Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Design / system / Data / EntityModel / EntityClassGenerator.cs / 1 / EntityClassGenerator.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Services.Design.Common; using System.Data.EntityModel; using System.Data.EntityModel.SchemaObjectModel; using System.Data.Metadata.Edm; using System.Diagnostics; using System.IO; using System.Xml; namespace System.Data.Common.Utils { internal static class EntityUtil { static internal void CheckArgumentNull(T value, string parameterName) where T : class { System.Data.Services.Design.EntityUtil.CheckArgumentNull (value, parameterName); } } } namespace System.Data.Services.Design { internal static class EntityUtil { static internal ArgumentException Argument(string error, string parameter) { ArgumentException e = new ArgumentException(error, parameter); return e; } static internal ArgumentNullException ArgumentNull(string parameter) { ArgumentNullException e = new ArgumentNullException(parameter); return e; } internal static void ThrowArgumentNullException(string parameterName) { throw ArgumentNull(parameterName); } static internal ArgumentException InvalidStringArgument(string parameterName) { return Argument(Strings.InvalidStringArgument(parameterName), parameterName); } static internal void CheckArgumentNull (T value, string parameterName) where T : class { if (null == value) { ThrowArgumentNullException(parameterName); } } static internal void CheckStringArgument(string value, string parameterName) { // Throw ArgumentNullException when string is null CheckArgumentNull(value, parameterName); // Throw ArgumentException when string is empty if (value.Length == 0) { throw InvalidStringArgument(parameterName); } } } /// /// Summary description for CodeGenerator. /// public sealed class EntityClassGenerator { #region Instance Fields LanguageOption _languageOption = LanguageOption.GenerateCSharpCode; EdmToObjectNamespaceMap _edmToObjectNamespaceMap = new EdmToObjectNamespaceMap(); #endregion #region Events ////// The event that is raised when a type is generated /// public event EventHandlerOnTypeGenerated; /// /// The event that is raised when a property is generated /// public event EventHandlerOnPropertyGenerated; #endregion #region Public Methods /// /// /// public EntityClassGenerator() { } ////// /// public EntityClassGenerator(LanguageOption languageOption) { _languageOption = EDesignUtil.CheckLanguageOptionArgument(languageOption, "languageOption"); } ////// Gets and Sets the Language to use for code generation. /// public LanguageOption LanguageOption { get { return _languageOption; } set { _languageOption = EDesignUtil.CheckLanguageOptionArgument(value, "value"); } } ////// Gets the map entries use to customize the namespace of .net types that are generated /// and referenced by the generated code /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public EdmToObjectNamespaceMap EdmToObjectNamespaceMap { get { return _edmToObjectNamespaceMap; } } public IListGenerateCode(XmlReader sourceReader, string targetFilePath) { EntityUtil.CheckArgumentNull(sourceReader, "sourceReader"); EntityUtil.CheckStringArgument(targetFilePath, "targetPath"); using (LazyTextWriterCreator target = new LazyTextWriterCreator(targetFilePath)) { // we do want to close the file return GenerateCode(sourceReader, target, null); } } /// /// Generate code by reading an EDMX schema from an XmlReader and outputting the code into a TextWriter /// /// Reader with a EDMX schema in it /// Target writer for the generated code /// Prefix to use for generated namespaces ////// Note that the NamespacePrefix is used as the only namespace for types in the same namespace /// as the default container, and as a prefix for the server-provided namespace for everything else. If /// this argument is null, the server-provided namespaces are used for all types. /// ///public IList GenerateCode(XmlReader sourceReader, TextWriter targetWriter, string namespacePrefix) { EntityUtil.CheckArgumentNull(sourceReader, "sourceReader"); EntityUtil.CheckArgumentNull(targetWriter, "targetWriter"); using (LazyTextWriterCreator target = new LazyTextWriterCreator(targetWriter)) { return GenerateCode(sourceReader, target, namespacePrefix); } // does not actually close the targetWriter - that is the caller's responsibility } private IList GenerateCode(XmlReader sourceReader, LazyTextWriterCreator target, string namespacePrefix) { NameTable nameTable = new NameTable(); const string DataWebMetadataNamespacePrefix = "m"; const string EdmxNamespacePrefix = "edmx"; const string DataWebMetadataNamespace = "http://schemas.microsoft.com/ado/2007/08/dataservices/metadata"; const string EdmV1Namespace = "http://schemas.microsoft.com/ado/2006/04/edm"; const string EdmV2Namespace = "http://schemas.microsoft.com/ado/2007/05/edm"; const string EdmxNamespace = "http://schemas.microsoft.com/ado/2007/06/edmx"; XmlNamespaceManager namespaceManager = new XmlNamespaceManager(nameTable); namespaceManager.AddNamespace(DataWebMetadataNamespacePrefix, DataWebMetadataNamespace); namespaceManager.AddNamespace(EdmxNamespacePrefix, EdmxNamespace); namespaceManager.AddNamespace("edmv1", EdmV1Namespace); namespaceManager.AddNamespace("edmv2", EdmV2Namespace); XmlDocument document = new XmlDocument(nameTable); document.Load(sourceReader); List readers = new List (); // Look for 1.0 schema elements XmlNodeList nodeList = document.SelectNodes("//edmv1:Schema", namespaceManager); for (int i = 0; i < nodeList.Count; i++) { readers.Add(nodeList[i].CreateNavigator().ReadSubtree()); } // Look for 1.1 schema elements nodeList = document.SelectNodes("//edmv2:Schema", namespaceManager); for (int i = 0; i < nodeList.Count; i++) { readers.Add(nodeList[i].CreateNavigator().ReadSubtree()); } List errors = new List (); EdmItemCollection itemCollection = new EdmItemCollection(readers); // generate code ClientApiGenerator generator = new ClientApiGenerator(null, itemCollection, this, errors, namespacePrefix); generator.GenerateCode(target); return errors; } #endregion #region Event Helpers /// /// Helper method that raises the TypeGenerated event /// /// The event arguments passed to the subscriber internal void RaiseTypeGeneratedEvent(TypeGeneratedEventArgs eventArgs) { if (this.OnTypeGenerated != null) { this.OnTypeGenerated(this, eventArgs); } } ////// Helper method that raises the PropertyGenerated event /// /// The event arguments passed to the subscriber internal void RaisePropertyGeneratedEvent(PropertyGeneratedEventArgs eventArgs) { if (this.OnPropertyGenerated != null) { this.OnPropertyGenerated(this, eventArgs); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Collections.Generic; using System.Data.Services.Design.Common; using System.Data.EntityModel; using System.Data.EntityModel.SchemaObjectModel; using System.Data.Metadata.Edm; using System.Diagnostics; using System.IO; using System.Xml; namespace System.Data.Common.Utils { internal static class EntityUtil { static internal void CheckArgumentNull(T value, string parameterName) where T : class { System.Data.Services.Design.EntityUtil.CheckArgumentNull (value, parameterName); } } } namespace System.Data.Services.Design { internal static class EntityUtil { static internal ArgumentException Argument(string error, string parameter) { ArgumentException e = new ArgumentException(error, parameter); return e; } static internal ArgumentNullException ArgumentNull(string parameter) { ArgumentNullException e = new ArgumentNullException(parameter); return e; } internal static void ThrowArgumentNullException(string parameterName) { throw ArgumentNull(parameterName); } static internal ArgumentException InvalidStringArgument(string parameterName) { return Argument(Strings.InvalidStringArgument(parameterName), parameterName); } static internal void CheckArgumentNull (T value, string parameterName) where T : class { if (null == value) { ThrowArgumentNullException(parameterName); } } static internal void CheckStringArgument(string value, string parameterName) { // Throw ArgumentNullException when string is null CheckArgumentNull(value, parameterName); // Throw ArgumentException when string is empty if (value.Length == 0) { throw InvalidStringArgument(parameterName); } } } /// /// Summary description for CodeGenerator. /// public sealed class EntityClassGenerator { #region Instance Fields LanguageOption _languageOption = LanguageOption.GenerateCSharpCode; EdmToObjectNamespaceMap _edmToObjectNamespaceMap = new EdmToObjectNamespaceMap(); #endregion #region Events ////// The event that is raised when a type is generated /// public event EventHandlerOnTypeGenerated; /// /// The event that is raised when a property is generated /// public event EventHandlerOnPropertyGenerated; #endregion #region Public Methods /// /// /// public EntityClassGenerator() { } ////// /// public EntityClassGenerator(LanguageOption languageOption) { _languageOption = EDesignUtil.CheckLanguageOptionArgument(languageOption, "languageOption"); } ////// Gets and Sets the Language to use for code generation. /// public LanguageOption LanguageOption { get { return _languageOption; } set { _languageOption = EDesignUtil.CheckLanguageOptionArgument(value, "value"); } } ////// Gets the map entries use to customize the namespace of .net types that are generated /// and referenced by the generated code /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly", MessageId = "Edm")] public EdmToObjectNamespaceMap EdmToObjectNamespaceMap { get { return _edmToObjectNamespaceMap; } } public IListGenerateCode(XmlReader sourceReader, string targetFilePath) { EntityUtil.CheckArgumentNull(sourceReader, "sourceReader"); EntityUtil.CheckStringArgument(targetFilePath, "targetPath"); using (LazyTextWriterCreator target = new LazyTextWriterCreator(targetFilePath)) { // we do want to close the file return GenerateCode(sourceReader, target, null); } } /// /// Generate code by reading an EDMX schema from an XmlReader and outputting the code into a TextWriter /// /// Reader with a EDMX schema in it /// Target writer for the generated code /// Prefix to use for generated namespaces ////// Note that the NamespacePrefix is used as the only namespace for types in the same namespace /// as the default container, and as a prefix for the server-provided namespace for everything else. If /// this argument is null, the server-provided namespaces are used for all types. /// ///public IList GenerateCode(XmlReader sourceReader, TextWriter targetWriter, string namespacePrefix) { EntityUtil.CheckArgumentNull(sourceReader, "sourceReader"); EntityUtil.CheckArgumentNull(targetWriter, "targetWriter"); using (LazyTextWriterCreator target = new LazyTextWriterCreator(targetWriter)) { return GenerateCode(sourceReader, target, namespacePrefix); } // does not actually close the targetWriter - that is the caller's responsibility } private IList GenerateCode(XmlReader sourceReader, LazyTextWriterCreator target, string namespacePrefix) { NameTable nameTable = new NameTable(); const string DataWebMetadataNamespacePrefix = "m"; const string EdmxNamespacePrefix = "edmx"; const string DataWebMetadataNamespace = "http://schemas.microsoft.com/ado/2007/08/dataservices/metadata"; const string EdmV1Namespace = "http://schemas.microsoft.com/ado/2006/04/edm"; const string EdmV2Namespace = "http://schemas.microsoft.com/ado/2007/05/edm"; const string EdmxNamespace = "http://schemas.microsoft.com/ado/2007/06/edmx"; XmlNamespaceManager namespaceManager = new XmlNamespaceManager(nameTable); namespaceManager.AddNamespace(DataWebMetadataNamespacePrefix, DataWebMetadataNamespace); namespaceManager.AddNamespace(EdmxNamespacePrefix, EdmxNamespace); namespaceManager.AddNamespace("edmv1", EdmV1Namespace); namespaceManager.AddNamespace("edmv2", EdmV2Namespace); XmlDocument document = new XmlDocument(nameTable); document.Load(sourceReader); List readers = new List (); // Look for 1.0 schema elements XmlNodeList nodeList = document.SelectNodes("//edmv1:Schema", namespaceManager); for (int i = 0; i < nodeList.Count; i++) { readers.Add(nodeList[i].CreateNavigator().ReadSubtree()); } // Look for 1.1 schema elements nodeList = document.SelectNodes("//edmv2:Schema", namespaceManager); for (int i = 0; i < nodeList.Count; i++) { readers.Add(nodeList[i].CreateNavigator().ReadSubtree()); } List errors = new List (); EdmItemCollection itemCollection = new EdmItemCollection(readers); // generate code ClientApiGenerator generator = new ClientApiGenerator(null, itemCollection, this, errors, namespacePrefix); generator.GenerateCode(target); return errors; } #endregion #region Event Helpers /// /// Helper method that raises the TypeGenerated event /// /// The event arguments passed to the subscriber internal void RaiseTypeGeneratedEvent(TypeGeneratedEventArgs eventArgs) { if (this.OnTypeGenerated != null) { this.OnTypeGenerated(this, eventArgs); } } ////// Helper method that raises the PropertyGenerated event /// /// The event arguments passed to the subscriber internal void RaisePropertyGeneratedEvent(PropertyGeneratedEventArgs eventArgs) { if (this.OnPropertyGenerated != null) { this.OnPropertyGenerated(this, eventArgs); } } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
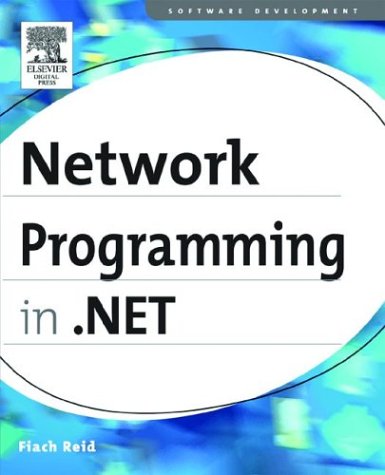
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PageCodeDomTreeGenerator.cs
- XmlIlVisitor.cs
- DataRowCollection.cs
- DateBoldEvent.cs
- PathData.cs
- CharacterString.cs
- DbDataRecord.cs
- PackWebRequestFactory.cs
- Property.cs
- XmlAttributeOverrides.cs
- XmlLinkedNode.cs
- WorkItem.cs
- DbBuffer.cs
- DateTimeConstantAttribute.cs
- GlobalizationAssembly.cs
- WindowsListViewSubItem.cs
- PeerInvitationResponse.cs
- ConsoleCancelEventArgs.cs
- DrawingGroup.cs
- UrlAuthFailedErrorFormatter.cs
- PenContext.cs
- WindowsMenu.cs
- ResourceDescriptionAttribute.cs
- FromRequest.cs
- XmlDataImplementation.cs
- SqlBuilder.cs
- EmptyCollection.cs
- WindowsGraphicsWrapper.cs
- ScrollChrome.cs
- MessageTransmitTraceRecord.cs
- AnnotationAuthorChangedEventArgs.cs
- IUnknownConstantAttribute.cs
- NullExtension.cs
- SecureStringHasher.cs
- OracleString.cs
- ResetableIterator.cs
- parserscommon.cs
- TrustSection.cs
- XmlComplianceUtil.cs
- SafeCoTaskMem.cs
- LocalTransaction.cs
- MetadataArtifactLoaderFile.cs
- NullableLongMinMaxAggregationOperator.cs
- DataSourceCacheDurationConverter.cs
- ListControlDataBindingHandler.cs
- SqlDataSourceConfigureSelectPanel.cs
- EqualityComparer.cs
- GridViewCellAutomationPeer.cs
- NameScope.cs
- OracleCommand.cs
- AuthenticationConfig.cs
- TreeNodeBindingDepthConverter.cs
- Command.cs
- _emptywebproxy.cs
- ProbeMatchesCD1.cs
- FormatConvertedBitmap.cs
- DateTime.cs
- Thumb.cs
- TcpDuplicateContext.cs
- Int16.cs
- CustomErrorsSectionWrapper.cs
- ListMarkerLine.cs
- SubMenuStyleCollectionEditor.cs
- MobileControlPersister.cs
- VisualStyleTypesAndProperties.cs
- Formatter.cs
- DataBinder.cs
- QilXmlWriter.cs
- XmlElementList.cs
- PageAdapter.cs
- MailAddressParser.cs
- XmlSchemaChoice.cs
- AsyncStreamReader.cs
- ipaddressinformationcollection.cs
- XPathScanner.cs
- BitmapFrame.cs
- DependentTransaction.cs
- CqlBlock.cs
- EventLogPermission.cs
- FactoryMaker.cs
- PrintDialog.cs
- TableRowGroup.cs
- RequestUriProcessor.cs
- ObjectHelper.cs
- ConfigurationManagerHelperFactory.cs
- CodeComment.cs
- BuildManagerHost.cs
- LayoutManager.cs
- Debugger.cs
- NativeMethods.cs
- VectorCollectionValueSerializer.cs
- Camera.cs
- XmlAttributeCollection.cs
- RoleService.cs
- MethodSignatureGenerator.cs
- QueueProcessor.cs
- FileSecurity.cs
- PropertyGrid.cs
- GridViewHeaderRowPresenter.cs
- RSAPKCS1SignatureFormatter.cs