Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / Primitives / CalendarDayButton.cs / 1305600 / CalendarDayButton.cs
//---------------------------------------------------------------------------- // // Copyright (C) Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System.Windows; using System.Windows.Automation.Peers; using System.Windows.Controls; using System.Windows.Data; namespace System.Windows.Controls.Primitives { ////// Represents a button control used in Calendar Control, which reacts to the Click event. /// public sealed class CalendarDayButton : Button { #region Constants ////// Default content for the CalendarDayButton /// private const int DEFAULTCONTENT = 1; #endregion ////// Static constructor /// static CalendarDayButton() { DefaultStyleKeyProperty.OverrideMetadata(typeof(CalendarDayButton), new FrameworkPropertyMetadata(typeof(CalendarDayButton))); } ////// Represents the CalendarDayButton that is used in Calendar Control. /// public CalendarDayButton() : base() { } #region Public Properties #region IsToday internal static readonly DependencyPropertyKey IsTodayPropertyKey = DependencyProperty.RegisterReadOnly( "IsToday", typeof(bool), typeof(CalendarDayButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for IsToday property /// public static readonly DependencyProperty IsTodayProperty = IsTodayPropertyKey.DependencyProperty; ////// True if the CalendarDayButton represents today /// public bool IsToday { get { return (bool)GetValue(IsTodayProperty); } } #endregion IsToday #region IsSelected internal static readonly DependencyPropertyKey IsSelectedPropertyKey = DependencyProperty.RegisterReadOnly( "IsSelected", typeof(bool), typeof(CalendarDayButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for IsSelected property /// public static readonly DependencyProperty IsSelectedProperty = IsSelectedPropertyKey.DependencyProperty; ////// True if the CalendarDayButton is selected /// public bool IsSelected { get { return (bool)GetValue(IsSelectedProperty); } } #endregion IsSelected #region IsInactive internal static readonly DependencyPropertyKey IsInactivePropertyKey = DependencyProperty.RegisterReadOnly( "IsInactive", typeof(bool), typeof(CalendarDayButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for IsActive property /// public static readonly DependencyProperty IsInactiveProperty = IsInactivePropertyKey.DependencyProperty; ////// True if the CalendarDayButton represents a day that falls in the currently displayed month /// public bool IsInactive { get { return (bool)GetValue(IsInactiveProperty); } } #endregion IsInactive #region IsBlackedOut internal static readonly DependencyPropertyKey IsBlackedOutPropertyKey = DependencyProperty.RegisterReadOnly( "IsBlackedOut", typeof(bool), typeof(CalendarDayButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for IsBlackedOut property /// public static readonly DependencyProperty IsBlackedOutProperty = IsBlackedOutPropertyKey.DependencyProperty; ////// True if the CalendarDayButton represents a blackout date /// public bool IsBlackedOut { get { return (bool)GetValue(IsBlackedOutProperty); } } #endregion IsBlackedOut #region IsHighlighted internal static readonly DependencyPropertyKey IsHighlightedPropertyKey = DependencyProperty.RegisterReadOnly( "IsHighlighted", typeof(bool), typeof(CalendarDayButton), new FrameworkPropertyMetadata(false, new PropertyChangedCallback(OnVisualStatePropertyChanged))); ////// Dependency property field for IsHighlighted property /// public static readonly DependencyProperty IsHighlightedProperty = IsHighlightedPropertyKey.DependencyProperty; ////// True if the CalendarDayButton represents a highlighted date /// public bool IsHighlighted { get { return (bool)GetValue(IsHighlightedProperty); } } #endregion IsHighlighted #endregion Public Properties #region Internal Properties internal Calendar Owner { get; set; } #endregion Internal Properties #region Public Methods #endregion Public Methods #region Protected Methods ////// Creates the automation peer for the CalendarDayButton. /// ///protected override AutomationPeer OnCreateAutomationPeer() { return new CalendarButtonAutomationPeer(this); } #endregion Protected Methods #region Internal Methods /// /// Change to the correct visual state for the button. /// /// /// true to use transitions when updating the visual state, false to /// snap directly to the new visual state. /// internal override void ChangeVisualState(bool useTransitions) { // Update the SelectionStates group if (IsSelected || IsHighlighted) { VisualStates.GoToState(this, useTransitions, VisualStates.StateSelected, VisualStates.StateUnselected); } else { VisualStateManager.GoToState(this, VisualStates.StateUnselected, useTransitions); } // Update the ActiveStates group if (!IsInactive) { VisualStates.GoToState(this, useTransitions, VisualStates.StateActive, VisualStates.StateInactive); } else { VisualStateManager.GoToState(this, VisualStates.StateInactive, useTransitions); } // Update the DayStates group if (IsToday && this.Owner != null && this.Owner.IsTodayHighlighted) { VisualStates.GoToState(this, useTransitions, VisualStates.StateToday, VisualStates.StateRegularDay); } else { VisualStateManager.GoToState(this, VisualStates.StateRegularDay, useTransitions); } // Update the BlackoutDayStates group if (IsBlackedOut) { VisualStates.GoToState(this, useTransitions, VisualStates.StateBlackoutDay, VisualStates.StateNormalDay); } else { VisualStateManager.GoToState(this, VisualStates.StateNormalDay, useTransitions); } // Update the FocusStates group if (IsKeyboardFocused) { VisualStates.GoToState(this, useTransitions, VisualStates.StateCalendarButtonFocused, VisualStates.StateCalendarButtonUnfocused); } else { VisualStateManager.GoToState(this, VisualStates.StateCalendarButtonUnfocused, useTransitions); } base.ChangeVisualState(useTransitions); } internal void NotifyNeedsVisualStateUpdate() { UpdateVisualState(); } internal void SetContentInternal(string value) { SetCurrentValueInternal(ContentControl.ContentProperty, value); } #endregion Internal Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
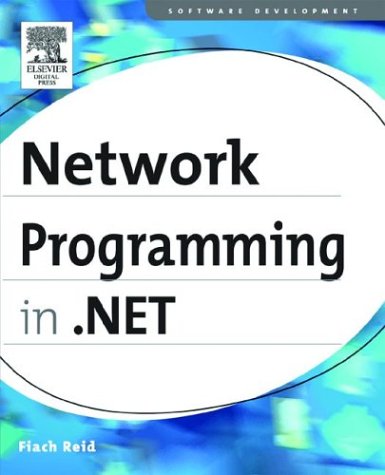
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewItemSelectionChangedEvent.cs
- LineServicesCallbacks.cs
- ApplicationException.cs
- SignedPkcs7.cs
- BitStream.cs
- DetailsViewDeleteEventArgs.cs
- CodeMemberField.cs
- CurrentChangingEventManager.cs
- BadImageFormatException.cs
- ProfileEventArgs.cs
- ObjectHelper.cs
- ProvidePropertyAttribute.cs
- LeaseManager.cs
- QueryableFilterUserControl.cs
- QilFactory.cs
- NamedElement.cs
- SwitchAttribute.cs
- BoolExpressionVisitors.cs
- DataGridItem.cs
- RegistryConfigurationProvider.cs
- NativeMethodsCLR.cs
- DNS.cs
- SecondaryViewProvider.cs
- BaseDataBoundControl.cs
- Compress.cs
- ClockGroup.cs
- NaturalLanguageHyphenator.cs
- ObjectDesignerDataSourceView.cs
- FlowLayout.cs
- DataObjectPastingEventArgs.cs
- HtmlElementErrorEventArgs.cs
- EraserBehavior.cs
- ExecutionEngineException.cs
- Timeline.cs
- ToolStripMenuItem.cs
- ProfilePropertySettings.cs
- InheritanceAttribute.cs
- IndentTextWriter.cs
- BitmapFrameEncode.cs
- XmlElementAttributes.cs
- XslAst.cs
- InputMethodStateChangeEventArgs.cs
- PerformanceCounterManager.cs
- TreeBuilder.cs
- Crypto.cs
- IntSecurity.cs
- SqlDelegatedTransaction.cs
- WindowsListViewItem.cs
- HtmlToClrEventProxy.cs
- HtmlInputRadioButton.cs
- StackOverflowException.cs
- DllNotFoundException.cs
- CommandLineParser.cs
- CmsInterop.cs
- ItemsPresenter.cs
- PrefixHandle.cs
- ColumnResult.cs
- EventRoute.cs
- ColumnHeaderCollectionEditor.cs
- SecuritySessionServerSettings.cs
- StringConverter.cs
- ConnectionConsumerAttribute.cs
- EmptyEnumerator.cs
- AddInDeploymentState.cs
- RegexStringValidatorAttribute.cs
- SQLDecimal.cs
- ResourceDescriptionAttribute.cs
- ToolStripPanelSelectionBehavior.cs
- TrustSection.cs
- RegexCompiler.cs
- DirectoryObjectSecurity.cs
- PrintDialogException.cs
- PageVisual.cs
- AuthorizationRuleCollection.cs
- _AcceptOverlappedAsyncResult.cs
- StylusEventArgs.cs
- SingleKeyFrameCollection.cs
- XmlCharacterData.cs
- ToolboxItem.cs
- ISSmlParser.cs
- AnnouncementService.cs
- ListenerAdapter.cs
- ProfileSettingsCollection.cs
- InputReferenceExpression.cs
- XmlConvert.cs
- XLinq.cs
- HTMLTextWriter.cs
- AsyncStreamReader.cs
- BitmapSource.cs
- WebMethodAttribute.cs
- CustomErrorCollection.cs
- QuarticEase.cs
- XsltFunctions.cs
- ReadOnlyMetadataCollection.cs
- CellTreeNodeVisitors.cs
- ChildTable.cs
- CharAnimationUsingKeyFrames.cs
- BindValidationContext.cs
- SelectorAutomationPeer.cs
- figurelength.cs