Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / Media / UniqueEventHelper.cs / 1305600 / UniqueEventHelper.cs
using System; using System.Collections; using System.Diagnostics; namespace System.Windows.Media { ////// Aids in making events unique. i.e. you register the same function for /// the same event twice, but it will only be called once for each time /// the event is invoked. /// /// UniqueEventHelper should only be accessed from the UI thread so that /// handlers that throw exceptions will crash the app, making the developer /// aware of the problem. /// internal class UniqueEventHelperwhere TEventArgs : EventArgs { /// /// Add the handler to the list of handlers associated with this event. /// If the handler has already been added, we simply increment the ref /// count. That way if this same handler has already been added, it /// won't be invoked multiple times when the event is raised. /// internal void AddEvent(EventHandlerhandler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] == null) { _htDelegates.Add(handler, 1); } else { int refCount = (int)_htDelegates[handler] + 1; _htDelegates[handler] = refCount; } } /// /// Removed the handler from the list of handlers associated with this /// event. If the handler has been added multiple times (more times than /// it has been removed), we simply decrement its ref count. /// internal void RemoveEvent(EventHandlerhandler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] != null) { int refCount = (int)_htDelegates[handler]; if (refCount == 1) { _htDelegates.Remove(handler); } else { _htDelegates[handler] = refCount - 1; } } } /// /// Enumerates all the keys in the hashtable, which must be EventHandlers, /// and invokes them. /// /// The sender for the callback. /// The args object to be sent by the delegate internal void InvokeEvents(object sender, TEventArgs args) { Debug.Assert((sender != null), "Sender is null"); if (_htDelegates != null) { Hashtable htDelegates = (Hashtable)_htDelegates.Clone(); foreach (EventHandlerhandler in htDelegates.Keys) { Debug.Assert((handler != null), "Event handler is null"); handler(sender, args); } } } /// /// Clones the event helper /// internal UniqueEventHelperClone() { UniqueEventHelper ueh = new UniqueEventHelper (); if (_htDelegates != null) { ueh._htDelegates = (Hashtable)_htDelegates.Clone(); } return ueh; } /// /// Ensures Hashtable is created so that event handlers can be added/removed /// private void EnsureEventTable() { if (_htDelegates == null) { _htDelegates = new Hashtable(); } } private Hashtable _htDelegates; } // internal class UniqueEventHelper { ////// Add the handler to the list of handlers associated with this event. /// If the handler has already been added, we simply increment the ref /// count. That way if this same handler has already been added, it /// won't be invoked multiple times when the event is raised. /// internal void AddEvent(EventHandler handler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] == null) { _htDelegates.Add(handler, 1); } else { int refCount = (int)_htDelegates[handler] + 1; _htDelegates[handler] = refCount; } } ////// Removed the handler from the list of handlers associated with this /// event. If the handler has been added multiple times (more times than /// it has been removed), we simply decrement its ref count. /// internal void RemoveEvent(EventHandler handler) { if (handler == null) { throw new System.ArgumentNullException("handler"); } EnsureEventTable(); if (_htDelegates[handler] != null) { int refCount = (int)_htDelegates[handler]; if (refCount == 1) { _htDelegates.Remove(handler); } else { _htDelegates[handler] = refCount - 1; } } } ////// Enumerates all the keys in the hashtable, which must be EventHandlers, /// and invokes them. /// /// The sender for the callback. /// The args object to be sent by the delegate internal void InvokeEvents(object sender, EventArgs args) { Debug.Assert((sender != null), "Sender is null"); if (_htDelegates != null) { Hashtable htDelegates = (Hashtable)_htDelegates.Clone(); foreach (EventHandler handler in htDelegates.Keys) { Debug.Assert((handler != null), "Event handler is null"); handler(sender, args); } } } ////// Clones the event helper /// internal UniqueEventHelper Clone() { UniqueEventHelper ueh = new UniqueEventHelper(); if (_htDelegates != null) { ueh._htDelegates = (Hashtable)_htDelegates.Clone(); } return ueh; } ////// Ensures Hashtable is created so that event handlers can be added/removed /// private void EnsureEventTable() { if (_htDelegates == null) { _htDelegates = new Hashtable(); } } private Hashtable _htDelegates; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
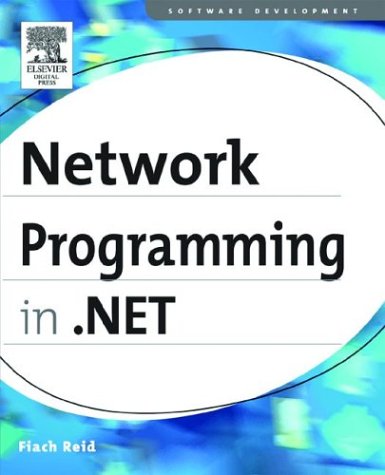
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SmtpSpecifiedPickupDirectoryElement.cs
- Parameter.cs
- AssociationEndMember.cs
- WorkflowMarkupSerializationProvider.cs
- AppLevelCompilationSectionCache.cs
- EntityDataSourceDataSelection.cs
- CallSiteOps.cs
- HttpTransportElement.cs
- XPathNodeList.cs
- ApplicationServiceHelper.cs
- Command.cs
- HScrollBar.cs
- TemplatePropertyEntry.cs
- UpdateCommand.cs
- ChildrenQuery.cs
- ErrorFormatterPage.cs
- ReadOnlyPropertyMetadata.cs
- ListItem.cs
- SmiXetterAccessMap.cs
- AlignmentYValidation.cs
- XmlUtil.cs
- CqlParser.cs
- DefaultAssemblyResolver.cs
- SkipStoryboardToFill.cs
- SchemaImporterExtensionElement.cs
- JsonWriter.cs
- TextRunCache.cs
- TagMapCollection.cs
- UnsafeNativeMethods.cs
- DataBoundControl.cs
- CommandLibraryHelper.cs
- StorageSetMapping.cs
- DefaultHttpHandler.cs
- InputMethodStateTypeInfo.cs
- TemplateAction.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- XmlTextReaderImplHelpers.cs
- EntityContainerEmitter.cs
- RuntimeHelpers.cs
- SystemResourceKey.cs
- EmbeddedObject.cs
- FixedSOMLineCollection.cs
- ToolTip.cs
- CreateUserWizardStep.cs
- GZipDecoder.cs
- CatalogPartCollection.cs
- DecimalFormatter.cs
- BidirectionalDictionary.cs
- SessionViewState.cs
- Context.cs
- SearchForVirtualItemEventArgs.cs
- FillRuleValidation.cs
- ConfigurationElementCollection.cs
- Encoder.cs
- WebCategoryAttribute.cs
- KnownBoxes.cs
- QuaternionIndependentAnimationStorage.cs
- SmiRecordBuffer.cs
- MulticastOption.cs
- WebBrowserNavigatedEventHandler.cs
- TableRowGroup.cs
- iisPickupDirectory.cs
- DbException.cs
- LineServicesCallbacks.cs
- DataControlLinkButton.cs
- SingleObjectCollection.cs
- FormsAuthenticationConfiguration.cs
- ItemPager.cs
- PathParser.cs
- WhitespaceRuleLookup.cs
- JavaScriptString.cs
- IdentityVerifier.cs
- WeakHashtable.cs
- RenderOptions.cs
- CalendarDayButton.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- codemethodreferenceexpression.cs
- ProviderConnectionPoint.cs
- SymbolEqualComparer.cs
- HostProtectionException.cs
- XamlPathDataSerializer.cs
- CommandHelper.cs
- ObjectCacheSettings.cs
- RightsManagementInformation.cs
- BinarySecretKeyIdentifierClause.cs
- Wildcard.cs
- StreamWriter.cs
- SamlSubjectStatement.cs
- OleDbPermission.cs
- ApplicationDirectoryMembershipCondition.cs
- DoubleAnimationClockResource.cs
- EnglishPluralizationService.cs
- SerialPinChanges.cs
- EntityCommandExecutionException.cs
- ReaderContextStackData.cs
- ResolveNameEventArgs.cs
- ButtonStandardAdapter.cs
- HtmlTable.cs
- MembershipPasswordException.cs
- EntryWrittenEventArgs.cs