Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Framework / System / Windows / Documents / TextServicesPropertyRanges.cs / 1 / TextServicesPropertyRanges.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextServicesPropertyRanges.cs // // History: // 08/01/2003 : yutakas - Ported from dotnet tree. // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using System.Collections; using System.Diagnostics; using System.Windows.Media; using System.Windows.Input; using System.Windows.Documents; using MS.Win32; namespace System.Windows.Documents { //----------------------------------------------------- // // TextServicesPropertyRanges class // //----------------------------------------------------- ////// The base class for property ranges of EditRecord /// internal class TextServicesPropertyRanges { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- internal TextServicesPropertyRanges( TextStore textstore, Guid guid) { _guid = guid; _textstore = textstore; } //------------------------------------------------------ // // Internal Method // //------------------------------------------------------ ////// OnRange virtual method. /// internal virtual void OnRange(UnsafeNativeMethods.ITfProperty property, int ecReadonly, UnsafeNativeMethods.ITfRange range) { } ////// Calback function for TextEditSink /// we track the property change here. /// ////// Critical - calls unmanaged code, commits raw input to the system /// [SecurityCritical] internal virtual void OnEndEdit(UnsafeNativeMethods.ITfContext context, int ecReadOnly, UnsafeNativeMethods.ITfEditRecord editRecord) { int fetched; UnsafeNativeMethods.IEnumTfRanges ranges; UnsafeNativeMethods.ITfProperty prop = null; ranges = GetPropertyUpdate(editRecord); UnsafeNativeMethods.ITfRange [] outRanges; outRanges = new UnsafeNativeMethods.ITfRange[1]; while (ranges.Next(1, outRanges, out fetched) == 0) { // // check the element has enabled dynamic property. // ITextPointer start; ITextPointer end; ConvertToTextPosition(outRanges[0], out start, out end); if (prop == null) context.GetProperty(ref _guid, out prop); UnsafeNativeMethods.IEnumTfRanges rangesProp; if (prop.EnumRanges(ecReadOnly, out rangesProp, outRanges[0]) == 0) { UnsafeNativeMethods.ITfRange [] outRangesProp; outRangesProp = new UnsafeNativeMethods.ITfRange[1]; while (rangesProp.Next(1, outRangesProp, out fetched) == 0) { OnRange(prop, ecReadOnly, outRangesProp[0]); Marshal.ReleaseComObject(outRangesProp[0]); } Marshal.ReleaseComObject(rangesProp); } Marshal.ReleaseComObject(outRanges[0]); } Marshal.ReleaseComObject(ranges); if (prop != null) Marshal.ReleaseComObject(prop); } //----------------------------------------------------- // // Protected Method // //------------------------------------------------------ ////// Convert ITfRange to two TextPositions. /// ////// Critical - calls unmanaged code (GetExtent) /// [SecurityCritical] protected void ConvertToTextPosition(UnsafeNativeMethods.ITfRange range, out ITextPointer start, out ITextPointer end) { UnsafeNativeMethods.ITfRangeACP rangeACP; int startIndex; int length; rangeACP = range as UnsafeNativeMethods.ITfRangeACP; rangeACP.GetExtent(out startIndex, out length); if (length < 0) { // Cicero bug! Length should never be less than zero. start = null; end = null; } else { start = _textstore.CreatePointerAtCharOffset(startIndex, LogicalDirection.Forward); end = _textstore.CreatePointerAtCharOffset(startIndex + length, LogicalDirection.Forward); } } ////// Get Cicero property value. /// ////// Critical - calls unmanaged code (GetValue) and expose the returned object directly. /// It could be any variant in a Cicero property in general. /// non Avalon derived class is blocked by link demand /// [UIPermissionAttribute(SecurityAction.LinkDemand, Unrestricted= true)] [SecurityCritical] protected static Object GetValue(int ecReadOnly, UnsafeNativeMethods.ITfProperty property, UnsafeNativeMethods.ITfRange range) { if (property == null) return null; Object obj; property.GetValue(ecReadOnly, range, out obj); return obj; } ////// Get ranges that the property is changed. /// ////// Critical - unsafe block to manipulate a pointer of a pointer to GUID. /// [SecurityCritical] private UnsafeNativeMethods.IEnumTfRanges GetPropertyUpdate( UnsafeNativeMethods.ITfEditRecord editRecord) { UnsafeNativeMethods.IEnumTfRanges ranges; unsafe { fixed (Guid *pguid = &_guid) { // // IntPtr p = (IntPtr)pguid; editRecord.GetTextAndPropertyUpdates(0, ref p, 1, out ranges); } } return ranges; } //----------------------------------------------------- // // Protected Properties // //----------------------------------------------------- ////// GUID of ITfProperty /// protected Guid Guid { get { return _guid; } } ////// Return TextStore that is associated to this property. /// protected TextStore TextStore { get { return _textstore; } } //----------------------------------------------------- // // Private Field // //------------------------------------------------------ // GUID of ITfProperty private Guid _guid; // The refrence of TextStore. private TextStore _textstore; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: TextServicesPropertyRanges.cs // // History: // 08/01/2003 : yutakas - Ported from dotnet tree. // //--------------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows.Threading; using System.Security; using System.Security.Permissions; using System.Collections; using System.Diagnostics; using System.Windows.Media; using System.Windows.Input; using System.Windows.Documents; using MS.Win32; namespace System.Windows.Documents { //----------------------------------------------------- // // TextServicesPropertyRanges class // //----------------------------------------------------- ////// The base class for property ranges of EditRecord /// internal class TextServicesPropertyRanges { //------------------------------------------------------ // // Constructors // //----------------------------------------------------- internal TextServicesPropertyRanges( TextStore textstore, Guid guid) { _guid = guid; _textstore = textstore; } //------------------------------------------------------ // // Internal Method // //------------------------------------------------------ ////// OnRange virtual method. /// internal virtual void OnRange(UnsafeNativeMethods.ITfProperty property, int ecReadonly, UnsafeNativeMethods.ITfRange range) { } ////// Calback function for TextEditSink /// we track the property change here. /// ////// Critical - calls unmanaged code, commits raw input to the system /// [SecurityCritical] internal virtual void OnEndEdit(UnsafeNativeMethods.ITfContext context, int ecReadOnly, UnsafeNativeMethods.ITfEditRecord editRecord) { int fetched; UnsafeNativeMethods.IEnumTfRanges ranges; UnsafeNativeMethods.ITfProperty prop = null; ranges = GetPropertyUpdate(editRecord); UnsafeNativeMethods.ITfRange [] outRanges; outRanges = new UnsafeNativeMethods.ITfRange[1]; while (ranges.Next(1, outRanges, out fetched) == 0) { // // check the element has enabled dynamic property. // ITextPointer start; ITextPointer end; ConvertToTextPosition(outRanges[0], out start, out end); if (prop == null) context.GetProperty(ref _guid, out prop); UnsafeNativeMethods.IEnumTfRanges rangesProp; if (prop.EnumRanges(ecReadOnly, out rangesProp, outRanges[0]) == 0) { UnsafeNativeMethods.ITfRange [] outRangesProp; outRangesProp = new UnsafeNativeMethods.ITfRange[1]; while (rangesProp.Next(1, outRangesProp, out fetched) == 0) { OnRange(prop, ecReadOnly, outRangesProp[0]); Marshal.ReleaseComObject(outRangesProp[0]); } Marshal.ReleaseComObject(rangesProp); } Marshal.ReleaseComObject(outRanges[0]); } Marshal.ReleaseComObject(ranges); if (prop != null) Marshal.ReleaseComObject(prop); } //----------------------------------------------------- // // Protected Method // //------------------------------------------------------ ////// Convert ITfRange to two TextPositions. /// ////// Critical - calls unmanaged code (GetExtent) /// [SecurityCritical] protected void ConvertToTextPosition(UnsafeNativeMethods.ITfRange range, out ITextPointer start, out ITextPointer end) { UnsafeNativeMethods.ITfRangeACP rangeACP; int startIndex; int length; rangeACP = range as UnsafeNativeMethods.ITfRangeACP; rangeACP.GetExtent(out startIndex, out length); if (length < 0) { // Cicero bug! Length should never be less than zero. start = null; end = null; } else { start = _textstore.CreatePointerAtCharOffset(startIndex, LogicalDirection.Forward); end = _textstore.CreatePointerAtCharOffset(startIndex + length, LogicalDirection.Forward); } } ////// Get Cicero property value. /// ////// Critical - calls unmanaged code (GetValue) and expose the returned object directly. /// It could be any variant in a Cicero property in general. /// non Avalon derived class is blocked by link demand /// [UIPermissionAttribute(SecurityAction.LinkDemand, Unrestricted= true)] [SecurityCritical] protected static Object GetValue(int ecReadOnly, UnsafeNativeMethods.ITfProperty property, UnsafeNativeMethods.ITfRange range) { if (property == null) return null; Object obj; property.GetValue(ecReadOnly, range, out obj); return obj; } ////// Get ranges that the property is changed. /// ////// Critical - unsafe block to manipulate a pointer of a pointer to GUID. /// [SecurityCritical] private UnsafeNativeMethods.IEnumTfRanges GetPropertyUpdate( UnsafeNativeMethods.ITfEditRecord editRecord) { UnsafeNativeMethods.IEnumTfRanges ranges; unsafe { fixed (Guid *pguid = &_guid) { // // IntPtr p = (IntPtr)pguid; editRecord.GetTextAndPropertyUpdates(0, ref p, 1, out ranges); } } return ranges; } //----------------------------------------------------- // // Protected Properties // //----------------------------------------------------- ////// GUID of ITfProperty /// protected Guid Guid { get { return _guid; } } ////// Return TextStore that is associated to this property. /// protected TextStore TextStore { get { return _textstore; } } //----------------------------------------------------- // // Private Field // //------------------------------------------------------ // GUID of ITfProperty private Guid _guid; // The refrence of TextStore. private TextStore _textstore; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
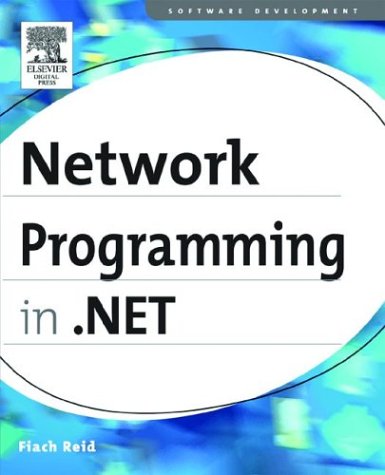
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FormViewPagerRow.cs
- CreateUserWizard.cs
- Semaphore.cs
- OdbcFactory.cs
- ConfigUtil.cs
- AddInProcess.cs
- XsltArgumentList.cs
- _NegoState.cs
- WindowsGraphicsCacheManager.cs
- ProjectionAnalyzer.cs
- AvTraceFormat.cs
- AutomationElement.cs
- CTreeGenerator.cs
- ColorTransform.cs
- HtmlEncodedRawTextWriter.cs
- ArithmeticException.cs
- CheckableControlBaseAdapter.cs
- ConfigurationLockCollection.cs
- AssemblyLoader.cs
- TraceHandlerErrorFormatter.cs
- TypeTypeConverter.cs
- XsdCachingReader.cs
- DictionaryEntry.cs
- xdrvalidator.cs
- XmlTextReaderImplHelpers.cs
- UserPersonalizationStateInfo.cs
- DaylightTime.cs
- WpfSharedBamlSchemaContext.cs
- FontUnitConverter.cs
- ToolStripGripRenderEventArgs.cs
- PenContexts.cs
- ElementAtQueryOperator.cs
- ImportContext.cs
- EntityDataSourceMemberPath.cs
- WebPartRestoreVerb.cs
- DocumentGridPage.cs
- LinqMaximalSubtreeNominator.cs
- OutKeywords.cs
- WebPartEventArgs.cs
- ListDependantCardsRequest.cs
- ScrollChrome.cs
- WebPartConnectionsCancelVerb.cs
- AssertSection.cs
- Thickness.cs
- DataGridTableCollection.cs
- ProtocolElementCollection.cs
- NumberFunctions.cs
- DataPager.cs
- DbConvert.cs
- EdgeProfileValidation.cs
- CompiledIdentityConstraint.cs
- wgx_commands.cs
- XPathNodePointer.cs
- Type.cs
- SpnegoTokenProvider.cs
- _UriSyntax.cs
- PathGradientBrush.cs
- InputElement.cs
- RotateTransform3D.cs
- DuplicateWaitObjectException.cs
- KernelTypeValidation.cs
- DesignerWebPartChrome.cs
- SQLInt32.cs
- SmtpTransport.cs
- TriggerCollection.cs
- ConfigXmlElement.cs
- HttpServerUtilityWrapper.cs
- InternalSendMessage.cs
- DPTypeDescriptorContext.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- DataSourceView.cs
- DataColumnCollection.cs
- LoginDesignerUtil.cs
- DeclaredTypeValidatorAttribute.cs
- WinFormsComponentEditor.cs
- DataGridViewMethods.cs
- DataBindingHandlerAttribute.cs
- BoundPropertyEntry.cs
- RefreshPropertiesAttribute.cs
- FrameworkContentElementAutomationPeer.cs
- BindingElementExtensionElement.cs
- SettingsProperty.cs
- ProgressBar.cs
- RawStylusActions.cs
- StringArrayConverter.cs
- BufferCache.cs
- CheckBoxBaseAdapter.cs
- EndEvent.cs
- XPathChildIterator.cs
- DocumentPageHost.cs
- DefaultTextStoreTextComposition.cs
- ZoneButton.cs
- BitmapEffectGroup.cs
- XmlSchemaCompilationSettings.cs
- SpeechEvent.cs
- TaskFileService.cs
- CodeComment.cs
- Codec.cs
- PathFigureCollectionConverter.cs
- KeyTimeConverter.cs