Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Themes / Aero / Microsoft / Windows / Themes / ProgressBarHighlightConverter.cs / 1305600 / ProgressBarHighlightConverter.cs
//---------------------------------------------------------------------------- // // Copyright (C) by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Globalization; using System.Threading; using System.Windows; using System.Windows.Controls.Primitives; using System.Windows.Data; using System.Windows.Media; using System.Windows.Media.Animation; namespace Microsoft.Windows.Themes { ////// The ProgressBarHighlightConverter class /// public class ProgressBarHighlightConverter : IMultiValueConverter { ////// Creates the brush for the ProgressBar /// /// ForegroundBrush, IsIndeterminate, Width, Height /// /// /// ///Brush for the ProgressBar public object Convert(object[] values, Type targetType, object parameter, CultureInfo culture) { // // Parameter Validation // Type doubleType = typeof(double); if (values == null || (values.Length != 3) || (values[0] == null) || (values[1] == null) || (values[2] == null) || !typeof(Brush).IsAssignableFrom(values[0].GetType()) || !doubleType.IsAssignableFrom(values[1].GetType()) || !doubleType.IsAssignableFrom(values[2].GetType())) { return null; } // // Conversion // Brush brush = (Brush)values[0]; double width = (double)values[1]; double height = (double)values[2]; // if an invalid height, return a null brush if (width <= 0.0 || Double.IsInfinity(width) || Double.IsNaN(width) || height <= 0.0 || Double.IsInfinity(height) || Double.IsNaN(height) ) { return null; } DrawingBrush newBrush = new DrawingBrush(); // Create a Drawing Brush that is 2x longer than progress bar track // // +-------------+.............. // | highlight | empty : // +-------------+.............: // // This brush will animate to the right. double twiceWidth = width * 2.0; // Set the viewport and viewbox to the 2*size of the progress region newBrush.Viewport = newBrush.Viewbox = new Rect(-width, 0, twiceWidth, height); newBrush.ViewportUnits = newBrush.ViewboxUnits = BrushMappingMode.Absolute; newBrush.TileMode = TileMode.None; newBrush.Stretch = Stretch.None; DrawingGroup myDrawing = new DrawingGroup(); DrawingContext myDrawingContext = myDrawing.Open(); // Draw the highlight myDrawingContext.DrawRectangle(brush, null, new Rect(-width, 0, width, height)); // Animate the Translation TimeSpan translateTime = TimeSpan.FromSeconds(twiceWidth / 200.0); // travel at 200px /second TimeSpan pauseTime = TimeSpan.FromSeconds(1.0); // pause 1 second between animations DoubleAnimationUsingKeyFrames animation = new DoubleAnimationUsingKeyFrames(); animation.BeginTime = TimeSpan.Zero; animation.Duration = new Duration(translateTime + pauseTime); animation.RepeatBehavior = RepeatBehavior.Forever; animation.KeyFrames.Add(new LinearDoubleKeyFrame(twiceWidth, translateTime)); TranslateTransform translation = new TranslateTransform(); // Set the animation to the XProperty translation.BeginAnimation(TranslateTransform.XProperty, animation); // Set the animated translation on the brush newBrush.Transform = translation; myDrawingContext.Close(); newBrush.Drawing = myDrawing; return newBrush; } ////// Not Supported /// /// value, as produced by target /// target types /// converter parameter /// culture information ///Nothing public object[] ConvertBack(object value, Type[] targetTypes, object parameter, CultureInfo culture) { return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
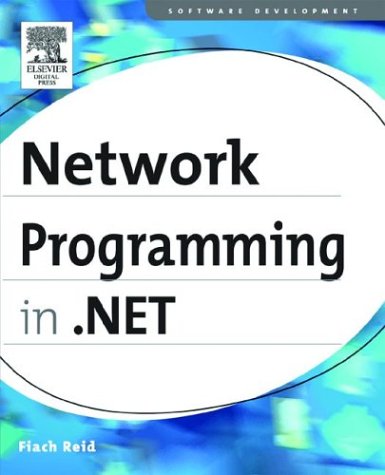
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MemberInfoSerializationHolder.cs
- HeaderLabel.cs
- EmbeddedMailObjectsCollection.cs
- DetailsViewDeletedEventArgs.cs
- TryCatchDesigner.xaml.cs
- SqlMethodAttribute.cs
- StorageScalarPropertyMapping.cs
- PathSegment.cs
- AuthenticatedStream.cs
- JobStaple.cs
- SemanticResolver.cs
- HtmlUtf8RawTextWriter.cs
- UIElementIsland.cs
- RowType.cs
- HwndTarget.cs
- XmlSchemaObject.cs
- SafeRightsManagementSessionHandle.cs
- EditorPartCollection.cs
- XmlQualifiedName.cs
- EntityDataSourceReferenceGroup.cs
- TreeBuilderXamlTranslator.cs
- EventWaitHandleSecurity.cs
- ExpressionNormalizer.cs
- FileSystemEventArgs.cs
- DbCommandDefinition.cs
- HttpModulesSection.cs
- FileAuthorizationModule.cs
- DataControlFieldHeaderCell.cs
- HtmlLink.cs
- DBNull.cs
- AttachedPropertyMethodSelector.cs
- CodeExpressionCollection.cs
- ElementAtQueryOperator.cs
- Vector3DValueSerializer.cs
- TextDecoration.cs
- Win32SafeHandles.cs
- BinaryFormatterSinks.cs
- ParallelTimeline.cs
- FlowLayoutPanel.cs
- BufferedGraphicsContext.cs
- entityreference_tresulttype.cs
- ApplicationFileParser.cs
- DependencyPropertyKind.cs
- WhitespaceReader.cs
- DataGridViewHitTestInfo.cs
- SettingsProviderCollection.cs
- ContextMenuStripGroup.cs
- SafeReversePInvokeHandle.cs
- ExpressionReplacer.cs
- RouteUrlExpressionBuilder.cs
- StringHandle.cs
- AuthenticationConfig.cs
- Stream.cs
- GroupBox.cs
- EnumType.cs
- CryptoApi.cs
- DataGridViewRow.cs
- ToolboxComponentsCreatedEventArgs.cs
- SizeAnimationClockResource.cs
- SqlExpander.cs
- mongolianshape.cs
- TimeSpanMinutesOrInfiniteConverter.cs
- IntSecurity.cs
- AffineTransform3D.cs
- XmlChildEnumerator.cs
- IntellisenseTextBox.designer.cs
- CommandEventArgs.cs
- RequiredFieldValidator.cs
- RoutedPropertyChangedEventArgs.cs
- XmlWellformedWriter.cs
- Rotation3D.cs
- DataSourceCache.cs
- ProgressBarAutomationPeer.cs
- FlowDocumentPageViewerAutomationPeer.cs
- ElementMarkupObject.cs
- ParseNumbers.cs
- TrackBarRenderer.cs
- CodeAttributeArgumentCollection.cs
- AuthenticationSection.cs
- COM2IProvidePropertyBuilderHandler.cs
- ApplicationProxyInternal.cs
- StreamedWorkflowDefinitionContext.cs
- AuthenticationManager.cs
- ObjectComplexPropertyMapping.cs
- DynamicValidatorEventArgs.cs
- SqlUDTStorage.cs
- DockProviderWrapper.cs
- Material.cs
- CookieProtection.cs
- Win32Native.cs
- WebPartTransformerAttribute.cs
- TemplateBamlTreeBuilder.cs
- ByteBufferPool.cs
- BaseCollection.cs
- MarshalDirectiveException.cs
- PrinterUnitConvert.cs
- X509CertificateStore.cs
- QilLoop.cs
- LogicalExpr.cs
- HttpPostedFile.cs