Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Core / System / Windows / FontStretch.cs / 1 / FontStretch.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: FontStretch structure. // // History: // 01/25/2005 [....] - Converted FontStretch from enum to a value type and moved it to a separate file. // //--------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using MS.Internal; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; namespace System.Windows { ////// FontStretch structure describes relative change from the normal aspect ratio /// as specified by a font designer for the glyphs in a font. /// [TypeConverter(typeof(FontStretchConverter))] [Localizability(LocalizationCategory.None)] public struct FontStretch : IFormattable { internal FontStretch(int stretch) { Debug.Assert(1 <= stretch && stretch <= 9); // We want the default zero value of new FontStretch() to correspond to FontStretches.Normal. // Therefore, the _stretch value is shifted by 5 relative to the OpenType stretch value. _stretch = stretch - 5; } ////// Creates a new FontStretch object that corresponds to the OpenType usWidthClass value. /// /// An integer value between 1 and 9 that corresponds /// to the usWidthClass definition in the OpenType specification. ///A new FontStretch object that corresponds to the stretchValue parameter. // Important note: when changing this method signature please make sure to update FontStretchConverter accordingly. public static FontStretch FromOpenTypeStretch(int stretchValue) { if (stretchValue < 1 || stretchValue > 9) throw new ArgumentOutOfRangeException("stretchValue", SR.Get(SRID.ParameterMustBeBetween, 1, 9)); return new FontStretch(stretchValue); } ////// Obtains OpenType usWidthClass value that corresponds to the FontStretch object. /// ///An integer value between 1 and 9 that corresponds /// to the usWidthClass definition in the OpenType specification. // Important note: when changing this method signature please make sure to update FontStretchConverter accordingly. public int ToOpenTypeStretch() { Debug.Assert(1 <= RealStretch && RealStretch <= 9); return RealStretch; } ////// Compares two font stretch values and returns an indication of their relative values. /// /// First object to compare. /// Second object to compare. ///A 32-bit signed integer indicating the lexical relationship between the two comparands. /// When the return value is less than zero this means that left is less than right. /// When the return value is zero this means that left is equal to right. /// When the return value is greater than zero this means that left is greater than right. /// public static int Compare(FontStretch left, FontStretch right) { return left._stretch - right._stretch; } ////// Checks whether a font stretch is less than another. /// /// First object to compare. /// Second object to compare. ///True if left is less than right, false otherwise. public static bool operator<(FontStretch left, FontStretch right) { return Compare(left, right) < 0; } ////// Checks whether a font stretch is less or equal than another. /// /// First object to compare. /// Second object to compare. ///True if left is less or equal than right, false otherwise. public static bool operator<=(FontStretch left, FontStretch right) { return Compare(left, right) <= 0; } ////// Checks whether a font stretch is greater than another. /// /// First object to compare. /// Second object to compare. ///True if left is greater than right, false otherwise. public static bool operator>(FontStretch left, FontStretch right) { return Compare(left, right) > 0; } ////// Checks whether a font stretch is greater or equal than another. /// /// First object to compare. /// Second object to compare. ///True if left is greater or equal than right, false otherwise. public static bool operator>=(FontStretch left, FontStretch right) { return Compare(left, right) >= 0; } ////// Checks whether two font stretch objects are equal. /// /// First object to compare. /// Second object to compare. ///Returns true when the font stretch values are equal for both objects, /// and false otherwise. public static bool operator==(FontStretch left, FontStretch right) { return Compare(left, right) == 0; } ////// Checks whether two font stretch objects are not equal. /// /// First object to compare. /// Second object to compare. ///Returns false when the font stretch values are equal for both objects, /// and true otherwise. public static bool operator!=(FontStretch left, FontStretch right) { return !(left == right); } ////// Checks whether the object is equal to another FontStretch object. /// /// FontStretch object to compare with. ///Returns true when the object is equal to the input object, /// and false otherwise. public bool Equals(FontStretch obj) { return this == obj; } ////// Checks whether an object is equal to another character hit object. /// /// FontStretch object to compare with. ///Returns true when the object is equal to the input object, /// and false otherwise. public override bool Equals(object obj) { if (!(obj is FontStretch)) return false; return this == (FontStretch)obj; } ////// Compute hash code for this object. /// ///A 32-bit signed integer hash code. public override int GetHashCode() { return RealStretch; } ////// Creates a string representation of this object based on the current culture. /// ////// A string representation of this object. /// public override string ToString() { // Delegate to the internal method which implements all ToString calls. return ConvertToString(null, null); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// string IFormattable.ToString(string format, IFormatProvider provider) { // Delegate to the internal method which implements all ToString calls. return ConvertToString(format, provider); } ////// Creates a string representation of this object based on the format string /// and IFormatProvider passed in. /// If the provider is null, the CurrentCulture is used. /// See the documentation for IFormattable for more information. /// ////// A string representation of this object. /// private string ConvertToString(string format, IFormatProvider provider) { string convertedValue; if (!FontStretches.FontStretchToString(RealStretch, out convertedValue)) { // This can happen only if _stretch member is corrupted. Invariant.Assert(false); } return convertedValue; } ////// We want the default zero value of new FontStretch() to correspond to FontStretches.Normal. /// Therefore, _stretch value is shifted by 5 relative to the OpenType stretch value. /// private int RealStretch { get { return _stretch + 5; } } private int _stretch; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
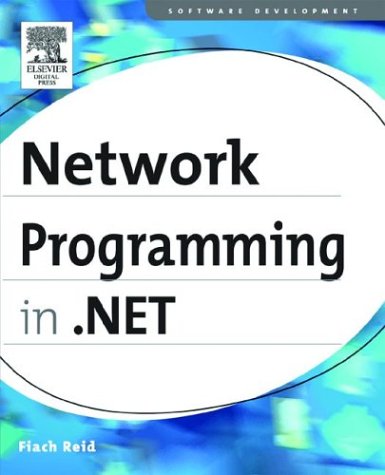
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DiffuseMaterial.cs
- StorageEntityContainerMapping.cs
- ToolStripPanelCell.cs
- CacheHelper.cs
- VariantWrapper.cs
- TableProviderWrapper.cs
- InputScopeAttribute.cs
- ObjectConverter.cs
- ParameterCollection.cs
- XslException.cs
- XmlSchemaInfo.cs
- FixedSOMTableCell.cs
- diagnosticsswitches.cs
- _ProxyChain.cs
- KeyFrames.cs
- FlowDocumentScrollViewer.cs
- TextParagraphCache.cs
- UpdateProgress.cs
- ToolStripContainer.cs
- IndentedWriter.cs
- ChangeTracker.cs
- FullTextState.cs
- PlacementWorkspace.cs
- ManagementException.cs
- InstanceData.cs
- ServicesUtilities.cs
- HttpApplication.cs
- DocumentPageViewAutomationPeer.cs
- MsdtcWrapper.cs
- FontEmbeddingManager.cs
- SelectManyQueryOperator.cs
- InstanceCreationEditor.cs
- CdpEqualityComparer.cs
- GenericTextProperties.cs
- Rotation3D.cs
- XmlSchemaNotation.cs
- FlowDocument.cs
- SQLDateTimeStorage.cs
- BulletDecorator.cs
- WriteTimeStream.cs
- SqlServer2KCompatibilityCheck.cs
- XmlSchemaGroup.cs
- BitConverter.cs
- ServiceDescription.cs
- StructuralObject.cs
- VisualProxy.cs
- CapabilitiesRule.cs
- DLinqColumnProvider.cs
- SchemaMerger.cs
- UniqueEventHelper.cs
- XmlQueryType.cs
- ComContractElementCollection.cs
- StdRegProviderWrapper.cs
- AlgoModule.cs
- EventProvider.cs
- MetadataCacheItem.cs
- SHA256Managed.cs
- Size3DValueSerializer.cs
- RefExpr.cs
- WorkflowOperationFault.cs
- JpegBitmapEncoder.cs
- XmlNamespaceMapping.cs
- ResponseBodyWriter.cs
- BaseParaClient.cs
- HuffmanTree.cs
- InputEventArgs.cs
- HtmlButton.cs
- _FtpControlStream.cs
- SmtpNtlmAuthenticationModule.cs
- SecurityException.cs
- PrivateFontCollection.cs
- SByteStorage.cs
- Exceptions.cs
- DocumentViewerConstants.cs
- ThreadAttributes.cs
- PropertyCondition.cs
- DiscoveryEndpoint.cs
- SqlMethodAttribute.cs
- DataGridTableCollection.cs
- Trace.cs
- KnownTypeHelper.cs
- Win32.cs
- MachinePropertyVariants.cs
- StateInitialization.cs
- CodeNamespace.cs
- XMLUtil.cs
- DateBoldEvent.cs
- Matrix.cs
- WriteStateInfoBase.cs
- WebPartHelpVerb.cs
- SafeHandle.cs
- PreservationFileReader.cs
- DataSourceView.cs
- ZipIOExtraFieldElement.cs
- XmlBinaryWriterSession.cs
- Font.cs
- RelativeSource.cs
- ArrayConverter.cs
- Monitor.cs
- SafeIUnknown.cs