Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / TextFormatting / GenericTextProperties.cs / 1305600 / GenericTextProperties.cs
//+------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: GenericTextProperties.cs // // Contents: Generic implementation of TextFormatter abstract classes // // Created: 3-9-2003 Worachai Chaoweeraprasit (wchao) // //----------------------------------------------------------------------- using System; using System.Diagnostics; using System.Windows; using System.Windows.Media; using System.Windows.Media.TextFormatting; using System.Globalization; namespace MS.Internal.TextFormatting { ////// Generic implementation of TextRunProperties /// internal sealed class GenericTextRunProperties : TextRunProperties { ////// Constructing TextRunProperties /// /// typeface /// text size /// text size for Truetype hinting program /// text culture info /// TextDecorations /// text foreground brush /// highlight background brush /// text vertical alignment to its container /// number substitution behavior to apply to the text; can be null, /// in which case the default number substitution method for the text culture is used public GenericTextRunProperties( Typeface typeface, double size, double hintingSize, TextDecorationCollection textDecorations, Brush foregroundBrush, Brush backgroundBrush, BaselineAlignment baselineAlignment, CultureInfo culture, NumberSubstitution substitution ) { _typeface = typeface; _emSize = size; _emHintingSize = hintingSize; _textDecorations = textDecorations; _foregroundBrush = foregroundBrush; _backgroundBrush = backgroundBrush; _baselineAlignment = baselineAlignment; _culture = culture; _numberSubstitution = substitution; } ////// Hash code generator /// ///TextRunProperties hash code public override int GetHashCode() { return _typeface.GetHashCode() ^ _emSize.GetHashCode() ^ _emHintingSize.GetHashCode() ^ ((_foregroundBrush == null) ? 0 : _foregroundBrush.GetHashCode()) ^ ((_backgroundBrush == null) ? 0 : _backgroundBrush.GetHashCode()) ^ ((_textDecorations == null) ? 0 : _textDecorations.GetHashCode()) ^ ((int)_baselineAlignment << 3) ^ ((int)_culture.GetHashCode() << 6) ^ ((_numberSubstitution == null) ? 0 : _numberSubstitution.GetHashCode()); } ////// Equality check /// ///objects equals public override bool Equals(object o) { if ((o == null) || !(o is TextRunProperties)) { return false; } TextRunProperties textRunProperties = (TextRunProperties)o; return _emSize == textRunProperties.FontRenderingEmSize && _emHintingSize == textRunProperties.FontHintingEmSize && _culture == textRunProperties.CultureInfo && _typeface.Equals(textRunProperties.Typeface) && ((_textDecorations == null) ? textRunProperties.TextDecorations == null : _textDecorations.ValueEquals(textRunProperties.TextDecorations)) && _baselineAlignment == textRunProperties.BaselineAlignment && ((_foregroundBrush == null) ? (textRunProperties.ForegroundBrush == null) : (_foregroundBrush.Equals(textRunProperties.ForegroundBrush))) && ((_backgroundBrush == null) ? (textRunProperties.BackgroundBrush == null) : (_backgroundBrush.Equals(textRunProperties.BackgroundBrush))) && ((_numberSubstitution == null) ? (textRunProperties.NumberSubstitution == null) : (_numberSubstitution.Equals(textRunProperties.NumberSubstitution))); } ////// Run typeface /// public override Typeface Typeface { get { return _typeface; } } ////// Em size of font used to format and display text /// public override double FontRenderingEmSize { get { return _emSize; } } ////// Em size of font to determine subtle change in font hinting default value is 12pt /// public override double FontHintingEmSize { get { return _emHintingSize; } } ////// Run text decoration /// public override TextDecorationCollection TextDecorations { get { return _textDecorations; } } ////// Run text foreground brush /// public override Brush ForegroundBrush { get { return _foregroundBrush; } } ////// Run text highlight background brush /// public override Brush BackgroundBrush { get { return _backgroundBrush; } } ////// Run vertical box alignment /// public override BaselineAlignment BaselineAlignment { get { return _baselineAlignment; } } ////// Run text Culture Info /// public override CultureInfo CultureInfo { get { return _culture; } } ////// Run typography properties /// public override TextRunTypographyProperties TypographyProperties { get{return null;} } ////// Run Text effects /// public override TextEffectCollection TextEffects { get { return null; } } ////// Number substitution /// public override NumberSubstitution NumberSubstitution { get { return _numberSubstitution; } } private Typeface _typeface; private double _emSize; private double _emHintingSize; private TextDecorationCollection _textDecorations; private Brush _foregroundBrush; private Brush _backgroundBrush; private BaselineAlignment _baselineAlignment; private CultureInfo _culture; private NumberSubstitution _numberSubstitution; } ////// Generic implementation of TextParagraphProperties /// internal sealed class GenericTextParagraphProperties : TextParagraphProperties { ////// Constructing TextParagraphProperties /// /// text flow direction /// logical horizontal alignment /// true if the paragraph is the first line in the paragraph /// true if the line is always collapsible /// default paragraph's default run properties /// text wrap option /// Paragraph line height /// line indentation public GenericTextParagraphProperties( FlowDirection flowDirection, TextAlignment textAlignment, bool firstLineInParagraph, bool alwaysCollapsible, TextRunProperties defaultTextRunProperties, TextWrapping textWrap, double lineHeight, double indent ) { _flowDirection = flowDirection; _textAlignment = textAlignment; _firstLineInParagraph = firstLineInParagraph; _alwaysCollapsible = alwaysCollapsible; _defaultTextRunProperties = defaultTextRunProperties; _textWrap = textWrap; _lineHeight = lineHeight; _indent = indent; } ////// Constructing TextParagraphProperties from another one /// /// source line props public GenericTextParagraphProperties(TextParagraphProperties textParagraphProperties) { _flowDirection = textParagraphProperties.FlowDirection; _defaultTextRunProperties = textParagraphProperties.DefaultTextRunProperties; _textAlignment = textParagraphProperties.TextAlignment; _lineHeight = textParagraphProperties.LineHeight; _firstLineInParagraph = textParagraphProperties.FirstLineInParagraph; _alwaysCollapsible = textParagraphProperties.AlwaysCollapsible; _textWrap = textParagraphProperties.TextWrapping; _indent = textParagraphProperties.Indent; } ////// This property specifies whether the primary text advance /// direction shall be left-to-right, right-to-left, or top-to-bottom. /// public override FlowDirection FlowDirection { get { return _flowDirection; } } ////// This property describes how inline content of a block is aligned. /// public override TextAlignment TextAlignment { get { return _textAlignment; } } ////// Paragraph's line height /// public override double LineHeight { get { return _lineHeight; } } ////// Indicates the first line of the paragraph. /// public override bool FirstLineInParagraph { get { return _firstLineInParagraph; } } ////// If true, the formatted line may always be collapsed. If false (the default), /// only lines that overflow the paragraph width are collapsed. /// public override bool AlwaysCollapsible { get { return _alwaysCollapsible; } } ////// Paragraph's default run properties /// public override TextRunProperties DefaultTextRunProperties { get { return _defaultTextRunProperties; } } ////// This property controls whether or not text wraps when it reaches the flow edge /// of its containing block box /// public override TextWrapping TextWrapping { get { return _textWrap; } } ////// This property specifies marker characteristics of the first line in paragraph /// public override TextMarkerProperties TextMarkerProperties { get { return null; } } ////// Line indentation /// public override double Indent { get { return _indent; } } ////// Set text flow direction /// internal void SetFlowDirection(FlowDirection flowDirection) { _flowDirection = flowDirection; } ////// Set text alignment /// internal void SetTextAlignment(TextAlignment textAlignment) { _textAlignment = textAlignment; } ////// Set line height /// internal void SetLineHeight(double lineHeight) { _lineHeight = lineHeight; } ////// Set text wrap /// internal void SetTextWrapping(TextWrapping textWrap) { _textWrap = textWrap; } private FlowDirection _flowDirection; private TextAlignment _textAlignment; private bool _firstLineInParagraph; private bool _alwaysCollapsible; private TextRunProperties _defaultTextRunProperties; private TextWrapping _textWrap; private double _indent; private double _lineHeight; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
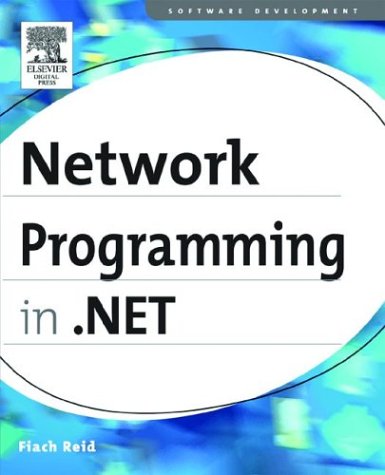
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CornerRadiusConverter.cs
- CustomCategoryAttribute.cs
- GroupItem.cs
- NativeMethods.cs
- ConnectAlgorithms.cs
- WebPartCollection.cs
- SafeProcessHandle.cs
- PackWebResponse.cs
- DbSourceParameterCollection.cs
- InvokeMethodActivity.cs
- IPPacketInformation.cs
- BitmapCodecInfo.cs
- EntryIndex.cs
- SqlUserDefinedAggregateAttribute.cs
- DataListItemCollection.cs
- ButtonBase.cs
- WebAdminConfigurationHelper.cs
- InfoCardKeyedHashAlgorithm.cs
- PerfCounters.cs
- LayoutTableCell.cs
- SessionEndingCancelEventArgs.cs
- KeysConverter.cs
- LicenseException.cs
- WithParamAction.cs
- ValidatorCollection.cs
- InfoCardTrace.cs
- UrlMappingCollection.cs
- SQLUtility.cs
- RMEnrollmentPage2.cs
- ExpressionTable.cs
- PropertyBuilder.cs
- FactoryGenerator.cs
- TemplateControl.cs
- ForEach.cs
- ImageInfo.cs
- SiteMapNodeCollection.cs
- ProgressBarHighlightConverter.cs
- IDQuery.cs
- HttpModuleCollection.cs
- WebPageTraceListener.cs
- WebPartConnectionsCloseVerb.cs
- ObjectDataSource.cs
- SettingsProviderCollection.cs
- OutputWindow.cs
- ListViewTableCell.cs
- ListItemCollection.cs
- AttachedPropertyBrowsableAttribute.cs
- PartialTrustHelpers.cs
- coordinatorscratchpad.cs
- IIS7UserPrincipal.cs
- ConstructorExpr.cs
- StreamGeometryContext.cs
- StaticResourceExtension.cs
- CompositeControl.cs
- TextSearch.cs
- StopStoryboard.cs
- RestHandler.cs
- EdmProperty.cs
- DetailsViewRow.cs
- DataGridViewTopLeftHeaderCell.cs
- ThumbButtonInfo.cs
- EntitySqlQueryState.cs
- EmptyArray.cs
- Atom10ItemFormatter.cs
- VideoDrawing.cs
- DocumentViewerBase.cs
- ArgumentNullException.cs
- XsdDateTime.cs
- XmlUTF8TextReader.cs
- ElapsedEventArgs.cs
- MultiByteCodec.cs
- RectValueSerializer.cs
- OdbcCommandBuilder.cs
- XsdDateTime.cs
- BamlLocalizableResource.cs
- EditorPartCollection.cs
- IndexOutOfRangeException.cs
- Focus.cs
- IsolatedStorageFile.cs
- EditorZone.cs
- MemberDomainMap.cs
- DataTableCollection.cs
- NameTable.cs
- FileSystemInfo.cs
- BitmapSource.cs
- TypeCodeDomSerializer.cs
- ResourceKey.cs
- ReaderWriterLockWrapper.cs
- XhtmlBasicFormAdapter.cs
- WebScriptServiceHostFactory.cs
- Emitter.cs
- HyperLinkColumn.cs
- ConnectionStringsExpressionBuilder.cs
- InvalidOperationException.cs
- SmtpFailedRecipientsException.cs
- SHA1CryptoServiceProvider.cs
- XmlName.cs
- AsyncStreamReader.cs
- HighlightComponent.cs
- NamespaceTable.cs