Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / DataObjectPastingEventArgs.cs / 1305600 / DataObjectPastingEventArgs.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DataObject.Pasting event arguments // //--------------------------------------------------------------------------- using System; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// Arguments for the DataObject.Pasting event. /// /// The DataObject.Pasting event is raising when an editor /// has inspected all formats available on a data object /// has choosen one of them as the most suitable and /// is ready for pasting it into a current selection. /// An application can inspect a DataObject, change, remove or /// add some data formats into it and decide whether to proceed /// with the pasting or cancel it. /// public sealed class DataObjectPastingEventArgs : DataObjectEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a DataObjectPastingEvent. /// This object created by editors executing a Copy/Paste /// and Drag/Drop comands. /// /// /// DataObject extracted from the Clipboard and intended /// for using in pasting. /// /// /// A flag indicating whether this operation is part of drag/drop. /// Pasting event is fired on drop. /// /// /// String identifying a format an editor has choosen /// as a candidate for applying in Paste operation. /// An application can change this choice after inspecting /// the content of data object. /// public DataObjectPastingEventArgs(IDataObject dataObject, bool isDragDrop, string formatToApply) // : base(System.Windows.DataObject.PastingEvent, isDragDrop) { if (dataObject == null) { throw new ArgumentNullException("dataObject"); } if (formatToApply == null) { throw new ArgumentNullException("formatToApply"); } if (formatToApply == string.Empty) { throw new ArgumentException(SR.Get(SRID.DataObject_EmptyFormatNotAllowed)); } if (!dataObject.GetDataPresent(formatToApply)) { throw new ArgumentException(SR.Get(SRID.DataObject_DataFormatNotPresentOnDataObject, formatToApply)); } _originalDataObject = dataObject; _dataObject = dataObject; _formatToApply = formatToApply; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// DataObject original extracted from the Clipboard. /// It's content cannot be changed to avoid side effects /// on subsequent paste operations in this or other /// applications. /// To change the content of a data object custon handler /// must create new instance of an object and assign it /// to DataObject property, which will be used by /// an editor to perform a paste operation. /// Initially both properties DataObject and SourceDataObject /// have the same value. DataObject property can be changed /// by a custom handler, SourceDataObject keeps original value. /// SourceDataObject can be useful in a case when several /// independent DataObjectPastingEventHandlers workone after onother. /// After one handler added its new DataObject SourceDataObject /// property allows other handlers to access original clipboard. /// public IDataObject SourceDataObject { get { return _originalDataObject; } } ////// DataObject suggested for a pasting operation. /// Originally this property has the same value as SourceDataObject. /// Custom handlers can change it by assigning some new dataobject. /// This new dataobject must have at least one format set to it, /// which will become a suggested format (FormatToAppy) when /// dataobject is assigned. Thus FormatToAlly is always consistent /// with current DataObject (but not necessarily with SourceDataObject). /// public IDataObject DataObject { get { return _dataObject; } set { string[] availableFormats; if (value == null) { throw new ArgumentNullException("value"); } availableFormats = value.GetFormats(/*autoConvert:*/false); if (availableFormats == null || availableFormats.Length == 0) { throw new ArgumentException(SR.Get(SRID.DataObject_DataObjectMustHaveAtLeastOneFormat)); } _dataObject = value; _formatToApply = availableFormats[0]; } } ////// String identifying a format an editor has choosen /// as a candidate for applying in Paste operation. /// An application can change this choice after inspecting /// the content of data object. /// The value assigned to FormatToApply must be present /// on a current DataObject. The invariant is /// this.DataObject.GetDataPresent(this.FormatToApply) === true. /// public string FormatToApply { get { return _formatToApply; } set { if (value == null) { throw new ArgumentNullException("value"); } if (!_dataObject.GetDataPresent(value)) { throw new ArgumentException(SR.Get(SRID.DataObject_DataFormatNotPresentOnDataObject, value)); } _formatToApply = value; } } #endregion Public Properties #region Protected Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ ////// The mechanism used to call the type-specific handler on the target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { DataObjectPastingEventHandler handler = (DataObjectPastingEventHandler)genericHandler; handler(genericTarget, this); } #endregion Protected Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private IDataObject _originalDataObject; private IDataObject _dataObject; private string _formatToApply; #endregion Private Fields } ////// The delegate to use for handlers that receive the DataObject.Pasting event. /// ////// An event handler for a DataObject.Pasting event. /// It is called when ah editor already made a decision /// what format (from available on the Cliipboard) /// to apply to selection. With this handler an application /// has a chance to inspect a content of DataObject extracted /// from the Clipboard and decide what format to use instead. /// There are four options for the handler here: /// a) to cancel the whole Paste/Drop event by calling /// DataObjectPastingEventArgs.CancelCommand method, /// b) change an editor's choice of format by setting /// new value for DataObjectPastingEventArgs.FormatToApply /// property (the new value is supposed to be understandable /// by an editor - it's application's code responsibility /// to act consistently with an editor; example is to /// replace "rich text" (xml) format to "plain text" format - /// both understandable by the TextEditor). /// c) choose it's own custom format, apply it to a selection /// and cancel a command for the following execution in an /// editor by calling DataObjectPastingEventArgs.CancelCommand /// method. This is how custom data formats are expected /// to be pasted. /// d) create new piece of data and suggest it in a new instance /// of DataObject. newDataObject instance must be created /// with some format set to it and assigned to DataObject property. /// SourceDataObject property keeps an original DataObject /// came from the Clipboard. This original dataobject cannot be changed. /// So by assigning new dataobject a custom handler can suggest /// ned data formats or change existing dataformats. /// public delegate void DataObjectPastingEventHandler(object sender, DataObjectPastingEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: DataObject.Pasting event arguments // //--------------------------------------------------------------------------- using System; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// Arguments for the DataObject.Pasting event. /// /// The DataObject.Pasting event is raising when an editor /// has inspected all formats available on a data object /// has choosen one of them as the most suitable and /// is ready for pasting it into a current selection. /// An application can inspect a DataObject, change, remove or /// add some data formats into it and decide whether to proceed /// with the pasting or cancel it. /// public sealed class DataObjectPastingEventArgs : DataObjectEventArgs { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Creates a DataObjectPastingEvent. /// This object created by editors executing a Copy/Paste /// and Drag/Drop comands. /// /// /// DataObject extracted from the Clipboard and intended /// for using in pasting. /// /// /// A flag indicating whether this operation is part of drag/drop. /// Pasting event is fired on drop. /// /// /// String identifying a format an editor has choosen /// as a candidate for applying in Paste operation. /// An application can change this choice after inspecting /// the content of data object. /// public DataObjectPastingEventArgs(IDataObject dataObject, bool isDragDrop, string formatToApply) // : base(System.Windows.DataObject.PastingEvent, isDragDrop) { if (dataObject == null) { throw new ArgumentNullException("dataObject"); } if (formatToApply == null) { throw new ArgumentNullException("formatToApply"); } if (formatToApply == string.Empty) { throw new ArgumentException(SR.Get(SRID.DataObject_EmptyFormatNotAllowed)); } if (!dataObject.GetDataPresent(formatToApply)) { throw new ArgumentException(SR.Get(SRID.DataObject_DataFormatNotPresentOnDataObject, formatToApply)); } _originalDataObject = dataObject; _dataObject = dataObject; _formatToApply = formatToApply; } #endregion Constructors //------------------------------------------------------ // // Public Properties // //----------------------------------------------------- #region Public Properties ////// DataObject original extracted from the Clipboard. /// It's content cannot be changed to avoid side effects /// on subsequent paste operations in this or other /// applications. /// To change the content of a data object custon handler /// must create new instance of an object and assign it /// to DataObject property, which will be used by /// an editor to perform a paste operation. /// Initially both properties DataObject and SourceDataObject /// have the same value. DataObject property can be changed /// by a custom handler, SourceDataObject keeps original value. /// SourceDataObject can be useful in a case when several /// independent DataObjectPastingEventHandlers workone after onother. /// After one handler added its new DataObject SourceDataObject /// property allows other handlers to access original clipboard. /// public IDataObject SourceDataObject { get { return _originalDataObject; } } ////// DataObject suggested for a pasting operation. /// Originally this property has the same value as SourceDataObject. /// Custom handlers can change it by assigning some new dataobject. /// This new dataobject must have at least one format set to it, /// which will become a suggested format (FormatToAppy) when /// dataobject is assigned. Thus FormatToAlly is always consistent /// with current DataObject (but not necessarily with SourceDataObject). /// public IDataObject DataObject { get { return _dataObject; } set { string[] availableFormats; if (value == null) { throw new ArgumentNullException("value"); } availableFormats = value.GetFormats(/*autoConvert:*/false); if (availableFormats == null || availableFormats.Length == 0) { throw new ArgumentException(SR.Get(SRID.DataObject_DataObjectMustHaveAtLeastOneFormat)); } _dataObject = value; _formatToApply = availableFormats[0]; } } ////// String identifying a format an editor has choosen /// as a candidate for applying in Paste operation. /// An application can change this choice after inspecting /// the content of data object. /// The value assigned to FormatToApply must be present /// on a current DataObject. The invariant is /// this.DataObject.GetDataPresent(this.FormatToApply) === true. /// public string FormatToApply { get { return _formatToApply; } set { if (value == null) { throw new ArgumentNullException("value"); } if (!_dataObject.GetDataPresent(value)) { throw new ArgumentException(SR.Get(SRID.DataObject_DataFormatNotPresentOnDataObject, value)); } _formatToApply = value; } } #endregion Public Properties #region Protected Methods //------------------------------------------------------ // // Protected Methods // //------------------------------------------------------ ////// The mechanism used to call the type-specific handler on the target. /// /// /// The generic handler to call in a type-specific way. /// /// /// The target to call the handler on. /// protected override void InvokeEventHandler(Delegate genericHandler, object genericTarget) { DataObjectPastingEventHandler handler = (DataObjectPastingEventHandler)genericHandler; handler(genericTarget, this); } #endregion Protected Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private IDataObject _originalDataObject; private IDataObject _dataObject; private string _formatToApply; #endregion Private Fields } ////// The delegate to use for handlers that receive the DataObject.Pasting event. /// ////// An event handler for a DataObject.Pasting event. /// It is called when ah editor already made a decision /// what format (from available on the Cliipboard) /// to apply to selection. With this handler an application /// has a chance to inspect a content of DataObject extracted /// from the Clipboard and decide what format to use instead. /// There are four options for the handler here: /// a) to cancel the whole Paste/Drop event by calling /// DataObjectPastingEventArgs.CancelCommand method, /// b) change an editor's choice of format by setting /// new value for DataObjectPastingEventArgs.FormatToApply /// property (the new value is supposed to be understandable /// by an editor - it's application's code responsibility /// to act consistently with an editor; example is to /// replace "rich text" (xml) format to "plain text" format - /// both understandable by the TextEditor). /// c) choose it's own custom format, apply it to a selection /// and cancel a command for the following execution in an /// editor by calling DataObjectPastingEventArgs.CancelCommand /// method. This is how custom data formats are expected /// to be pasted. /// d) create new piece of data and suggest it in a new instance /// of DataObject. newDataObject instance must be created /// with some format set to it and assigned to DataObject property. /// SourceDataObject property keeps an original DataObject /// came from the Clipboard. This original dataobject cannot be changed. /// So by assigning new dataobject a custom handler can suggest /// ned data formats or change existing dataformats. /// public delegate void DataObjectPastingEventHandler(object sender, DataObjectPastingEventArgs e); } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
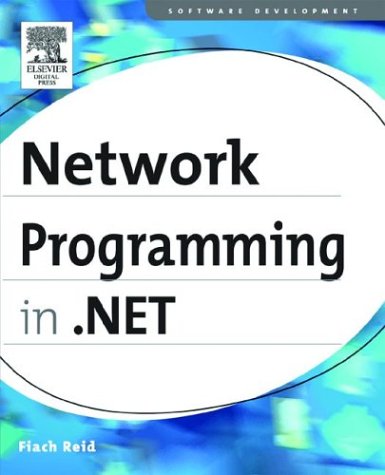
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PeerApplication.cs
- XmlDocumentSurrogate.cs
- Subordinate.cs
- CFStream.cs
- TaiwanCalendar.cs
- UpWmlMobileTextWriter.cs
- OperationPickerDialog.cs
- NativeMethods.cs
- FileRecordSequence.cs
- PasswordBoxAutomationPeer.cs
- ComplexTypeEmitter.cs
- SystemShuttingDownException.cs
- ContentDisposition.cs
- TransformCollection.cs
- ValidationHelper.cs
- TypeResolvingOptions.cs
- AssertFilter.cs
- StreamingContext.cs
- GPRECT.cs
- MediaEntryAttribute.cs
- LinkTarget.cs
- RegexCapture.cs
- VScrollProperties.cs
- FixedSOMGroup.cs
- ChannelFactory.cs
- WinCategoryAttribute.cs
- DataGridViewDataConnection.cs
- IsolatedStoragePermission.cs
- RowParagraph.cs
- elementinformation.cs
- DisableDpiAwarenessAttribute.cs
- DeviceContext.cs
- FixedSOMTableRow.cs
- ListItemCollection.cs
- OdbcConnectionFactory.cs
- Literal.cs
- ScrollChrome.cs
- UriTemplateMatchException.cs
- BuildResultCache.cs
- EntityClassGenerator.cs
- ReferenceConverter.cs
- SqlBuffer.cs
- ColumnBinding.cs
- WmpBitmapDecoder.cs
- TableLayoutStyle.cs
- UserPersonalizationStateInfo.cs
- ObjectQueryExecutionPlan.cs
- DotExpr.cs
- TextEditorMouse.cs
- CheckBoxDesigner.cs
- TableStyle.cs
- DataGridViewSelectedCellCollection.cs
- UICuesEvent.cs
- AuthenticationConfig.cs
- ComboBox.cs
- PanningMessageFilter.cs
- ConfigurationSectionGroupCollection.cs
- SmtpLoginAuthenticationModule.cs
- MetadataArtifactLoaderCompositeResource.cs
- GeometryHitTestParameters.cs
- XmlRawWriterWrapper.cs
- ClonableStack.cs
- LZCodec.cs
- PropertyFilterAttribute.cs
- SingleAnimationUsingKeyFrames.cs
- SerializationFieldInfo.cs
- DataViewSettingCollection.cs
- DataColumn.cs
- Pen.cs
- OleDbConnectionInternal.cs
- Label.cs
- WindowsScrollBar.cs
- OAVariantLib.cs
- CompilationUtil.cs
- RuleSettings.cs
- HashCryptoHandle.cs
- OpCopier.cs
- Html32TextWriter.cs
- SystemWebExtensionsSectionGroup.cs
- DataSourceUtil.cs
- updatecommandorderer.cs
- DetailsViewPageEventArgs.cs
- X509Chain.cs
- TypeListConverter.cs
- odbcmetadatacollectionnames.cs
- InitializationEventAttribute.cs
- TileBrush.cs
- DataGridViewColumnConverter.cs
- ManagedIStream.cs
- DictionaryItemsCollection.cs
- TextBlockAutomationPeer.cs
- SqlTransaction.cs
- FixedPageAutomationPeer.cs
- HuffModule.cs
- ColorAnimationUsingKeyFrames.cs
- SmtpDigestAuthenticationModule.cs
- MultiBinding.cs
- BaseTransportHeaders.cs
- MetadataSerializer.cs
- UrlMappingsModule.cs