Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / System / Windows / DurationConverter.cs / 1305600 / DurationConverter.cs
//------------------------------------------------------------------------------ // Microsoft Windows Client Platform // Copyright (c) Microsoft Corporation, 2004 // // File: DurationConverter.cs //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.ComponentModel.Design.Serialization; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Security; namespace System.Windows { ////// Provides a type converter to convert Duration to and from other representations. /// public class DurationConverter : TypeConverter { ////// CanConvertFrom - Returns whether or not this class can convert from a given type /// ///public override bool CanConvertFrom(ITypeDescriptorContext td, Type t) { if (t == typeof(string)) { return true; } else { return false; } } /// /// TypeConverter method override. /// /// ITypeDescriptorContext /// Type to convert to ///true if conversion is possible ///public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if ( destinationType == typeof(InstanceDescriptor) || destinationType == typeof(string)) { return true; } else { return false; } } /// /// ConvertFrom /// ///public override object ConvertFrom( ITypeDescriptorContext td, CultureInfo cultureInfo, object value) { string stringValue = value as string; // Override the converter for sentinel values if (stringValue != null) { stringValue = stringValue.Trim(); if (stringValue == "Automatic") { return Duration.Automatic; } else if (stringValue == "Forever") { return Duration.Forever; } } TimeSpan duration = TimeSpan.Zero; if(_timeSpanConverter == null) { _timeSpanConverter = new TimeSpanConverter(); } duration = (TimeSpan)_timeSpanConverter.ConvertFrom(td, cultureInfo, value); return new Duration(duration); } /// /// TypeConverter method implementation. /// /// ITypeDescriptorContext /// current culture (see CLR specs) /// value to convert from /// Type to convert to ///converted value ////// /// Critical: calls InstanceDescriptor ctor which LinkDemands /// PublicOK: can only make an InstanceDescriptor for Duration, not an arbitrary class /// [SecurityCritical] public override object ConvertTo( ITypeDescriptorContext context, CultureInfo cultureInfo, object value, Type destinationType) { if (destinationType != null && value is Duration) { Duration durationValue = (Duration)value; if (destinationType == typeof(InstanceDescriptor)) { MemberInfo mi; if (durationValue.HasTimeSpan) { mi = typeof(Duration).GetConstructor(new Type[] { typeof(TimeSpan) }); return new InstanceDescriptor(mi, new object[] { durationValue.TimeSpan }); } else if (durationValue == Duration.Forever) { mi = typeof(Duration).GetProperty("Forever"); return new InstanceDescriptor(mi, null); } else { Debug.Assert(durationValue == Duration.Automatic); // Only other legal duration type mi = typeof(Duration).GetProperty("Automatic"); return new InstanceDescriptor(mi, null); } } else if (destinationType == typeof(string)) { return durationValue.ToString(); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, cultureInfo, value, destinationType); } private static TimeSpanConverter _timeSpanConverter; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
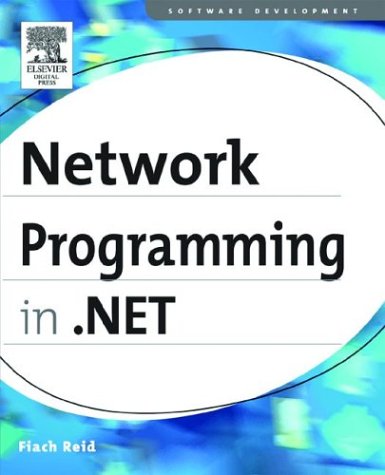
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ColorAnimation.cs
- ClientSponsor.cs
- InvalidateEvent.cs
- SetterBaseCollection.cs
- MulticastIPAddressInformationCollection.cs
- CommonProperties.cs
- AssociationTypeEmitter.cs
- ThreadPool.cs
- SiteMapPath.cs
- FontStretch.cs
- XmlSchemaSimpleTypeUnion.cs
- GZipStream.cs
- TrueReadOnlyCollection.cs
- RedBlackList.cs
- CompoundFileDeflateTransform.cs
- DateTimeParse.cs
- Timer.cs
- ArgIterator.cs
- DataGridViewCellConverter.cs
- CqlGenerator.cs
- AutomationElement.cs
- XmlDownloadManager.cs
- PropertyDescriptorComparer.cs
- UriExt.cs
- TextEditorDragDrop.cs
- ConfigurationStrings.cs
- ThreadAbortException.cs
- VariantWrapper.cs
- AsyncOperationManager.cs
- StrokeNodeOperations.cs
- MarshalByRefObject.cs
- XmlObjectSerializer.cs
- LookupNode.cs
- DataGridViewCellStyleChangedEventArgs.cs
- LinkConverter.cs
- Environment.cs
- RemotingAttributes.cs
- GridItem.cs
- EntitySqlQueryCacheEntry.cs
- ClientProtocol.cs
- GeometryCombineModeValidation.cs
- SpellerStatusTable.cs
- Globals.cs
- SourceLineInfo.cs
- LinkConverter.cs
- ConversionHelper.cs
- DataSourceNameHandler.cs
- MappedMetaModel.cs
- MissingMethodException.cs
- TypeConverter.cs
- Visual3DCollection.cs
- ToolStripPanelSelectionGlyph.cs
- ManifestResourceInfo.cs
- DBCSCodePageEncoding.cs
- ParentControlDesigner.cs
- CodeBinaryOperatorExpression.cs
- CorrelationValidator.cs
- RemoteTokenFactory.cs
- SiteMapHierarchicalDataSourceView.cs
- EventLogConfiguration.cs
- MiniMapControl.xaml.cs
- TemplatedWizardStep.cs
- GridViewItemAutomationPeer.cs
- XmlCompatibilityReader.cs
- DbConnectionFactory.cs
- URLMembershipCondition.cs
- WebPartConnectionsCancelEventArgs.cs
- ObjectStorage.cs
- TextAction.cs
- DocComment.cs
- RoutedCommand.cs
- InternalTypeHelper.cs
- MaterialGroup.cs
- EncryptionUtility.cs
- MDIWindowDialog.cs
- SelfIssuedAuthRSAPKCS1SignatureFormatter.cs
- RelationshipEnd.cs
- CodeAttributeArgument.cs
- SlipBehavior.cs
- Semaphore.cs
- DetailsView.cs
- FileDialog.cs
- DataGridCellsPresenter.cs
- PersonalizationStateInfo.cs
- HwndSubclass.cs
- PersonalizableAttribute.cs
- BrowserCapabilitiesFactoryBase.cs
- AvTraceFormat.cs
- BatchStream.cs
- RotateTransform3D.cs
- CheckBoxRenderer.cs
- ListViewContainer.cs
- SchemaNames.cs
- SharedMemory.cs
- NCryptNative.cs
- CapabilitiesSection.cs
- RemoteAsymmetricSignatureFormatter.cs
- AsymmetricAlgorithm.cs
- ContentIterators.cs
- UserInitiatedNavigationPermission.cs