[3] ) - 0x80000000); hours = (int)spanval[4] - 60; minutes = (int)spanval[5] - 60; seconds = (int)spanval[6] - 60; fsecs = (int)( (long)( (int)spanval[7] << 24 | (int)spanval[8] << 16 | (int)spanval[9] << 8 | (int)spanval[10] ) - 0x80000000); } public bool IsNull { get { return (null == _value); } } public TimeSpan Value { get { if (IsNull) { throw ADP.DataIsNull(); } TimeSpan result = ToTimeSpan(_value); return result; } } public int Days { get { if (IsNull) { throw ADP.DataIsNull(); } int day, hour, minute, second, fsec; Unpack( _value, out day, out hour, out minute, out second, out fsec); return day; } } public int Hours { get { if (IsNull) { throw ADP.DataIsNull(); } int day, hour, minute, second, fsec; Unpack( _value, out day, out hour, out minute, out second, out fsec); return hour; } } public int Minutes { get { if (IsNull) { throw ADP.DataIsNull(); } int day, hour, minute, second, fsec; Unpack( _value, out day, out hour, out minute, out second, out fsec); return minute; } } public int Seconds { get { if (IsNull) { throw ADP.DataIsNull(); } int day, hour, minute, second, fsec; Unpack( _value, out day, out hour, out minute, out second, out fsec); return second; } } public int Milliseconds { get { if (IsNull) { throw ADP.DataIsNull(); } int day, hour, minute, second, fsec; Unpack( _value, out day, out hour, out minute, out second, out fsec); int milliseconds = (int)((fsec / FractionalSecondsPerTick) / TimeSpan.TicksPerMillisecond); return milliseconds; } } public int CompareTo (object obj) { if (obj.GetType() == typeof(OracleTimeSpan)) { OracleTimeSpan odt = (OracleTimeSpan)obj; // If both values are Null, consider them equal. // Otherwise, Null is less than anything. if (IsNull) { return odt.IsNull ? 0 : -1; } if (odt.IsNull) { return 1; } // Neither value is null, do the comparison. int days1, hours1, minutes1, seconds1, fsecs1; int days2, hours2, minutes2, seconds2, fsecs2; Unpack( _value, out days1, out hours1, out minutes1, out seconds1, out fsecs1); Unpack( odt._value, out days2, out hours2, out minutes2, out seconds2, out fsecs2); int delta; delta = (days1 - days2); if (0 != delta) return delta; delta = (hours1 - hours2); if (0 != delta) return delta; delta = (minutes1 - minutes2); if (0 != delta) return delta; delta = (seconds1 - seconds2); if (0 != delta) return delta; delta = (fsecs1 - fsecs2); if (0 != delta) return delta; return 0; } throw ADP.WrongType(obj.GetType(), typeof(OracleTimeSpan)); } public override bool Equals(object value) { if (value is OracleTimeSpan) { return (this == (OracleTimeSpan)value).Value; } else { return false; } } public override int GetHashCode() { return IsNull ? 0 : _value.GetHashCode(); } static internal TimeSpan MarshalToTimeSpan (NativeBuffer buffer, int valueOffset) { byte[] rawValue = buffer.ReadBytes(valueOffset, 11); TimeSpan result = ToTimeSpan(rawValue); return result; } static internal int MarshalToNative (object value, NativeBuffer buffer, int offset) { byte[] from; if ( value is OracleTimeSpan ) { from = ((OracleTimeSpan)value)._value; } else { TimeSpan ts = (TimeSpan)value; from = new byte[11]; Pack(from, ts.Days, ts.Hours, ts.Minutes, ts.Seconds, (int)(ts.Ticks % TimeSpan.TicksPerSecond) * FractionalSecondsPerTick); } buffer.WriteBytes(offset, from, 0, 11); return 11; } public static OracleTimeSpan Parse(string s) { TimeSpan ts = TimeSpan.Parse(s); return new OracleTimeSpan(ts); } public override string ToString() { if (IsNull) { return ADP.NullString; } string retval = Value.ToString(); return retval; } static private TimeSpan ToTimeSpan(byte[] rawValue) { int days, hours, minutes, seconds, fsecs; Unpack( rawValue, out days, out hours, out minutes, out seconds, out fsecs); long tickcount = (days * TimeSpan.TicksPerDay) + (hours * TimeSpan.TicksPerHour) + (minutes * TimeSpan.TicksPerMinute) + (seconds * TimeSpan.TicksPerSecond); if (fsecs < 100 || fsecs > 100) { // DEVNOTE: Yes, there's a mismatch in the precision between Oracle, // (which has 9 digits) and System.TimeSpan (which has 7 // digits); All the other providers truncate the precision, // so we do as well. tickcount += ((long)fsecs / 100); } TimeSpan result = new TimeSpan(tickcount); return result; } //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// // // Operators // //////////////////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////////////////// public static OracleBoolean Equals(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator == return (x == y); } public static OracleBoolean GreaterThan(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator > return (x > y); } public static OracleBoolean GreaterThanOrEqual(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator >= return (x >= y); } public static OracleBoolean LessThan(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator < return (x < y); } public static OracleBoolean LessThanOrEqual(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator <= return (x <= y); } public static OracleBoolean NotEquals(OracleTimeSpan x, OracleTimeSpan y) { // Alternative method for operator != return (x != y); } public static explicit operator TimeSpan(OracleTimeSpan x) { if (x.IsNull) { throw ADP.DataIsNull(); } return x.Value; } public static explicit operator OracleTimeSpan(string x) { return OracleTimeSpan.Parse(x); } public static OracleBoolean operator== (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) == 0); } public static OracleBoolean operator> (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) > 0); } public static OracleBoolean operator>= (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) >= 0); } public static OracleBoolean operator< (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) < 0); } public static OracleBoolean operator<= (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) <= 0); } public static OracleBoolean operator!= (OracleTimeSpan x, OracleTimeSpan y) { return (x.IsNull || y.IsNull) ? OracleBoolean.Null : new OracleBoolean(x.CompareTo(y) != 0); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
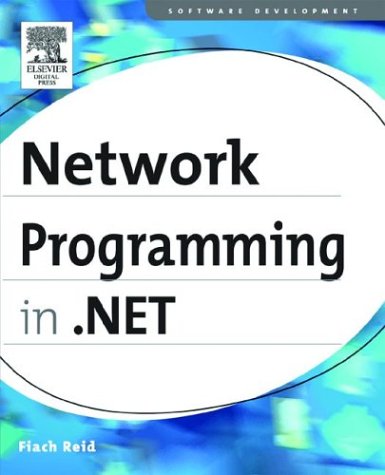
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WorkflowServiceNamespace.cs
- QilGenerator.cs
- NetworkCredential.cs
- GroupStyle.cs
- BitmapPalettes.cs
- XmlHierarchyData.cs
- GridLengthConverter.cs
- IgnoreDataMemberAttribute.cs
- UnionCodeGroup.cs
- ZipIOExtraFieldZip64Element.cs
- OracleTimeSpan.cs
- ProvidersHelper.cs
- ITextView.cs
- XmlEnumAttribute.cs
- IisTraceWebEventProvider.cs
- XmlTextReaderImpl.cs
- InvalidateEvent.cs
- KeyMatchBuilder.cs
- XPathArrayIterator.cs
- StringBuilder.cs
- ShutDownListener.cs
- CodeGroup.cs
- BuiltInPermissionSets.cs
- DataGridViewCellStyleChangedEventArgs.cs
- DbFunctionCommandTree.cs
- TypeSystem.cs
- XmlRawWriter.cs
- _PooledStream.cs
- EntityDataSourceStatementEditorForm.cs
- ByteAnimationBase.cs
- DuplexChannel.cs
- CriticalFinalizerObject.cs
- ProgressBarAutomationPeer.cs
- DataSource.cs
- Label.cs
- ReliableChannelBinder.cs
- SystemIPInterfaceStatistics.cs
- TextProperties.cs
- SmtpFailedRecipientsException.cs
- KeySplineConverter.cs
- PreviewKeyDownEventArgs.cs
- ISessionStateStore.cs
- smtppermission.cs
- VerificationException.cs
- PolicyReader.cs
- State.cs
- QualifierSet.cs
- Compilation.cs
- FrameworkObject.cs
- TextServicesPropertyRanges.cs
- MemberBinding.cs
- InputProviderSite.cs
- CollaborationHelperFunctions.cs
- DbConnectionPoolGroupProviderInfo.cs
- VirtualDirectoryMappingCollection.cs
- relpropertyhelper.cs
- DataConnectionHelper.cs
- ConstraintStruct.cs
- RichTextBoxAutomationPeer.cs
- GenericUriParser.cs
- CustomBindingElementCollection.cs
- OracleTimeSpan.cs
- ObjectDataSource.cs
- JsonUriDataContract.cs
- EdmProviderManifest.cs
- RangeValueProviderWrapper.cs
- RegionIterator.cs
- BooleanToVisibilityConverter.cs
- SafeRightsManagementQueryHandle.cs
- OutOfProcStateClientManager.cs
- LinqDataSourceValidationException.cs
- Queue.cs
- ThreadStateException.cs
- GregorianCalendarHelper.cs
- ObservableCollection.cs
- GeneralTransform2DTo3D.cs
- TraceXPathNavigator.cs
- InvalidFilterCriteriaException.cs
- SqlFacetAttribute.cs
- KnownColorTable.cs
- DurableInstance.cs
- ContainerControl.cs
- TypeBrowserDialog.cs
- Variable.cs
- ErrorLog.cs
- fixedPageContentExtractor.cs
- FillBehavior.cs
- ClientConvert.cs
- RubberbandSelector.cs
- DES.cs
- QueryRewriter.cs
- MatrixCamera.cs
- QilSortKey.cs
- regiisutil.cs
- ValidatedControlConverter.cs
- Aggregates.cs
- DataTableClearEvent.cs
- Image.cs
- SqlExpressionNullability.cs
- FixedHyperLink.cs